更多Python学习内容:ipengtao.com
数据库连接池在大多数Web应用中是一个至关重要的组件,有助于提高数据库访问效率和性能。本文将详细介绍如何使用Python创建和使用数据库连接池,通过丰富的示例代码和全面的讲解,帮助读者更好地理解和应用这一关键的技术。
连接池的基本原理
首先,深入了解数据库连接池的基本原理。了解连接池如何管理和分发数据库连接,以及如何通过复用连接减少连接建立的开销。
以下是一个简单的连接池实现示例:
import sqlite3
from queue import Queue
from threading import Lock
class ConnectionPool:
def __init__(self, max_connections):
self.max_connections = max_connections
self.connections = Queue(max_connections)
self.lock = Lock()
def _create_connection(self):
return sqlite3.connect('your_database.db')
def get_connection(self):
with self.lock:
if self.connections.qsize() < self.max_connections:
connection = self._create_connection()
self.connections.put(connection)
return self.connections.get()
def release_connection(self, connection):
self.connections.put(connection)
使用连接池进行数据库操作
接下来,演示如何在实际应用中使用连接池执行数据库操作。
以下是一个使用SQLite数据库的示例:
from contextlib import contextmanager
pool = ConnectionPool(max_connections=5)
@contextmanager
def get_connection():
connection = pool.get_connection()
try:
yield connection
finally:
pool.release_connection(connection)
def execute_query(query):
with get_connection() as conn:
cursor = conn.cursor()
cursor.execute(query)
result = cursor.fetchall()
return result
连接池的配置和性能优化
连接池的配置和性能优化是确保数据库连接池稳定、高效运行的关键因素。以下是一些关于连接池配置和性能优化的实践:
配置连接池参数
-
最大连接数(max_connections): 根据应用的并发访问量和数据库的容量,设置连接池能够支持的最大连接数。这可以防止连接池过载,导致性能下降。
-
最小空闲连接数(min_idle_connections): 维持一定数量的空闲连接,以减少连接创建和释放的开销,提高响应速度。
-
连接超时时间(connection_timeout): 设置连接的最大空闲时间,超过这个时间没有被使用的连接将被释放,防止连接长时间占用资源。
-
最大连接空闲时间(max_idle_time): 控制连接在连接池中的最大空闲时间,过长的空闲时间可能导致连接失效。
class ConnectionPool:
def __init__(self, max_connections, min_idle_connections, connection_timeout, max_idle_time):
# ...
pool = ConnectionPool(max_connections=10, min_idle_connections=5, connection_timeout=10, max_idle_time=3600)
处理连接超时
在高并发环境中,连接超时是一个常见的问题。通过设置合理的连接超时时间,可以避免连接长时间占用而不被使用的情况。当连接超时时,连接池应当及时释放连接,并在必要时重新创建新的连接。
class ConnectionPool:
def get_connection(self):
with self.lock:
if self.connections.qsize() < self.max_connections:
connection = self._create_connection()
self.connections.put((connection, time.time()))
return self.connections.get()
def release_connection(self, connection):
self.connections.put((connection, time.time()))
def _cleanup_connections(self):
while not self.connections.empty():
connection, last_used_time = self.connections.get()
if time.time() - last_used_time > self.max_idle_time:
connection.close()
else:
self.connections.put((connection, last_used_time))
实现连接的健康检查
通过定期对连接进行健康检查,可以确保连接池中的连接都是可用的。健康检查可以包括简单的PING命令或执行一些简单的SQL查询来验证连接的有效性。
class ConnectionPool:
def _health_check(self):
while True:
time.sleep(60) # 健康检查间隔
with self.lock:
for _ in range(self.connections.qsize()):
connection, _ = self.connections.get()
try:
# 执行健康检查,例如执行一些SQL查询
cursor = connection.cursor()
cursor.execute("SELECT 1")
except Exception as e:
# 连接不可用,重新创建连接
connection = self._create_connection()
finally:
self.connections.put((connection, time.time()))
日志记录和监控
在连接池中添加适当的日志记录,以便能够在需要时追踪连接的获取和释放情况。监控连接池的性能指标,例如连接池中的连接数、空闲连接数、使用率等,以便及时发现潜在问题。
import logging
class ConnectionPool:
def __init__(self, max_connections, min_idle_connections, connection_timeout, max_idle_time):
# ...
self.logger = logging.getLogger("connection_pool")
def get_connection(self):
self.logger.info("Connection requested")
# ...
def release_connection(self, connection):
self.logger.info("Connection released")
