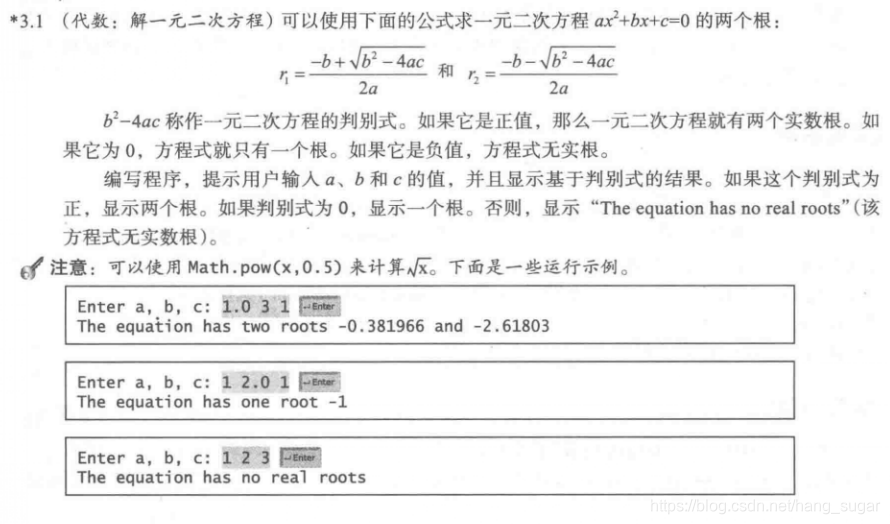
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter a, b, c: ");
double a = input.nextDouble();
double b = input.nextDouble();
double c = input.nextDouble();
double t = Math.pow(b , 2) - 4 * a * c;
double r1 , r2;
if (t > 0) {
r1 = ((-b) + Math.pow(t , 0.5)) / (2 * a);
r2 = ((-b) - Math.pow(t , 0.5)) / (2 * a);
System.out.println("The equation has two roots " + r1 + " and " + r2);
}
else if (t == 0) {
r1 = ((-b) + Math.pow(t , 0.5)) / (2 * a);
System.out.println("The equation has one root " + r1);
}
else{
System.out.println("The equation has no real roots");
}
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
int number1 = (int)(System.currentTimeMillis() % 10);
int number2 = (int)(System.currentTimeMillis() / 7 % 10);
int number3 = (int)(System.currentTimeMillis() / 4 % 10);
Scanner input = new Scanner(System.in);
System.out.print("What is " + number1 +" + "+ number2 +" + "+ number3 +" ? ");
int answer = input.nextInt();
System.out.println(number1 +" + "+ number2 +" + "+ number3 + " = " + answer +" is " +
(number1 + number2 + number3 == answer));
}
}
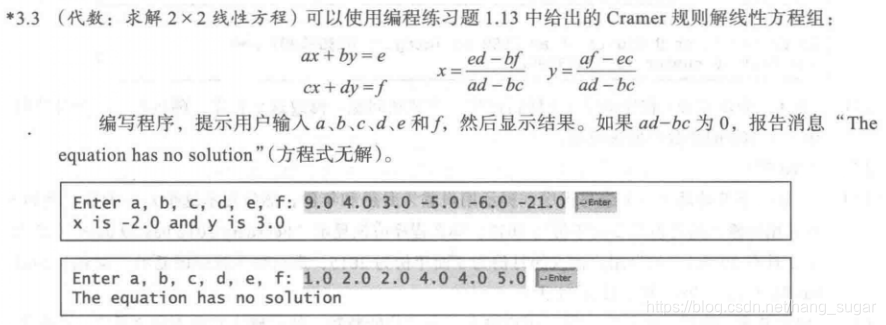
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter a, b, c, d, e, f:");
double a = input.nextDouble();
double b = input.nextDouble();
double c = input.nextDouble();
double d = input.nextDouble();
double e = input.nextDouble();
double f = input.nextDouble();
double t = a * d - b * c , x , y ;
if(t != 0){
x = ((e * d - b * f) / t);
y = ((a * f - e * c) / t);
System.out.println("x is " + x + " and " + "y is " +y);
}
else{
System.out.println("The equation has no solution");
}
}
}

public class tt1 {
public static void main(String args[]){
int month = (int)(System.currentTimeMillis() % 13);
switch (month){
case 1 : System.out.println("The number " + month + " is " + "January"); break;
case 2 : System.out.println("The number " + month + " is " + "February"); break;
case 3 : System.out.println("The number " + month + " is " + "March"); break;
case 4 : System.out.println("The number " + month + " is " + "April"); break;
case 5 : System.out.println("The number " + month + " is " + "May"); break;
case 6 : System.out.println("The number " + month + " is " + "June"); break;
case 7 : System.out.println("The number " + month + " is " + "July"); break;
case 8 : System.out.println("The number " + month + " is " + "August"); break;
case 9 : System.out.println("The number " + month + " is " + "September"); break;
case 10 : System.out.println("The number " + month + " is " + "October"); break;
case 11 : System.out.println("The number " + month + " is " + "November"); break;
