这段时间由于公司需要,在做C/S的东西,自然考虑优先用SWING实现,自然遇到了日期选择控件的问题,在网上GOOGLE了,发现了一个不错的控件,故转载之.
参考界面如下:
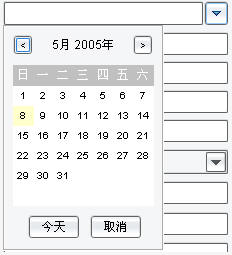
//
DatePicker.java
package
com.kxsoft.component;
//
修改
import
java.awt.
*
;
import
java.awt.event.
*
;
import
java.util.GregorianCalendar;
import
java.util.Date;
import
java.util.Calendar;
import
java.text.DateFormat;
import
java.text.FieldPosition;

import
javax.swing.
*
;
import
javax.swing.plaf.BorderUIResource;


public
final
class
DatePicker
extends
JPanel
...
{

private static final long serialVersionUID = 1L;

private static final int startX = 10;

private static final int startY = 60;

private static final Font smallFont = new Font("Dialog", Font.PLAIN, 10);

private static final Font largeFont = new Font("Dialog", Font.PLAIN, 12);

private static final Insets insets = new Insets(2, 2, 2, 2);

private static final Color highlight = new Color(255, 255, 204);

private static final Color white = new Color(255, 255, 255);

private static final Color gray = new Color(204, 204, 204);

private Component selectedDay = null;

private GregorianCalendar selectedDate = null;

private GregorianCalendar originalDate = null;
private boolean hideOnSelect = true;
private final JButton backButton = new JButton();
private final JLabel monthAndYear = new JLabel();
private final JButton forwardButton = new JButton();

private final JLabel[] dayHeadings = new JLabel[]...{
new JLabel("日"),
new JLabel("一"),
new JLabel("二"),
new JLabel("三"),
new JLabel("四"),
new JLabel("五"),
new JLabel("六")};

private final JLabel[][] daysInMonth = new JLabel[][]...{

...{new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel()},

...{new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel()},

...{new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel()},

...{new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel()},

...{new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel()},

...{new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel(),
new JLabel()}
};

private final JButton todayButton = new JButton();
private final JButton cancelButton = new JButton();


public DatePicker() ...{
super();
selectedDate = getToday();
init();
}

public DatePicker(final Date initialDate) ...{
super();
if (null == initialDate)
selectedDate = getToday();
else
(selectedDate = new GregorianCalendar()).setTime(initialDate);
originalDate = new GregorianCalendar(
selectedDate.get(Calendar.YEAR),
selectedDate.get(Calendar.MONTH),
selectedDate.get(Calendar.DATE));
init();
}

public boolean isHideOnSelect() ...{
return hideOnSelect;
}

public void setHideOnSelect(final boolean hideOnSelect) ...{

if (this.hideOnSelect != hideOnSelect) ...{
this.hideOnSelect = hideOnSelect;
initButtons(false);
}
}

public Date getDate() ...{
if (null != selectedDate)
return selectedDate.getTime();
return null;
}

private void init() ...{
setLayout(new AbsoluteLayout());
this.setMinimumSize(new Dimension(161, 226));
this.setMaximumSize(getMinimumSize());
this.setPreferredSize(getMinimumSize());
this.setBorder(new BorderUIResource.EtchedBorderUIResource());

backButton.setFont(smallFont);
backButton.setText("<");
backButton.setMargin(insets);
backButton.setDefaultCapable(false);

backButton.addActionListener(new ActionListener() ...{

public void actionPerformed(final ActionEvent evt) ...{
onBackClicked(evt);
}
});
add(backButton, new AbsoluteConstraints(10, 10, 20, 20));

monthAndYear.setFont(largeFont);
monthAndYear.setHorizontalAlignment(JTextField.CENTER);
monthAndYear.setText(formatDateText(selectedDate.getTime()));
add(monthAndYear, new AbsoluteConstraints(30, 10, 100, 20));
forwardButton.setFont(smallFont);
forwardButton.setText(">");
forwardButton.setMargin(insets);
forwardButton.setDefaultCapable(false);

forwardButton.addActionListener(new ActionListener() ...{

public void actionPerformed(final ActionEvent evt) ...{
onForwardClicked(evt);
}
});
add(forwardButton, new AbsoluteConstraints(130, 10, 20, 20));

int x = startX;

