#include<iostream>
using namespace std;
template<typename T>
void myswap(T& a, T& b) {
T temp = a;
a = b;
b = temp;
}
template<class T>
void mysort(T a[],int len) {
for (int i = 0; i < len; i++) {
int max = i;
for (int j = i + 1; j < len; j++) {
if (a[max] < a[j])
{
max = j;
}
}
if (max != i) {//交换下标
myswap(a[max], a[i]);
}
}
}
template<typename T>
void printArray(T a[], int len) {
for (int i = 0; i < len; i++) {
cout << a[i] << "";
}
cout << endl;
}
void test01() {
char charr[] = "bcmnf";
int num = sizeof(charr) / sizeof(char);
mysort(charr, num);
printArray(charr, num);
}
void test02() {
int cc[] = { 2,1,3,8 };
int num = sizeof(cc) / sizeof(int);
mysort(cc, num);
printArray(cc, num);
}
int main() {
test01();
test02();
system("pause");
return 0;
}
【C++】模板,数组排序
最新推荐文章于 2024-11-12 13:55:23 发布
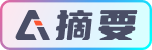