case 12 : System.out.println("The number " + month + " is " + "December"); break;
}
}
}
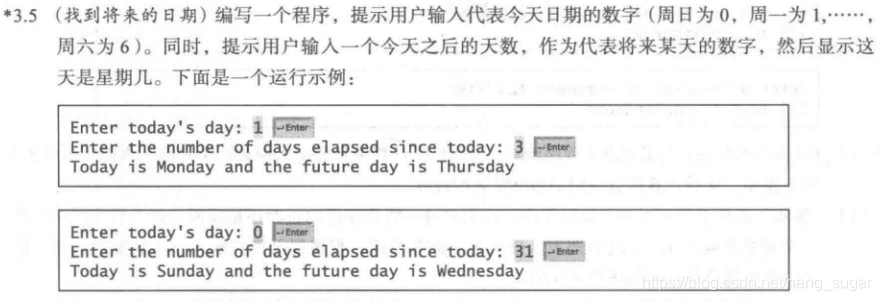
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter today's day: ");
int today = input.nextInt();
System.out.print("Enter the number of days elapsed since today: ");
int days = input.nextInt();
switch (today){
case 0 : System.out.print("Today is Sunday ");break;
case 1 : System.out.print("Today is Monday ");break;
case 2 : System.out.print("Today is Tuesday ");break;
case 3 : System.out.print("Today is Wednesday ");break;
case 4 : System.out.print("Today is Thursday ");break;
case 5 : System.out.print("Today is Friday ");break;
case 6 : System.out.print("Today is Saturday ");break;
}
days = days % 7;
int future = days + today;
if(future > 7){
future = future - 7;
}
switch (future){
case 0 : System.out.println("and the future day is Sunday ");break;
case 1 : System.out.println("and the future day is Monday ");break;
case 2 : System.out.println("and the future day is Tuesday ");break;
case 3 : System.out.println("and the future day is Wednesday ");break;
case 4 : System.out.println("and the future day is Thursday ");break;
case 5 : System.out.println("and the future day is Friday ");break;
case 6 : System.out.println("and the future day is Saturday ");break;
}
}
}
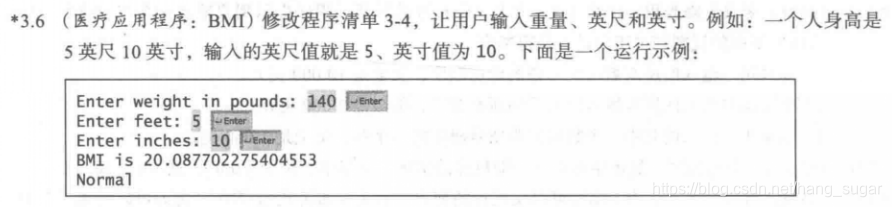
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter weight in pounds:");
double weight = input.nextDouble();
System.out.print("Enter feet:");
double feet = input.nextDouble();
System.out.print("Enter inches:");
double inches = input.nextDouble();
inches = feet * 12 + inches;
final double KIL0GRAMS_PER_P0UND = 0.45359237;
final double METERS_PER_INCH = 0.0254;
double weightInKilograms = weight * KIL0GRAMS_PER_P0UND;
double heightInMeters = inches * METERS_PER_INCH;
double bmi = weightInKilograms / (heightInMeters * heightInMeters);
System.out.println("BMI is " + bmi);
if (bmi < 18.5)
System.out.println("Underweight");
else if (bmi < 25)
System.out.println("Normal");
else if (bmi < 30)
System.out.println("Overweight");
else
System.out.println("Obese");
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter an amount in double, for example 11.56:");
double amount = input.nextDouble();
int remainingAmount = (int)(amount * 100);
int numberOfOneDollars = remainingAmount / 100;
remainingAmount = remainingAmount % 100;
int numberOfQuarters = remainingAmount / 25;
remainingAmount = remainingAmount % 25;
int numberOfDimes = remainingAmount / 10;
remainingAmount = remainingAmount % 10;
