【UnrolledLinkedList2023】2023全国大学生软件测试大赛开发者测试省赛覆盖率高分答案
题目详情
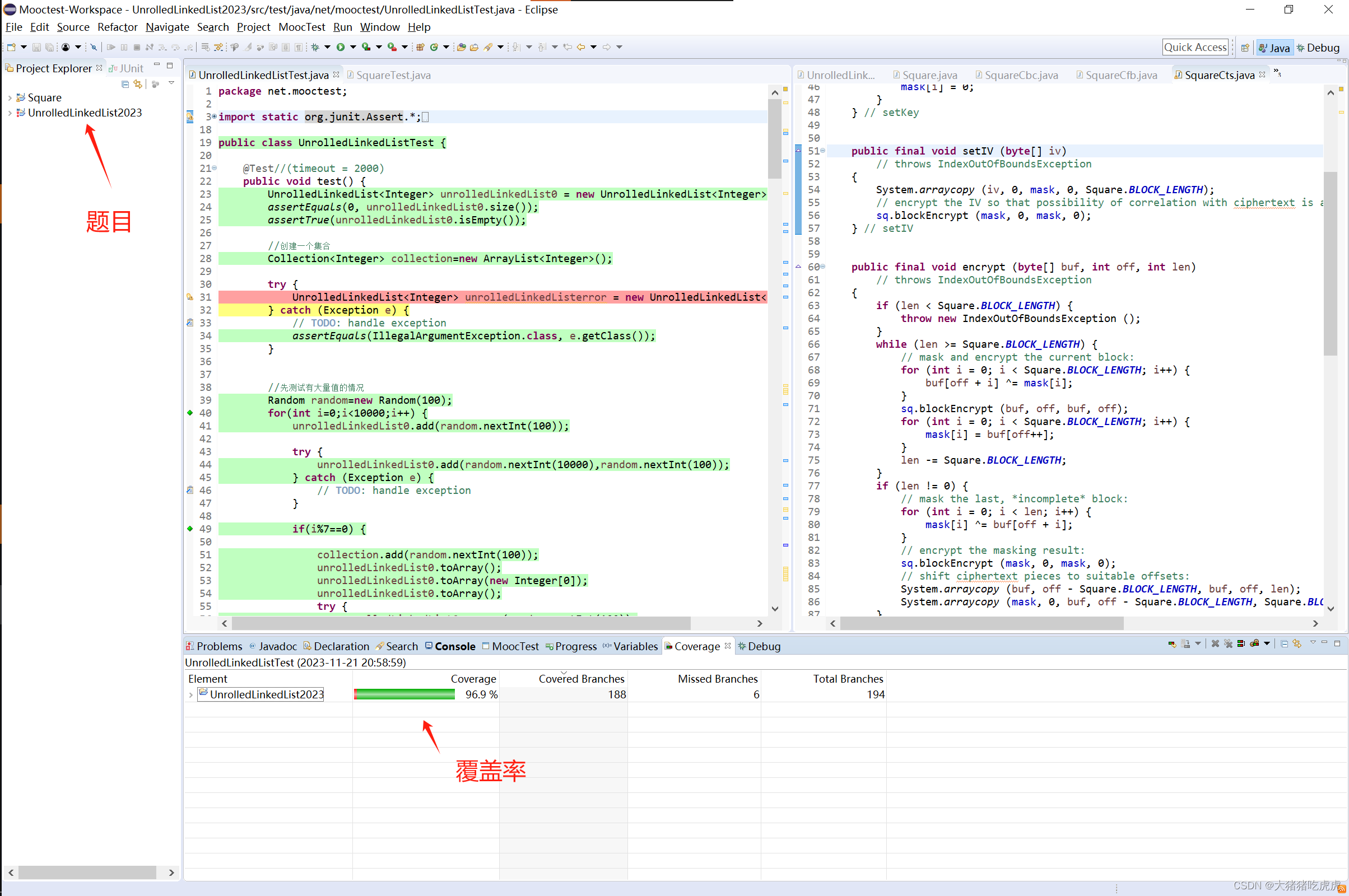
题解代码(直接全部复制到test类中即可)
package net.mooctest;
import static org.junit.Assert.*;
import java.util.ArrayList;
import java.util.Collection;
import java.util.ConcurrentModificationException;
import java.util.Iterator;
import java.util.ListIterator;
import java.util.NoSuchElementException;
import java.util.Random;
import org.junit.Test;
import org.omg.CORBA.INTERNAL;
import net.mooctest.UnrolledLinkedList.Node;
import net.mooctest.UnrolledLinkedList.ULLIterator;
public class UnrolledLinkedListTest {
@Test
public void test() {
UnrolledLinkedList<Integer> unrolledLinkedList0 = new UnrolledLinkedList<Integer>();
assertEquals(0, unrolledLinkedList0.size());
assertTrue(unrolledLinkedList0.isEmpty());
Collection<Integer> collection=new ArrayList<Integer>();
try {
UnrolledLinkedList<Integer> unrolledLinkedListerror = new UnrolledLinkedList<Integer>(5);
} catch (Exception e) {
assertEquals(IllegalArgumentException.class, e.getClass());
}
Random random=new Random(100);
for(int i=0;i<10000;i++) {
unrolledLinkedList0.add(random.nextInt(100));
try {
unrolledLinkedList0.add(random.nextInt(10000),random.nextInt(100));
} catch (Exception e) {
}
if(i%7==0) {
collection.add(random.nextInt(100));
unrolledLinkedList0.toArray();
unrolledLinkedList0.toArray(new Integer[0]);
unrolledLinkedList0.toArray();
try {
unrolledLinkedList0.remove(random.nextInt(100));
unrolledLinkedList0.indexOf(random.nextInt(100));
unrolledLinkedList0.lastIndexOf(random.nextInt(100));
unrolledLinkedList0.set(random.nextInt(10000), random.nextInt(100));
unrolledLinkedList0.get(random.nextInt(10000));
unrolledLinkedList0.listIterator(random.nextInt(100));
Iterator<Integer> iterator = unrolledLinkedList0.iterator();
ListIterator<Integer> listIterator = unrolledLinkedList0.listIterator();
while (listIterator.hasNext()) {
listIterator.next();
}
while (listIterator.hasPrevious()) {
listIterator.previous();
}
try {
listIterator.nextIndex();
listIterator.previousIndex();
listIterator.remove();
} catch (Exception e) {
}
