Maria and I were updating the NerdDinner sample app (not done yet, but soon) and were looking at various ways to do bundling and minification of the JSS and CS. There's runtime bundling on ASP.NET 4.x but in recent years web developers have used tools like Grunt or Gulp to orchestrate a client-side build process to squish their assets. The key is to find a balance that gives you easy access to development versions of JS/CSS assets when at dev time, while making it "zero work" to put minified stuff into production. Additionally, some devs don't need the Grunt/Gulp/npm overhead while others absolutely do. So how do you find balance? Here's how it works.
Maria和我正在更新NerdDinner示例应用程序(尚未完成,但很快就完成了),并且正在寻找各种方法来捆绑和最小化JSS和CS。 ASP.NET 4.x上存在运行时捆绑,但是近年来,Web开发人员已使用Grunt或Gulp之类的工具来协调客户端构建过程以压缩其资产。 关键是要找到一个平衡点,使您可以在开发时轻松访问JS / CSS资产的开发版本,同时将最小化的东西投入生产“零工作”。 此外,有些开发人员不需要Grunt / Gulp / npm开销,而其他开发人员则绝对需要。 那么,您如何找到平衡点? 运作方式如下。
I'm in Visual Studio 2017 and I go File | New Project | ASP.NET Core Web App. Bundling isn't on by default but the configuration you need IS included by default. It's just minutes to enable and it's quite nice.
我在Visual Studio 2017中,进入文件| 新项目| ASP.NET Core Web App。 捆绑默认情况下未启用,但默认情况下包含您需要的配置。 启用仅需几分钟,这非常不错。
In my Solution Explorer is a "bundleconfig.json" like this:
在我的解决方案资源管理器中,像这样的“ bundleconfig.json”:
// Configure bundling and minification for the project.
// More info at https://go.microsoft.com/fwlink/?LinkId=808241
[
{
"outputFileName": "wwwroot/css/site.min.css",
// An array of relative input file paths. Globbing patterns supported
"inputFiles": [
"wwwroot/css/site.css"
]
},
{
"outputFileName": "wwwroot/js/site.min.js",
"inputFiles": [
"wwwroot/js/site.js"
],
// Optionally specify minification options
"minify": {
"enabled": true,
"renameLocals": true
},
// Optionally generate .map file
"sourceMap": false
}
]
Pretty simple. Ins and outs. At the top of the VS editor you'll see this yellow prompt. VS knows you're in a bundleconfig.json and in order to use it effectively in VS you grab a small extension. To be clear, it's NOT required. It just makes it easier. The source is at https://github.com/madskristensen/BundlerMinifier. Slip this UI section if you just want Build-time bundling.
很简单来龙去脉。 在VS编辑器的顶部,您会看到黄色的提示。 VS知道您在bundleconfig.json中,为了在VS中有效使用它,您需要获取一个小扩展名。 需要明确的是,它不是必需的。 它只是使它更容易。 来源位于https://github.com/madskristensen/BundlerMinifier 。 如果只需要构建时捆绑,请滑动此UI部分。
If getting a prompt like this bugs you, you can turn all prompting off here:
如果收到这样的提示使您感到烦恼,则可以在此处关闭所有提示:
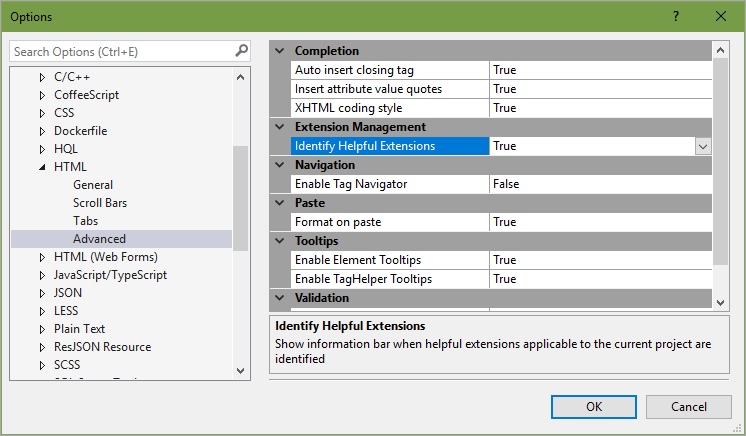
Look at your Solution Explorer. See under site.css and site.js? There are associated minified versions of those files. They aren't really "under" them. They are next to them on the disk, but this hierarchy is a nice way to see that they are associated, and that one generates the other.
查看您的解决方案资源管理器。 在site.css和site.js下看到? 有那些文件的关联的缩小版本。 他们并不是真正的“下”。 它们在磁盘上紧挨着它们,但是这种层次结构是一种很好的方式,可以看到它们是关联的,并且一个会生成另一个。
Right click on your project and you'll see this Bundler & Minifier menu:
右键单击您的项目,您将看到此Bundler&Minifier菜单:
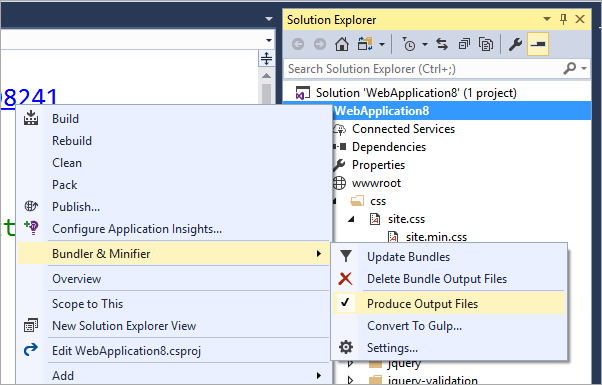
You can manually update your Bundles with this item as well as see settings and have bundling show up in the Task Runner Explorer.
