目录
0. 前言
- 流程控制语句:控制程序的执行流程。
- 顺序结构:程序默认流程
分支结构:if、switch
循环结构:for、while、do…while
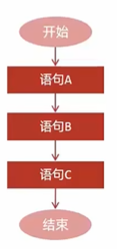
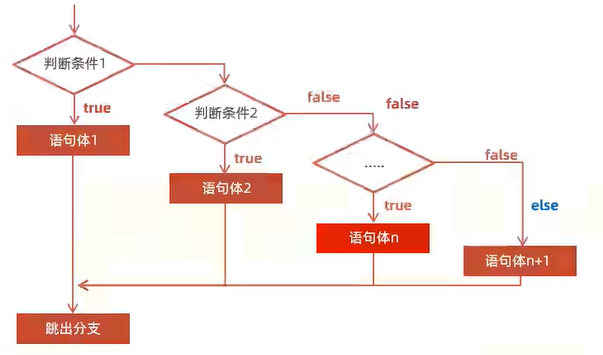
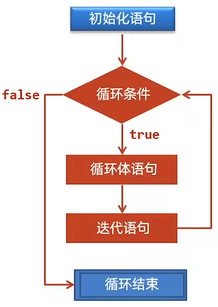
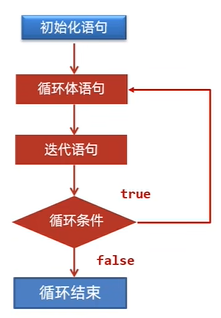
顺序(左)if分支(中)for和while循环(右1)do…while循环(右2)
1. 顺序结构
顺序结构:JAVA的基本结构,除非特别指明,否则就按照顺序一句一句执行。
public static void main(String[] args) {
System.out.println("Hello1");
System.out.println("Hello2");
System.out.println("Hello3");
System.out.println("Hello4");
System.out.println("Hello5");
}
//按照自上而下的顺序执行!依次输出。
2. 分支结构
2.1 if
-
作用:根据条件表达式判定的结果(true、false)决定执行某个分支。
-
特点:适合做区间匹配,在功能上远大于switch。
-
格式
-
执行流程
- 语句1
条件表达式的值为true,执行语句体;否则不执行。 - 语句2
①条件表达式的值为true,执行语句体1。
②否则转入else,执行语句体2。 - 语句3
①条件表达式1 的值为true,执行语句体1。
②否则转入else if,条件表达式2 的值为true,执行语句体2。
③否则转入else if,条件表达式3 的值为true,执行语句体3。
④条件表达式都不满足,转入else,执行语句体n+1;
- 语句1
public static void main(String[] args) {
//IF
// 格式1:if
// int beat = 118;
// if (beat < 60 || beat > 100){
// System.out.println("你的心跳为:" + beat +",不太正常!");
// }
// 格式2:if else
// int beat = 80;
// if (beat < 60 || beat > 100){
// System.out.println("你的心跳为:" + beat +",不太正常!");
// }else{
// System.out.println("你的心跳为:" + beat +",正常!");
// }
// 格式3:if else if else if else
int beat = 30;
if (beat < 60){
System.out.println("你的心跳为:" + beat +",过低!");
}else if (beat > 100){
System.out.println("你的心跳为:" + beat +",过高!");
}else{
System.out.println("你的心跳为:" + beat +",正常!");
}
}
2.2 switch
- 根据与表达式匹配条件去执行分支,结构清晰,格式良好。
- 特点:适合做值匹配。
- 格式
- 执行流程:
①先执行表达式的值,与case后的值进行匹配。
②匹配哪个case的值为true就执行哪个case, 遇到break就跳出switch分支。
③如果case后的值都不匹配则执行default代码。
public static void main(String[] args) {
// switch
System.out.println("please input the week:");
Scanner sc = new Scanner(System.in);
String weekday = sc.next();
switch (weekday){
case "Monday":
System.out.println("the first day!");
break;
case "Tuesday":
System.out.println("the second day!");
break;
case "Wednesday":
System.out.println("the third day!");
break;
case "Thursday":
System.out.println("the fourth day!");
break;
case "Friday":
System.out.println("the fifth day!");
break;
default:
System.out.println("take a rest!");
}
}
2.3 switch的注意事项
- 表达式类型只能是byte、short、 int、char; JDK5开始支持枚举, JDK7开始支持String、不支持double、float. long。
- case后的值不允许重复,且只能是字面量, 不能是变量。
- 写break,否则会出现穿透现象。
enum Day {
one, two, three;
}
public static void main(String[] args) {
// switch注意事项
// 1.表达式类型 byte、short、 int、char、枚举、String。不支持double、float、long。
Day daily = Day.one;
switch (daily){
}
byte a = 1;
switch (a + 1){
}
short b = 2;
switch (b + 1){
}
int c = 3;
switch (c+1){
}
char d = 'd';
switch (d){
}
String e = "one";
switch (e){
}
// 2.case后的值不允许重复,且只能是字面量、不能是变量。
int i = 3;
switch (i){
case 3: //报错!!!case后的值不允许重复
break;
case 3: //报错!!!case后的值不允许重复
break;
case a: //报错!!!case后的值只能是字面量
break;
}
// 3.写**break**,否则会出现穿透现象。
int j = 3;
switch (j){
case 3: //没有写**break**,出现穿透现象,执行case 3 和case 4 和case 5。
System.out.println(3); //3
case 4:
System.out.println(4); //4
case 5:
System.out.println(5); //5
break;
}
}
2.4 switch穿透性
- case中 没有写break,会产生switch穿透。
- 作用:多个case分支的执行代码一样时,可以用穿透性把流
程集中到同一处进行处理,这样可以简化代码。
3. 循环结构
3.1 for循环
- 控制代码反复执行多次
- 格式
- 执行流程
①首先执行初始化条件。
②判断循环条件(条件判断语句):返回true,执行到循环体语句。 然后执行迭代语句(条件控制语句)。
③不断重复②,直到判断循环条件:返回false,循环立即结束!
