制作:哈哈
1.人人对战
2.人机对战
3胜负判定 (横向 纵向 左下至右上 左上至右下)
4 合法性检测 (重复落子 超出边界)
部分数据定义无用(懒得改)
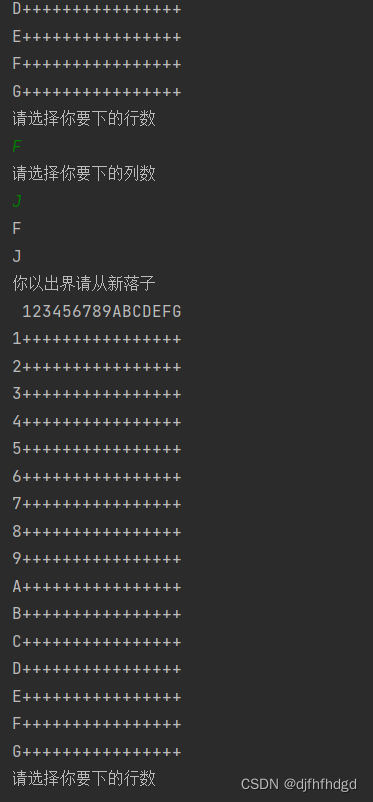
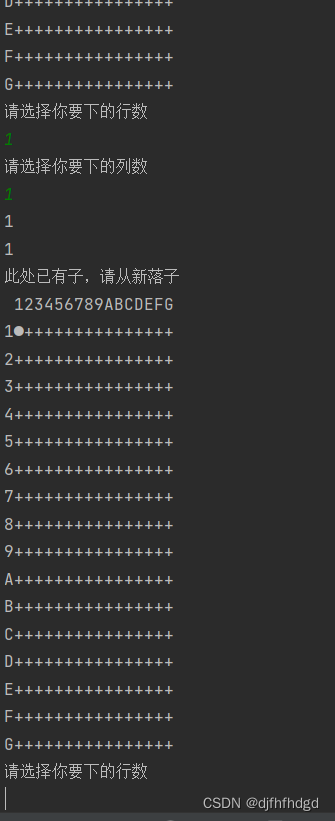
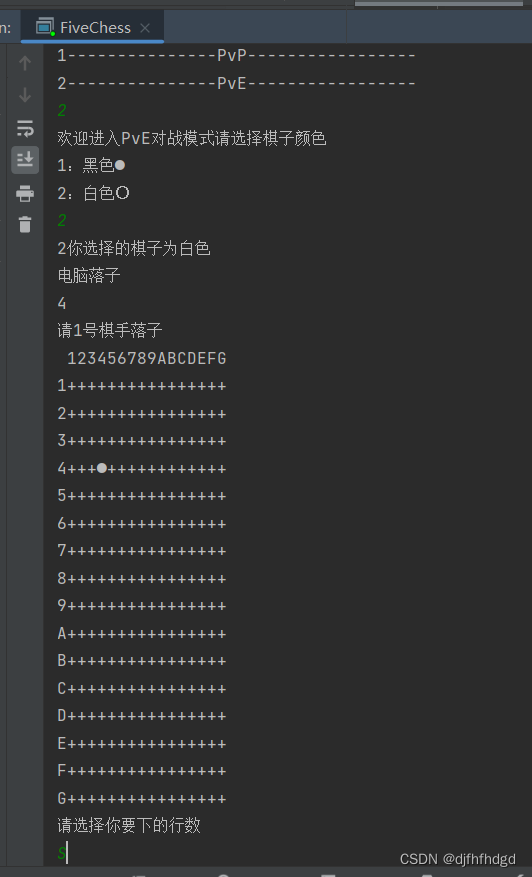
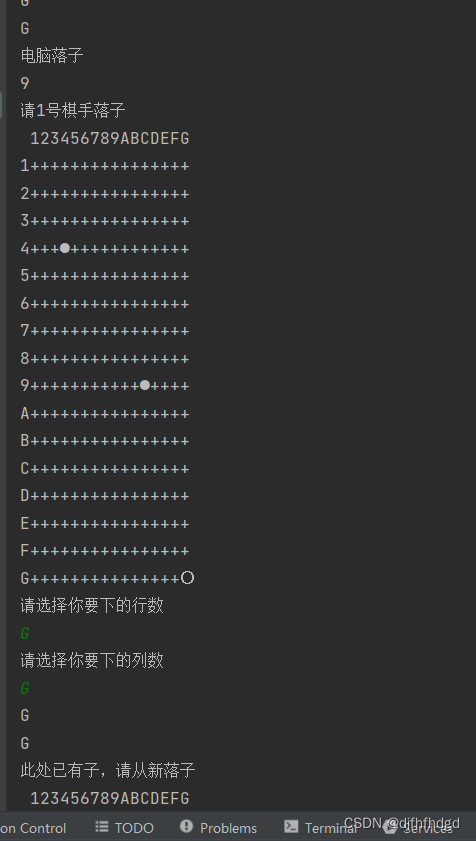
/**制作人:哈哈
* */
/** 源码仅供参考借鉴使用
* */
/**
* 1.人人对战
* 2.人机对战
* 3胜负判定 (横向 纵向 左下至右上 左上至右下)
* 4 合法性检测 (重复落子 超出边界)
* 部分数据定义无用(懒得改)
* */
import java.util.Objects;
import java.util.Scanner;
import static javafx.scene.paint.Color.color;
public class FiveChess {
// 定义棋盘的大小
public static int BOARD_SIZE = 17;
// 定义一个二维数组来充当棋盘
public static char[][] board = new char[BOARD_SIZE][BOARD_SIZE];
String black = "●"; // 黑子
String white = "○"; // 白子
public void initBoard() {
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[i].length; j++) { /**数组填充棋盘*/
board[i][j] = '+';
}
}
}
// public static char[] num = new char[]{'1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F', 'G'};
public static void rowcolumn() { /**board.length =17,向数组中插入坐标*/
char[] num = new char[]{'1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F', 'G'};
for (int i = 1; i < board.length; i++) {
board[0][i] = num[i - 1];
board[0][0] = ' ';
board[i][0] = num[i - 1];
}
}
public void printBoard() {
// 打印毎个数组元素
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board.length; j++) { /**打印棋盘*/
// 打印数组元素后不换行
System.out.print(board[i][j]);
}
// 毎打印完一行数组元素后输出一个换行符
System.out.print("\n");
}
}
public static void main(String[] args) {/**主函数*/
FiveChess gb = new FiveChess();
gb.initBoard();//加载棋盘
gb.rowcolumn();//加载棋盘坐标
System.out.println(board.length);
System.out.println("---------欢迎进入五指棋对战游戏----------");
System.out.println("-------------请选择对战模式-------------");
System.out.println("1---------------PvP-----------------");
System.out.println("2---------------PvE-----------------");
//System.out.println(board.length); 测试
int f = 0;
Scanner sc = new Scanner(System.in);
f = sc.nextInt();
gb.mood(f);
}
public void mood(int f) {/**模式选着函数*/
FiveChess gb = new FiveChess();
if (f == 1) {
System.out.println("欢迎进入PvP对战模式");
System.out.println("请1号棋手选择棋子颜色");
System.out.println("你始终为1号棋手");
String color = choiceColor();
gb.PvP(color);
} else if (f == 2) {
System.out.print("欢迎进入PvE对战模式");
// gb.text(); //数据测试调用
gb.PvE();
}
}
public boolean isOut(String row, String column) { /**边界判断
*/
System.out.println(row);
System.out.println(column);
if ((Objects.equals(row, "1") || Objects.equals(row, "2") || Objects.equals(row, "3") || Objects.equals(row, "4") ||
