常用组件
package com.mygdx.game;
import com.badlogic.gdx.Application;
import com.badlogic.gdx.ApplicationAdapter;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.InputProcessor;
import com.badlogic.gdx.graphics.Color;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.Texture;
import com.badlogic.gdx.graphics.g2d.Animation;
import com.badlogic.gdx.graphics.g2d.BitmapFont;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.graphics.g2d.TextureRegion;
import com.badlogic.gdx.graphics.glutils.ShapeRenderer;
import com.badlogic.gdx.math.Vector2;
import com.badlogic.gdx.scenes.scene2d.Actor;
import com.badlogic.gdx.scenes.scene2d.Stage;
import com.badlogic.gdx.scenes.scene2d.ui.Image;
import com.badlogic.gdx.scenes.scene2d.ui.ImageButton;
import com.badlogic.gdx.scenes.scene2d.ui.Label;
import com.badlogic.gdx.scenes.scene2d.ui.Skin;
import com.badlogic.gdx.scenes.scene2d.ui.Table;
import com.badlogic.gdx.scenes.scene2d.utils.TextureRegionDrawable;
import com.badlogic.gdx.utils.Align;
import com.badlogic.gdx.utils.viewport.ScreenViewport;
public class MyGdxGame extends ApplicationAdapter {
Skin skin;
Stage stage;
SpriteBatch batch;
Actor root;
ShapeRenderer renderer;
Label.LabelStyle style;
BitmapFont font;
Image VictoriaImage;
TextureRegion region;
Texture tex;
TextureRegionDrawable up;
TextureRegionDrawable down;
TextureRegion buttonUp;
TextureRegion buttonDown;
ImageButton button;
@Override
public void create () {
batch = new SpriteBatch();
renderer = new ShapeRenderer();
skin = new Skin(Gdx.files.internal("data/uiskin.json"));
skin.getAtlas().getTextures().iterator().next().setFilter(Texture.TextureFilter.Nearest, Texture.TextureFilter.Nearest);
skin.getFont("default-font").getData().markupEnabled = true;
float scale = 1;
skin.getFont("default-font").getData().setScale(scale);
stage = new Stage(new ScreenViewport());
Gdx.input.setInputProcessor(stage);
font = new BitmapFont(Gdx.files.internal("data/default.fnt"),
Gdx.files.internal("data/default.png"), false);
style = new Label.LabelStyle(font, font.getColor());
Gdx.input.setInputProcessor(stage);
Label label1 = new Label("Label \n Test", style);
label1.setPosition(300, 100);
label1.setFontScale(2);
label1.setColor(Color.GREEN);
stage.addActor(label1);
tex = new Texture(Gdx.files.internal("data/badlogic.jpg"));
region = new TextureRegion(tex, 0, 0, 256, 256);
VictoriaImage = new Image(region);
VictoriaImage.setPosition(500, 100);
VictoriaImage.setOrigin(0, 0);