for (int ii = 0; ii < dayHeadings.length; ii++) ...{
dayHeadings[ii].setOpaque(true);
dayHeadings[ii].setBackground(Color.LIGHT_GRAY);
dayHeadings[ii].setForeground(Color.WHITE);
dayHeadings[ii].setHorizontalAlignment(JLabel.CENTER);
add(dayHeadings[ii], new AbsoluteConstraints(x, 40, 21, 21));
x += 20;
}
x = startX;
int y = startY;

for (int ii = 0; ii < daysInMonth.length; ii++) ...{

for (int jj = 0; jj < daysInMonth[ii].length; jj++) ...{
daysInMonth[ii][jj].setOpaque(true);
daysInMonth[ii][jj].setBackground(white);
daysInMonth[ii][jj].setFont(smallFont);
daysInMonth[ii][jj].setHorizontalAlignment(JLabel.CENTER);
daysInMonth[ii][jj].setText("");

daysInMonth[ii][jj].addMouseListener(new MouseAdapter() ...{

public void mouseClicked(final MouseEvent evt) ...{
onDayClicked(evt);
}
});
add(daysInMonth[ii][jj], new AbsoluteConstraints(x, y, 21, 21));
x += 20;
}
x = startX;
y += 20;
}

initButtons(true);

calculateCalendar();
}


private void initButtons(final boolean firstTime) ...{

if (firstTime) ...{
final Dimension buttonSize = new Dimension(68, 24);
todayButton.setText("今天");
todayButton.setMargin(insets);
todayButton.setMaximumSize(buttonSize);
todayButton.setMinimumSize(buttonSize);
todayButton.setPreferredSize(buttonSize);
todayButton.setDefaultCapable(true);
todayButton.setSelected(true);

todayButton.addActionListener(new ActionListener() ...{

public void actionPerformed(final ActionEvent evt) ...{
onToday(evt);
}
});

cancelButton.setText("取消");
cancelButton.setMargin(insets);
cancelButton.setMaximumSize(buttonSize);
cancelButton.setMinimumSize(buttonSize);
cancelButton.setPreferredSize(buttonSize);

cancelButton.addActionListener(new ActionListener() ...{

public void actionPerformed(final ActionEvent evt) ...{
onCancel(evt);
}
});

} else ...{
this.remove(todayButton);
this.remove(cancelButton);
}


if (hideOnSelect) ...{
add(todayButton, new AbsoluteConstraints(25, 190, 52, -1));
add(cancelButton, new AbsoluteConstraints(87, 190, 52, -1));

} else ...{
add(todayButton, new AbsoluteConstraints(55, 190, 52, -1));
}
}


private void onToday(final java.awt.event.ActionEvent evt) ...{
selectedDate = getToday();
setVisible(!hideOnSelect);

if (isVisible()) ...{
monthAndYear.setText(formatDateText(selectedDate.getTime()));
calculateCalendar();
}
}


private void onCancel(final ActionEvent evt) ...{
selectedDate = originalDate;
setVisible(!hideOnSelect);
}


private void onForwardClicked(final java.awt.event.ActionEvent evt) ...{
final int day = selectedDate.get(Calendar.DATE);
selectedDate.set(Calendar.DATE, 1);
selectedDate.add(Calendar.MONTH, 1);
selectedDate.set(Calendar.DATE,
Math.min(day, calculateDaysInMonth(selectedDate)));
monthAndYear.setText(formatDateText(selectedDate.getTime()));
calculateCalendar();
}


private void onBackClicked(final java.awt.event.ActionEvent evt) ...{
final int day = selectedDate.get(Calendar.DATE);
selectedDate.set(Calendar.DATE, 1);
selectedDate.add(Calendar.MONTH, -1);
selectedDate.set(Calendar.DATE,
Math.min(day, calculateDaysInMonth(selectedDate)));
monthAndYear.setText(formatDateText(selectedDate.getTime()));
calculateCalendar();
}


private void onDayClicked(final java.awt.event.MouseEvent evt) ...{
final javax.swing.JLabel fld = (javax.swing.JLabel) evt.getSource();

if (!"".equals(fld.getText())) ...{
fld.setBackground(highlight);
selectedDay = fld;
selectedDate.set(
Calendar.DATE,
Integer.parseInt(fld.getText()));
setVisible(!hideOnSelect);
}
}


private static GregorianCalendar getToday() ...{
final GregorianCalendar gc = new GregorianCalendar();
gc.set(Calendar.HOUR_OF_DAY, 0);
gc.set(Calendar.MINUTE, 0);
gc.set(Calendar.SECOND, 0);
gc.set(Calendar.MILLISECOND, 0);
return gc;
}


private void calculateCalendar() ...{

if (null != selectedDay) ...{
selectedDay.setBackground(white);
selectedDay = null;
}

