Lambda functions are special types of functions that are not expressed as regular functions. Lambda functions do not have any function name to call and they are created for single line simple functions. The lambda function will eliminate the def
keyword for a function definition.
Lambda函数是特殊类型的函数,未表示为常规函数。 Lambda函数没有要调用的任何函数名,它们是为单行简单函数创建的。 lambda函数将消除函数定义的def
关键字。
Lambda functions are also called a small anonymous function where it does not have any function name. In the following part of the tutorial, we will see that lambda functions can take different types of arguments.
Lambda函数也称为小型匿名函数,其中没有任何函数名。 在本教程的以下部分中,我们将看到lambda函数可以采用不同类型的参数。
Lambda函数的使用特性和用例 (Lambda Function Use Characteristics and Use Cases)
- As a single line function definition it only contains expressions and does not contain any statements in its body.作为单行函数定义,它仅包含表达式,并且其主体中不包含任何语句。
- It is written as a single line of execution 它被写为一行执行
- Lambda functions are easy to read because of its simplicity and atomicity.Lambda函数由于其简单性和原子性而易于阅读。
- Lambda functions do not support type annotations. Lambda函数不支持类型注释。
- Lambda functions can be immediately called after definition by adding parameters in the same line. 通过在同一行中添加参数,可以在定义后立即调用Lambda函数。
Lambda的语法 (Syntax of Lambda)
The syntax of the Lambda function definition is very different than the other Python keywords and structures.
Lambda函数定义的语法与其他Python关键字和结构非常不同。
VAR = lambda ARG1, ARG2, ... : EXPRESSION
- `VAR` is the variable name where the lambda function will be assigned. VAR是将在其中分配lambda函数的变量名称。
- `lambda` is the keyword used to define and create a lambda function lambda是用于定义和创建lambda函数的关键字
- `ARG1` ,`ARG2` , … are arguments for the lambda function. We can use a single argument or multiple arguments. As they are optional we can also provide zero argument. “ ARG1”,“ ARG2”,……是lambda函数的参数。 我们可以使用一个或多个参数。 由于它们是可选的,因此我们还可以提供零参数。
- `EXPRESSION` ist the body of the lambda function which will be executed every time the lambda function is called EXPRESSION是lambda函数的主体,每次调用lambda函数时都会执行
Lambda示例(Lambda Example)
We will start with a simple example where we will provide a single argument to the lambda function. We will provide the argument name as a
and the lambda function will be assigned to the x
.
我们将从一个简单的示例开始,在该示例中,我们将为lambda函数提供一个参数。 我们将以a
作为参数名称,并将lambda函数分配给x
。
x = lambda a: "This is the "+a
print(x("poftut.com"))
x = lambda a: a*a*a
print(x(3))
print(x(4))
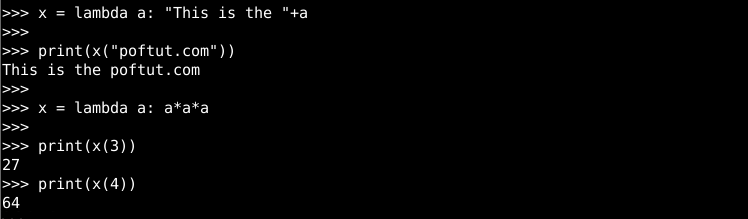
- In the first example, we will provide the “poftut.com” to the lambda function and the function will return the string “This is the poftut.com”.在第一个示例中,我们将为lambda函数提供“ poftut.com”,该函数将返回字符串“ This is the poftut.com”。
- In the second example, we provide 3 to the lambda function and it is multiplied 3 times with itself. 在第二个示例中,我们为lambda函数提供3并将其自身乘以3倍。
- In the second example, we provide 4 to the lambda function and it is multiplied 3 times with itself. 在第二个示例中,我们为lambda函数提供4,并将其自身乘以3倍。
没有参数的Lambda (Lambda without Argument)
We can use the lambda function without providing any argument. We will just put the expression part of the lambda function and use the newly created Lambda function. In this example, we will create the lambda function and assign it to the x
where the function will print “This is the X” to the screen.