int numberOfNickels = remainingAmount /5;
remainingAmount =remainingAmount % 5;
int numberOfPennies = remainingAmount;
System.out.println("Your amount " + amount + " consists of ");
if(numberOfOneDollars == 1)
System.out.println(" " + numberOfOneDollars + " dollar");
else if (numberOfOneDollars > 1)
System.out.println(" " + numberOfOneDollars + " dollars");
if(numberOfQuarters == 1)
System.out.println(" " + numberOfQuarters + " quarter");
else if (numberOfQuarters > 1)
System.out.println(" " + numberOfQuarters + " quarters");
if(numberOfDimes == 1)
System.out.println(" " + numberOfDimes + " dime");
else if (numberOfDimes > 1)
System.out.println(" " + numberOfDimes + " dimes");
if(numberOfNickels == 1)
System.out.println(" " + numberOfNickels + " nickel");
else if (numberOfNickels > 1)
System.out.println(" " + numberOfNickels + " nickels");
if(numberOfPennies == 1)
System.out.println(" " + numberOfPennies + " penny");
else if (numberOfPennies > 1)
System.out.println(" " + numberOfPennies + " pennies");
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter a,b,c:");
int a = input.nextInt();
int b = input.nextInt();
int c = input.nextInt();
int t;
if (a > b){
t = a;
a = b;
b = t;
}
if (a > c){
t = a;
a = c;
c = t;
}
if (b > c){
t = b;
b = c;
c = t;
}
System.out.println("The result is :" + a + " " + b + " " + c);
}
}
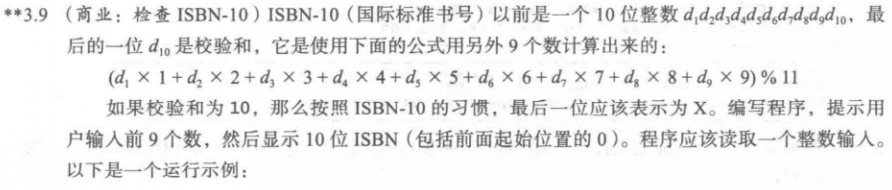

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter number: ");
int d1 , d2 , d3 , d4 , d5 , d6 , d7 , d8 , d9 , retain;
int number = input.nextInt();
retain = number;
d1 = 0;
d2 = number / 10000000;
number = number % 10000000;
d3 = number / 1000000;
number = number % 1000000;
d4 = number / 100000;
number = number % 100000;
d5 = number / 10000;
number = number % 10000;
d6 = number / 1000;
number = number % 1000;
d7 = number / 100;
number = number % 100;
d8 = number / 10;
number = number % 10;
d9 = number;
int t =(d1 * 1 + d2 * 2 + d3 * 3 + d4 * 4 + d5 * 5 + d6 * 6 + d7 * 7 + d8 * 8 + d9 * 9) % 11;
if(t == 10)
System.out.println("The ISBN-10 number is " + "0" + retain + "X");
else
System.out.println("The ISBN-10 number is " + "0" + retain + t);
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
int number1 = (int)(Math.random() * 10);
int number2 = (int)(Math.random() * 10);
System.out.print("What is " + number1 + "+" + number2 + " ? ");
Scanner input = new Scanner(System.in);
int answer = input.nextInt();
if (number1 + number2 == answer)
System.out.println("Your are correct!");
else{
System.out.println("Your answer is wrong.");
System.out.println(number1 + "+" + number2 + " should be " + (number1 + number2));
}
