题目
https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem&category=29&problem=1208&mosmsg=Submission+received+with+ID+17405109
解题思路
- 模拟题,F命令用广搜加入候选pixel时,用hash判断是否已经在队列里或者已经被考虑过,之前用ArrayList的contains方法直接超时好多!
- 用含有丰富数据结构的java写,真是快了很多,但是因为对一些数据理解不够,遇到一些问题
- 注意参数中x,y和数组中的行和列是刚好相反的
- V和H命令注意边界的大小,可能X1>X2
- 用Java写程序,包含main()的public类必须叫Main
通过代码
import java.io.*;
import java.util.*;
class position {
int row;
int col;
position(int row, int col) {
this.row = row;
this.col = col;
}
@Override
public boolean equals(Object o) {
if (o instanceof position) {
position toCompare = (position) o;
return this.row == toCompare.row && this.col == toCompare.col;
}
return false;
}
}
public class Main {
static void clear_table(char[][] table, int M, int N) {
for (int i = 0; i < M; ++i)
for (int j = 0; j < N; ++j)
table[i][j] = 'O';
}
static void clear_tag(boolean[][] table, int M, int N) {
for (int i = 0; i < M; ++i)
for (int j = 0; j < N; ++j)
table[i][j] = false;
}
static boolean inTable(position p, int M, int N) {
if (p.row >= 0 && p.row < M && p.col >= 0 && p.col < N)
return true;
else
return false;
}
public static void main(String[] args) {
try {
Scanner sc = new Scanner(System.in);
char[][] table = null;
int M = 0;
int N = 0;
String command;
boolean stop = false;
int row, col, row1, row2, col1, col2;
char C;
while (!stop && (command = sc.nextLine()) != null) {
String[] arg = command.trim().split("\\s+");
switch (arg[0]) {
case "I":
M = Integer.parseInt(arg[2]);
N = Integer.parseInt(arg[1]);
table = new char[M][N];
clear_table(table, M, N);
break;
case "C":
clear_table(table, M, N);
break;
case "L":
col = Integer.parseInt(arg[1]) - 1;
row = Integer.parseInt(arg[2]) - 1;
C = arg[3].charAt(0);
table[row][col] = C;
break;
case "V":
col = Integer.parseInt(arg[1]) - 1;
row1 = Integer.parseInt(arg[2]) - 1;
row2 = Integer.parseInt(arg[3]) - 1;
C = arg[4].charAt(0);
if (row1 > row2) {
