1. FreeTextBox.js
/**/
/**************************************************************
*
* FreeTextBox編輯器
* 作者:limin_he(limin_he@maxense.com)
* 日期:2006-10-30
*
**************************************************************/


//
FreeTextBox對象
function
FreeTextBox(panel)

...
{
this.panelObject = panel; //當前對象
this.isIe = true; //當前瀏覽器是否為IE
this.editMode = "designMode"; //當前編輯模式
this.designEditor = null; //設計編輯器
this.htmlEditor = null; //HTML編輯器
this.Width = 650;
this.Height = 60;
this.MaxHeight = 360;
//this.Init(); //初使化
}

//
對象初使化
FreeTextBox.prototype.Init
=
function
()

...
{
//創建框架
function CreateFrame(sender)

...{
var div = document.createElement("DIV");
div.style.width = sender.Width;
return div;
}
//創建工具欄
function CreateToolbar(sender)

...{
var toolBar = new FreeTextBox.ToolBar(sender);
return toolBar.toolBar;
}
//創建設計編輯區
function CreateDesignEditor(sender)

...{
var iframe = document.createElement("iframe");
iframe.style.width = sender.Width;
iframe.style.height = sender.Height;
return iframe;
}
//創建Html編輯區
function CreateHtmlEditor(sender)

...{
var textArea = document.createElement("textarea");
textArea.style.width = sender.Width;
textArea.style.height = sender.Height;
textArea.style.border = "solid 1px #cccccc";
return textArea;
}
//設計編輯區初使化
function DesignEditor_Init(sender,e)

...{
var doc = sender.contentWindow.document;
doc.open();
doc.write("<html><body topmargin='0px' leftmargin='0' rightmargin='0' bottommargin='0' ></body></html>");
doc.close();
doc.body.contentEditable = true;
doc.sender = sender;
doc.e = e;
doc.onkeydown = function()

...{
if(this.sender.contentWindow.event.keyCode==13)

...{
if(e.MaxHeight>=e.Height)

...{
this.sender.style.height = parseInt(e.Height) + 20;
e.Height = parseInt(this.sender.style.height);
}
}
}
}
//Html編輯區初使化
function HtmlEditor_Init(sender,e)

...{
sender.style.display = "none";
sender.onkeydown = function()

...{
if(event.keyCode==13)

...{
if(e.MaxHeight>=e.Height)

...{
this.style.height = parseInt(e.Height) + 20;
e.Height = parseInt(this.style.height);
}
}
}
}
//創建狀態欄
function CreateStatus(sender)

...{
var div = document.createElement("DIV");
div.style.marginTop = -3;
var btnDesign = document.createElement("INPUT");
btnDesign.type = "Button";
btnDesign.value = "設計";
btnDesign.disabled = true;
btnDesign.style.width = "60px";
btnDesign.style.height = "30px";
btnDesign.style.backgroundColor = "#FFFFFF";
btnDesign.style.border = "solid 1px #cccccc";
btnDesign.style.cursor = "hand";
var btnHtml = document.createElement("INPUT");
btnHtml.type = "Button";
btnHtml.value = "HTML";
btnHtml.style.width = "60px";
btnHtml.style.height = "30px";
btnHtml.style.border = "solid 1px #cccccc";
btnHtml.style.cursor = "hand";
var btnBrowse = document.createElement("INPUT");
btnBrowse.type = "Button";
btnBrowse.value = "瀏覽";
btnBrowse.style.width = "60px";
btnBrowse.style.height = "30px";
btnBrowse.style.border = "solid 1px #cccccc";
btnBrowse.style.cursor = "hand";
div.appendChild(btnDesign);
div.appendChild(btnHtml);
div.appendChild(btnBrowse);
btnDesign.sender = sender;
btnHtml.sender = sender;
btnBrowse.sender = sender;
btnDesign.btnHtml = btnHtml;
btnHtml.btnDesign = btnDesign;
btnDesign.onclick = function()

...{
this.sender.setMode("designMode");
this.disabled = true;
this.btnHtml.disabled = false;
this.btnHtml.style.backgroundColor = "";
this.style.backgroundColor = "#FFFFFF";
this.style.borderTop = "0px";
}
btnHtml.onclick = function()

...{
this.sender.setMode("htmlMode");
this.disabled = true;
this.btnDesign.disabled = false;
this.btnDesign.style.backgroundColor = "";
this.style.backgroundColor = "#FFFFFF";
this.style.borderTop = "0px";