Objects.equals(row, "5") || Objects.equals(row, "6") || Objects.equals(row, "7") || Objects.equals(row, "8") ||
Objects.equals(row, "9") || Objects.equals(row, "A") || Objects.equals(row, "B") || Objects.equals(row, "C") ||
Objects.equals(row, "D") || Objects.equals(row, "E") || Objects.equals(row, "F") || Objects.equals(row, "G")) &&
(Objects.equals(column, "1") || Objects.equals(column, "2") || Objects.equals(column, "3") || Objects.equals(column, "4") ||
Objects.equals(column, "5") || Objects.equals(column, "6") || Objects.equals(column, "7") || Objects.equals(column, "8") ||
Objects.equals(column, "9") || Objects.equals(column, "A") || Objects.equals(column, "B") || Objects.equals(column, "C") ||
Objects.equals(column, "D") || Objects.equals(column, "E") || Objects.equals(column, "F") || Objects.equals(column, "G"))) {
return false;
} else {
return true;
}
}
public static boolean isOk(int x, int y) {
if (String.valueOf(board[x][y]).equals("●") || String.valueOf(board[x][y]).equals("○")) {
return false;
} /**占位判断*/
return true;
}
public void down(String color) { /**落子*/ /**每次执行时都会先检查坐标是否合法*/
FiveChess gb = new FiveChess();
gb.printBoard();
Scanner input = new Scanner(System.in);
System.out.println("请选择你要下的行数");
String row = input.next();
System.out.println("请选择你要下的列数");
String column = input.next();
//System.out.println(gb.isOut(row,column));//函数功能检测
if (gb.isOut(row, column)) {
System.out.println("你以出界请从新落子");
gb.down(color);
}
for (int i = 1; i < board.length; i++) { /**根据输入的字符反推数组位置*/
for (int j = 1; j < board.length; j++) {
String row1 = new String(String.valueOf(board[0][i]));
String column1 = new String(String.valueOf(board[j][0]));
if (Objects.equals(row1, row) && Objects.equals(column1, column)) {
// System.out.println(i);//函数功能检测
// System.out.println(j);
int x = i;
int y = j;
if (isOk(x, y)) {
if (color == black) {
board[x][y] = '●';
} else if (color == white) {
board[x][y] = '○';
}
} else if (!isOk(x, y)) {
System.out.println("此处已有子,请从新落子");
gb.down(color);
}
break; /**找到坐标跳出循环*/
}
}
}
}
/**
* public void people(String color) {
* FiveChess gb = new FiveChess();
* if(PvP(color)=="1"){
* System.out.println("请1号棋手落子");
* gb.down(black);
* System.out.println("请2号棋手落子");
* gb.down(white);
* gb.people(color);
* } else if (PvP(color)=="2") {
* System.out.println("请2号棋手落子");
* gb.down(black);
* System.out.println("请1号棋手落子");
* gb.down(white);
* gb.people(color);
* }