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter month number 1~12: ");
int month = input.nextInt();
System.out.print("Enter year number: ");
int year = input.nextInt();
if (month == 2){
if (year % 4 == 0 && year % 100 != 0 || year % 400 ==0){
System.out.println("February " + year + " has 29 days");
}
else{
System.out.println("February " + year + " has 28 days");
}
}
else{
switch (month){
case 1 : System.out.println("January "+ year + " has 31 days");break;
case 3 : System.out.println("March "+ year + " has 31 days");break;
case 4 : System.out.println("April "+ year + " has 30 days");break;
case 5 : System.out.println("May "+ year + " has 31 days");break;
case 6 : System.out.println("June "+ year + " has 30 days");break;
case 7 : System.out.println("July "+ year + " has 31 days");break;
case 8 : System.out.println("August "+ year + " has 31 days");break;
case 9 : System.out.println("September "+ year + " has 30 days");break;
case 10 : System.out.println("October "+ year + " has 31 days");break;
case 11 : System.out.println("November "+ year + " has 30 days");break;
case 12 : System.out.println("December "+ year + " has 31 days");break;
}
}
}
}
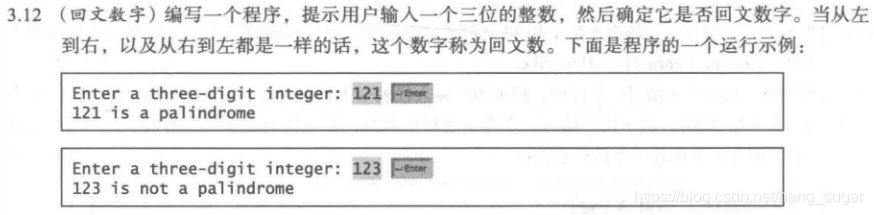
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter a three-digit integer: ");
int number = input.nextInt();
int front , later;
later = number % 10;
front = number / 100;
if (later == front)
System.out.println(number + " is a palindrome");
else
System.out.println(number + " is not a palindrome");
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.println("(0-single filer, 1-married jointly or " );
System.out.println("qualifying widow(er), 2-married separately, 3-head of ");
System.out.print("household) Enter the filing status: ");
int status = input.nextInt();
System.out.print("Enter the taxable income : ");
double income = input.nextDouble();
double tax = 0;
if (status == 0){
if (income <= 8350)
tax = income * 0.10;
else if (income <= 33950)
tax = 8350 * 0.10 + (income - 8350) * 0.15;
else if (income <= 82250)
tax = 8350 * 0.10 + (33950 - 8350) * 0.15 + (income - 33950) * 0.25;
else if (income <= 171550)
tax = 8350 * 0.10 + (33950 - 8350) * 0.15 + (82250 - 33950) * 0.25 + (income - 82250) * 0.28;
else if (income <= 372950)
tax = 8350 * 0.10 + (33950 - 8350) * 0.15 + (82250 - 33950) * 0.25 + (171550 - 82250) * 0.28 + (income - 171550) * 0.33;
else
tax = 8350 * 0.10 + (33950 - 8350) * 0.15 + (82250 - 33950) * 0.25 + (171550 - 82250) * 0.28 + (372950 - 171550) * 0.33 + (income - 372950) * 0.35;
}
else if (status == 1){
if (income <= 16700)
tax = income * 0.10;
else if (income <= 67900)
