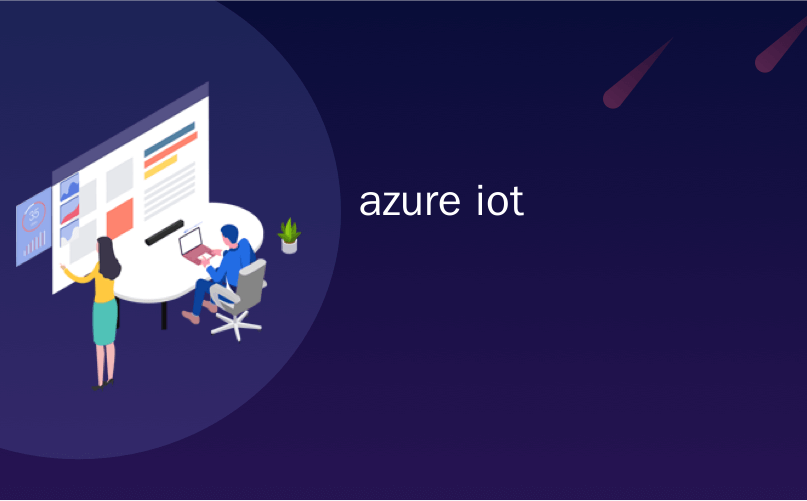
azure iot
My vacation continues. Yesterday I had shoulder surgery (adhesive capsulitis release) so today I'm messing around with Azure IoT Hub. I had some devices on my desk - some of which I had never really gotten around to exploring - and I thought I'd see if I could accomplish something.
我的假期还在继续。 昨天我进行了肩部手术(释放粘膜性囊膜炎),所以今天我在玩弄Azure IoT中心。 我的桌子上有一些设备-其中一些我从未真正探索过-我想知道我是否可以完成某些工作。
I've got a Particle Photon here, as well as a Tessel 2, a LattePanda, Funduino, and Onion Omega. A few days ago I was able to get the Onion Omega to show my blood sugar on a small OLED screen, which was cool. Tonight I'm going to try to hook the Particle Photon up to the Azure IoT hub for monitoring.
我在这里有一个粒子光子,还有Tessel 2,LattePanda,Funduino和Onion Omega 。 几天前,我能够买到Onion Omega,在一个很小的OLED屏幕上显示血糖,这很酷。 今晚,我将尝试将粒子光子连接到Azure IoT中心进行监视。
The Photon is a tiny little device with Wi-Fi built-in. It's super easy to setup and it has a cloud-based IDE with tons of examples written in C and Node.js for you to use. Particle Photon also has a node.js based command line. From there you can list out your Photons, see their available functions, and even call functions over the internet! A hacker's delight, to be sure.
Photon是内置Wi-Fi的微型设备。 它非常易于设置,并且具有基于云的IDE,其中包含大量用C和Node.js编写的示例供您使用。 粒子光子还具有基于node.js的命令行。 在这里,您可以列出光子,查看其可用功能,甚至可以通过Internet调用功能! 可以肯定,黑客的喜悦。
Here's a standard "blink an LED" Hello world on a Photon. This one creates a cloud function called "led" and binds it to the "ledToggle" method. Those cloud methods take a string, so there's no enum for the on/off command.
这是光子上的标准“闪烁LED” Hello世界。 这将创建一个称为“ led”的云函数,并将其绑定到“ ledToggle”方法。 这些云方法采用字符串,因此on / off命令没有枚举。
int led1 = D0;
int led2 = D7;
void setup() {
pinMode(led1, OUTPUT);
pinMode(led2, OUTPUT);
Spark.function("led",ledToggle);
digitalWrite(led1, LOW);
digitalWrite(led2, LOW);
}
void loop() {
}
int ledToggle(String command) {
if (command=="on") {
digitalWrite(led1,HIGH);
digitalWrite(led2,HIGH);
return 1;
}
else if (command=="off") {
digitalWrite(led1,LOW);
digitalWrite(led2,LOW);
return 0;
}
else {
return -1;
}
}
From the command line I can use the Particle command line interface (CLI) to enumerate my devices:
从命令行,我可以使用粒子命令行界面(CLI)枚举我的设备:
C:\Users\scott>particle list
hansel_photon [390039000647xxxxxxxxxxx] (Photon) is online
Functions:
int led(String args)
See how it doesn't just enumerate devices, but also cloud methods that hang off devices? LOVE THIS.