我们可以使用lambda函数而无需提供任何参数。 我们将只放入lambda函数的表达式部分,并使用新创建的Lambda函数。 在此示例中,我们将创建lambda函数并将其分配给x
,该函数将在屏幕上显示“ This is X”。
x = lambda : "This is the X"
print(x())
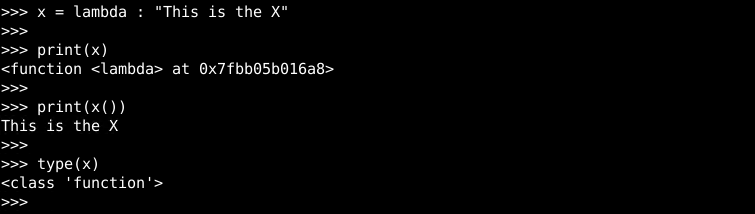
直接调用Lambda函数 (Call Lambda Function Directly)
Lambda function can be also called directly without assigning it to a variable. We will just provide the parameters after the lambda function definition. We will also surround the lambda function and provided arguments with brackets. In the following examples, we will do math and print “This site is poftut.com”.
Lambda函数也可以直接调用而无需将其分配给变量。 我们只在lambda函数定义之后提供参数。 我们还将围绕lambda函数,并为参数提供方括号。 在以下示例中,我们将进行数学运算并打印“此站点为poftut.com”。
(lambda x: x + 1)(2)
(lambda x: x * x + 1)(3)
(lambda x: "This site is "+x)("poftut.com")
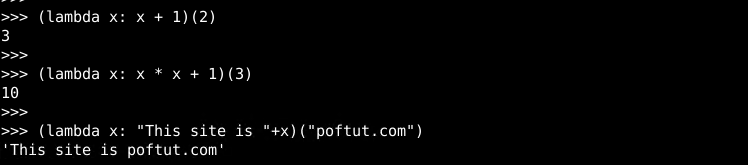
具有多个参数的Lambda(Lambda with Multiple Arguments)
Lambda functions can be used without argument or a single argument or multiple arguments. We can provide multiple arguments in a row which is also called positional arguments. In this example, we will provide arguments a as 1 , b as 2 and c as 5 like below.
Lambda函数可以不带参数,也可以不带单个参数或多个参数。 我们可以连续提供多个参数,这也称为位置参数。 在此示例中,我们将提供参数a作为1,b作为2,c作为5,如下所示。
x = lambda a, b, c: a + b + c
print(x(1, 2, 5))
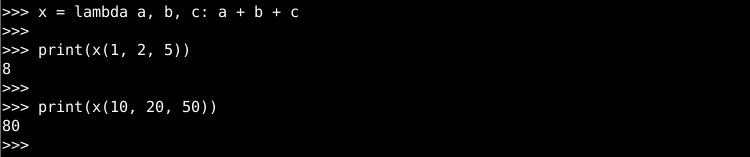
带命名参数的Lambda (Lambda with Named Arguments)
We can use named arguments in lambda functions. We will set the c
value as 5 by default. If the c is not provided to the lambda function call it will be assumed as 5. If provided the provided new value will be used.
我们可以在lambda函数中使用命名参数。 默认情况下,我们将c
值设置为5。 如果未向lambda函数调用提供c,则将其假定为5。如果提供,将使用提供的新值。
x = lambda a, b, c=5 : a + b + c
print(x(1, 2))
print(x(10, 20))
print(x(1, 2, 10))
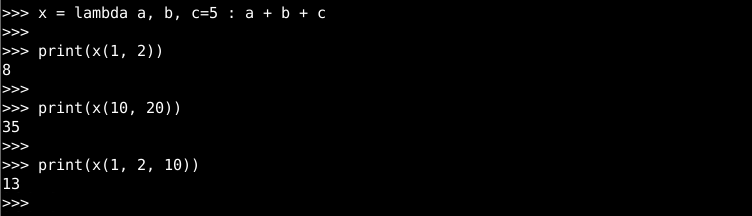
具有可变参数列表的Lambda (Lambda with Variable List Of Arguments)
If the provided arguments list and the count are variable we have to use args
keyword which can map variable-length arguments. We will use *args
as variable-length arguments and use inside the expression.
