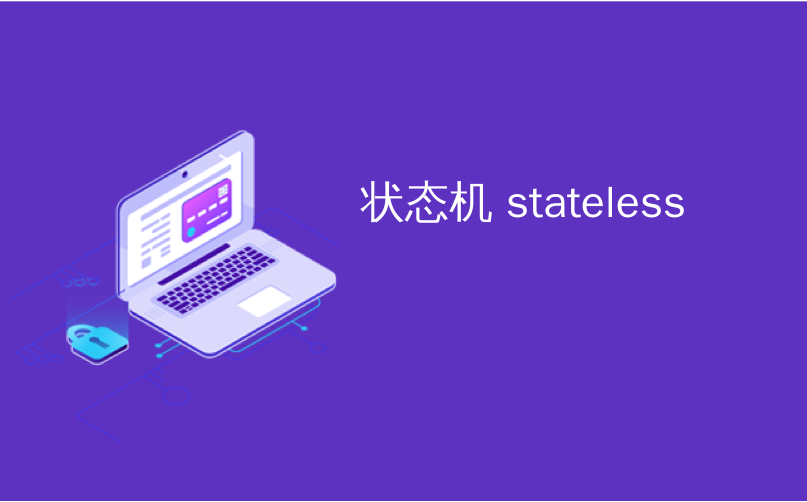
状态机 stateless
State Machines and business processes that describe a series of states seem like they'll be easy to code but you'll eventually regret trying to do it yourself. Sure, you'll start with a boolean, then two, then you'll need to manage three states and there will be an invalid state to avoid then you'll just consider quitting all together. ;)
描述一系列状态的状态机和业务流程似乎很容易编写代码,但是您最终会后悔自己尝试这样做。 当然,您将以一个布尔值开始,然后是两个,然后您将需要管理三个状态,并且将存在一个无效状态,以避免出现这种情况,然后您将考虑同时退出所有状态。 ;)
"Stateless" is a simple library for creating state machines in C# code. It's recently been updated to support .NET Core 1.0. They achieved this not by targeting .NET Core but by writing to the .NET Standard. Just like API levels in Android abstract away the many underlying versions of Android, .NET Standard is a set of APIs that all .NET platforms have to implement. Even better, the folks who wrote Stateless 3.0 targeted .NET Standard 1.0, which is the broadest and most compatible standard - it basically works everywhere and is portable across the .NET Framework on Windows, .NET Core on Windows, Mac, and LInux, as well as Windows Store apps and all phones.
“ Stateless ”是一个简单的库,用于使用C#代码创建状态机。 它最近已更新为支持.NET Core 1.0。 他们不是通过针对.NET Core而是通过编写.NET Standard来实现这一目标的。 就像Android中的API级别抽象了许多底层的Android版本一样,.NET Standard是所有.NET平台都必须实现的一组API。 更好的是,编写Stateless 3.0的人们针对的是.NET Standard 1.0,这是最广泛和最兼容的标准-它基本上可以在任何地方使用,并且可以跨Windows的.NET Framework,Windows,Mac的LME.NET和LInux进行移植,以及Windows Store应用和所有手机。
Sure, there's Windows Workflow, but it may be overkill for some projects. In Nicholas Blumhardt's words:
当然,这里有Windows Workflow,但对于某些项目来说可能有些过头了。 用尼古拉斯·布鲁姆哈特(Nicholas Blumhardt)的话说:
...over time, the logic that decided which actions were allowed in each state, and what the state resulting from an action should be, grew into a tangle of
if
andswitch
. Inspired by Simple State Machine, I eventually refactored this out into a little state machine class that was configured declaratively: in this state, allow this trigger, transition to this other state, and so-on.……随着时间的流逝,决定每个状态中允许哪些动作以及该动作应导致的状态是什么的逻辑,变成了
if
和switch
的纠结。 受简单状态机的启发,我最终将其重构为声明式配置的一个小的状态机类:在此状态下,允许此触发器,过渡到该另一状态,如此等等。
You can use state machines for anything. You can certainly describe high-level business state machines, but you can also easily model IoT device state, user interfaces, and more.
您可以将状态机用于任何事物。 您当然可以描述高级业务状态机,但是也可以轻松地为IoT设备状态,用户界面等建模。
Even better, Stateless also serialize your state machine to a standard text-based "DOT Graph" format that can then be generated into an SVG or PNG like this with http://www.webgraphviz.com. It's super nice to be able to visualize state machines at runtime.