看到它不仅枚举设备,还枚举使设备挂起的云方法吗? 喜欢这个。
I can get a secret API Key from the Particle Photon's cloud based Console. Then using my Device ID and auth token I can call the method...with an HTTP request! How much easier could this be?
我可以从Particle Photon的基于云的Console中获得一个秘密的API密钥。 然后使用我的设备ID和身份验证令牌,可以使用HTTP请求来调用该方法! 这有多容易?
C:\Users\scott\>curl https://api.particle.io/v1/devices/390039000647xxxxxxxxx/led -d access_token=31fa2e6f --insecure -d arg="on"
{
"id": "390039000647xxxxxxxxx",
"last_app": "",
"connected": true,
"return_value": 1
}
At this moment the LED on the Particle Photon turns on. I'm going to change the code a little and add some telemetry using the Particle's online code editor.
此时,粒子光子上的LED点亮。 我将稍作更改代码,并使用Particle的在线代码编辑器添加一些遥测。
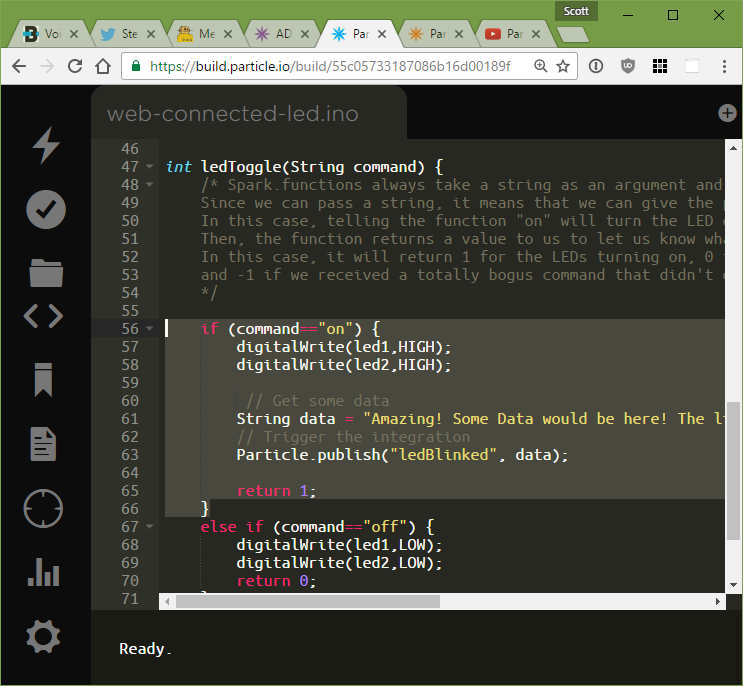
They've got a great online code editor, but I could also edit and compile the code locally:
他们有一个很棒的在线代码编辑器,但是我也可以在本地编辑和编译代码:
C:\Users\scott\Desktop>particle compile photon webconnected.ino
Compiling code for photon
Including:
webconnected.ino
attempting to compile firmware
downloading binary from: /v1/binaries/5858b74667ddf87fb2a2df8f
saving to: photon_firmware_1482209089877.bin
Memory use:
text data bss dec hex filename
6156 12 1488 7656 1de8
Compile succeeded.
Saved firmware to: C:\Users\scott\Desktop\photon_firmware_1482209089877.bin
I'll change the code to announce an "Event" when I turn on the LED.
打开LED灯时,我将更改代码以宣布“事件”。
if (command=="on") {
digitalWrite(led1,HIGH);
digitalWrite(led2,HIGH);
String data = "Amazing! Some Data would be here! The light is on.";
Particle.publish("ledBlinked", data);
return 1;
}
I can head back over to the http://console.particle.io and see these events live on the web:
我可以回到http://console.particle.io并在网上看到这些事件:
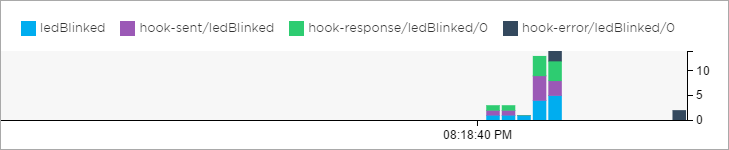
Particle also supports integration with Google Cloud and Azure IoT Hub. Azure IoT Hub allows you to manage billions of devices and all their many billions of events. I just have a few, but we all have to start somewhere. ;)
粒子还支持与Google Cloud和Azure IoT中心的集成。 Azure IoT中心使您可以管理数十亿个设备及其数十亿个事件。 我只有几个,但我们都必须从某个地方开始。 ;)
I created a free Azure IoT Hub in my Azure Account...
