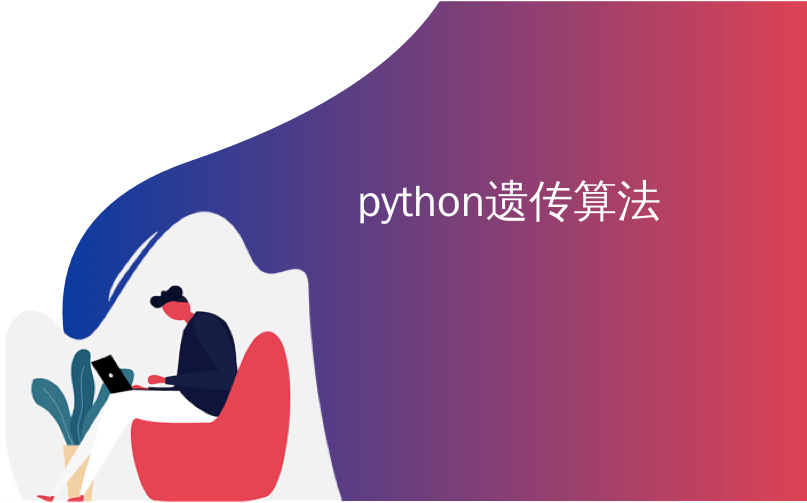
python遗传算法
带有Python的AI –遗传算法 (AI with Python – Genetic Algorithms)
This chapter discusses Genetic Algorithms of AI in detail.
本章详细讨论了AI的遗传算法。
什么是遗传算法? (What are Genetic Algorithms?)
Genetic Algorithms (GAs) are search based algorithms based on the concepts of natural selection and genetics. GAs are a subset of a much larger branch of computation known as Evolutionary Computation.
遗传算法(GA)是基于搜索的算法,基于自然选择和遗传学的概念。 GA是称为进化计算的更大计算分支的子集。
GAs were developed by John Holland and his students and colleagues at the University of Michigan, most notably David E. Goldberg. It has since been tried on various optimization problems with a high degree of success.
GA由John Holland和密歇根大学的学生和同事开发,最著名的是David E. Goldberg。 此后,它已在各种优化问题上得到了成功的尝试。
In GAs, we have a pool of possible solutions to the given problem. These solutions then undergo recombination and mutation (like in natural genetics), produces new children, and the process is repeated for various generations. Each individual (or candidate solution) is assigned a fitness value (based on its objective function value) and the fitter individuals are given a higher chance to mate and yield fitter individuals. This is in line with the Darwinian Theory of Survival of the Fittest.
在GA中,我们为给定问题提供了可能的解决方案池。 这些溶液然后进行重组和突变(就像在自然遗传学中一样),产生了新的孩子,并且这一过程重复了好几代。 为每个个体(或候选解决方案)分配一个适应度值(基于其目标函数值),使钳工个体有更高的机会交配并产生钳工个体。 这与适者生存的达尔文生存理论一致。
Thus, it keeps evolving better individuals or solutions over generations, till it reaches a stopping criterion.
因此,它会不断发展更好的个人或解决方案,直到达到停止标准为止。
Genetic Algorithms are sufficiently randomized in nature, but they perform much better than random local search (where we just try random solutions, keeping track of the best so far), as they exploit historical information as well.
遗传算法本质上具有足够的随机性,但它们的性能也比随机局部搜索(我们只尝试随机解,跟踪迄今为止的最佳方案)要好得多,因为它们也利用了历史信息。
如何使用GA解决优化问题? (How to Use GA for Optimization Problems?)
Optimization is an action of making design, situation, resource and system, as effective as possible. The following block diagram shows the optimization process −
优化是使设计,情况,资源和系统尽可能有效的一种动作。 以下框图显示了优化过程-

遗传算法优化过程的各个阶段 (Stages of GA mechanism for optimization process)
The following is a sequence of steps of GA mechanism when used for optimization of problems.
以下是GA机制用于优化问题时的一系列步骤。
Step 1 − Generate the initial population randomly.
步骤1-随机生成初始种群。
Step 2 − Select the initial solution with best fitness values.
步骤2-选择具有最佳适应性值的初始解。
Step 3 − Recombine the selected solutions using mutation and crossover operators.
步骤3-使用变异和交叉算子重组所选的解决方案。
Step 4 − Insert an offspring into the population.
步骤4-将后代插入种群。
Step 5 − Now, if the stop condition is met, return the solution with their best fitness value. Else go to step 2.