final GregorianCalendar c = new GregorianCalendar(
selectedDate.get(Calendar.YEAR),
selectedDate.get(Calendar.MONTH),
1);

final int maxDay = calculateDaysInMonth(c);

final int selectedDay = Math.min(maxDay, selectedDate.get(
Calendar.DATE));

int dow = c.get(Calendar.DAY_OF_WEEK);

for (int dd = 0; dd < dow; dd++) ...{
daysInMonth[0][dd].setText("");
}

int week;

do ...{
week = c.get(Calendar.WEEK_OF_MONTH);
dow = c.get(Calendar.DAY_OF_WEEK);
final JLabel fld = this.daysInMonth[week - 1][dow - 1];
fld.setText(Integer.toString(c.get(Calendar.DATE)));

if (selectedDay == c.get(Calendar.DATE)) ...{
fld.setBackground(highlight);
this.selectedDay = fld;
}
if (c.get(Calendar.DATE) >= maxDay)
break;
c.add(Calendar.DATE, 1);
} while (c.get(Calendar.DATE) <= maxDay);

week--;

for (int ww = week; ww < daysInMonth.length; ww++) ...{

for (int dd = dow; dd < daysInMonth[ww].length; dd++) ...{
daysInMonth[ww][dd].setText("");
}
dow = 0;
}

c.set(Calendar.DATE, selectedDay);
selectedDate = c;
}


private static int calculateDaysInMonth(final Calendar c) ...{
int daysInMonth = 0;

switch (c.get(Calendar.MONTH)) ...{
case 0:
case 2:
case 4:
case 6:
case 7:
case 9:
case 11:
daysInMonth = 31;
break;
case 3:
case 5:
case 8:
case 10:
daysInMonth = 30;
break;
case 1:
final int year = c.get(Calendar.YEAR);
daysInMonth =
(0 == year % 1000) ? 29 :
(0 == year % 100) ? 28 :
(0 == year % 4) ? 29 : 28;
break;
}
return daysInMonth;
}


private static String formatDateText(final Date dt) ...{
final DateFormat df = DateFormat.getDateInstance(DateFormat.LONG);

final StringBuffer mm = new StringBuffer();
final StringBuffer yy = new StringBuffer();
final FieldPosition mmfp = new FieldPosition(DateFormat.MONTH_FIELD);
final FieldPosition yyfp = new FieldPosition(DateFormat.YEAR_FIELD);
df.format(dt, mm, mmfp);
df.format(dt, yy, yyfp);
return (mm.toString().substring(mmfp.getBeginIndex(), mmfp.getEndIndex()) +
"月 " + yy.toString().substring(yyfp.getBeginIndex(), yyfp.getEndIndex()) + "年");
}

}
//
DataField.java
package
com.kxsoft.component;

import
java.awt.BorderLayout;
import
java.awt.Color;
import
java.awt.Insets;
import
java.awt.Point;
import
java.awt.event.ActionEvent;
import
java.awt.event.ActionListener;
import
java.awt.event.ComponentAdapter;
import
java.awt.event.ComponentEvent;
import
java.awt.event.MouseAdapter;
import
java.awt.event.MouseEvent;
import
java.awt.event.MouseMotionAdapter;
import
java.net.URL;
import
java.text.DateFormat;
import
java.text.ParseException;
import
java.util.Date;

import
javax.swing.ImageIcon;
import
javax.swing.JButton;
import
javax.swing.JDialog;
import
javax.swing.JFrame;
import
javax.swing.JPanel;
import
javax.swing.JTextField;


public
final
class
DateField
extends
JPanel
...
{

private static final long serialVersionUID = 1L;

private final JTextField dateText = new JTextField(12);

private final JButton dropdownButton = new JButton();

private DatePicker dp;

private JDialog dlg;

Point origin = new Point();


final class Listener extends ComponentAdapter ...{
//日期选择面板消失时调用的方法

public void componentHidden(final ComponentEvent evt) ...{
final Date dt = ((DatePicker) evt.getSource()).getDate();
if (null != dt)
dateText.setText(dateToString(dt));
dlg.dispose();
}

}


public DateField() ...{
super();
init();
}


public DateField(final Date initialDate) ...{
super();
init();
dateText.setText(dateToString(initialDate));
}


public Date getDate() ...{
return stringToDate(dateText.getText());
}


public void setDate(Date date) ...{
String v = dateToString(date);

if (v == null) ...{
v = "";
}
dateText.setText(v);
}


private void init() ...{
setLayout(new BorderLayout());

dateText.setText("");
dateText.setEditable(false);
dateText.setBackground(new Color(255, 255, 255));
add(dateText, BorderLayout.CENTER);