# ...
与不同数据库的适配
在连接池的实现中,与不同数据库的适配是确保系统能够在多种数据库系统中无缝运行的重要步骤。
以下是一个关于如何进行不同数据库适配的示例:
import psycopg2
import pymysql
class ConnectionPool:
def __init__(self, driver, max_connections, **kwargs):
self.driver = driver
self.max_connections = max_connections
self.connections = Queue(max_connections)
self.lock = Lock()
self.kwargs = kwargs
def _create_connection(self):
if self.driver == 'postgres':
return psycopg2.connect(**self.kwargs)
elif self.driver == 'mysql':
return pymysql.connect(**self.kwargs)
# Add more adaptors as needed
else:
raise ValueError(f"Unsupported database driver: {self.driver}")
# Example usage
postgres_pool = ConnectionPool(driver='postgres', max_connections=10, user='user', password='password', host='localhost', port=5432, database='mydb')
mysql_pool = ConnectionPool(driver='mysql', max_connections=10, user='user', password='password', host='localhost', port=3306, database='mydb')
通过在ConnectionPool
类中添加适配不同数据库的逻辑,可以根据传入的driver
参数选择合适的数据库连接方式。这里的示例中包含了PostgreSQL和MySQL的适配,你可以根据实际需要添加更多数据库的适配。
注意,每个数据库驱动都有其独特的连接参数,上述示例中的**kwargs
允许传递任意数量的关键字参数给数据库连接函数,以满足不同数据库的连接需求。
异步连接池
在异步Web框架中,异步连接池是确保应用程序能够高效处理并发请求的关键组件。以下是如何使用asyncio
库创建异步数据库连接池的示例:
import asyncio
import aiomysql
class AsyncConnectionPool:
def __init__(self, max_connections, loop, **kwargs):
self.max_connections = max_connections
self.connections = asyncio.Queue(max_connections)
self.loop = loop
self.kwargs = kwargs
async def _create_connection(self):
return await aiomysql.connect(loop=self.loop, **self.kwargs)
async def get_connection(self):
if self.connections.qsize() < self.max_connections:
connection = await self._create_connection()
await self.connections.put(connection)
return await self.connections.get()
async def release_connection(self, connection):
await self.connections.put(connection)
# Example usage
async def example_usage():
loop = asyncio.get_event_loop()
async_pool = AsyncConnectionPool(
max_connections=10,
loop=loop,
user='user',
password='password',
host='localhost',
port=3306,
db='mydb'
)
async with async_pool.get_connection() as conn:
# Perform database operations using conn
pass
loop.run_until_complete(async_pool.release_connection(conn))
loop = asyncio.get_event_loop()
loop.run_until_complete(example_usage())
在这个例子中,使用了aiomysql
库来创建异步数据库连接。连接的获取和释放通过异步队列实现,确保了在异步环境中的高效操作。get_connection
和release_connection
方法都是异步的,与async with
结构一起使用以确保在使用完连接后能够正确释放。
安全性和异常处理
在连接池的实现中,确保安全性和有效地处理异常是非常重要的。以下是一些关于安全性和异常处理的最佳实践:
1. 异常处理
在连接池中,可能会遇到各种异常情况,例如数据库连接失败、连接超时等。在代码中添加适当的异常处理,以确保系统在异常情况下能够正常工作。
class ConnectionPool:
def _create_connection(self):
try:
# 尝试创建数据库连接
# ...
except Exception as e:
# 处理连接创建失败的情况
raise ConnectionError(f"Failed to create a database connection: {e}")
2. 安全性考虑
确保连接池的配置和使用符合安全性要求。避免在代码中硬编码敏感信息,例如数据库密码。在生产环境中,使用安全的配置管理工具或环境变量来存储敏感信息。
import os
class ConnectionPool:
def __init__(self):
self.user = os.getenv('DB_USER')
self.password = os.getenv('DB_PASSWORD')
# ...
3. 连接池日志记录
在连接池的关键操作中添加适当的日志记录,以便在需要时进行故障排除。日志记录可以包括连接的获取、释放以及异常情况的记录。
import logging
class ConnectionPool:
def __init__(self):
self.logger = logging.getLogger("connection_pool")
def get_connection(self):
self.logger.info("Connection requested")
# ...
def release_connection(self, connection):
self.logger.info("Connection released")
