google可能为了安全考虑,在5.1.+后调用activitymanager.getRunningAppProcesses()方法只能返回你自己应用的进程,那如何在5.1.+后获取运行中进程呢?一个大神stackoverflow给出了答案(点击跳转)。如果你能熟练的导入第三方库,那么相信你可以不用向下看了,如果你选择向下看,那我会用白话文教你一步步实现。首先到这位答主的github上下载他上传的开源库,梯子自备(点击跳转)。没有梯子可以到我的个人百度云下载:链接:http://pan.baidu.com/s/1kVjjPsF 密码: ag6u
下载完成后解压目录结构
我们只需要其中的红线框住的部分,但是直接导入,肯定会处很多的问题,我们先来处理一部分,打开libsuperuser
其中你框选的三个文件是我们要注意的。如果要导入的文件中有build.gradle或AndroidManifest.xml、project.properties文件,需要将其用记事本打开后,将里面的gradle及Android版本修改为自己使用的,如果不知道的话可以新建一个工程或者打开以前建的工程中的相关文件进行对比查看。
修改完成后来到android studio的project视图下将他粘贴进去
粘贴进去肯定会叫你同步,然后就同步罗,会发现一些问题,其中常见的是提示:
Error:(2, 0) Plugin with id ‘com.github.dcendents.android-maven’ not found
Error:(2, 0) Plugin with id ‘com.jfrog.bintray.gradle’ not found
这是因为有两个插件我们没有装上,我们来到Project下,在那个build.grade里面添加全局依赖
将这两个依赖的插件写上,建议写一个同步一次分两次进行,第二次下载需要比较长的时间
classpath 'com.github.dcendents:android-maven-gradle-plugin:1.3'
classpath "com.jfrog.bintray.gradle:gradle-bintray-plugin:1.0"
填写好后我们会发现没有编译错误了,那我们要怎么去用添加的这个开源库呢??来到android视图下的build.gradle(Module.app)下添加依赖
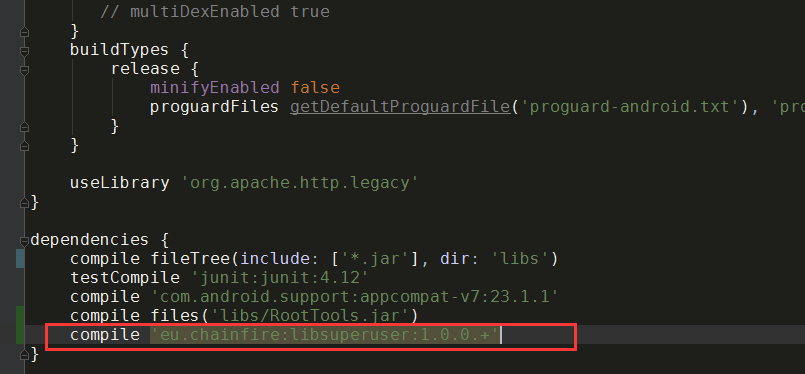
compile 'eu.chainfire:libsuperuser:1.0.0.+'
重新同步后,我们就可以调用里面的方法了,新建一个ProgressManager类
import android.content.Context;
import android.content.pm.ApplicationInfo;
import android.content.pm.PackageInfo;
import android.content.pm.PackageManager;
import android.os.Build;
import android.os.Parcel;
import android.os.Parcelable;
import java.util.ArrayList;
import java.util.List;
import eu.chainfire.libsuperuser.Shell;
/**
* @author Jared Rummler
*/
public class ProcessManager {
private static final String TAG = "ProcessManager";
private static final String APP_ID_PATTERN;
static {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN_MR1) {
APP_ID_PATTERN = "u\\d+_a\\d+";
} else {
APP_ID_PATTERN = "app_\\d+";
}
}
public static List<Process> getRunningProcesses() {
List<Process> processes = new ArrayList<>();
List<String> stdout = Shell.SH.run("toolbox ps -p -P -x -c");
for (String line : stdout) {
try {
processes.add(new Process(line));
} catch (Exception e) {
android.util.Log.d(TAG, "Failed parsing line " + line);
}
}
return processes;
}
public static List<Process> getRunningApps() {
List<Process> processes = new ArrayList<>();
List<String> stdout = Shell.SH.run("toolbox ps -p -P -x -c");
int myPid = android.os.Process.myPid();
for (String line : stdout) {
try {
Process process = new Process(line);
if (process.user.matches(APP_ID_PATTERN)) {
if (process.ppid == myPid || process.name.equals("toolbox")) {
continue;
}
processes.add(process);
}
} catch (Exception e) {
android.util.Log.d(TAG, "Failed parsing line " + line);
}
}
return processes;
}
public static class Process implements Parcelable {
/** User name */
public final String user;
/** User ID */
public final int uid;
/** Processes ID */
public final int pid;
/** Parent processes ID */
public final int ppid;
/** virtual memory size of the process in KiB (1024-byte units). */
public final long vsize;
/** resident set size, the non-swapped physical memory that a task has used (in kiloBytes). */
public final long rss;
public final int cpu;
/** The priority */
public final int priority;
/** The priority, <a href="https://en.wikipedia.org/wiki/Nice_(Unix)">niceness</a> level */
public final int niceness;