如果提供的参数列表和计数是变量,则必须使用args
关键字,它可以映射可变长度的参数。 我们将使用*args
作为变长参数,并在表达式内部使用。
x = lambda *args : sum(args)
x(1,2,3)
x(1)
x(1,2)
x(1,2,3,4)
x(1,2,3,4,5)
x(1,2,3,4,5,6)
x(1,2,3,4,5,6,7)
x(1,2,3,4,5,6,7,8)
x(1,2,3,4,5,6,7,8,9)
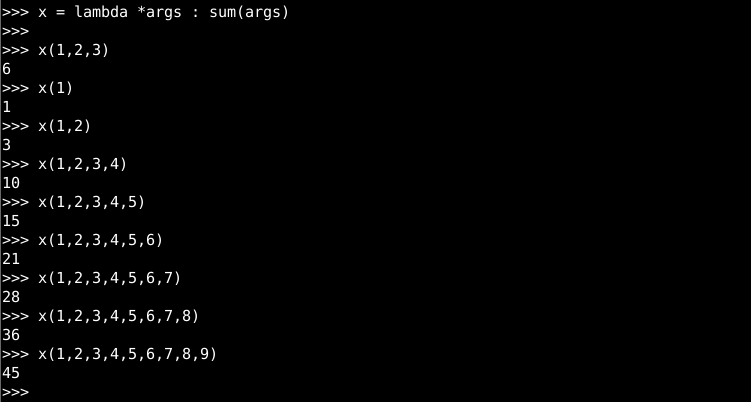
带关键字参数可变列表的Lambda (Lambda with Variable List Of Keyword Arguments)
We can also use a variable list of keywords inside the Lambda functions. We will use kwargs
keyword and select their values with the values()
function. We will provide the arguments in different names.
我们还可以在Lambda函数中使用关键字的可变列表。 我们将使用kwargs
关键字,并通过values()
函数选择其值。 我们将以不同的名称提供参数。
x = lambda **kwargs : sum(kwargs.values())
x(a=1 , b=2 )
x(a=1 , b=2 , c=3 )
x(a=1 , b=2 , c=3 , d=4 )
x(a=1 , b=2 , c=3 , d=4 , e=5 )
x(a=1 , b=2 , c=3 , d=4 , e=5 , f=6 )
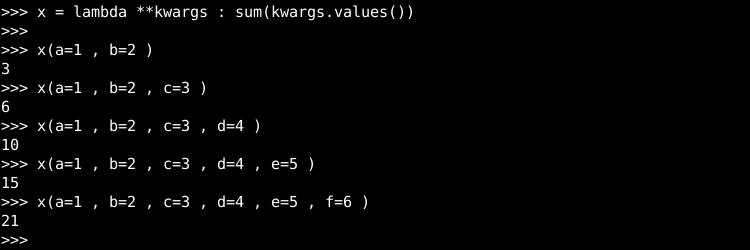
带Python交互式Shell的Lambda (Lambda with Python Interactive Shell)
Lambda provides special usage with the Python interactive shell. We can create a lambda function without a variable assignment and call it later with the underscore _
operator. But we can only call the lambda function for one time after definition. If we try to call the lambda function the second time after the definition we will get an exception.
