Major.java
package com.mygdx.game;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.Texture;
import com.badlogic.gdx.graphics.g2d.Animation;
import com.badlogic.gdx.graphics.g2d.Batch;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.graphics.g2d.TextureRegion;
import com.badlogic.gdx.scenes.scene2d.Actor;
import com.badlogic.gdx.scenes.scene2d.Group;
import com.badlogic.gdx.scenes.scene2d.InputEvent;
import com.badlogic.gdx.scenes.scene2d.InputListener;
import com.badlogic.gdx.scenes.scene2d.ui.ImageButton;
import com.badlogic.gdx.scenes.scene2d.utils.ClickListener;
import com.badlogic.gdx.scenes.scene2d.utils.TextureRegionDrawable;
public class Major extends Actor{
int iconWidth = 32, iconHeight = 48;
public static float x ;
public static float y ;
public float statetime;
Texture texture;
TextureRegion currentFrame;
ImageButton buttonL;
ImageButton buttonR;
Animation aniRight;
Animation aniLeft;
Animation aniIdle;
STATE state;
enum STATE {
Left,Right,Idel
};
public Major(float x,float y) {
this.x = x;
this.y = y;
this.statetime = 0;
this.show();
state = STATE.Idel;
}
@Override
public void draw(Batch batch, float parentAlpha) {
statetime += Gdx.graphics.getDeltaTime();
this.update();
this.check();
batch.draw(currentFrame, x, y, iconWidth*2, iconHeight*2);
}
public void update() {
if(state == STATE.Left){
this.x -= 3.0f;
if(this.x < 100) this.x = 100;
}else if (state == STATE.Right) {
this.x +=3.0f;
if(this.x > 1800) this.x = 1800;
}
this.x = x;
}
public void check() {
if(state == STATE.Left) {
currentFrame = aniLeft.getKeyFrame(statetime, true);
}else if (state == STATE.Right) {
currentFrame = aniRight.getKeyFrame(statetime, true);
}else if (state == STATE.Idel) {
currentFrame = aniIdle.getKeyFrame(statetime, true);
}
}
public void show() {
texture = new Texture(Gdx.files.internal("data/animation.png"));
TextureRegion[][] spilt = TextureRegion.split(texture, iconWidth, iconHeight);
TextureRegion[][] buttons = TextureRegion.split(texture, iconWidth, iconHeight);
TextureRegion[] regionR = spilt[2];
aniRight = new Animation(0.1f, regionR);
TextureRegion[] regionL = spilt[1];
aniLeft = new Animation(0.1f, regionL);
TextureRegion[] regionI = new TextureRegion[1];
regionI[0] = spilt[0][0];
aniIdle = new Animation(0.1f, regionI);
TextureRegion[] buttonsRegion = new TextureRegion[4];
buttonsRegion[0] = buttonsRegion[1] = new TextureRegion(texture, 0, 192, 64, 64);
buttonsRegion[2] = buttonsRegion[3] = new TextureRegion(texture, 64, 192, 64, 64);
buttonL = new ImageButton(new TextureRegionDrawable(buttonsRegion[0]), new TextureRegionDrawable(buttonsRegion[1]));
buttonR = new ImageButton(new TextureRegionDrawable(buttonsRegion[2]), new TextureRegionDrawable(buttonsRegion[3]));