/** Real time priority */
public final int realTimePriority;
/** 0 (sched_other), 1 (sched_fifo), and 2 (sched_rr). */
public final int schedulingPolicy;
/** The scheduling policy. Either "bg", "fg", "un", "er", or "" */
public final String policy;
/** address of the kernel function where the process is sleeping */
public final String wchan;
public final String pc;
/**
* Possible states:
* <p/>
* "D" uninterruptible sleep (usually IO)
* <p/>
* "R" running or runnable (on run queue)
* <p/>
* "S" interruptible sleep (waiting for an event to complete)
* <p/>
* "T" stopped, either by a job control signal or because it is being traced
* <p/>
* "W" paging (not valid since the 2.6.xx kernel)
* <p/>
* "X" dead (should never be seen)
* </p>
* "Z" defunct ("zombie") process, terminated but not reaped by its parent
*/
public final String state;
/** The process name */
public final String name;
/** user time in milliseconds */
public final long userTime;
/** system time in milliseconds */
public final long systemTime;
private Process(String line) throws Exception {
String[] fields = line.split("\\s+");
user = fields[0];
uid = android.os.Process.getUidForName(user);
pid = Integer.parseInt(fields[1]);
ppid = Integer.parseInt(fields[2]);
vsize = Integer.parseInt(fields[3]) * 1024;
rss = Integer.parseInt(fields[4]) * 1024;
cpu = Integer.parseInt(fields[5]);
priority = Integer.parseInt(fields[6]);
niceness = Integer.parseInt(fields[7]);
realTimePriority = Integer.parseInt(fields[8]);
schedulingPolicy = Integer.parseInt(fields[9]);
if (fields.length == 16) {
policy = "";
wchan = fields[10];
pc = fields[11];
state = fields[12];
name = fields[13];
userTime = Integer.parseInt(fields[14].split(":")[1].replace(",", "")) * 1000;
systemTime = Integer.parseInt(fields[15].split(":")[1].replace(")", "")) * 1000;
} else {
policy = fields[10];
wchan = fields[11];
pc = fields[12];
state = fields[13];
name = fields[14];
userTime = Integer.parseInt(fields[15].split(":")[1].replace(",", "")) * 1000;
systemTime = Integer.parseInt(fields[16].split(":")[1].replace(")", "")) * 1000;
}
}
private Process(Parcel in) {
user = in.readString();
uid = in.readInt();
pid = in.readInt();
ppid = in.readInt();
vsize = in.readLong();
rss = in.readLong();
cpu = in.readInt();
priority = in.readInt();
niceness = in.readInt();
realTimePriority = in.readInt();
schedulingPolicy = in.readInt();
policy = in.readString();
wchan = in.readString();
pc = in.readString();
state = in.readString();
name = in.readString();
userTime = in.readLong();
systemTime = in.readLong();
}
public String getPackageName() {
if (!user.matches(APP_ID_PATTERN)) {
return null;
} else if (name.contains(":")) {
return name.split(":")[0];
}
return name;
}
public PackageInfo getPackageInfo(Context context, int flags)
throws PackageManager.NameNotFoundException
{
String packageName = getPackageName();
if (packageName == null) {
throw new PackageManager.NameNotFoundException(name + " is not an application process");
}
return context.getPackageManager().getPackageInfo(packageName, flags);
}
public ApplicationInfo getApplicationInfo(Context context, int flags)
throws PackageManager.NameNotFoundException
{
String packageName = getPackageName();
if (packageName == null) {