您可以使用此项目手动更新捆绑包,也可以查看设置并在Task Runner Explorer中显示捆绑。
建立时间最小化 (Build Time Minification)
The VSIX (VS extension) gives you the small menu and some UI hooks, but if you want to have your bundles updated at build time (useful if you don't use VS!) then you'll want to add a NuGet package called BuildBundlerMinifier.
VSIX(VS扩展)为您提供了小菜单和一些UI挂钩,但是如果您想在构建时更新捆绑包(如果不使用VS则很有用!),那么您将需要添加一个名为NuGet的软件包BuildBundlerMinifier 。
You can add this NuGet package SEVERAL ways. Which is awesome.
您可以通过多种方式添加此NuGet包。 太棒了
- Add it from the Manage NuGet Packages menu从Manage NuGet Packages菜单添加
- Add it from the command line via "dotnet add package BuildBundlerMinifier"通过“ dotnet添加软件包BuildBundlerMinifier”从命令行添加它
Note that this adds it to your csproj without you having to edit it! It's like "nuget install" but adds references to projects! The dotnet CLI is lovely.
请注意,这无需编辑即可将其添加到您的csproj中! 就像“ nuget install”一样,但是添加了对项目的引用! dotnet CLI非常可爱。
If you have the VSIX installed, just right-click the bundleconfig.json and click "Enable bundle on build..." and you'll get the NuGet package.
如果您安装了VSIX,只需右键单击bundleconfig.json并单击“在构建中启用捆绑...”,您将获得NuGet软件包。
If you have the VSIX installed, just right-click the bundleconfig.json and click "Enable bundle on build..." and you'll get the NuGet package.
如果您安装了VSIX,只需右键单击bundleconfig.json并单击“在构建时启用捆绑...”,您将获得NuGet软件包。
Now bundling will run on build...
现在捆绑将在构建中运行...
c:\WebApplication8\WebApplication8>dotnet build
Microsoft (R) Build Engine version 15
Copyright (C) Microsoft Corporation. All rights reserved.
Bundler: Begin processing bundleconfig.json
Bundler: Done processing bundleconfig.json
WebApplication8 -> c:\WebApplication8\bin\Debug\netcoreapp1.1\WebApplication8.dll
Build succeeded.
0 Warning(s)
0 Error(s)
...even from the command line with "dotnet build." It's all integrated.
...甚至在命令行中使用“ dotnet build”。 全部集成。
This is nice for VS Code or users of other editors. Here's how it would work entirely from the command prompt:
这对VS Code或其他编辑器的用户来说非常好。 这是完全在命令提示符下工作的方式:
$ dotnet new mvc
$ dotnet add package BuildBundlerMinifier
$ dotnet restore
$ dotnet run
进阶:使用Gulp处理捆绑/缩小 (Advanced: Using Gulp to handle Bundling/Minifying)
If you outgrow this bundler or just like Gulp, you can right click and Convert to Gulp!
如果您不再使用捆绑器,或者像Gulp一样,可以右键单击并转换为Gulp!
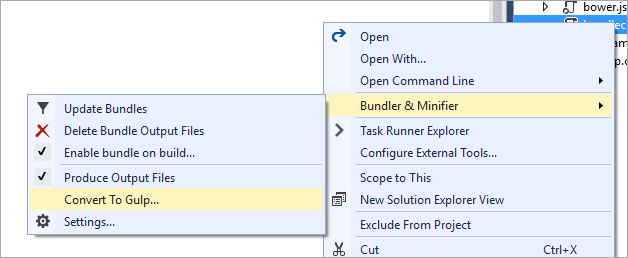
Now you'll get a gulpfile.js that uses the bundleconfig.json and you've got full control:
现在,您将获得一个使用bundleconfig.json的gulpfile.js,并且可以完全控制:
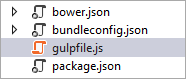
And during the conversion you'll get the npm packages you need to do the work automatically:
在转换期间,您将获得自动完成工作所需的npm软件包:
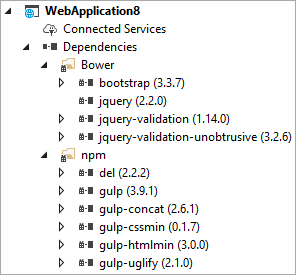
I've found this to be a good balance that can get quickly productive with a project that gets bundling without npm/node, but I can easily grow to a larger, more npm/bower/gulp-driven front-end developer-friendly app.
我发现这是一个很好的平衡点,可以在没有npm / node的情况下进行捆绑的项目快速提高生产率,但是我可以轻松地成长为更大,更npm / bower / gulp驱动的前端开发人员友好型应用程序。
翻译自: https://www.hanselman.com/blog/options-for-css-and-js-bundling-and-minification-with-aspnet-core