tax = 16700 * 0.10 + (income - 16700) * 0.15;
else if (income <= 137050)
tax = 16700 * 0.10 + (67900 - 16700) * 0.15 + (income - 67900) * 0.25;
else if (income <= 208850)
tax = 16700 * 0.10 + (67900 - 16700) * 0.15 + (137050 - 67900) * 0.25 + (income - 137050) * 0.28;
else if (income <= 372950)
tax = 16700 * 0.10 + (67900 - 16700) * 0.15 + (137050 - 67900) * 0.25 + (208850 - 137050) * 0.28 + (income - 208850) * 0.33;
else
tax = 16700 * 0.10 + (67900 - 16700) * 0.15 + (137050 - 67900) * 0.25 + (208850 - 137050) * 0.28 + (372950 - 208850) * 0.33 + (income - 372950) * 0.35;
}
else if (status == 2){
if (income <= 8350)
tax = income * 0.10;
else if (income <= 33950)
tax = 8350 * 0.10 + (income - 8350) * 0.15;
else if (income <= 68525)
tax = 8350 * 0.10 + (33950 - 8350) * 0.15 + (income - 33950) * 0.25;
else if (income <= 104425)
tax = 8350 * 0.10 + (33950 - 8350) * 0.15 + (68525 - 33950) * 0.25 + (income - 68525) * 0.28;
else if (income <= 186475)
tax = 8350 * 0.10 + (33950 - 8350) * 0.15 + (68525 - 33950) * 0.25 + (104425 - 68525) * 0.28 + (income - 104425) * 0.33;
else
tax = 8350 * 0.10 + (33950 - 8350) * 0.15 + (68525 - 33950) * 0.25 + (104425 - 68525) * 0.28 + (186475 - 104425) * 0.33 + (income - 186475) * 0.35;
}
else{
if (income <= 11950)
tax = income * 0.10;
else if (income <= 45500)
tax = 11950 * 0.10 + (income - 11950) * 0.15;
else if (income <= 117450)
tax = 11950 * 0.10 + (45500 - 11950) * 0.15 + (income - 45500) * 0.25;
else if (income <= 190200)
tax = 11950 * 0.10 + (45500 - 11950) * 0.15 + (117451 - 45500) * 0.25 + (income - 117451) * 0.28;
else if (income <= 375950)
tax = 11950 * 0.10 + (45500 - 11950) * 0.15 + (117451 - 45500) * 0.25 + (190200- 117451) * 0.28 + (income - 190200) * 0.33;
else
tax = 11950 * 0.10 + (45500 - 11950) * 0.15 + (117451 - 45500) * 0.25 + (190200- 117451) * 0.28 + (372950 - 190200) * 0.33 + (income - 372950) * 0.35;
}
System.out.println("Tax is " + (int)(tax * 100) / 100.0);
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
int result = (int)(Math.random() *100 % 2);
Scanner input = new Scanner(System.in);
System.out.print("Enter your guess: ");
int guess = input.nextInt();
if (guess == result)
System.out.println("Your guess is right!");
else
System.out.println("Your guess not is right!");
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
int lottery = (int)(Math.random() * 1000);
Scanner input = new Scanner(System.in);
System.out.print("Enter your lottery (three digits): ");
int guess = input.nextInt();
int retain_lottery=lottery;
int lotteryDigit1 = lottery / 100;
lottery = lottery % 100;
int lotteryDigit2 = lottery / 10;
