本文让我们一起来学习如何利用Silverlight的HtmlPage.Document对象,实现在Silverlight操作Cookies.
首先创建一个新的Silverlight应用程序,如图:
为使用HtmlPage.Document对象,我们要引入命名空间
1、添加key-value键值对
2、根据key删除
3、根据key读取value
4、读取cookie所有内容
5、判断key-value是否在cookie中存在
代码如下:
using
System;
using
System.Net;
using
System.Windows;
using
System.Windows.Controls;
using
System.Windows.Documents;
using
System.Windows.Ink;
using
System.Windows.Input;
using
System.Windows.Media;
using
System.Windows.Media.Animation;
using
System.Windows.Shapes;
using
System.Windows.Browser;
//
引入此空间以便于使用Cookie操作
namespace
SLCookieOperate
{
public class SLCookieHelp
{
#region Cookie相关操作函数

#region 设置持久时间长的Cookie
/// <summary>
/// 设置持久时间长的Cookie
/// </summary>
/// <param name="key">the cookie key</param>
/// <param name="value">the cookie value</param>
public static void SetCookie(string key, string value)
{

string oldCookie = HtmlPage.Document.GetProperty("cookie") as String;

DateTime expiration = DateTime.UtcNow + TimeSpan.FromDays(2000);

string cookie = String.Format("{0}={1};expires={2}", key, value, expiration.ToString("R"));

HtmlPage.Document.SetProperty("cookie", cookie);

}
#endregion

#region 读取一个已经存在的Cookie
/// <summary>
/// 读取一个已经存在的Cookie
/// </summary>
/// <param name="key">cookie key</param>
/// <returns>null if the cookie does not exist, otherwise the cookie value</returns>
public static string GetCookie(string key)
{
string[] cookies = HtmlPage.Document.Cookies.Split(';');
key += '=';
foreach (string cookie in cookies)
{
string cookieStr = cookie.Trim();
if (cookieStr.StartsWith(key, StringComparison.OrdinalIgnoreCase))
{
string[] vals = cookieStr.Split('=');
if (vals.Length >= 2)
{
return vals[1];
}
return string.Empty;
}
}

return null;
}
#endregion

#region 删除特定的Cookie(清空它的Value值,过期值设置为-1天)
/// <summary>
/// 删除特定的Cookie(清空它的Value值,过期值设置为-1天)
/// </summary>
/// <param name="key">the cookie key to delete</param>

public static void DeleteCookie(string key)
{
string oldCookie = HtmlPage.Document.GetProperty("cookie") as String;
DateTime expiration = DateTime.UtcNow - TimeSpan.FromDays(1);
string cookie = String.Format("{0}=;expires={1}", key, expiration.ToString("R"));
HtmlPage.Document.SetProperty("cookie", cookie);
}
#endregion

#region 判定指定的key-value对是否在cookie中存在
public static bool Exists(String key, String value)
{
return HtmlPage.Document.Cookies.Contains(
String.Format("{0}={1}", key, value)
);
}
#endregion

#region 获取当前cookie内容
public static string getCookieContent()
{
return HtmlPage.Document.GetProperty("cookie") as String;
}
#endregion

#endregion
}
}
创建用户界面如下图:
用户界面xaml代码如下:
Page.xaml.cs的代码如下:
首先创建一个新的Silverlight应用程序,如图:
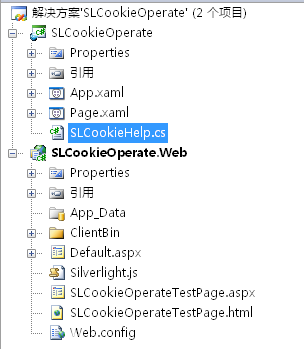
为使用HtmlPage.Document对象,我们要引入命名空间
using
System.Windows.Browser;
//
引入此空间以便于使用Cookie操作
为操作Cookie,我们创建一个类:SLCookieHelp.cs,此类的操作包括:
1、添加key-value键值对
2、根据key删除
3、根据key读取value
4、读取cookie所有内容
5、判断key-value是否在cookie中存在
代码如下:





































































