}
btnBrowse.onclick = function()

...{
var newwin = window.open("about:blank");
newwin.document.write(this.sender.innerHTML());
}
return div;
}
var panel = CreateFrame(this); //主對象
var toolbar = CreateToolbar(this); //工具欄
this.designEditor = CreateDesignEditor(this); //設計編輯區
this.htmlEditor = CreateHtmlEditor(this); //HTML編輯區
var statusbar = CreateStatus(this); //狀態欄
panel.appendChild(toolbar);
panel.appendChild(this.designEditor);
panel.appendChild(this.htmlEditor);
panel.appendChild(statusbar);
this.panelObject.appendChild(panel);
if(this.editMode == "designMode")

...{
//當前為設計模式
DesignEditor_Init(this.designEditor,this); //初使化編輯區
HtmlEditor_Init(this.htmlEditor,this);
}
else

...{
//當前為HTML模式
}
}

//
執行命定
FreeTextBox.prototype.execCommand
=
function
(cmdID, UI, param)

...
{
this.focusEditor();
cmdID = cmdID.toLowerCase();
switch (cmdID)

...{
case "insertimage":
this.insertImage();
break;
case "inserttable":
this.insertTable();
break;
case "createlink":
this.createLink();
break;
case "forecolor":
var doc = this.designEditor.contentWindow.document;
var rec = window.showModalDialog("../FTB/FTB_SelectColor.aspx","","dialogWidth:400px;DialogHeight=350px;status:no");
if(rec!=null)

...{
this.designEditor.contentWindow.document.execCommand("forecolor", false, rec);
}
break;
case "backcolor":
var doc = this.designEditor.contentWindow.document;
var rec = window.showModalDialog("../FTB/FTB_SelectColor.aspx","","dialogWidth:400px;DialogHeight=350px;status:no");
if(rec!=null)

...{
this.designEditor.contentWindow.document.execCommand("BackColor", false, rec);
}
break;
case "saveas":
this.designEditor.contentWindow.document.execCommand("SaveAs", UI, "*.htm");
break;
case "lefttoright":
var el = this.getParentElement();
el.style.direction = "ltr";
break;
case "righttoleft":
var el = this.getParentElement();
el.style.direction = "rtl";
break;
default:this.designEditor.contentWindow.document.execCommand(cmdID, UI, param);
}
}

//
插入圖片
FreeTextBox.prototype.insertImage
=
function
()

...
{
this.focusEditor();
var doc = this.designEditor.contentWindow.document;
var rec = window.showModalDialog("../FTB/FTB_InsertImage.aspx?if=&cif=&icon=&flags=9","","dialogWidth:600px;DialogHeight=460px;status:no");
if(rec!=null)

...{
var sel = this.getSelection();
var range = this.createRange(sel);
this.designEditor.contentWindow.document.execCommand("insertimage", false, rec["filename"]);
var img = this.getParentElement();
img.alt = rec["desc"];
img.style.width = rec["width"];
img.style.height = rec["height"];
}
}

//
插入表格
FreeTextBox.prototype.insertTable
=
function
()

...
{
this.focusEditor();
var doc = this.designEditor.contentWindow.document;
var rec = window.showModalDialog("../FTB/FTB_InsertTable.aspx","","dialogWidth:430px;DialogHeight=250px;status:no");
if(rec!=null)

...{
var sel = this.getSelection();
var range = this.createRange(sel);
var table = this.designEditor.contentWindow.document.createElement("table");
table.style.width = rec["width"];
table.style.height = rec["height"];
table.border = rec["border"];
table.cellpadding = rec["cellpadding"];
table.cellspacing = rec["cellspacing"];
table.summary = rec["desc"];
var tbody = doc.createElement("tbody");
table.appendChild(tbody);
for(var i=0; i<rec["rows"]; ++i)

...{
var tr = doc.createElement("tr");
tbody.appendChild(tr);
for (var j = 0; j < rec["cols"]; ++j)

...{
var td = doc.createElement("td");
td.valign = rec["valigncells"];
td.align = rec["haligncells"];
tr.appendChild(td);
}
}
if(this.isIe)

...{
range.pasteHTML(table.outerHTML);
}
else

...{
this.designEditor.insertNodeAtSelection(table);
}
}
}

//
創建連接
FreeTextBox.prototype.createLink
=
function
()