int lotteryDigit3 = lottery % 10;
int guessDigit1 = guess / 100;
guess = guess % 100;
int guessDigit2 = guess / 10;
int guessDigit3 = guess % 10;
System.out.println("The lottery is " + retain_lottery);
if (guess == lottery)
System.out.println("Exact match: you win $10,000");
else if (guessDigit1 == lotteryDigit2 && guessDigit2 == lotteryDigit3 && guessDigit3 ==lotteryDigit1)
System.out.println("Exact match: you win $3,000");
else if (guessDigit1 == lotteryDigit1 ||guessDigit2 == lotteryDigit2 || guessDigit3 == lotteryDigit3)
System.out.println("Exact match: you win $1,000");
else
System.out.println("Sorry,no match");
}
}

import java.util.Random;
public class tt1 {
public static void main(String args[]){
int maxX = 50 , minX = -50;
int maxY = 100 , minY = -100;
Random random = new Random();
int x = random.nextInt(maxX) % (maxX - minX +1) + minX;
int y = random.nextInt(maxY) % (maxY - minY +1) + minY;
System.out.println("( " + x + " , " + y + " )");
}
}
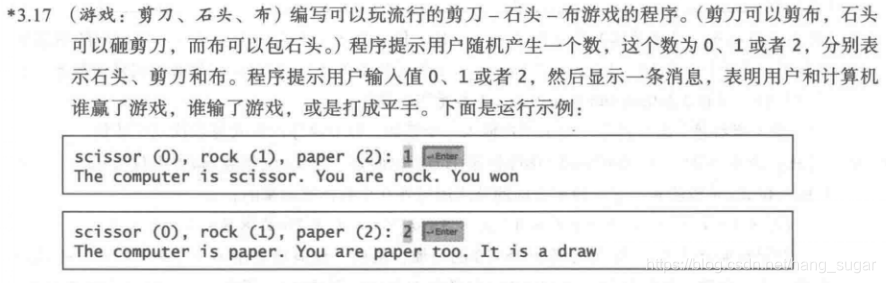
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
int computer = (int)(Math.random() * 100 % 3);
Scanner input = new Scanner(System.in);
System.out.print("scissor (0), rock (1), paper (2): ");
int you = input.nextInt();
if(computer == you){
if(computer == 0)
System.out.println("The computer is rock. You are rock too. Tt is a draw ");
if(computer == 1)
System.out.println("The computer is scissor. You are scissor too. Tt is a draw ");
if(computer == 3)
System.out.println("The computer is paper. You are paper too. Tt is a draw ");
}
else if (computer == 0){
if(you == 1)
System.out.println("The computer is rock. You are scissor. You lost");
if(you == 2)
System.out.println("The computer is rock. You are paper. You win");
}
else if (computer == 1){
if(you == 0)
System.out.println("The computer is scissor. You are rock. You win");
if(you == 2)
System.out.println("The computer is scissor. You are paper. You lost");
}
else if (computer == 2){
if(you == 0)
System.out.println("The computer is paper. You are rock. You lost");
if(you == 1)
System.out.println("The computer is paper. You are scissor. You win");
}
}
}

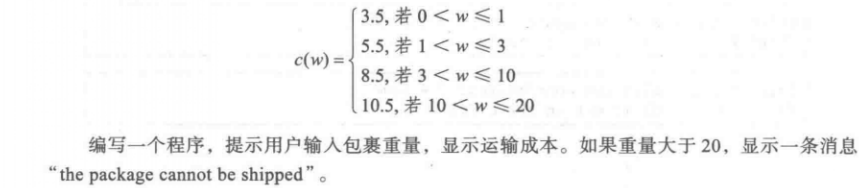
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter weight: ");
int w = input.nextInt();
if(w > 0 && w <=1)
System.out.println("The dollar is $3.5");
else if (w >1 && w <= 3)
System.out.println("The dollar is $5.5");
else if (w > 3 && w <= 10)
System.out.println("The dollar is $8.5");
else if (w > 10 && w <= 20)
System.out.println("The dollar is $10.5");
else
System.out.println("the package cannot be shipped");