buttonL.setOrigin(0, 0);
buttonL.setPosition(100, 100);
buttonL.setSize(124, 124);
buttonL.setScale(2.0f);
buttonL.getImage().setFillParent(true);
buttonR.setOrigin(0, 0);
buttonR.setPosition(100 + 124 + 100, 100);
buttonR.setSize(124, 124);
buttonR.setScale(2.0f);
buttonR.getImage().setFillParent(true);
buttonL.addListener(new InputListener(){
@Override
public void touchUp(InputEvent event, float x, float y,
int pointer, int button) {
state = STATE.Idel;
super.touchUp(event, x, y, pointer, button);
}
@Override
public boolean touchDown(InputEvent event, float x, float y,
int pointer, int button) {
state = STATE.Left;
return true;
}
});
buttonR.addListener(new InputListener(){
@Override
public void touchUp(InputEvent event, float x, float y,
int pointer, int button) {
state = STATE.Idel;
super.touchUp(event, x, y, pointer, button);
}
@Override
public boolean touchDown(InputEvent event, float x, float y,
int pointer, int button) {
state = STATE.Right;
return true;
}
});
}
}
MyGdxGame.java
package com.mygdx.game;
import com.badlogic.gdx.Application;
import com.badlogic.gdx.ApplicationAdapter;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.InputProcessor;
import com.badlogic.gdx.graphics.Color;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.Texture;
import com.badlogic.gdx.graphics.g2d.Animation;
import com.badlogic.gdx.graphics.g2d.BitmapFont;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.graphics.g2d.TextureRegion;
import com.badlogic.gdx.graphics.glutils.ShapeRenderer;
import com.badlogic.gdx.math.Vector2;
import com.badlogic.gdx.scenes.scene2d.Actor;
import com.badlogic.gdx.scenes.scene2d.Stage;
import com.badlogic.gdx.scenes.scene2d.ui.Image;
import com.badlogic.gdx.scenes.scene2d.ui.ImageButton;
import com.badlogic.gdx.scenes.scene2d.ui.Label;
import com.badlogic.gdx.scenes.scene2d.ui.Skin;
import com.badlogic.gdx.scenes.scene2d.ui.Table;
import com.badlogic.gdx.scenes.scene2d.utils.TextureRegionDrawable;
import com.badlogic.gdx.utils.Align;
import com.badlogic.gdx.utils.viewport.ScreenViewport;
public class MyGdxGame extends ApplicationAdapter {
Stage stage;
Major major;
@Override
public void create () {
stage = new Stage(new ScreenViewport());
Gdx.input.setInputProcessor(stage);
major = new Major(100, 400);
stage.addActor(major);
stage.addActor(major.buttonL);
stage.addActor(major.buttonR);
}
@Override
public void dispose () {
stage.dispose();
}
@Override
public void render () {
Gdx.gl.glClearColor(1, 1, 0, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
stage.act(Math.min(Gdx.graphics.getDeltaTime(), 1 / 30f));
stage.draw();
}
@Override
public void resize (int width, int height) {
stage.getViewport().update(width, height, true);
}
}
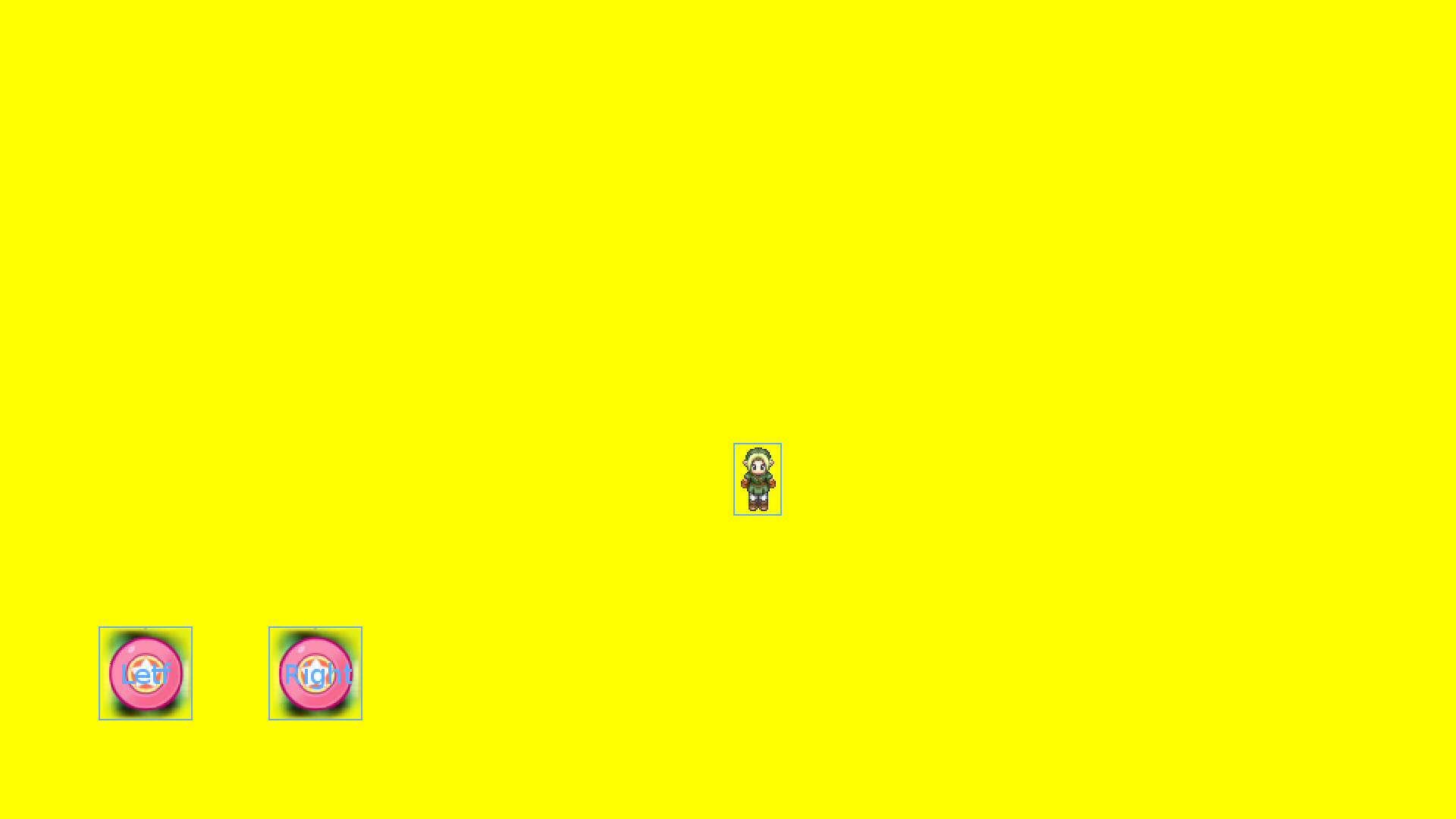