Python pathlib module provides an object-oriented approach to work with files and directories. The pathlib module has classes to work with Unix as well as Windows environments. The best part is that we don’t have to worry about the underlying operating system, the pathlib module takes care of using the appropriate class based on the operating system.
Python pathlib模块提供了一种面向对象的方法来处理文件和目录。 pathlib模块具有用于Unix和Windows环境的类。 最好的部分是,我们不必担心底层操作系统,pathlib模块负责根据操作系统使用适当的类。
Python pathlib路径类 (Python pathlib Path Class)
Path is the most important class in the pathlib module. This is the entry point of all the functions provided by pathlib module. It takes care of instantiating the concrete path implementation based on the operating system and make the code platform-independent.
路径是pathlib模块中最重要的类。 这是pathlib模块提供的所有功能的切入点。 它负责实例化基于操作系统的具体路径实现,并使代码独立于平台。
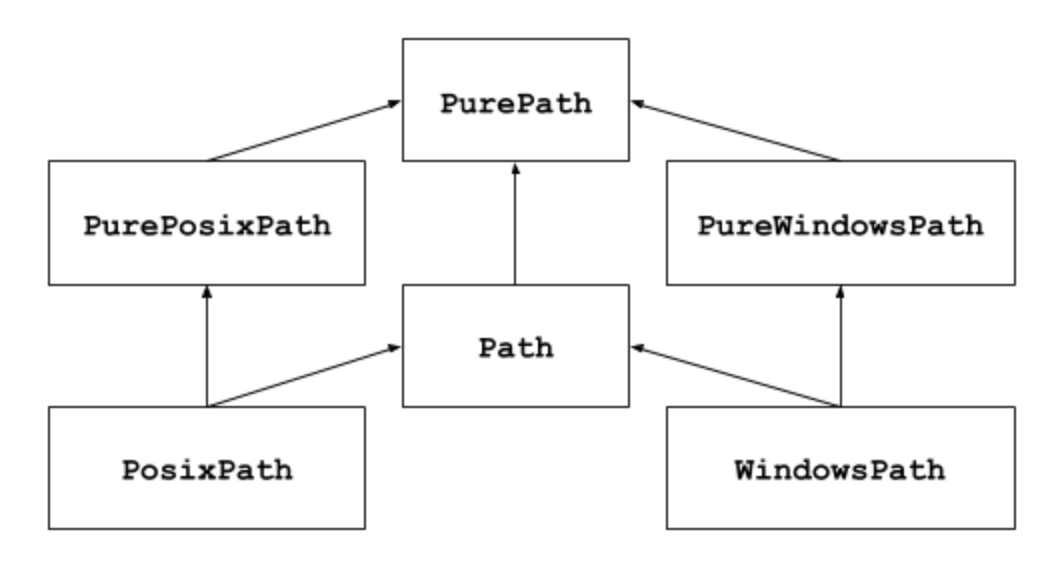
Python pathlib Module
Python pathlib模块
Let’s look into some examples of using pathlib module.
让我们看一些使用pathlib模块的示例。
1.列出目录中的子目录和文件 (1. List Subdirectories and Files inside a Directory)
We can use Path iterdir() function to iterate over the files in a directory. Then we can use is_dir() function to differentiate between a file and a directory.
我们可以使用Path iterdir()函数来遍历目录中的文件。 然后,我们可以使用is_dir()函数来区分文件和目录。
from pathlib import Path
# list subdirectories and files inside a directory
path = Path("/Users/pankaj/temp")
subdirs = []
files = []
for x in path.iterdir(): # iterate over the files in the path
if x.is_dir(): # condition to check if the file is a directory
subdirs.append(x)
else:
files.append(x)
print(subdirs)
print(files)
Output:
输出:
[PosixPath('/Users/pankaj/temp/spring-webflow-samples'), PosixPath('/Users/pankaj/temp/image-optim'), PosixPath('/Users/pankaj/temp/jersey2-example')]
[PosixPath('/Users/pankaj/temp/test123.py'), PosixPath('/Users/pankaj/temp/.txt'), PosixPath('/Users/pankaj/temp/xyz.txt'), PosixPath('/Users/pankaj/temp/.DS_Store'), PosixPath('/Users/pankaj/temp/db.json'), PosixPath('/Users/pankaj/temp/Test.java'), PosixPath('/Users/pankaj/temp/routes.json'), PosixPath('/Users/pankaj/temp/itertools.py')]
If you run the same program in Windows, you will get instances of WindowsPath.
如果您在Windows中运行相同的程序,则将获得WindowsPath实例。
2.列出特定类型的文件 (2. Listing specific type of files)
We can use Path glob() function to iterate over a list of files matching the given pattern. Let’s use this function to print all the python scripts inside a directory.
我们可以使用Path glob()函数遍历匹配给定模式的文件列表。 让我们使用此功能来打印目录中的所有python脚本。
from pathlib import Path
path = Path("/Users/pankaj/temp")
python_files = path.glob('**/*.py')
for pf in python_files:
print(pf)
Output:
输出:

Python Pathlib List Files
Python Pathlib列表文件
3.解决符号链接到规范路径 (3. Resolving Symbolic Links to Canonical Path)
We can use resolve() function to convert the symbolic links to their canonical path.
我们可以使用resolve()函数将符号链接转换为其规范路径。
py2_path = Path("/usr/bin/python2.7")
print(py2_path)
print(py2_path.resolve())
Output:
输出:
/usr/bin/python2.7
/System/Library/Frameworks/Python.framework/Versions/2.7/bin/python2.7
4.检查文件或目录是否存在 (4. Check if a File or Directory Exists)
The Path exists() function returns True if the path exists, otherwise it returns False.