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter three side: ");
int a = input.nextInt();
int b = input.nextInt();
int c = input.nextInt();
if((a * a + b * b) >(c * c) && (a * a + c * c) >(b * b) && (c * c + b * b) >(a * a))
System.out.println("This is legal!");
else
System.out.println("This is illegal");
}
}

import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter the temperature in Fahrenheit between -58F and 41F :");
double t = input.nextDouble();
System.out.print("Enter the wind speed (>-2) in miles per hour:");
double v = input.nextDouble();
double Twc;
if((t >= -58 && t <=41) && (v >= 2)){
Twc = 35.74 + 0.6215 * t - 35.75 * Math.pow(v,0.16) + 0.4275 * t * Math.pow(v,0.16);
Twc = (int)(Twc * 100000);
Twc = Twc / 100000;
System.out.println("The wind chill index is " + Twc);
}
else
System.out.println("The open temperature or wind speed is illegal");
}
}
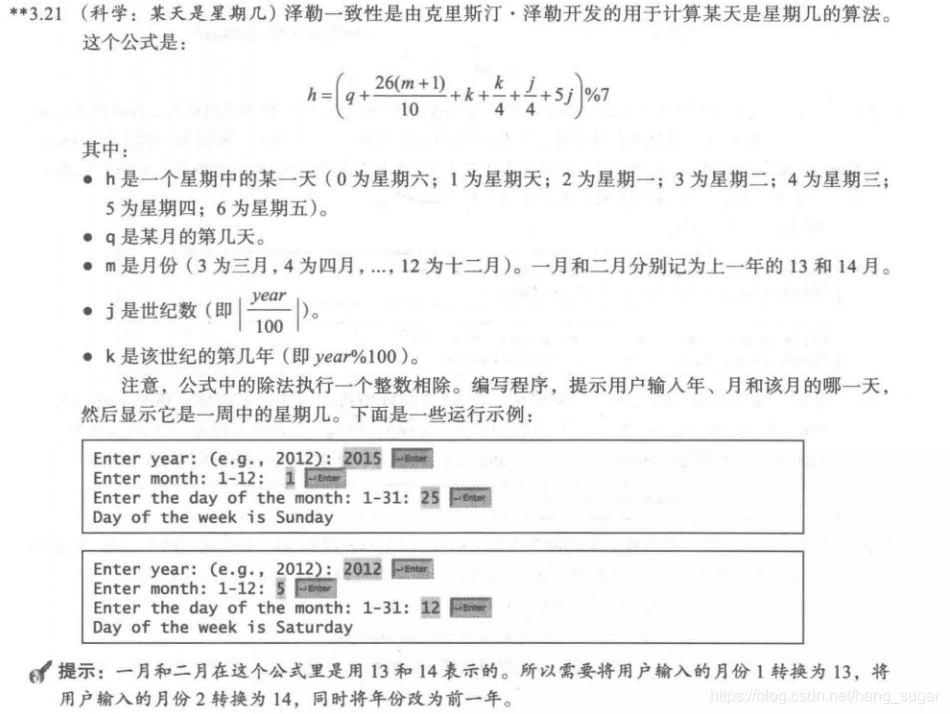
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter year:(e.g., 2012):");
int year = input.nextInt();
System.out.print("Enter month: 1-12:");
int m = input.nextInt();
if (m == 1){
m = 13;
year = year -1;
}
if (m == 2) {
m = 14;
year = year -1;
}
System.out.print("Enter the day of the month: 1-31:");
int q = input.nextInt();
int j = year / 100;
int k = year % 100;
int h = (q + 26 * (m + 1) / 10 + k + k / 4 + j / 4 + 5 * j) % 7;
switch (h){
case 0 : System.out.println("Day of the week is Saturday");break;
case 1 : System.out.println("Day of the week is Sunday");break;
case 2 : System.out.println("Day of the week is Monday");break;
case 3 : System.out.println("Day of the week is Tuesday");break;
case 4 : System.out.println("Day of the week is Wednesday");break;
case 5 : System.out.println("Day of the week is Thursday");break;
case 6 : System.out.println("Day of the week is Friday");break;
}
}
}

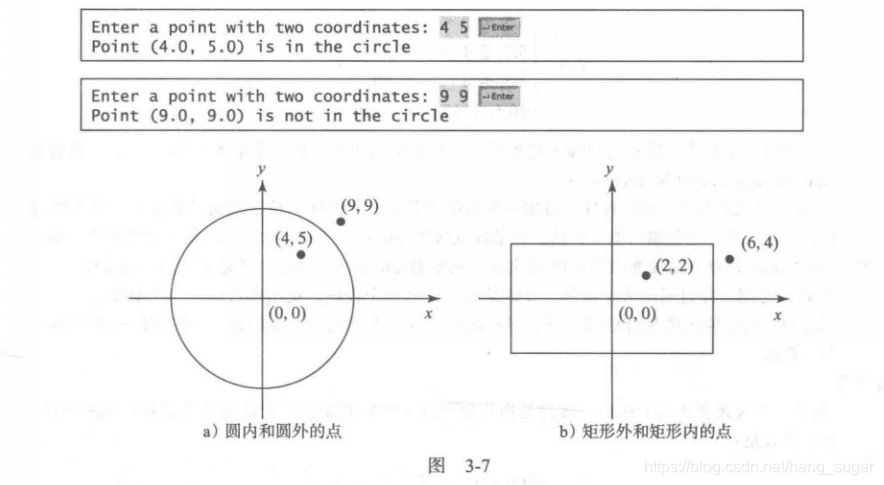
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter a point with two coordinates: ");
double x = input.nextDouble();
double y = input.nextDouble();
Double x0 = 0.0 , y0 = 0.0 ;
double length = Math.pow(Math.pow(x - x0 , 2) + Math.pow(y - y0 , 2), 0.5);
if (length <= 10.0)
System.out.println("Point (" + x + " , " + y + ") is in the circle");
else
System.out.println("Point (" + x + " , " + y + ") is not in the circle");
}
}
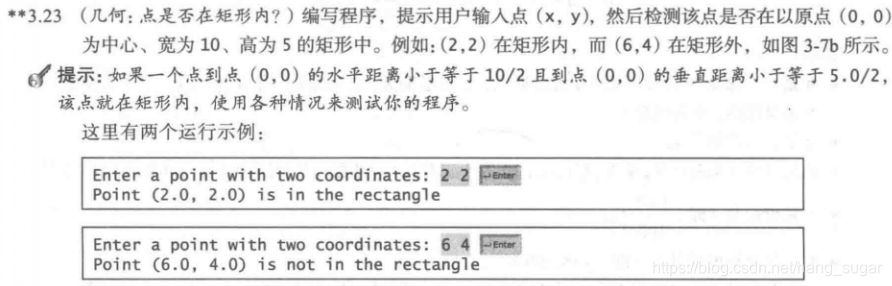
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter a point with two coordinates: ");
double x = input.nextDouble();
double y = input.nextDouble();
if (x <= 10.0 / 2 && x >= -(10.0 /2) && y <= 5.0 / 2 && y >= -(5.0 / 2))