} catch (Exception e) {
}
}
}
assertEquals(false, unrolledLinkedList0.isEmpty());
assertEquals(false, unrolledLinkedList0.remove((Object) null));
assertEquals(-1, unrolledLinkedList0.indexOf((Object) null));
assertEquals(-1, unrolledLinkedList0.lastIndexOf((Object) null));
try {
unrolledLinkedList0.retainAll(null);
} catch (Exception e) {
assertEquals(NullPointerException.class, e.getClass());
}
try {
unrolledLinkedList0.removeAll(null);
} catch (Exception e) {
assertEquals(NullPointerException.class, e.getClass());
}
try {
unrolledLinkedList0.addAll(null);
} catch (Exception e) {
assertEquals(NullPointerException.class, e.getClass());
}
try {
unrolledLinkedList0.containsAll(null);
} catch (Exception e) {
assertEquals(NullPointerException.class, e.getClass());
}
assertEquals(true, unrolledLinkedList0.containsAll(collection));
assertEquals(true, unrolledLinkedList0.addAll(collection));
assertEquals(false, unrolledLinkedList0.retainAll(collection));
assertEquals(true, unrolledLinkedList0.removeAll(collection));
try {
unrolledLinkedList0.listIterator(-1);
} catch (Exception e) {
assertEquals(IndexOutOfBoundsException.class, e.getClass());
}
UnrolledLinkedList<Integer> unrolledLinkedList1 = new UnrolledLinkedList<Integer>();
unrolledLinkedList1.size=10;
for(int i=0;i<=10;i++) {
unrolledLinkedList1.add(i);
}
UnrolledLinkedList<Integer> unrolledLinkedList2 = new UnrolledLinkedList<Integer>();
for(int i=0;i<3;i++) {
unrolledLinkedList2.add(i);
}
Integer[] as=new Integer[3];
as[0]=0;
as[1]=1;
as[2]=2;
assertArrayEquals(as,unrolledLinkedList2.toArray() );
assertArrayEquals(as,unrolledLinkedList2.toArray(new Integer[3]) );
Integer integer1=1;
Integer integer5=5;
Integer integer2=2;
assertEquals(integer1, unrolledLinkedList2.<Integer>get(1));
assertEquals(integer2, unrolledLinkedList2.<Integer>get(2));
assertEquals(integer2, unrolledLinkedList2.<Integer>remove(2));
assertEquals(false, unrolledLinkedList2.remove(null));
try {
unrolledLinkedList1.listIterator(20);
} catch (Exception e) {
}
Integer[] a=new Integer[50];
assertArrayEquals(a,unrolledLinkedList1.toArray(a));