int tmp = row1;
row1 = row2;
row2 = tmp;
}
for (int i = row1; i <= row2; ++i)
table[i][col] = C;
break;
case "H":
col1 = Integer.parseInt(arg[1]) - 1;
col2 = Integer.parseInt(arg[2]) - 1;
row = Integer.parseInt(arg[3]) - 1;
C = arg[4].charAt(0);
if (col1 > col2) {
int tmp = col1;
col1 = col2;
col2 = tmp;
}
for (int j = col1; j <= col2; ++j)
table[row][j] = C;
break;
case "K":
col1 = Integer.parseInt(arg[1]) - 1;
row1 = Integer.parseInt(arg[2]) - 1;
col2 = Integer.parseInt(arg[3]) - 1;
row2 = Integer.parseInt(arg[4]) - 1;
C = arg[5].charAt(0);
for (int i = row1; i <= row2; ++i)
for (int j = col1; j <= col2; ++j)
table[i][j] = C;
break;
case "F":
col = Integer.parseInt(arg[1]) - 1;
row = Integer.parseInt(arg[2]) - 1;
C = arg[3].charAt(0);
char old = table[row][col];
if (C != old) {
ArrayList<position> queue = new ArrayList<position>();
queue.add(new position(row, col));
boolean[][] inqueue = new boolean[M][N];
clear_tag(inqueue, M, N);
boolean[][] foot = new boolean[M][N];
clear_tag(foot, M, N);
while (!queue.isEmpty()) {
position p = queue.get(0);
queue.remove(0);
table[p.row][p.col] = C;
inqueue[p.row][p.col] = false;
foot[p.row][p.col] = true;
position[] candi = new position[4];
candi[0] = new position(p.row, p.col - 1);
candi[1] = new position(p.row, p.col + 1);
candi[2] = new position(p.row - 1, p.col);
candi[3] = new position(p.row + 1, p.col);
for (position can : candi)
if (inTable(can, M, N) && !inqueue[can.row][can.col] && !foot[can.row][can.col]
&& table[can.row][can.col] == old) {
queue.add(can);
inqueue[can.row][can.col] = true;
}
}
}
break;
case "S":
System.out.println(arg[1]);
for (int i = 0; i < M; ++i) {
for (int j = 0; j < N; ++j)
System.out.print(table[i][j]);
System.out.println();
}
break;
case "X":
stop = true;
break;
default:
break;
}
}
} catch (Exception e) {
return;
}
}
}
运行截图
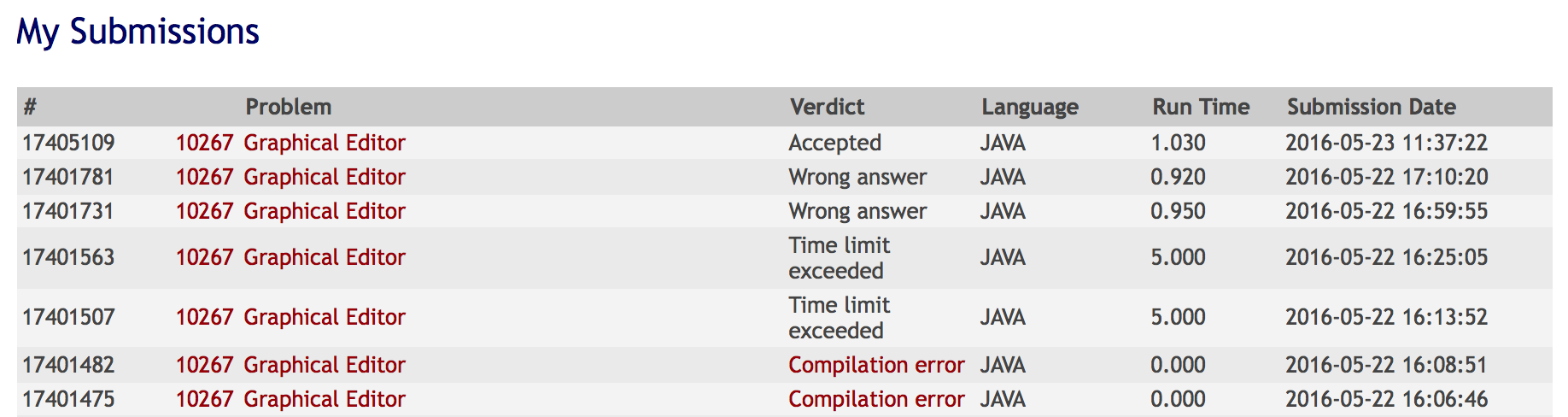
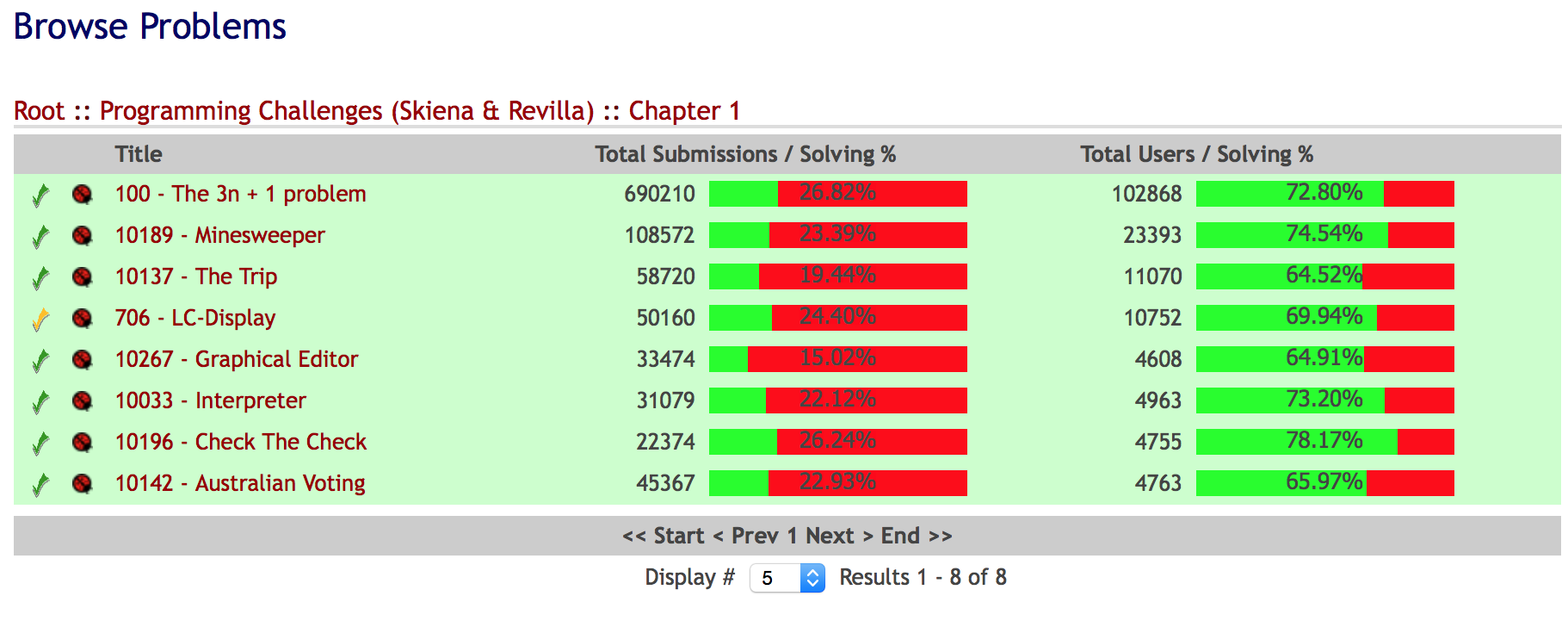