如果路径存在,则Path exist()函数将返回True,否则返回False。
path = Path("/Users/pankaj/temp")
print(path.exists()) # True
path = Path("/Users/pankaj/temp/random1234")
print(path.exists()) # False
5.打开和读取文件内容 (5. Opening and Reading File Contents)
We can use Path open() function to open the file. It returns a file object like the built-in open() function.
我们可以使用Path open()函数打开文件。 它返回一个文件对象,如内置的open()函数。
file_path = Path("/Users/pankaj/temp/test.py")
if file_path.exists() and file_path.is_file():
with file_path.open() as f:
print(f.readlines())
Output:
输出:
['import os\n', '\n', 'print("Hello World")\n']
6.获取文件信息 (6. Getting Information of the File)
The Path object stat() function make the stat() system call and return the results. The output is the same as the os module stat() function.
Path对象的stat()函数进行stat()系统调用并返回结果。 输出与os模块的 stat()函数相同。
file_path = Path("/Users/pankaj/temp/test.py")
print(file_path.stat())
Output:
输出:
os.stat_result(st_mode=33188, st_ino=8623963104, st_dev=16777220, st_nlink=1, st_uid=501, st_gid=20, st_size=32, st_atime=1566476310, st_mtime=1566476242, st_ctime=1566476242)
7.获取文件或目录名称 (7. Getting the File or Directory Name)
We can use “name” property to get the file name from the path object.
我们可以使用“名称”属性从路径对象获取文件名。
print(Path("/Users/pankaj/temp/test.py").name)
print(Path("/Users/pankaj/temp/").name)
print("Path without argument Name :", Path().name)
Output:
输出:
test.py
temp
Path without argument Name :
8.创建和删除目录 (8. Creating and Deleting a Directory)
We can use mkdir() function to create a directory. We can use rmdir() to delete an empty directory. If there are files, then we have to delete them first and then delete the directory.
我们可以使用mkdir()函数创建目录。 我们可以使用rmdir()删除一个空目录。 如果有文件,那么我们必须先删除它们,然后再删除目录。
directory = Path("/Users/pankaj/temp/temp_dir")
print(directory.exists()) # False
directory.mkdir()
print(directory.exists()) # True
directory.rmdir()
print(directory.exists()) # False
9.更改文件模式 (9. Change File Mode)
file = Path("/Users/pankaj/temp/test.py")
file.chmod(0o777)
The chmod() function behaves same as os.chmod() function to change the file permissions.
chmod()函数的行为与os.chmod()函数相同,以更改文件许可权。
10.获取文件组和所有者名称 (10. Getting File Group and Owner Name)
file = Path("/Users/pankaj/temp/test.py")
print(file.group()) # staff
print(file.owner()) # pankaj
11.展开〜至规范路径 (11. Expand ~ to Canonical Path)
path = Path("~/temp")
print(path) # ~/temp
path = path.expanduser()
print(path) # /Users/pankaj/temp
12. CWD和原路 (12. CWD and Home Path)
print(Path.cwd())
print(Path.home())
Output:
输出:
/Users/pankaj/Documents/PycharmProjects/PythonTutorials/hello-world
/Users/pankaj
13.连接两条路径 (13. Joining Two Paths)
path = Path.home()
path = path.joinpath(Path("temp"))
print(path) # /Users/pankaj/temp
14.创建一个空文件 (14. Creating an empty file)
Just like Unix touch command, Path has touch() function to create an empty file. You should have the permissions to create the file. Otherwise, the file won’t be created and there will be no error thrown.
就像Unix touch命令一样,Path具有touch()函数来创建一个空文件。 您应该具有创建文件的权限。 否则,将不会创建该文件,并且不会引发任何错误。
new_file = Path("/Users/pankaj/temp/xyz.txt")
print(new_file.exists()) # False
new_file.touch()
print(new_file.exists()) # True
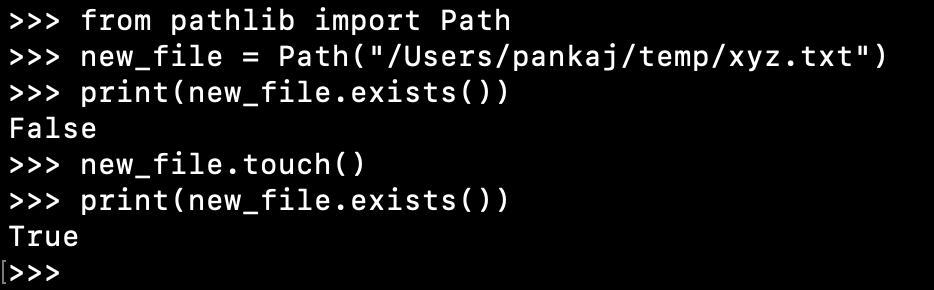
Python Pathlib Create New File
Python Pathlib创建新文件
结论 (Conclusion)
Python pathlib module is very useful in working with files and directories in an object-oriented way. The loosely coupled and platform-independent code makes it more attractive to use.
Python pathlib模块在以面向对象的方式处理文件和目录时非常有用。 松散耦合且独立于平台的代码使它的使用更具吸引力。
Reference: Official Docs – pathlib module
参考 : 官方文档– pathlib模块
翻译自: https://www.journaldev.com/32158/python-pathlib-module-14-practical-examples