Collection<Integer> collection2=new ArrayList<>();
collection2.add(50);
assertEquals(false, unrolledLinkedList1.contains(20));
assertEquals(false, unrolledLinkedList1.containsAll(collection2));
assertEquals(false, unrolledLinkedList1.removeAll(collection2));
assertEquals(true, unrolledLinkedList1.retainAll(collection2));
ListIterator<Integer> listIterator1 = unrolledLinkedList0.listIterator();
while (listIterator1.hasPrevious()) {
listIterator1.previous();
}
try {
listIterator1.previous();
} catch (Exception e) {
assertEquals(NoSuchElementException.class, e.getClass());
}
ListIterator<Integer> listIterator2 = unrolledLinkedList0.listIterator();
while (listIterator2.hasNext()) {
listIterator2.next();
}
try {
listIterator2.next();
} catch (Exception e) {
assertEquals(NoSuchElementException.class, e.getClass());
}
unrolledLinkedList1.size=100;
try {
unrolledLinkedList1.listIterator(60);
} catch (Exception e) {
}
assertEquals(true, unrolledLinkedList1.add(1));
ULLIterator uIterator=unrolledLinkedList0.new ULLIterator(null, 0, 0);
uIterator.expectedModCount=10;
try {
uIterator.checkForModification();
} catch (Exception e) {
assertEquals(ConcurrentModificationException.class, e.getClass());
}
assertEquals(false, unrolledLinkedList1.remove(null));
assertEquals(false, unrolledLinkedList0.remove(null));
UnrolledLinkedList<Integer> unrolledLinkedList3 = new UnrolledLinkedList<Integer>(16);
unrolledLinkedList3.add(null);
unrolledLinkedList3.add(null);
unrolledLinkedList3.add(null);
assertEquals(2, unrolledLinkedList3.lastIndexOf(null));
assertEquals(0, unrolledLinkedList3.indexOf(null));
assertEquals(true, unrolledLinkedList3.remove(null));
unrolledLinkedList3.add(null);
unrolledLinkedList3.add(null);
unrolledLinkedList3.add(2);
unrolledLinkedList3.add(3);
assertEquals(true, unrolledLinkedList3.remove(null));
Node node=unrolledLinkedList0.new Node();
Node node1=unrolledLinkedList0.new Node();
Node node2=unrolledLinkedList0.new Node();
node.next=node1;
node1.next=null;
unrolledLinkedList1.lastNode=node1;
unrolledLinkedList1.mergeWithNextNode(node);
unrolledLinkedList0.clear();
}
}