步骤5-现在,如果满足停止条件,则以最佳适应度值返回解决方案。 否则转到步骤2。
安装必要的软件包 (Installing Necessary Packages)
For solving the problem by using Genetic Algorithms in Python, we are going to use a powerful package for GA called DEAP. It is a library of novel evolutionary computation framework for rapid prototyping and testing of ideas. We can install this package with the help of the following command on command prompt −
为了通过在Python中使用遗传算法解决问题,我们将使用一个称为DEAP的功能强大的GA软件包。 它是一个新颖的进化计算框架库,用于思想的快速原型制作和测试。 我们可以在命令提示符下的以下命令的帮助下安装此软件包-
pip install deap
If you are using anaconda environment, then following command can be used to install deap −
如果您使用anaconda环境,那么可以使用以下命令来安装deap-
conda install -c conda-forge deap
使用遗传算法实施解决方案 (Implementing Solutions using Genetic Algorithms)
This section explains you the implementation of solutions using Genetic Algorithms.
本节说明使用遗传算法的解决方案的实现。
生成位模式 (Generating bit patterns)
The following example shows you how to generate a bit string that would contain 15 ones, based on the One Max problem.
以下示例显示了如何根据一个最大问题生成一个包含15个位字符串的位字符串。
Import the necessary packages as shown −
导入所需的软件包,如下所示:
import random
from deap import base, creator, tools
Define the evaluation function. It is the first step to create a genetic algorithm.
定义评估功能。 这是创建遗传算法的第一步。
def eval_func(individual):
target_sum = 15
return len(individual) - abs(sum(individual) - target_sum),
Now, create the toolbox with the right parameters −
现在,使用正确的参数创建工具箱-
def create_toolbox(num_bits):
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
Initialize the toolbox
初始化工具箱
toolbox = base.Toolbox()
toolbox.register("attr_bool", random.randint, 0, 1)
toolbox.register("individual", tools.initRepeat, creator.Individual,
toolbox.attr_bool, num_bits)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
Register the evaluation operator −
注册评估运算符-
toolbox.register("evaluate", eval_func)
Now, register the crossover operator −
现在,注册交叉运算符-
toolbox.register("mate", tools.cxTwoPoint)
Register a mutation operator −
注册一个变异算子-
toolbox.register("mutate", tools.mutFlipBit, indpb = 0.05)
Define the operator for breeding −
定义育种运算符-
toolbox.register("select", tools.selTournament, tournsize = 3)
return toolbox
if __name__ == "__main__":
num_bits = 45
toolbox = create_toolbox(num_bits)
random.seed(7)
population = toolbox.population(n = 500)
probab_crossing, probab_mutating = 0.5, 0.2
num_generations = 10
print('\nEvolution process starts')
Evaluate the entire population −
评估整个人口-
fitnesses = list(map(toolbox.evaluate, population))
for ind, fit in zip(population, fitnesses):
ind.fitness.values = fit
print('\nEvaluated', len(population), 'individuals')
Create and iterate through generations −
几代人创建和迭代-
for g in range(num_generations):
print("\n- Generation", g)
Selecting the next generation individuals −
选择下一代人-
offspring = toolbox.select(population, len(population))
Now, clone the selected individuals −
现在,克隆所选的个人-
offspring = list(map(toolbox.clone, offspring))
Apply crossover and mutation on the offspring −
对子代进行交叉和突变-
for child1, child2 in zip(offspring[::2], offspring[1::2]):
if random.random() < probab_crossing:
toolbox.mate(child1, child2)
Delete the fitness value of child
删除孩子的健身价值
del child1.fitness.values
del child2.fitness.values
Now, apply mutation −
现在,应用突变-
for mutant in offspring:
if random.random() < probab_mutating:
toolbox.mutate(mutant)
del mutant.fitness.values
Evaluate the individuals with an invalid fitness −
评估个人的无效适应度-
invalid_ind = [ind for ind in offspring if not ind.fitness.valid]
fitnesses = map(toolbox.evaluate, invalid_ind)
for ind, fit in zip(invalid_ind, fitnesses):
ind.fitness.values = fit
print('Evaluated', len(invalid_ind), 'individuals')
Now, replace population with next generation individual −
现在,用下一代个人代替人口-
population[:] = offspring
Print the statistics for the current generations −
打印当前几代的统计信息-
fits = [ind.fitness.values[0] for ind in population]
length = len(population)
mean = sum(fits) / length
sum2 = sum(x*x for x in fits)
std = abs(sum2 / length - mean**2)**0.5
print('Min =', min(fits), ', Max =', max(fits))
print('Average =', round(mean, 2), ', Standard deviation =',
round(std, 2))
print("\n- Evolution ends")
Print the final output −
打印最终输出-
best_ind = tools.selBest(population, 1)[0]
print('\nBest individual:\n', best_ind)
print('\nNumber of ones:', sum(best_ind))
Following would be the output:
Evolution process starts
Evaluated 500 individuals
- Generation 0
Evaluated 295 individuals
Min = 32.0 , Max = 45.0
Average = 40.29 , Standard deviation = 2.61
- Generation 1
Evaluated 292 individuals
Min = 34.0 , Max = 45.0
Average = 42.35 , Standard deviation = 1.91
- Generation 2
Evaluated 277 individuals
Min = 37.0 , Max = 45.0
Average = 43.39 , Standard deviation = 1.46
… … … …
- Generation 9
Evaluated 299 individuals
Min = 40.0 , Max = 45.0
Average = 44.12 , Standard deviation = 1.11
- Evolution ends
Best individual:
[0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1,
1, 0, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 0,
1, 0, 0, 1, 1, 1, 0, 0, 1, 0, 1]
Number of ones: 15
符号回归问题 (Symbol Regression Problem)
It is one of the best known problems in genetic programming. All symbolic regression problems use an arbitrary data distribution, and try to fit the most accurate data with a symbolic formula. Usually, a measure like the RMSE (Root Mean Square Error) is used to measure an individual’s fitness. It is a classic regressor problem and here we are using the equation 5x3-6x2+8x=1. We need to follow all the steps as followed in the above example, but the main part would be to create the primitive sets because they are the building blocks for the individuals so the evaluation can start. Here we will be using the classic set of primitives.