System.out.println("Point (" + x + " , " + y + ") is in the rectangle");
else
System.out.println("Point (" + x + " , " + y + ") is not in the rectangle");
}
}

public class tt1 {
public static void main(String args[]){
int number = (int)(Math.random() * 13 + 1);
int flower = (int)(Math.random() * 4 + 1);
System.out.print("The card you pick is ");
switch (number){
case 1 : System.out.print("Ace ");break;
case 2 : System.out.print("2 ");break;
case 3 : System.out.print("3 ");break;
case 4 : System.out.print("4 ");break;
case 5 : System.out.print("5 ");break;
case 6 : System.out.print("6 ");break;
case 7 : System.out.print("7 ");break;
case 8 : System.out.print("8 ");break;
case 9 : System.out.print("9 ");break;
case 10 : System.out.print("10 ");break;
case 11 : System.out.print("Jack ");break;
case 12 : System.out.print("Queen ");break;
case 13 : System.out.print("King ");break;
}
switch (flower){
case 1 : System.out.print(" of Clubs");break;
case 2 : System.out.print(" of Diamonds");break;
case 3 : System.out.print(" of Hearts");break;
case 4 : System.out.print(" of Spades");break;
}
}
}
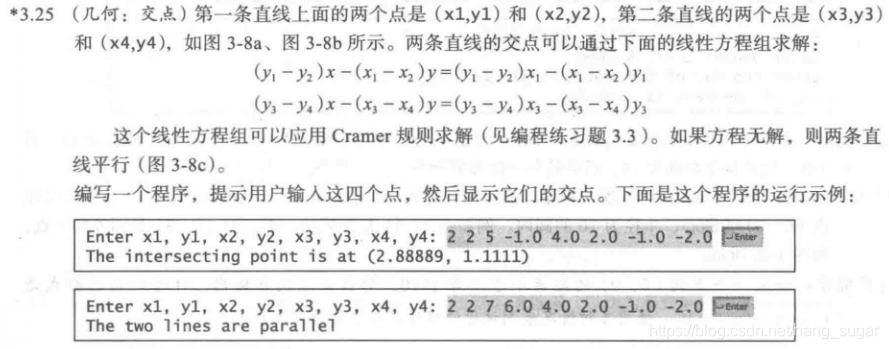
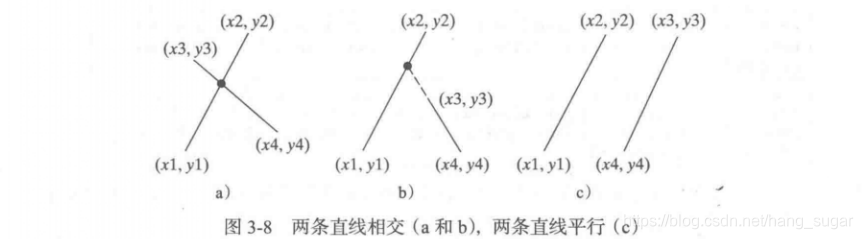
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter x1, y1, x2, y2, x3, y3, x4, y4:");
double x1 = input.nextDouble();
double y1 = input.nextDouble();
double x2 = input.nextDouble();
double y2 = input.nextDouble();
double x3 = input.nextDouble();
double y3 = input.nextDouble();
double x4 = input.nextDouble();
double y4 = input.nextDouble();
double t = (y1-y2)*(x4-x3)-(y3-y4)*(x2-x1);
if ( t != 0 ){
double x = (((y1-y2)*x1-(x1-x2)*y1)*(x4-x3) - (x2-x1)*((y3-y4)*x3-(x3-x4)*y3)) / t;
double y = (((y3-y4)*x3-(x3-x4)*y3)*(y1-y2) - (y3-y4)*((y1-y2)*x1-(x1-x2)*y1)) / t;
System.out.println("The intersecting point is at ( " + x + " , " + y + " )");
}
else
System.out.println("The two lines are parallel");
}
}
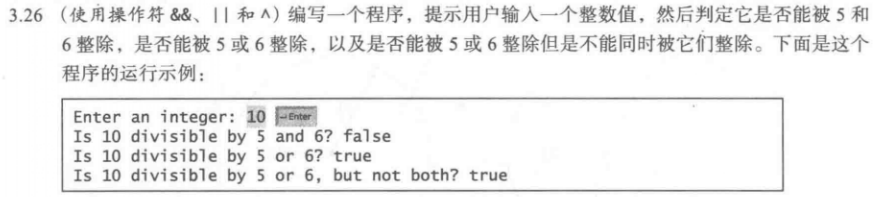
import java.util.Scanner;
public class tt1 {
public static void main(String args[]){
Scanner input = new Scanner(System.in);
System.out.print("Enter an integer: ");
int number = input.nextInt();
System.out.print("Is 10 divisible by 5 and 6? ");
if(number / 6 == 1 && number / 5 == 1)
System.out.println("true");
else
System.out.println("false");
System.out.print("Is 10 divisible by 5 or 6? ");
if(number / 6 == 1 || number / 5 == 1)
System.out.println("true");
else
System.out.println("false");
System.out.print("Is 10 divisible by 5 or 6,but not both? ");
if((number / 6 == 1 && number / 5 != 1) || (number / 6 !=1 && number / 5 ==1))
System.out.println("true");
else
System.out.println("false");
}
}