throw new PackageManager.NameNotFoundException(name + " is not an application process");
}
return context.getPackageManager().getApplicationInfo(packageName, flags);
}
@Override
public int describeContents() {
return 0;
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeString(user);
dest.writeInt(uid);
dest.writeInt(pid);
dest.writeInt(ppid);
dest.writeLong(vsize);
dest.writeLong(rss);
dest.writeInt(cpu);
dest.writeInt(priority);
dest.writeInt(niceness);
dest.writeInt(realTimePriority);
dest.writeInt(schedulingPolicy);
dest.writeString(policy);
dest.writeString(wchan);
dest.writeString(pc);
dest.writeString(state);
dest.writeString(name);
dest.writeLong(userTime);
dest.writeLong(systemTime);
}
public static final Creator<Process> CREATOR = new Creator<Process>() {
public Process createFromParcel(Parcel source) {
return new Process(source);
}
public Process[] newArray(int size) {
return new Process[size];
}
};
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 126
- 127
- 128
- 129
- 130
- 131
- 132
- 133
- 134
- 135
- 136
- 137
- 138
- 139
- 140
- 141
- 142
- 143
- 144
- 145
- 146
- 147
- 148
- 149
- 150
- 151
- 152
- 153
- 154
- 155
- 156
- 157
- 158
- 159
- 160
- 161
- 162
- 163
- 164
- 165
- 166
- 167
- 168
- 169
- 170
- 171
- 172
- 173
- 174
- 175
- 176
- 177
- 178
- 179
- 180
- 181
- 182
- 183
- 184
- 185
- 186
- 187
- 188
- 189
- 190
- 191
- 192
- 193
- 194
- 195
- 196
- 197
- 198
- 199
- 200
- 201
- 202
- 203
- 204
- 205
- 206
- 207
- 208
- 209
- 210
- 211
- 212
- 213
- 214
- 215
- 216
- 217
- 218
- 219
- 220
- 221
- 222
- 223
- 224
- 225
- 226
- 227
- 228
- 229
- 230
- 231
- 232
- 233
- 234
- 235
- 236
- 237
- 238
- 239
- 240
- 241
- 242
- 243
- 244
- 245
- 246
- 247
- 248
- 249
- 250
- 251
- 252
- 253
- 254
- 255
- 256
- 257
- 258
- 259
- 260
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 126
- 127
- 128
- 129
- 130
- 131
- 132
- 133
- 134
- 135
- 136
- 137
- 138
- 139
- 140
- 141
- 142
- 143
- 144
- 145
- 146
- 147
- 148
- 149
- 150
- 151
- 152
- 153
- 154
- 155
- 156
- 157
- 158
- 159
- 160
- 161
- 162
- 163
- 164
- 165
- 166
- 167
- 168
- 169
- 170
- 171
- 172
- 173
- 174
- 175
- 176
- 177
- 178
- 179
- 180
- 181
- 182
- 183
- 184
- 185
- 186
- 187
- 188
- 189
- 190
- 191
- 192
- 193
- 194
- 195
- 196
- 197
- 198
- 199
- 200
- 201
- 202
- 203
- 204
- 205
- 206
- 207
- 208
- 209
- 210
- 211
- 212
- 213
- 214
- 215
- 216
- 217
- 218
- 219
- 220
- 221
- 222
- 223
- 224
- 225
- 226
- 227
- 228
- 229
- 230
- 231
- 232
- 233
- 234
- 235
- 236
- 237
- 238
- 239
- 240
- 241
- 242
- 243
- 244
- 245
- 246
- 247
- 248
- 249
- 250
- 251
- 252
- 253
- 254
- 255
- 256
- 257
- 258
- 259
- 260
通过这个类我们就可以获得运行中的进程了
List<ProcessManager.Process> runningProcesses = ProcessManager.getRunningProcesses();
但是有很多进程都没有名字,如果你想让用户直观的管理进程的话可能需要下面这个循环过滤没有名字的线程
List<ProcessManager.Process> Processes=new ArrayList<>()
PackageManager pm = getPackageManager()
runningProcesses = ProcessManager.getRunningProcesses()
for (ProcessManager.Process runningProcesse : runningProcesses) {
String packname = runningProcesse.getPackageName()
try {
ApplicationInfo applicationInfo = pm.getApplicationInfo(packname, 0)
} catch (PackageManager.NameNotFoundException e) {
e.printStackTrace()
continue
}
Processes.add(runningProcesse)
}