它是基因编程中最著名的问题之一。 所有符号回归问题都使用任意数据分布,并尝试使用符号公式拟合最准确的数据。 通常,使用诸如RMSE(均方根误差)之类的量度来衡量个人的健康状况。 这是一个经典的回归问题,在这里我们使用等式5x 3 -6x 2 + 8x = 1 。 我们需要按照上面示例中的所有步骤进行操作,但是主要部分是创建原始集,因为它们是个体的构建块,因此可以开始评估。 在这里,我们将使用经典的原语集。
The following Python code explains this in detail −
以下Python代码详细解释了这一点-
import operator
import math
import random
import numpy as np
from deap import algorithms, base, creator, tools, gp
def division_operator(numerator, denominator):
if denominator == 0:
return 1
return numerator / denominator
def eval_func(individual, points):
func = toolbox.compile(expr=individual)
return math.fsum(mse) / len(points),
def create_toolbox():
pset = gp.PrimitiveSet("MAIN", 1)
pset.addPrimitive(operator.add, 2)
pset.addPrimitive(operator.sub, 2)
pset.addPrimitive(operator.mul, 2)
pset.addPrimitive(division_operator, 2)
pset.addPrimitive(operator.neg, 1)
pset.addPrimitive(math.cos, 1)
pset.addPrimitive(math.sin, 1)
pset.addEphemeralConstant("rand101", lambda: random.randint(-1,1))
pset.renameArguments(ARG0 = 'x')
creator.create("FitnessMin", base.Fitness, weights = (-1.0,))
creator.create("Individual",gp.PrimitiveTree,fitness=creator.FitnessMin)
toolbox = base.Toolbox()
toolbox.register("expr", gp.genHalfAndHalf, pset=pset, min_=1, max_=2)
toolbox.expr)
toolbox.register("population",tools.initRepeat,list, toolbox.individual)
toolbox.register("compile", gp.compile, pset = pset)
toolbox.register("evaluate", eval_func, points = [x/10. for x in range(-10,10)])
toolbox.register("select", tools.selTournament, tournsize = 3)
toolbox.register("mate", gp.cxOnePoint)
toolbox.register("expr_mut", gp.genFull, min_=0, max_=2)
toolbox.register("mutate", gp.mutUniform, expr = toolbox.expr_mut, pset = pset)
toolbox.decorate("mate", gp.staticLimit(key = operator.attrgetter("height"), max_value = 17))
toolbox.decorate("mutate", gp.staticLimit(key = operator.attrgetter("height"), max_value = 17))
return toolbox
if __name__ == "__main__":
random.seed(7)
toolbox = create_toolbox()
population = toolbox.population(n = 450)
hall_of_fame = tools.HallOfFame(1)
stats_fit = tools.Statistics(lambda x: x.fitness.values)
stats_size = tools.Statistics(len)
mstats = tools.MultiStatistics(fitness=stats_fit, size = stats_size)
mstats.register("avg", np.mean)
mstats.register("std", np.std)
mstats.register("min", np.min)
mstats.register("max", np.max)
probab_crossover = 0.4
probab_mutate = 0.2
number_gen = 10
population, log = algorithms.eaSimple(population, toolbox,
probab_crossover, probab_mutate, number_gen,
stats = mstats, halloffame = hall_of_fame, verbose = True)
Note that all the basic steps are same as used while generating bit patterns. This program will give us the output as min, max, std (standard deviation) after 10 number of generations.
请注意,所有基本步骤都与生成位模式时使用的步骤相同。 该程序将在十代后提供给我们输出的最小值,最大值,std(标准偏差)。
python遗传算法