Lambda通过Python交互式shell提供了特殊用法。 我们可以创建不带变量分配的lambda函数,并稍后使用下划线_
运算符调用它。 但是定义后,我们只能调用lambda函数一次。 如果我们在定义之后第二次尝试调用lambda函数,我们将获得异常。
>>> lambda x: "This site is "+x
>>> _("POFTUT.com")
>>> lambda x: x * x + 1
>>> _(3)
>>> _(4)
>>> lambda x: x * x + 1
>>> _(4)
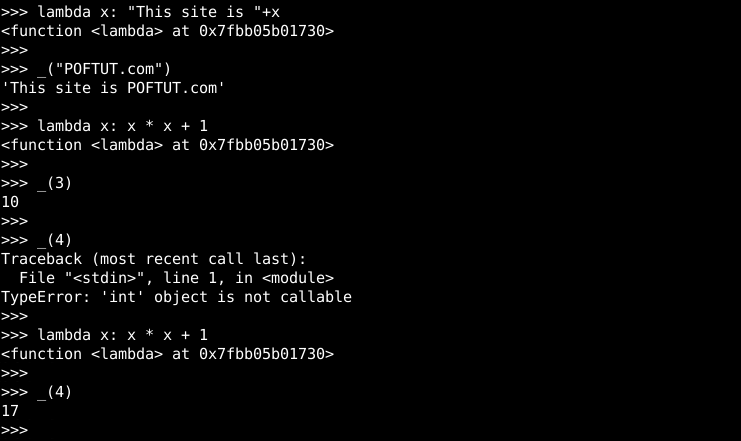
带filter()函数的Lambda (Lambda with filter() Function)
filter()
function is used to filter a sequence of variables according to the situation which is defined in a function. Lambda functions are very useful to create a situation for filtering. Using the lambda function will also make the filter more readable.
filter()
函数用于根据函数中定义的情况过滤变量序列。 Lambda函数对于创建过滤条件非常有用。 使用lambda函数还将使过滤器更具可读性。
mynumbers = [ 2 , 4 , 5 , 34 , 245 , 56 , 4 , 7 , 8 , 0 , 45 ]
filtered_numbers = list(filter(lambda x: (x%2 !=0), mynumbers))
print(filtered_numbers)
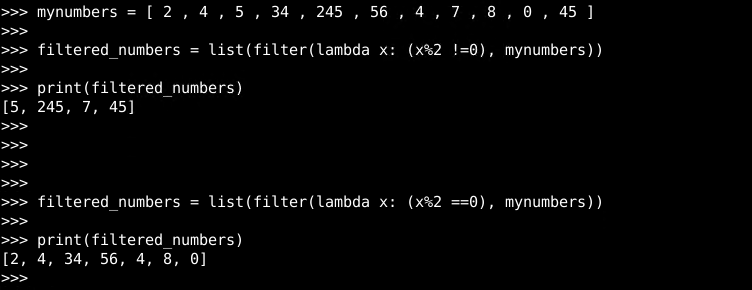
带map()函数的Lambda (Lambda with map() Function)
map()
function is used to run a given function on the given list elements one by one. Lambda function another popular use case is using it with given list elements by using map() function. In this example, we will use lambda function in order to calculate square of the given list elements one by one.
map()
函数用于在给定列表元素上一对一地运行给定函数。 Lambda函数的另一个流行用例是通过使用map()函数将其与给定的列表元素一起使用。 在此示例中,我们将使用lambda函数来一一计算给定列表元素的平方。
mynumbers = [ 2 , 4 , 5 , 34 , 245 , 56 , 4 , 7 , 8 , 0 , 45 ]
filtered_numbers = list(map(lambda x: x * x , mynumbers))
print(filtered_numbers)

带reduce()函数的Lambda (Lambda with reduce() Function)
reduce()
is another interesting function wherefrom the given list multiple elements can be processed by the given function. As a function lambda can be used very efficiently for simple operations. In this example, we will sum for 2 neighbor elements in each step or reduce.
reduce()
是另一个有趣的函数,从给定列表中可以通过给定函数处理多个元素。 作为一个函数,lambda可以非常有效地用于简单的操作。 在此示例中,我们将在每个步骤中对2个相邻元素求和或归约。
mynumbers = [ 2 , 4 , 5 , 34 , 245 , 56 , 4 , 7 , 8 , 0 , 45 ]
filtered_numbers = reduce(lambda x, y: x+y , mynumbers)
print(filtered_numbers)

翻译自: https://www.poftut.com/python-lambda-anonymous-function-tutorial-with-examples/