* }
*/
public String PvP(String color) { /**PVP模式*/
FiveChess gb = new FiveChess();
// System.out.println("请1号棋手选择棋子颜色");
//String color = choiceColor();
String peoplennumber = null;
System.out.println(color);
if (Objects.equals(color, black)) {
for(int i=0;;i++){ /**胜利后跳出无尽循环,结束游戏*/
System.out.println("请1号棋手落子");
gb.down(black);
peoplennumber = String.valueOf(1);
if (gb.isWin(color, peoplennumber) == true) {
gb.printBoard();
break;
}
System.out.println("请2号棋手落子");
gb.down(white);
if (gb.isWin(color, peoplennumber) == true) {
gb.printBoard();
break;
}
color = black;
//gb.PvP(color);
}
} else if (Objects.equals(color, white)) {
for(int j=0;;j++) {
System.out.println("请2号棋手落子");
gb.down(black);
peoplennumber = String.valueOf(2);
if (gb.isWin(color, peoplennumber) == true) {
gb.printBoard();
break;
}
System.out.println("请1号棋手落子"); /**黑子先下,第一次落子无输赢,不判断*/
gb.down(white);
if (gb.isWin(color, peoplennumber) == true) {
gb.printBoard();
break;
}
color = white;
//gb.PvP(color);
}
}
return peoplennumber;
}
public String choiceColor() { /**颜色选着*/
FiveChess gb = new FiveChess();
System.out.println("1:黑色●");
System.out.println("2:白色○");
Scanner input = new Scanner(System.in);
String choice = input.next();
String color = null;
System.out.print(choice);
if (Integer.parseInt(choice) == 1) {/**数据类型转换*/
System.out.println("你选择的棋子为黑色");
color = black;
} else if (Integer.parseInt(choice) == 2) {
System.out.println("你选择的棋子为白色");
color = white;
} else {
gb.choiceColor();
}
// System.out.println(color);//函数功能检测
return color;
}
public boolean isWin(String color, String peoplennumber) { /**输赢判断*/
/**
* 横向检测
* */boolean win = false;
for (int x = 1; x < board.length - 4; x++) {
for (int y = 1; y < board.length - 4; y++) {
if (board[x][y] == '●'
&& board[x][y + 1] == '●'
&& board[x][y + 2] == '●'
&& board[x][y + 3] == '●'
&& board[x][y + 4] == '●') {
System.out.println("黑棋获胜");
win = true;
break;
} else if (board[x][y] == '○'
&& board[x][y + 1] == '○'
&& board[x][y + 2] == '○'
&& board[x][y + 3] == '○'
&& board[x][y + 4] == '○') {
System.out.println("白棋获胜");
win = true;
break;
}else if (board[x][y] == '●'
&& board[x+1][y] == '●'
&& board[x+2][y] == '●'
&& board[x+3][y] == '●'
&& board[x+4][y] == '●') {
System.out.println("黑棋获胜");
win = true;
break;
}else if (board[x][y] == '○'
&& board[x+1][y] == '○'
&& board[x+2][y] == '○'
&& board[x+3][y] == '○'
&& board[x+4][y] == '○') {
System.out.println("白棋获胜");
win = true;
break;
}
}
}