...
{
this.focusEditor();
var doc = this.designEditor.contentWindow.document;
var rec = window.showModalDialog("../FTB/FTB_CreateLink.aspx","","dialogWidth:430px;DialogHeight=200px;status:no");
if(rec!=null)

...{
var sel = this.getSelection();
var range = this.createRange(sel);
this.designEditor.contentWindow.document.execCommand("createlink", false, rec["url"]);
var a = this.getParentElement();
a.target = rec["target"];
a.title = rec["title"];
}
}

//
設置顯示模式
FreeTextBox.prototype.setMode
=
function
(mode)

...
{
if (typeof mode == "undefined")

...{
mode = ((this.editMode == "htmlMode") ? "designMode" : "htmlMode");
}
switch (mode)

...{
case "htmlMode":
this.htmlEditor.style.display = "block";
this.designEditor.style.display = "none";
this.htmlEditor.style.height = this.Height;
this.htmlEditor.value = this.outerHTML();
this.editMode = "htmlMode";
break;
case "designMode":
this.htmlEditor.style.display = "none";
this.designEditor.style.display = "block";
this.designEditor.style.height = this.Height;
var str = this.outerHTML();
str = str.replace("<html><body topmargin='0px' leftmargin='0' rightmargin='0' bottommargin='0' >","");
str = str.replace("</body></html>","");
this.designEditor.contentWindow.document.body.innerHTML = str;
this.editMode = "designMode";
break;
}
}

//
獲得或設置自己的內容
FreeTextBox.prototype.outerHTML
=
function
(value)

...
{
if(value==null)

...{
if(this.editMode=="designMode")

...{
return "<html><body topmargin='0px' leftmargin='0' rightmargin='0' bottommargin='0' >" + this.designEditor.contentWindow.document.body.innerHTML + "</body></html>";
}
else if(this.editMode=="htmlMode")

...{
return this.htmlEditor.value;
}
}
else

...{
if(this.editMode=="designMode")

...{
this.designEditor.contentWindow.document.body.innerHTML = value;
}
else if(this.editMode=="htmlMode")

...{
this.htmlEditor.value = value;
}
}
}
//
獲得或設置內容
FreeTextBox.prototype.innerHTML
=
function
(value)

...
{
if(value==null)

...{
if(this.editMode=="designMode")

...{
return this.designEditor.contentWindow.document.body.innerHTML;
}
else if(this.editMode=="htmlMode")

...{
var str = this.htmlEditor.value;
str = str.replace("<html><body topmargin='0px' leftmargin='0' rightmargin='0' bottommargin='0' >","");
str = str.replace("</body></html>","");
return str;
}
}
else

...{
if(this.editMode=="designMode")

...{
this.designEditor.contentWindow.document.body.innerHTML = value;
}
else if(mode=="htmlMode")

...{
this.htmlEditor.value = value;
}
}
}

//
指定編輯區獲得焦點
FreeTextBox.prototype.focusEditor
=
function
()

...
{
if(this.editMode=="designMode")

...{
this.designEditor.contentWindow.focus();
}
else if(mode=="htmlMode")

...{
this.htmlEditor.focus();
}
}

//
獲得當前選擇對象的父對象
FreeTextBox.prototype.getParentElement
=
function
()

...
{
var sel = this.getSelection();
var range = this.createRange(sel);
if(this.isIe)

...{
switch (sel.type)

...{
case "Text":
case "None":
return range.parentElement();
case "Control":
return range.item(0);
default:
return this.designEditor.contentWindow.document.body;
}
}
else

...{
try

...{
var p = range.commonAncestorContainer;
if (!range.collapsed && range.startContainer == range.endContainer &&
range.startOffset - range.endOffset <= 1 && range.startContainer.hasChildNodes())
p = range.startContainer.childNodes[range.startOffset];
while (p.nodeType == 3)

...{
p = p.parentNode;
}
return p;
}
catch(e)

...{
return null;
}
}
}

//
獲得當前選擇的對象
FreeTextBox.prototype.getSelection
=
function
()

...
{
if(this.isIe)

...{
return this.designEditor.contentWindow.document.selection;
}
else

...{
return this.designEditor.contentWindow.getSelection();
}
}

//
獲得當前選擇區
FreeTextBox.prototype.createRange
=
function
(sender)

...
{
if(this.isIe)

...{
return sender.createRange();
}
else

...{
this.focusEditor();
if(typeof sender != "undefined")

...{
try

...{
return sender.getRangeAt(0);
}
catch(e)