URL url = DateField.class.getResource("/com/kxsoft/component/dropdown.gif");//修改路径,取你本地的图片路径.
ImageIcon icon = new ImageIcon(url);
dropdownButton.setIcon(icon);
dropdownButton.setMargin(new Insets(2, 2, 2, 2));

dropdownButton.addActionListener(new ActionListener() ...{

public void actionPerformed(final ActionEvent evt) ...{
onButtonClick(evt);
}
});
add(dropdownButton, BorderLayout.EAST);
}


private void onButtonClick(final java.awt.event.ActionEvent evt) ...{
if ("".equals(dateText.getText()))
dp = new DatePicker();
else
dp = new DatePicker(stringToDate(dateText.getText()));
dp.addComponentListener(new Listener());

final Point p = dateText.getLocationOnScreen();
p.setLocation(p.getX(), p.getY() - 1 + dateText.getSize().getHeight());

dlg = new JDialog(new JFrame(), true);


dlg.addMouseListener(new MouseAdapter() ...{

public void mousePressed(MouseEvent e) ...{
origin.x = e.getX();
origin.y = e.getY();
}
});

dlg.addMouseMotionListener(new MouseMotionAdapter() ...{

public void mouseDragged(MouseEvent e) ...{
Point p = dlg.getLocation();
dlg.setLocation(p.x + e.getX() - origin.x, p.y + e.getY() - origin.y);
}
});

dlg.setLocation(p);
dlg.setResizable(false);
dlg.setUndecorated(true);
dlg.getContentPane().add(dp);
dlg.pack();
dlg.setVisible(true);
}


private static String dateToString(final Date dt) ...{
if (null != dt)
return DateFormat.getDateInstance(DateFormat.LONG).format(dt);
return null;
}


private static Date stringToDate(final String s) ...{

try ...{
return DateFormat.getDateInstance(DateFormat.LONG).parse(s);

} catch (ParseException e) ...{
return null;
}
}


public static void main(String[] args) ...{
JDialog dlg = new JDialog(new JFrame(), true);
DateField df = new DateField();
dlg.getContentPane().add(df);
dlg.pack();
dlg.setVisible(true);
System.out.println(df.getDate().toString());
System.exit(0);
}
}
package
com.hzlongway.hero.tools;

//
AbsoluteConstraints.java
import
java.awt.Dimension;
import
java.awt.Point;


public
class
AbsoluteConstraints
implements
java.io.Serializable
...
{
static final long serialVersionUID = 5261460716622152494L;

public int x;

public int y;

public int width = -1;

public int height = -1;


public AbsoluteConstraints(Point pos) ...{
this(pos.x, pos.y);
}


public AbsoluteConstraints(int x, int y) ...{
this.x = x;
this.y = y;
}


public AbsoluteConstraints(Point pos, Dimension size) ...{
this.x = pos.x;
this.y = pos.y;

if (size != null) ...{
this.width = size.width;
this.height = size.height;

}
}


public AbsoluteConstraints(int x, int y, int width, int height) ...{
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}


public int getX() ...{
return x;
}


public int getY() ...{
return y;
}


public int getWidth() ...{
return width;
}


public int getHeight() ...{
return height;
}


public String toString() ...{
return super.toString() + " [x=" + x + ", y=" + y + ", width=" + width
+ ", height=" + height + "]";
}
}
package
com.hzlongway.hero.tools;

//
AbsoluteConstraints.java
import
java.awt.Dimension;
import
java.awt.Point;


public
class
AbsoluteConstraints
implements
java.io.Serializable
...
{
static final long serialVersionUID = 5261460716622152494L;

public int x;

public int y;

public int width = -1;

public int height = -1;


public AbsoluteConstraints(Point pos) ...{
this(pos.x, pos.y);
}


public AbsoluteConstraints(int x, int y) ...{
this.x = x;
this.y = y;
}


public AbsoluteConstraints(Point pos, Dimension size) ...{
this.x = pos.x;
this.y = pos.y;

if (size != null) ...{
this.width = size.width;
this.height = size.height;

}
}


public AbsoluteConstraints(int x, int y, int width, int height) ...{
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}


public int getX() ...{
return x;
}


public int getY() ...{
return y;
}


public int getWidth() ...{
return width;
}


public int getHeight() ...{
return height;
}


public String toString() ...{
return super.toString() + " [x=" + x + ", y=" + y + ", width=" + width
+ ", height=" + height + "]";
}
}
原帖地址点击此处.