for (int x = 1; x < board.length; x++) {/**纵向检测*/
for (int y = 1; y < board.length - 4; y++) {
if (board[x][y] == '●'
&& board[x][y + 1] == '●'
&& board[x][y + 2] == '●'
&& board[x][y + 3] == '●'
&& board[x][y + 4] == '●') {
System.out.println("黑棋获胜");
win = true;
break;
} else if (board[x][y] == '○'
&& board[x][y + 1] == '○'
&& board[x][y + 2] == '○'
&& board[x][y + 3] == '○'
&& board[x][y + 4] == '○') {
System.out.println("白棋获胜");
win = true;
break;
}
}
}
for (int x = 1; x < board.length-4; x++) {
for (int y = 1; y < board.length; y++) {
if (board[x][y] == '●'
&& board[x + 1][y] == '●'
&& board[x + 2][y] == '●'
&& board[x + 3][y] == '●'
&& board[x + 4][y] == '●') {
System.out.println("黑棋获胜");
win = true;
break;
} else if (board[x][y] == '○'
&& board[x + 1][y] == '○'
&& board[x + 2][y] == '○'
&& board[x + 3][y] == '○'
&& board[x + 4][y] == '○') {
System.out.println("白棋获胜");
win = true;
break;
}
}
}
for (int x = 1; x < board.length-4; x++) {
for (int y = 1; y < board.length-4; y++) {
if (board[x][y] == '●'
&& board[x + 1][y+1] == '●'
&& board[x + 2][y+2] == '●' /**从左上到右下*/
&& board[x + 3][y+3] == '●'
&& board[x + 4][y+4] == '●') {
System.out.println("黑棋获胜");
win = true;
break;
} else if (board[x][y] == '○'
&& board[x + 1][y+1] == '○'
&& board[x + 2][y+2] == '○'
&& board[x + 3][y+3] == '○'
&& board[x + 4][y+4] == '○') {
System.out.println("白棋获胜");
win = true;
break;}
}
}
for (int x = board.length-1; x >4; x--) {
for (int y = 1; y < board.length-4; y++) {
if (board[x][y] == '●'
&& board[x-1][y+1] == '●'
&& board[x-2][y+2] == '●' /**从左下到右上*/
&& board[x-3][y+3] == '●'
&& board[x-4][y+4] == '●') {
System.out.println("黑棋获胜");
win = true;
break;}
else if (board[x][y] == '○'
&& board[x-1][y+1] == '○'
&& board[x-2][y+2] == '○'
&& board[x-3][y+3] == '○'
&& board[x-4][y+4] == '○') {
System.out.println("白棋获胜");
win = true;
break;}
}
}
return win;
}
public void PvE(){ /**PVE模式*/
FiveChess gb = new FiveChess();
System.out.println("请选择棋子颜色");
String computercolor,peoplennumber = null;
computercolor =choiceColor();
if(Objects.equals(computercolor, black)){
for(int e=0;;e++) { /**胜利后跳出无尽循环,结束游戏*/
System.out.println("请1号棋手落子");
gb.down(black);
peoplennumber = String.valueOf(1);
if (gb.isWin(computercolor, peoplennumber)==true) {
gb.printBoard();
break;
}
gb.Computer(white);
if (gb.isWin(computercolor, peoplennumber)==true) {
gb.printBoard();
break;
}
}
}
else if (Objects.equals(computercolor, white)) {
for(int e=0;;e++){