...{
return this.designEditor.contentWindow.document.createRange();
}
}
else

...{
return this.designEditor.contentWindow.document.createRange();
}
}
}


//
工具欄對象
FreeTextBox.ToolBar
=
function
(sender)

...
{
this.toolBar = null;
this.sender = sender;
this.Init();
this.CreateTools();
}
//
工具欄初使化
FreeTextBox.ToolBar.prototype.Init
=
function
()

...
{
var div = document.createElement("DIV");
this.toolBar = div;
}
//
創建工具欄各工具
FreeTextBox.ToolBar.prototype.CreateTools
=
function
()

...
{
var div = document.createElement("DIV");
div.style.borderLeft="1px solid #EBEBEB";
div.style.borderRight="1px solid #EBEBEB";
//div.appendChild(this.CreateTool("split","FTB/images/officeXP/separator.gif",""));
div.appendChild(this.CreateTool("復原","../FTB/images/officeXP/undo.gif","undo"));
div.appendChild(this.CreateTool("重做","../FTB/images/officeXP/redo.gif","redo"));
div.appendChild(this.CreateTool("清除格式","../FTB/images/officeXP/removeformat.gif",""));
div.appendChild(this.CreateTool("split","../FTB/images/officeXP/separator.gif",""));
div.appendChild(this.CreateTool("剪切","../FTB/images/officeXP/cut.gif","cut"));
div.appendChild(this.CreateTool("刪除","../FTB/images/officeXP/delete.gif","delete"));
div.appendChild(this.CreateTool("粘貼","../FTB/images/officeXP/paste.gif","paste"));
div.appendChild(this.CreateTool("split","../FTB/images/officeXP/separator.gif",""));
div.appendChild(this.CreateTool("全屏對齊","../FTB/images/officeXP/justifyfull.gif","justifyfull"));
div.appendChild(this.CreateTool("左對齊","../FTB/images/officeXP/justifyleft.gif","justifyleft"));
div.appendChild(this.CreateTool("居中對劉","../FTB/images/officeXP/justifycenter.gif","justifycenter"));
div.appendChild(this.CreateTool("右對齊","../FTB/images/officeXP/justifyright.gif","justifyright"));
div.appendChild(this.CreateTool("split","../FTB/images/officeXP/separator.gif",""));
div.appendChild(this.CreateTool("粗體","../FTB/images/officeXP/bold.gif","bold"));
div.appendChild(this.CreateTool("斜體","../FTB/images/officeXP/italic.gif","italic"));
div.appendChild(this.CreateTool("下劃線","../FTB/images/officeXP/underline.gif","underline"));
div.appendChild(this.CreateTool("split","../FTB/images/officeXP/separator.gif",""));
div.appendChild(this.CreateTool("編號列表","../FTB/images/officeXP/numberedlist.gif","insertorderedlist"));
div.appendChild(this.CreateTool("列表項","../FTB/images/officeXP/bullets.gif","insertunorderedlist"));
div.appendChild(this.CreateTool("split","../FTB/images/officeXP/separator.gif",""));
div.appendChild(this.CreateTool("保存為","../FTB/images/officeXP/save.gif","SaveAs"));
div.appendChild(this.CreateTool("列印","../FTB/images/officeXP/print.gif","print"));
div.appendChild(this.CreateTool("split","../FTB/images/officeXP/separator.gif",""));
//var brCtrl = document.createElement("br");
//div.appendChild(brCtrl);
//div.appendChild(this.CreateTool("split","FTB/images/officeXP/separator.gif",""));
div.appendChild(this.CreateTool("縮進","../FTB/images/officeXP/indent.gif","indent"));
div.appendChild(this.CreateTool("取消縮進","../FTB/images/officeXP/outdent.gif","outdent"));
div.appendChild(this.CreateTool("split","../FTB/images/officeXP/separator.gif",""));
div.appendChild(this.CreateToolFontName());
div.appendChild(this.CreateToolFontSize());
div.appendChild(this.CreateTool("字體顏色","../FTB/images/officeXP/fontforecolorpicker.gif","forecolor"));
div.appendChild(this.CreateTool("背景色","../FTB/images/officeXP/fontbackcolorpicker.gif","backcolor"));
div.appendChild(this.CreateTool("split","../FTB/images/officeXP/separator.gif",""));
div.appendChild(this.CreateTool("插入圖片","../FTB/images/officeXP/insertimage.gif","insertimage"));
div.appendChild(this.CreateTool("插入表格","../FTB/images/officeXP/inserttable.gif","inserttable"));
div.appendChild(this.CreateTool("創建連接","../FTB/images/officeXP/createlink.gif","createlink"));
div.appendChild(this.CreateTool("清除連接","../FTB/images/officeXP/unlink.gif","unlink"));
div.appendChild(this.CreateTool("split","../FTB/images/officeXP/separator.gif",""));

this.toolBar.appendChild(div)
}

//
創建工具
FreeTextBox.ToolBar.prototype.CreateTool
=
function
(imgDesc,imgSrc,command)