public static void main(String[] args) {
for (int i = 0; i < 10; i++){
System.out.println(i); // 0 1 2 3 4 5 6 7 8 9
}
for (int i1 = 0; i1 < 10; i1 += 2){
System.out.println(i1); // 0 2 4 6 8
}
}
- 案例
- 求和:1~5
public static void main(String[] args) {
//求和1~5
int sum = 0;
for (int a = 1; a <= 5; a++){
sum += a;
}
System.out.println(sum); //15
- 求和1~10之间的奇数
public static void main(String[] args) {
//求和1~10之间的奇数
int sum = 0; //求和定义在循环外面
for (int i = 1; i <= 10; i += 2) {
sum += i;
System.out.println(i);//1 3 5 7 9
}
System.out.println(sum);//25
}
- 需求:在控制台输出所有的“水仙花数” 。水仙花数,指的是一个三位数 + 个位、十位、百位的数字立方和等于原数。
例如153 3*3*3 + 5*5*5 + 1*1*1 = 153
思路:- 获取所有的三位数,准备进行筛选,最小的三位数为100,最大的三位数为999,使用for循环获取
- 获取每一个三位数的个位,十位,百位,做if语句判断是否是水仙花数
System.out.println("the flower number:");
for (int i = 100; i < 1000; i++) {
int a = i % 10; //个
int b = i / 10 % 10; //十
int c = i / 100; //百
if (Math.pow(a, 3) + Math.pow(b, 3) + Math.pow(c, 3) == i){
System.out.println(i); //153 370 371 407
}
}
3.2 while循环
- 先判断循环条件,再执行
- 格式
- 执行流程
①执行初始化语句
②判断循环条件(条件判断语句),如果是false,循环结束;如果是true,继续
③执行循环体语句
④执行迭代语句(条件控制语句)
⑤回到②继续
public static void main(String[] args) {
//while
int i = 0;
while (i < 3){ //条件判断语句(循环条件)
System.out.println(i);
i++; //条件控制语句(迭代语句)
}
}
- for 和 while 区别
- for:知道循环几次
- while:不知道循环几次,如下例的珠穆朗玛峰,条件判断语句为(paperHt != peakHt)
- 案例
需求:世界最高山峰是珠穆朗玛峰(8844.43米=8844430毫米),假如有一张足够大的纸,厚度是0.1毫米。折叠多少次,可以折成珠穆朗玛峰的高度?
public static void main(String[] args) {
double peakHt = 8844430;
double paperHt = 0.1;
int count = 0;
while(paperHt <= peakHt){
paperHt *= 2;
count ++;
}
System.out.println("需要折叠次数:" + count); //需要折叠次数:24
}
3.3 do…while循环
- 先执行,再判断循环条件。
- 特点:一定会先执行一次循环体。
- 格式
- 执行流程
① 执行初始化语句
② 执行循环体语句
③ 执行迭代语句(条件控制语句)
④ 执行循环条件(条件判断语句),如果是false,循环结束;如果是true,继续执行
⑤ 回到②继续
public static void main(String[] args) {
int i = 0;
do{
System.out.println(i); //0 1 2
i++;
}while(i < 3);
}
- 对比 for while do…while
- ① for和while循环:先判断后执行
先判断条件是否成立,再决定是否执行循环体
② do…while循环:先执行后判断
先执行一次循环体,再判断条件是否成立,是否继续执行循环体
- ① for循环:通常用于知道循环几次
条件控制语句所控制的自增变量,因为归属for循环的语法结构中,在for循环结束后,就不能再次被访问到了
② while循环:不知道循环几次
条件控制语句所控制的自增变量,对于while循环来说不归属其语法结构中,在while循环结束后,该变量还可以继续使用
public static void main(String[] args) { //IF for (int i = 0; i < 3; i++) { System.out.println(i); } System.out.println(i);// 报错!循环体外,超出变量i作用范围 } public static void main(String[] args) { //while int i = 0; while (i < 3){ //条件判断语句(循环条件) System.out.println(i); i++; //条件控制语句(迭代语句) } System.out.println(i); }
- ① for和while循环:先判断后执行
3.4 死循环
- 一直循环的执行下去,如果没有干预不会停止下来
- 格式
public static void main(String[] args) {
for(;;){
System.out.println("for");
}
while(true){
System.out.println("while");
}
do {
System.out.println("do…while");
}while (true);
}
- 案例
需求:系统密码是520,请用户不断输入密码验证,验证失败输出“密码错误”,验证成功则输出“欢迎进入系统!”