System.out.println("电脑落子");
gb.Computer(black);
peoplennumber = String.valueOf(2);
if (gb.isWin(computercolor, peoplennumber)==true) {
gb.printBoard();
break;
}
System.out.println("请1号棋手落子");
if (gb.isWin(computercolor, peoplennumber)==true) {
gb.printBoard();
break;
}
gb.down(white);
}
}
}
public void Computer(String color){
FiveChess gb = new FiveChess();
String peoplennumber=null;
String comoutercolor;
comoutercolor= color;
int x = (int) (Math.random() * 17) ; // 生成一个1~16之间的随机数
int y = (int) (Math.random() * 17) ; // 生成一个1~16之间的随机数
System.out.println(x);
if (isOk(x, y)) { //合法性检查 //没必要
if (color == black) {
board[x][y] = '●';
} else if (color == white) {
board[x][y] = '○';
}
}else {
gb.Computer(comoutercolor);
}
}
}
/*注释代码为其他灵感,暂未使用*/
/**public void text(){ //数据测试//输赢坐下到右上
for (int x = board.length-1; x > 5; x--) {
for (int y = 1; y < board.length-4; y++) {
System.out.println("x"+x);
System.out.println("y"+y);
}}
}*/
/**if (color == black && peoplennumber == "1") {
System.out.println("1号选手获胜");
break;
} else if (color == white && peoplennumber == "1") {
System.out.println("2号选手获胜");
break;
} else if (color == black && peoplennumber == "2") {
System.out.println("2号选手获胜");
break;
} else if (color == white && peoplennumber == "2") {
System.out.println("1号选手获胜");
break;
}*/
/**if (board[x][y] == board[x + 1][y]
&& board[x + 1][y] == board[x + 2][y]
&& board[x + 2][y] == board[x + 3][y]
&& board[x + 3][y] == board[x + 4][y]) {
if (color == black && peoplennumber == "1") {
System.out.println("1号选手获胜");
break;
} else if (color == white && peoplennumber == "1") {
System.out.println("2号选手获胜");
break;
} else if (color == black && peoplennumber == "2") {
System.out.println("2号选手获胜");
break;
} else if (color == white && peoplennumber == "2") {
System.out.println("1号选手获胜");
break;
}
if (board[x][y] == board[x + 1][y + 1]
&& board[x + 1][y + 1] == board[x + 2][y + 2]
&& board[x + 2][y + 2] == board[x + 3][y + 3]
&& board[x + 3][y + 3] == board[x + 4][y + 4]) {
if (color == black && peoplennumber == "1") {
System.out.println("1号选手获胜");
break;
} else if (color == white && peoplennumber == "1") {
System.out.println("2号选手获胜");
break;
} else if (color == black && peoplennumber == "2") {
System.out.println("2号选手获胜");
break;
} else if (color == white && peoplennumber == "2") {
System.out.println("1号选手获胜");
break;
}
}
}
*/
/**
* 1.人人对战
* 2.人机对战
* 3胜负判定 (横向 纵向 左下至右上 左上至右下)
* 4 合法性检测 (重复落子 超出边界)
* 部分数据定义无用(懒得改)
* */