更好的是,Stateless还可以将状态机序列化为基于文本的标准“ DOT Graph”格式,然后可以使用http://www.webgraphviz.com将其生成为SVG或PNG。 能够在运行时可视化状态机非常好。
使用无状态建模简单状态机 (Modeling a Simple State Machine with Stateless)
Let's look at a few code examples. You start by describing some finite states as an enum, and some finite "triggers" that cause a state to change. Like a switch could have On and Off as states and Toggle as a trigger.
让我们看一些代码示例。 您首先将一些有限状态描述为一个枚举,然后将某些引起状态改变的有限“触发器”描述为枚举。 就像开关可以将“打开”和“关闭”作为状态,而将“ Toggle”作为触发器。
A more useful example is the Bug Tracker included in the Stateless source on GitHub. To start with here are the states of a Bug and the Triggers that cause state to change:
一个更有用的示例是GitHub上的Stateless源中包含的Bug Tracker。 从这里开始是Bug的状态和导致状态更改的触发器:
enum State { Open, Assigned, Deferred, Resolved, Closed }
enum Trigger { Assign, Defer, Resolve, Close }
You then have your initial state, define your StateMachine, and if you like, you can pass Parameters when a state is trigger. For example, if a Bug is triggered with Assign you can pass in "Scott" so the bug goes into the Assigned state - assigned to Scott.
然后,您将拥有初始状态,定义StateMachine,并且如果愿意,可以在触发状态时传递参数。 例如,如果通过分配触发了错误,则可以传递“ Scott”,以便使错误进入“已分配”状态-分配给Scott。
State _state = State.Open;
StateMachine<State, Trigger> _machine;
StateMachine<State, Trigger>.TriggerWithParameters<string> _assignTrigger;
string _title;
string _assignee;
Then, in this example, the Bug constructor describes the state machine using a fluent interface that reads rather nicely.
然后,在此示例中,Bug构造函数使用流畅的接口描述了状态机,该接口读起来非常好。
public Bug(string title)
{
_title = title;
_machine = new StateMachine<State, Trigger>(() => _state, s => _state = s);
_assignTrigger = _machine.SetTriggerParameters<string>(Trigger.Assign);
_machine.Configure(State.Open)
.Permit(Trigger.Assign, State.Assigned);
_machine.Configure(State.Assigned)
.SubstateOf(State.Open)
.OnEntryFrom(_assignTrigger, assignee => OnAssigned(assignee))
.PermitReentry(Trigger.Assign)
.Permit(Trigger.Close, State.Closed)
.Permit(Trigger.Defer, State.Deferred)
.OnExit(() => OnDeassigned());
_machine.Configure(State.Deferred)
.OnEntry(() => _assignee = null)
.Permit(Trigger.Assign, State.Assigned);
}
For example, when the State is Open, it can be Assigned. But as this is written (you can change it) you can't close a Bug that is Open but not Assigned. Make sense?
例如,当状态为“打开”时,可以对其进行分配。 但是随着本文的撰写(您可以更改),您无法关闭已打开但未分配的错误。 有道理?
When the Bug is Assigned, you can Close it, Defer it, or Assign it again. That's PermitReentry(). Also, notice that Assigned is a Substate of Open.
分配错误后,您可以关闭,推迟或再次分配它。 那是PermitReentry()。 另外,请注意,Assigned是Open的子状态。
You can have events that are fired as states change. Those events can take actions as you like.
您可以拥有随着状态变化而触发的事件。 这些事件可以采取您喜欢的动作。
void OnAssigned(string assignee)
{
if (_assignee != null && assignee != _assignee)
SendEmailToAssignee("Don't forget to help the new employee.");
_assignee = assignee;
SendEmailToAssignee("You own it.");
}
void OnDeassigned()
{
SendEmailToAssignee("You're off the hook.");
}
void SendEmailToAssignee(string message)
{
Console.WriteLine("{0}, RE {1}: {2}", _assignee, _title, message);
}
With a nice State Machine library like Stateless you can quickly model states that you'd ordinarily do with a "big ol' switch statement."
使用像Stateless这样的不错的State Machine库,您可以快速建模通常用“ big ol'switch语句”执行的状态。
What have you used for state machines like this in your projects?
您在项目中将什么用于这样的状态机?
翻译自: https://www.hanselman.com/blog/stateless-30-a-state-machine-library-for-net-core
状态机 stateless