int password = 520;
Scanner sc = new Scanner(System.in);
System.out.println("请输入3位密码登录:");
while(true){
int passkey = sc.nextInt();
if(passkey != password){
System.out.println("密码错误");
}else{
break;
}
}
System.out.println("欢迎进入系统!");
3.5 循环嵌套
- 循环中又包含循环
- 格式
- 特点
外部循环每循环一次,内部循环全部执行完一次
public static void main(String[] args) {
for (int i = 0; i < 3; i++) {
System.out.println("---i---" + i);
for (int j = 10; j < 13; j++) {
System.out.println("j:" + j);
}
}
}
- 案例
需求:输出四行五列的*
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 5; j++) {
System.out.print('*');
}
System.out.println();
}
4. 跳转关键字
4.1 break
- 跳出,并结束 所在循环的执行
- 只能用于结束所在循环,或者结束所在switch分支的执行。
- 案例
public static void main(String[] args) {
//预计7天封控,第三天下发文件,疫情从地球消失了
for (int i = 1; i < 8; i++) {
System.out.println("封控:" + i); //封控:1 封控:2 封控:3
if (i == 3){
break;
}
}
System.out.println("解封"); //解封
}
4.2 continue
- 跳过本次循环,进入下次循环
- 注意: continue只能在循环中进行使用!
- 案例
//预计7天封控,第三天下发文件,你可以出门采购一次
for (int i = 1; i < 8; i++) {
if (i == 3){
continue;
}
System.out.println("封控:" + i); //封控:1 封控:2 封控:4 封控:5 封控:6 封控:7
}
5. 案例-随机数Random类
- Random类似Scanner,也是Java提供好的API,
- 功能:产生随机数。
- 使用步骤
① 导入包
import java.util.Random;
② 创建对象
Random r = new Random();
③ 产生随机数
int num = r.nextInt(10); //0~9
注意事项: nextInt(n):生成0~n-1随机数
技巧: nextInt(n)+1:生成1~n随机数
a~ b的随机数:a-a ~ b-a,nextInt(b-a+1)+a
//1. 导包(自动导入)
import java.util.Random;
public class RandomTest {
//random
public static void main(String[] args) {
//2. 创建对象
Random rd = new Random();
//3.生成随机数
int num = rd.nextInt(5);
System.out.println(num);
}
}
//random生成随机数3~5--->减3:0~2--->[0,3)+3
public static void main(String[] args) {
Random rd = new Random();
int num = rd.nextInt(3)+3;
System.out.println(num);
}
- 技巧
- 案例
需求:程序自动生成一个1-100之间的数字,使用程序实现猜出这个数字是多少?
当猜错的时候根据不同情况给出相应的提示:
A. 如果猜的数字比真实数字大,提示你猜的数据大了
B. 如果猜的数字比真实数字小,提示你猜的数据小了
C. 如果猜的数字与真实数字相等,提示恭喜你猜中了
public static void main(String[] args) {
Random rd = new Random();
int num = rd.nextInt(100)+1;
Scanner sc = new Scanner(System.in);
System.out.println("please begin:");
int say = sc.nextInt();
while(say != num){
if (say > num){
System.out.println("more big,one more time");
say = sc.nextInt();
}else {
System.out.println("more little,one more time");
say = sc.nextInt();
}
}
System.out.println("finish");
或者
import java.util.Scanner;
import java.util.Random;
public class Test {
public static void main(String[] args){
// 1. 准备Random和Scanner对象, 分别用于产生随机数和键盘录入
Random r = new Random();
Scanner sc = new Scanner(System.in);
// 2. 使用Random产生一个1-100之间的数, 作为要猜的数
int randomNum = r.nextInt(100) + 1;
System.out.println("请输入您猜的数据:");
// 5. 以上内容需要多次进行, 但无法预估用户输入几次可以猜测正确, 使用while(true)死循环包裹
while(true){
// 3. 键盘录入用户猜的的数据
int num = sc.nextInt();
// 4. 使用录入的数据(用户猜的数据)和随机数(要猜的数据)进行比较, 并给出提示
if(num > randomNum){
System.out.println("猜大了");
}else if(num < randomNum){
System.out.println("猜小了");
}else{
// 6. 猜对之后, break结束.
System.out.println("恭喜,猜中了");
break;
}
}
}
}