我在Azure帐户中创建了一个免费的Azure IoT中心...
And made a shared access policy for my Particle Devices.
并为我的粒子设备制定了共享访问策略。
Then I told Particle about Azure in their Integrations system.
然后,我向粒子公司介绍了其集成系统中的Azure。
The Azure IoT SDKS on GitHub at https://github.com/Azure/azure-iot-sdks/releases have both a Windows-based Azure IoT Explorer and a command-line one called IoT Hub Explorer.
GitHub上位于https://github.com/Azure/azure-iot-sdks/releases上的Azure IoT SDKS既有基于Windows的Azure IoT Explorer,又有一个称为IoT Hub Explorer的命令行。
I logged in to the IoT Hub Explorer using the connection string from the Azure Portal:
我使用来自Azure门户的连接字符串登录到IoT中心浏览器:
iothub-explorer login "HostName=HanselIoT.azure-devices.net;SharedAccessKeyName=particle-iot-hub;SharedAccessKey=rdWUVMXs="
Then I'll run "iothub-explorer monitor-events" passing in the device ID and the connection string for the shared access policy. Monitor-events is cool because it'll hang and just output the events as they're flowing through the whole system.
然后,我将运行“ iothub-explorer monitor-events ”,传入共享访问策略的设备ID和连接字符串。 Monitor-events很酷,因为它会挂起并在事件流经整个系统时将其输出。
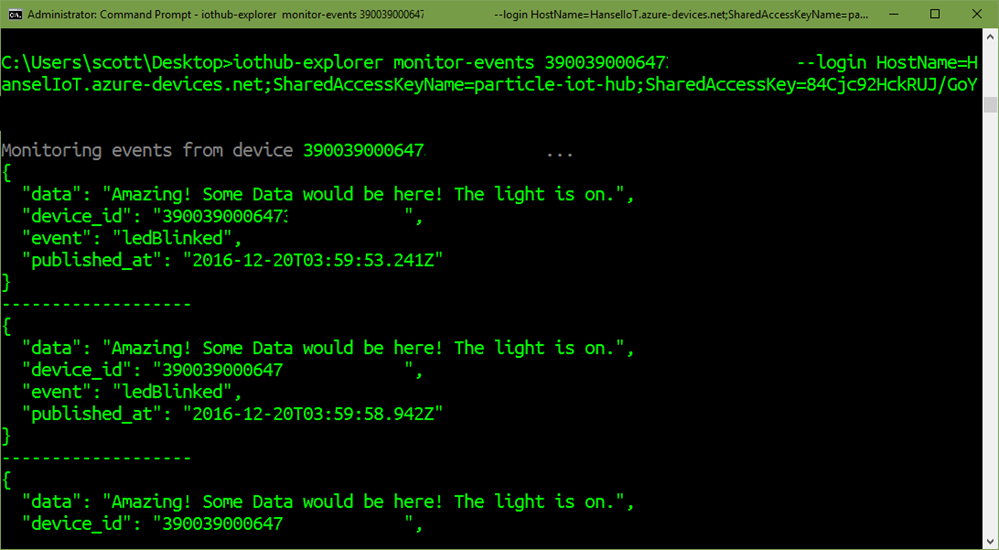
So I'm able to call methods on the Particle using their cloud, and monitor events from within Azure IoT Hub. I can explore diagnostics data and query huge amounts of device-to-cloud data that would potentially flow in from my hardware devices.
因此,我能够使用它们的云在粒子上调用方法,并从Azure IoT中心内监视事件。 我可以浏览诊断数据并查询可能从我的硬件设备流入的大量设备到云数据。
The IoT Hub Limits are very generous for free/hobbyist users as we learn to develop. I haven't paid anything yet. However, it can scale to thousands of messages a second per unit! That means millions of messages a second if you need it.
随着我们学习开发, IoT中心限制对于免费/业余用户非常慷慨。 我还没有付钱。 但是,它每秒可以扩展到数千条消息! 这意味着如果您需要,每秒可以发送数百万条消息。
I can definitely see how the the value an IoT Hub solution like this would add up quickly after you've got more than one device. Text files don't really scale. Even if I just IoT'ed up my house, it would be nice to have all that data flowing into a single hub I could manage and query securely.
我绝对可以看到拥有多个设备的IoT中心解决方案的价值将如何Swift增加。 文本文件并不能真正缩放。 即使我只是物联网,也可以将所有数据流到一个我可以安全管理和查询的集线器中,这还是很好的。
azure iot