...
{
var div = document.createElement("DIV");
div.style.display = "inline";
div.style.cursor = "hand";
div.sender = this.sender;
var img = document.createElement("img");
img.src = imgSrc;
img.title = imgDesc;
if(command !="")

...{
img.style.width = "21px";
img.style.height = "20px";
}
div.appendChild(img);
div.onclick = function()

...{
if(command !="")

...{
this.sender.execCommand(command);
}
}
div.onmouseover = function()

...{
this.style.border = "1px solid";
this.style.borderColor = "ButtonHighlight ButtonShadow ButtonShadow ButtonHighlight";
}
div.onmouseout = function()

...{
this.style.border = "";
this.style.borderColor = "";
}
return div;
}
//
創建工具-字體
FreeTextBox.ToolBar.prototype.CreateToolFontName
=
function
()

...
{
var e = document.createElement("select");
e.sender = this.sender;
e.title = "字體";
e.options[0] = new Option("Arial","Arial");
e.options[1] = new Option("Georgia","Georgia");
e.options[2] = new Option("Tahoma","Tahoma");
e.onchange = function()

...{
var v = this.options[this.selectedIndex].value;
this.sender.execCommand("fontname",false,v);
}
return e;
}
//
創建工具-字體大小
FreeTextBox.ToolBar.prototype.CreateToolFontSize
=
function
()

...
{
var e = document.createElement("select");
e.sender = this.sender;
e.title = "字體大小";
e.options[0] = new Option("字號","");
e.options[1] = new Option("1 (8pt)","1");
e.options[2] = new Option("2 (10pt)","2");
e.options[3] = new Option("3 (12pt)","3");
e.options[4] = new Option("4 (14pt)","4");
e.options[5] = new Option("5 (18pt)","5");
e.options[6] = new Option("6 (24pt)","6");
e.options[7] = new Option("7 (36pt)","7");
e.onchange = function()

...{
var v = this.options[this.selectedIndex].value;
if(v=="") v="12";
this.sender.execCommand("fontsize",false,parseInt(v));
}
return e;
}
2. 其中的圖片可以直接使用FreeTextBox中的圖片,彈出窗口也可以直接使用(只需修改下路徑)
3. 使用方法:
1. 引用./public/JScript/FreeTextBox.js這個文件
2. 創建對象
window.onload = function()
{
//設定編輯器中的內容
str = "設定編輯器中的內容";
//創建編輯器對象;參數為編輯器的容器
ftb1 = new FreeTextBox(document.all("free1"));
//編輯器的寬,默認為500
ftb1.Width = 600;
//編輯器的高度,默認為60
ftb1.Height = 60;
//編輯器的最大高度,默認為360
ftb1.MaxHeight = 400;
//初使化編輯器
ftb1.Init()
//設定編輯器的內容
ftb1.innerHTML(str);
ftb2 = new FreeTextBox(document.all("free2"));
ftb2.Width = 800;
ftb2.Height = 100;
ftb2.Init()
window.document.form1.onsubmit = checkForm;
}
function checkForm()
{
document.getElementById("FreeText1").value = ftb1.innerHTML();
document.getElementById("FreeText2").value = ftb2.innerHTML();
return true;
}
3.後湍取值
Response.Write("FreeTextBox1的值:" + FreeText1.Value + "")
Response.Write("FreeTextBox1的值:" + FreeText2.Value + "")
<input id="FreeText1" runat="server" type="hidden" />
<div id="free1" />
4. 樣式圖片
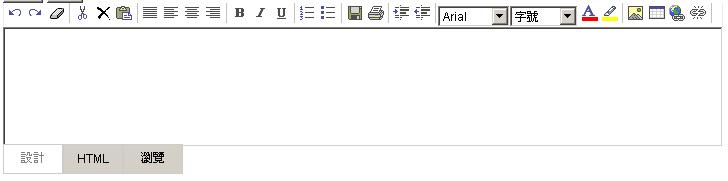