Description
In order to understand early civilizations, archaeologists often study texts written in ancient languages. One such language, used in Egypt more than 3000 years ago, is based on characters called hieroglyphs. Figure C.1 shows six hieroglyphs and their names. In this problem, you will write a program to recognize these six characters.
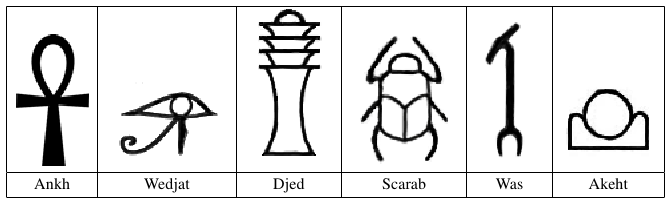
Input
The input consists of several test cases, each of which describes an image containing one or more hieroglyphs chosen from among those shown in Figure C.1. The image is given in the form of a series of horizontal scan lines consisting of black pixels (represented by 1) and white pixels (represented by 0). In the input data, each scan line is encoded in hexadecimal notation. For example, the sequence of eight pixels10011100 (one black pixel, followed by two white pixels, and so on) would be represented in hexadecimal notation as 9c. Only digits and lowercase letters a through f are used in the hexadecimal encoding. The first line of each test case contains two integers, H and W. H (0 < H200) is the number of scan lines in the image. W (0 < W
50) is the number of hexadecimal characters in each line. The next H lines contain the hexadecimal characters of the image, working from top to bottom. Input images conform to the following rules:
- The image contains only hieroglyphs shown in Figure C.1.
- Each image contains at least one valid hieroglyph.
- Each black pixel in the image is part of a valid hieroglyph.
- Each hieroglyph consists of a connected set of black pixels and each black pixel has at least one other black pixel on its top, bottom, left, or right side.
- The hieroglyphs do not touch and no hieroglyph is inside another hieroglyph.
- Two black pixels that touch diagonally will always have a common touching black pixel.
- The hieroglyphs may be distorted but each has a shape that is topologically equivalent to one of the symbols in Figure C.1. (Two figures are topologically equivalent if each can be transformed into the other by stretching without tearing.)
The last test case is followed by a line containing two zeros.
Output
For each test case, display its case number followed by a string containing one character for each hieroglyph recognized in the image, using the following code:
Ankh: A
Wedjat: J
Djed: D
Scarab: S
Was: W
Akhet: K
In each output string, print the codes in alphabetic order. Follow the format of the sample output.
The sample input contains descriptions of test cases shown in Figures C.2 and C.3. Due to space constraints not all of the sample input can be shown on this page.
Sample Input
100 25 0000000000000000000000000 0000000000000000000000000 ...(50 lines omitted)... 00001fe0000000000007c0000 00003fe0000000000007c0000 ...(44 lines omitted)... 0000000000000000000000000 0000000000000000000000000 150 38 00000000000000000000000000000000000000 00000000000000000000000000000000000000 ...(75 lines omitted)... 0000000003fffffffffffffffff00000000000 0000000003fffffffffffffffff00000000000 ...(69 lines omitted)... 00000000000000000000000000000000000000 00000000000000000000000000000000000000 0 0
Sample Output
Case 1: AKW Case 2: AAAAA第一次读完题目之后我并没有理解题目的意思,多读了几遍之后我发现自己有个地方是理解错的:输入的是16进制数,需要转化成2进制数才能继续求解。这道题有一点很麻烦就是输入的图形可以是拉伸之后的图形。在这种情况之下,我们必须通过一些特征值来判断图形。我们发现,每个文字的“洞”的个数是不一样的,所以我们可以来数洞的个数。现在题目的整体思路我们已经捋清楚了,接下来我来具体说一下求解过程:
1.将读入的十六进制字符转化为二进制数。
2.通过dfs把各个“四连通块”编上号,同时将黑色连通块保存起来。
3.统计黑色连通块相邻的白色连通块的数量(除了编号为1的,因为1号是图形之外的空白区域)
4.根据“洞”的个数输出相应的图形编号,并按字典序输出。代码如下:
#include<algorithm>
#include<cstring>
#include<cstdio>
#include<vector>
#include<set>
using namespace std;
const int maxh=200+5;
const int maxw=50*4+5;
char bin[256][5],line[maxw];
int color[maxh][maxw],pic[maxh][maxw],w,h;
void change(char s,int r,int c)
{
for(int i=0;i<4;i++)
pic[r][c+i]=bin[s][i]-'0';
}
const int value1[4]={-1,1,0,0};
const int value2[4]={0,0,-1,1};
void dfs(int r,int c,int dic)
{
color[r][c]=dic;
for(int i=0;i<4;i++)
{
int r2=r+value1[i];
int c2=c+value2[i];
if(r2>=0&&r2<h&&c2>=0&&c2<w&&color[r2][c2]==0&&pic[r][c]==pic[r2][c2])
dfs(r2,c2,dic);
}
}
vector<set<int> > recognize;
void check(int r,int c)
{
for(int i=0;i<4;i++)
{
int r2=r+value1[i];
int c2=c+value2[i];
if(r2>=0&&r2<h&&c2>=0&&c2<w&&pic[r2][c2]==0&&color[r2][c2]!=1)
recognize[color[r][c]].insert(color[r2][c2]);
}
}
const char mes[]={"WAKJSD"};
char Recognize(int n)
{
int i=recognize[n].size();
return mes[i];
}
int main()
{
strcpy(bin['0'],"0000");
strcpy(bin['1'],"0001");
strcpy(bin['2'],"0010");
strcpy(bin['3'],"0011");
strcpy(bin['4'],"0100");
strcpy(bin['5'],"0101");
strcpy(bin['6'],"0110");
strcpy(bin['7'],"0111");
strcpy(bin['8'],"1000");
strcpy(bin['9'],"1001");
strcpy(bin['a'],"1010");
strcpy(bin['b'],"1011");
strcpy(bin['c'],"1100");
strcpy(bin['d'],"1101");
strcpy(bin['e'],"1110");
strcpy(bin['f'],"1111");
int Case=0;
while(scanf("%d%d",&h,&w)==2&&h)
{
memset(pic,0,sizeof(pic));
for(int i=0;i<h;i++)
{
scanf("%s",line);
for(int j=0;j<w;j++)
change(line[j],i+1,j*4+1);
}
h=h+2;
w=4*w+2;
memset(color,0,sizeof(color));
vector<int> cc;
int cnt=0;
for(int i=0;i<h;i++)
for(int j=0;j<w;j++)
{
if(!color[i][j])
{
dfs(i,j,++cnt);
if(pic[i][j]==1) cc.push_back(cnt);
}
}
recognize.clear();
recognize.resize(cnt+1);
for(int i=0;i<h;i++)
for(int j=0;j<w;j++)
{
if(pic[i][j]==1)
check(i,j);
}
vector<char> code;
for(int i=0;i<cc.size();i++)
{
code.push_back(Recognize(cc[i]));
}
sort(code.begin(),code.end());
printf("Case %d: ",++Case);
for(int i=0;i<code.size();i++)
{
printf("%c",code[i]);
}
printf("\n");
}
return 0;
}