# ...
4. 监控连接池性能
在生产环境中,连接池的性能监控是必不可少的。通过记录关键指标,例如连接数、空闲连接数、连接获取和释放时间等,可以及时发现潜在的性能问题。
import time
class ConnectionPool:
def __init__(self):
self.metrics = {'connections': 0, 'idle_connections': 0}
def get_connection(self):
# ...
self.metrics['connections'] += 1
def release_connection(self, connection):
# ...
self.metrics['idle_connections'] += 1
def report_metrics(self):
print(f"Total connections: {self.metrics['connections']}, Idle connections: {self.metrics['idle_connections']}")
通过定期调用report_metrics
方法,可以实时了解连接池的性能状况。
pool = ConnectionPool()
pool.get_connection()
pool.release_connection(connection)
pool.report_metrics()
总结
通过本文,读者将深入了解数据库连接池的实现原理和在Python中的具体应用。丰富的示例代码和全面的讲解将帮助读者更好地理解并成功实现一个高效可靠的数据库连接池。希望这篇文章能够成为读者学习和应用数据库连接池的有力指南。
感兴趣的小伙伴,赠送全套Python学习资料,包含面试题、简历资料等具体看下方。
一、Python所有方向的学习路线
Python所有方向的技术点做的整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照下面的知识点去找对应的学习资源,保证自己学得较为全面。
二、Python必备开发工具
工具都帮大家整理好了,安装就可直接上手!
三、最新Python学习笔记
当我学到一定基础,有自己的理解能力的时候,会去阅读一些前辈整理的书籍或者手写的笔记资料,这些笔记详细记载了他们对一些技术点的理解,这些理解是比较独到,可以学到不一样的思路。
四、Python视频合集
观看全面零基础学习视频,看视频学习是最快捷也是最有效果的方式,跟着视频中老师的思路,从基础到深入,还是很容易入门的。
五、实战案例
纸上得来终觉浅,要学会跟着视频一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
六、面试宝典
简历模板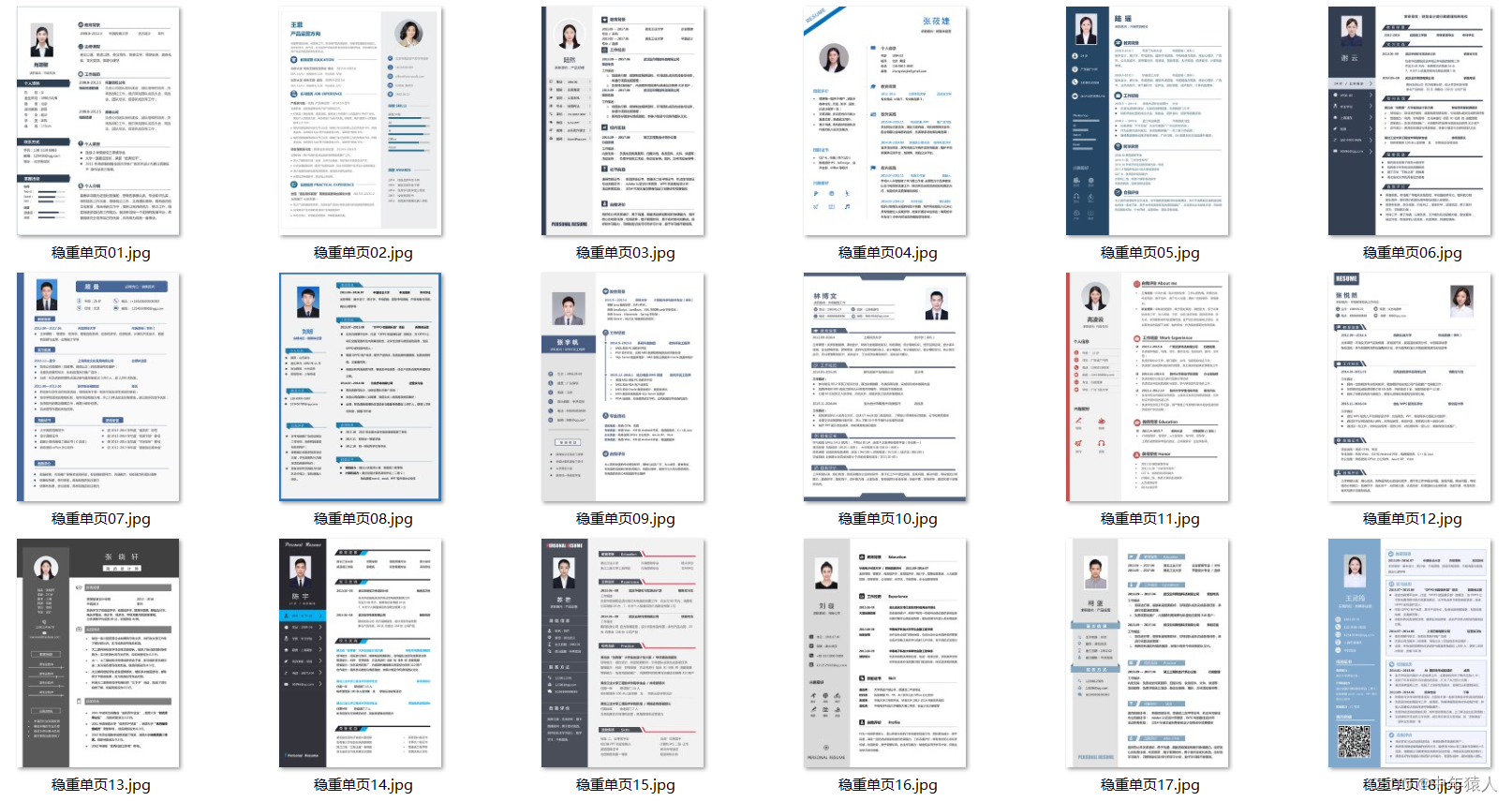
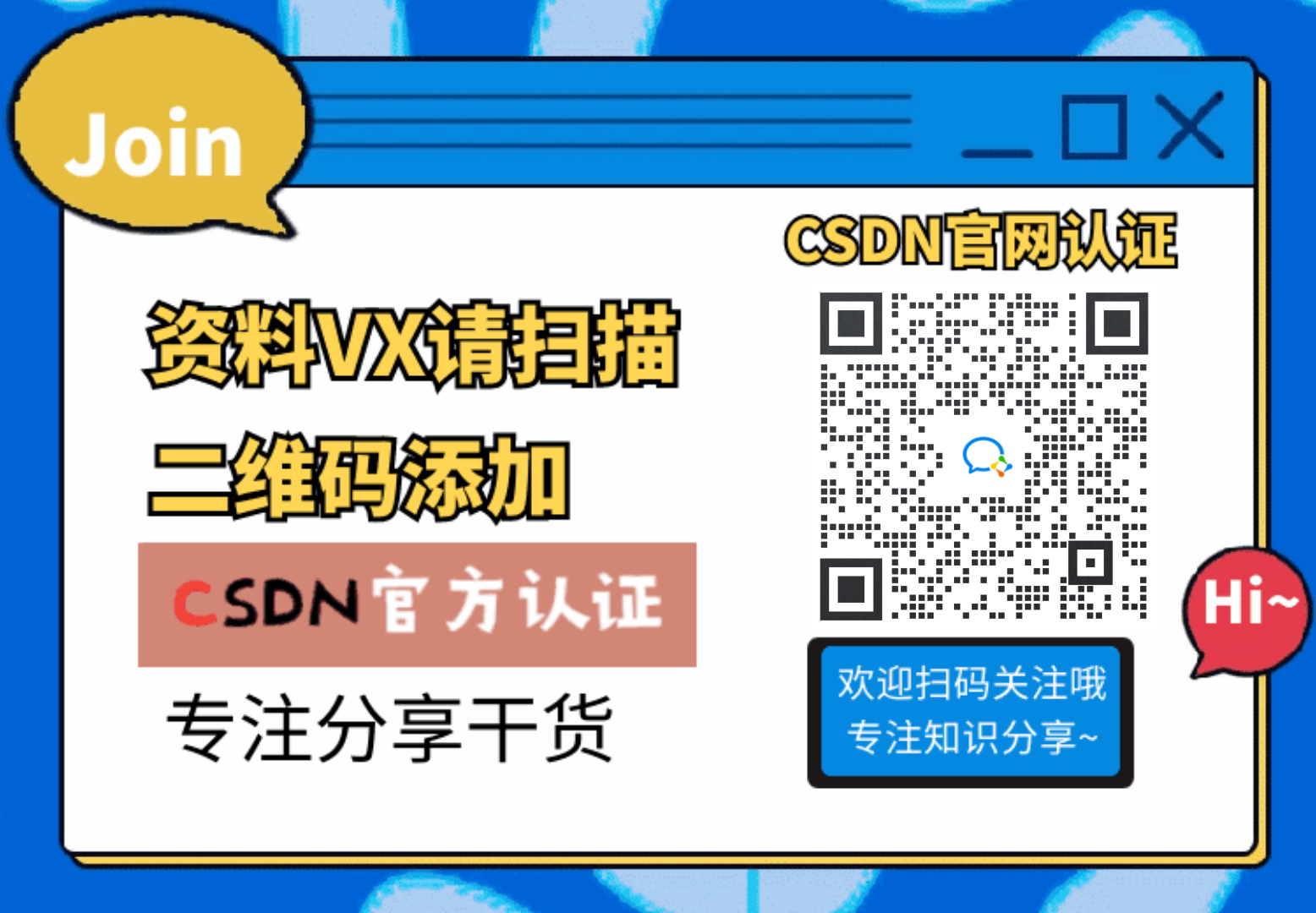