Sequelize是用于Postgres,MySQL,MariaDB,SQLite和Microsoft SQL Server的基于promise的Node.js ORM工具。它具有可靠的事务支持,关系,急切和延迟加载,读取复制等功能。
Sequelize遵循语义版本控制,并支持Node v10及更高版本。
sequelize的npm地址:https://www.npmjs.com/package/sequelize
安装:
npm i sequelize
其要和一个数据库结合使用,这里我们选择mysql, 而在我们的koa2工程中, 我们使用mysql2库来操作数据库
npm i mysql2
一. 连接MySQL数据库
- 首先导入模块
const { Sequelize } = require('sequelize');
- 用导入的Sequelize 实例化一个sequelize对象:
const sequelize = new Sequelize('db1', 'root', 'xxxxx', {
host: 'localhost', //数据库地址
dialect: 'mysql', //指定连接的数据库类型
pool: {
max: 5, //连接池最大连接数量
min: 0, //最小连接数量
idle: 10000, //如果一个线程 10秒内么有被使用过的话,就释放
},
})
这样就完成了数据库链接了
我们可以测试一下:
sequelize对象提供了一个authenticate方法,可以测试数据库链接https://sequelize.org/master/class/lib/sequelize.js~Sequelize.html#instance-method-authenticate
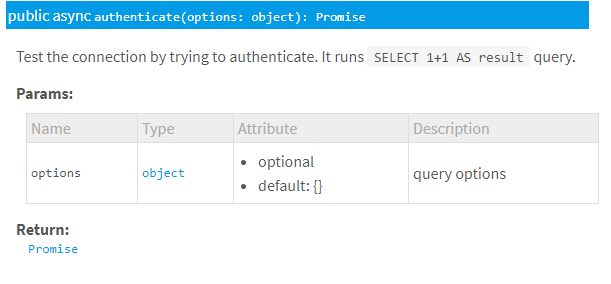
我们可以看到它return 一个promise, 所以我们应该在一个async方法里处理这个promise
例如: 我们在index.js里写一个访问127.0.0.1就告诉我们数据库连接是否成功的API,可以:
const router = require('koa-router')()
const { Sequelize } = require('sequelize');
const sequelize = new Sequelize('db1', 'root', 'xxxxxx', {
host: 'localhost', //数据库地址
dialect: 'mysql', //指定连接的数据库类型
pool: {
max: 5, //连接池最大连接数量
min: 0, //最小连接数量
idle: 10000, //如果一个线程 10秒内么有被使用过的话,就释放
},
})
router.get('/', async (ctx, next) => {
let str1
str1 = await sequelize
.authenticate()
.then(() => {
return '连接数据库成功'
})
.catch(err => {
return '连接数据库失败' + err
});
ctx.body = str1
})
module.exports = router
二. 创建模型:(获取表)
https://sequelize.org/master/manual/model-basics.html
1. 创建模型
连接数据库成功后, 怎么使用ORM的方式把数据库用起来呢? 答: 创建模型
模型是Sequelize的本质。模型是代表数据库中表的抽象。
Sequelize中的模型有一个名称。此名称不必与它在数据库中表示的表的名称相同。通常,模型具有单数名称(例如User),而表具有复数名称(例如Users),尽管这是完全可配置的。
通过创建模型,我们将MySQL中的表和模型对应起来
class Student extends Model { }//创建一个类,继承Model
Student.init({
id: { type:DataTypes.INTEGER, primaryKey: true },
name: DataTypes.STRING,
age: DataTypes.INTEGER,
score: DataTypes.FLOAT,
brithday: DataTypes.DATE,
}, { sequelize, modelName: 'Student' })
除了这种声明方法,还有一种方法把一张表声明为一个model,这种方法本质上还是在调用init
函数, 用哪种随你喜欢
const Student = sequelize.define('Student111', {
id: { type: DataTypes.INTEGER, primaryKey: true },
name: DataTypes.STRING,
age: DataTypes.INTEGER,
score: DataTypes.FLOAT,
brithday: DataTypes.DATE,
}, {sequelize})
2. 模型同步
定义模型时,您要告诉Sequelize有关数据库中表的一些信息。但是,如果该表实际上甚至不存在于数据库中怎么办?如果存在,但具有不同