VictoriaImage.setSize(256, 256);
stage.addActor(VictoriaImage);
tex = new Texture(Gdx.files.internal("data/badlogic.jpg"));
TextureRegion[][] tmp = TextureRegion.split(tex, 128, 256);
buttonUp = tmp[0][0];
buttonDown = tmp[0][1];
up = new TextureRegionDrawable(buttonUp);
down = new TextureRegionDrawable(buttonDown);
button = new ImageButton(up, down);
button.setPosition(800, 100);
stage.addActor(button);
Table table = new Table();
stage.addActor(table);
table.setPosition(0, 100);
table.debug();
table.add(new Label("This is regular text.", skin));
table.row();
table.add(new Label("This is regular text\nwith a newline.", skin));
table.row();
Label label3 = new Label("This is [RED]regular text\n\nwith newlines,\naligned bottom, right.", skin);
label3.setColor(Color.GREEN);
label3.setAlignment(Align.bottom | Align.right);
table.add(label3).minWidth(200 * scale).minHeight(110 * scale).fill();
table.row();
Label label4 = new Label("This is regular text with NO newlines, wrap enabled and aligned bottom, right.", skin);
label4.setWrap(true);
label4.setAlignment(Align.bottom | Align.right);
table.add(label4).minWidth(200 * scale).minHeight(110 * scale).fill();
table.row();
Label label5 = new Label("This is regular text with\n\nnewlines, wrap\nenabled and aligned bottom, right.", skin);
label5.setWrap(true);
label5.setAlignment(Align.bottom | Align.right);
table.add(label5).minWidth(200 * scale).minHeight(110 * scale).fill();
table.pack();
}
@Override
public void dispose () {
stage.dispose();
skin.dispose();
}
@Override
public void render () {
Gdx.gl.glClearColor(0.2f, 0.2f, 0.2f, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
stage.act(Math.min(Gdx.graphics.getDeltaTime(), 1 / 30f));
stage.draw();
float x = 40, y = 40;
BitmapFont font = skin.getFont("default-font");
batch.begin();
font.draw(batch, "The quick brown fox jumped over the lazy cow.", x, y);
batch.end();
drawLine(x, y - font.getDescent(), x + 1000, y - font.getDescent());
drawLine(x, y - font.getCapHeight() + font.getDescent(), x + 1000, y - font.getCapHeight() + font.getDescent());
}
public void drawLine (float x1, float y1, float x2, float y2) {
renderer.setProjectionMatrix(batch.getProjectionMatrix());
renderer.begin(ShapeRenderer.ShapeType.Line);
renderer.line(x1, y1, x2, y2);
renderer.end();
}
@Override
public void resize (int width, int height) {
stage.getViewport().update(width, height, true);
}
}
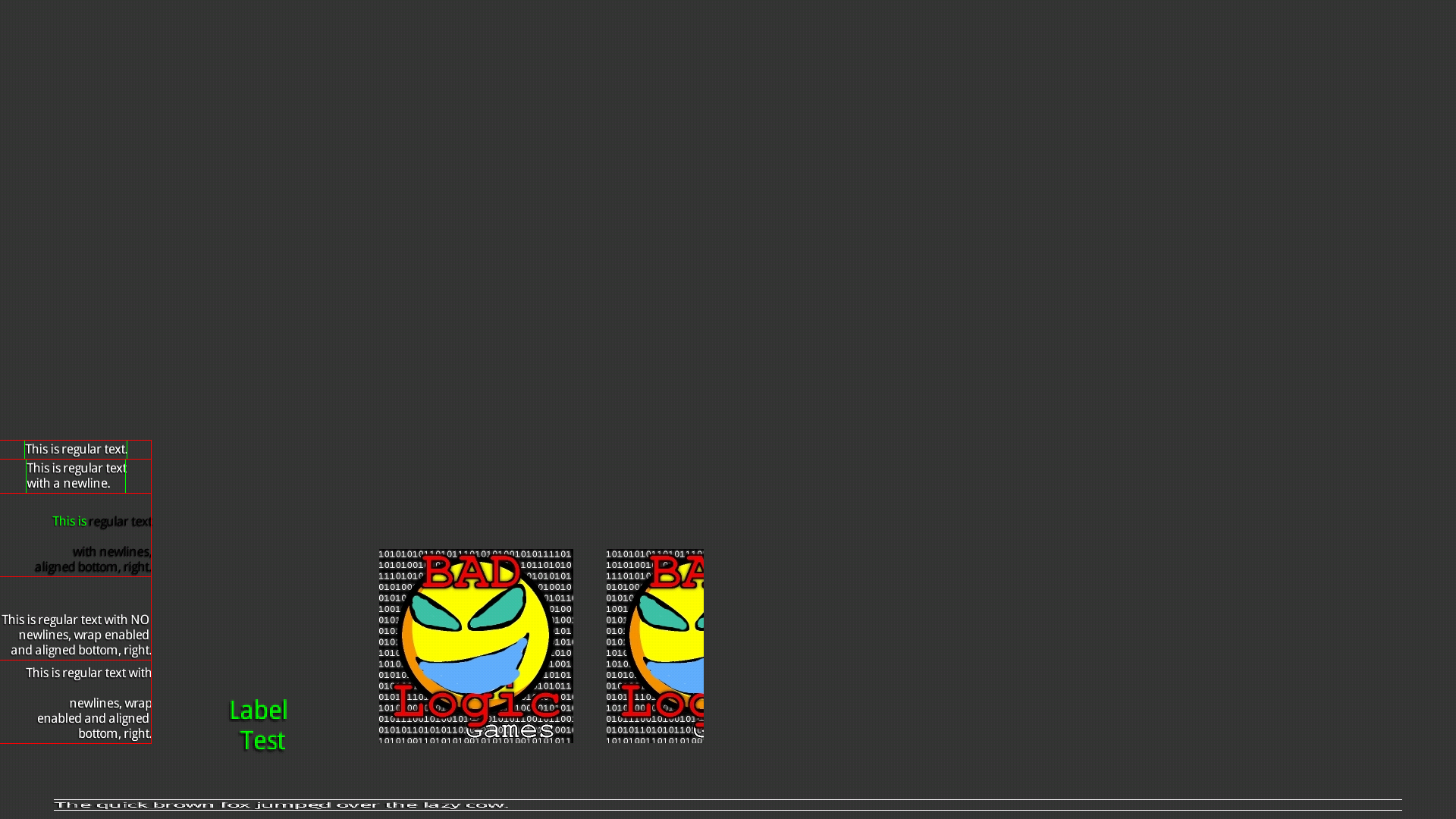