创建用户界面如下图:
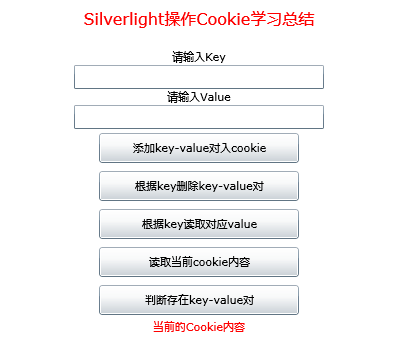
用户界面xaml代码如下:
<
UserControl x:Class
=
"
SLCookieOperate.Page
"
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
Width = " 400 " Height = " 400 " >
< Grid x:Name = " LayoutRoot " Background = " White " >
< StackPanel >
< TextBlock Text = " Silverlight操作Cookie学习总结 " TextAlignment = " Center " Margin = " 8 " FontSize = " 16 " Foreground = " red " ></ TextBlock >
< TextBlock Text = " 请输入Key " TextAlignment = " Center " Margin = " 0,10,0,0 " ></ TextBlock >
< TextBox x:Name = " txtBxKey " Width = " 250 " ></ TextBox >
< TextBlock Text = " 请输入Value " TextAlignment = " Center " ></ TextBlock >
< TextBox x:Name = " txtBxValue " Width = " 250 " ></ TextBox >
< Button Width = " 200 " Height = " 30 " Content = " 添加key-value对入cookie " Margin = " 4 " Click = " Button_Click " ></ Button >
< Button Width = " 200 " Height = " 30 " Content = " 根据key删除key-value对 " Margin = " 4 " Click = " Button_Click_1 " ></ Button >
< Button Width = " 200 " Height = " 30 " Content = " 根据key读取对应value " Margin = " 4 " Click = " Button_Click_2 " ></ Button >
< Button Width = " 200 " Height = " 30 " Content = " 读取当前cookie内容 " Margin = " 4 " Click = " Button_Click_4 " ></ Button >
< Button Width = " 200 " Height = " 30 " Content = " 判断存在key-value对 " Margin = " 4 " Click = " Button_Click_3 " ></ Button >
< TextBlock Text = " 当前的Cookie内容 " TextAlignment = " Center " Foreground = " Red " ></ TextBlock >
< TextBlock x:Name = " tbCookie " TextAlignment = " Center " Foreground = " Green " TextWrapping = " Wrap " Width = " 280 " ></ TextBlock >
</ StackPanel >
</ Grid >
</ UserControl >
xmlns = " http://schemas.microsoft.com/winfx/2006/xaml/presentation "
xmlns:x = " http://schemas.microsoft.com/winfx/2006/xaml "
Width = " 400 " Height = " 400 " >
< Grid x:Name = " LayoutRoot " Background = " White " >
< StackPanel >
< TextBlock Text = " Silverlight操作Cookie学习总结 " TextAlignment = " Center " Margin = " 8 " FontSize = " 16 " Foreground = " red " ></ TextBlock >
< TextBlock Text = " 请输入Key " TextAlignment = " Center " Margin = " 0,10,0,0 " ></ TextBlock >
< TextBox x:Name = " txtBxKey " Width = " 250 " ></ TextBox >
< TextBlock Text = " 请输入Value " TextAlignment = " Center " ></ TextBlock >
< TextBox x:Name = " txtBxValue " Width = " 250 " ></ TextBox >
< Button Width = " 200 " Height = " 30 " Content = " 添加key-value对入cookie " Margin = " 4 " Click = " Button_Click " ></ Button >
< Button Width = " 200 " Height = " 30 " Content = " 根据key删除key-value对 " Margin = " 4 " Click = " Button_Click_1 " ></ Button >
< Button Width = " 200 " Height = " 30 " Content = " 根据key读取对应value " Margin = " 4 " Click = " Button_Click_2 " ></ Button >
< Button Width = " 200 " Height = " 30 " Content = " 读取当前cookie内容 " Margin = " 4 " Click = " Button_Click_4 " ></ Button >
< Button Width = " 200 " Height = " 30 " Content = " 判断存在key-value对 " Margin = " 4 " Click = " Button_Click_3 " ></ Button >
< TextBlock Text = " 当前的Cookie内容 " TextAlignment = " Center " Foreground = " Red " ></ TextBlock >
< TextBlock x:Name = " tbCookie " TextAlignment = " Center " Foreground = " Green " TextWrapping = " Wrap " Width = " 280 " ></ TextBlock >
</ StackPanel >
</ Grid >
</ UserControl >
Page.xaml.cs的代码如下:
using
System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.Threading;
using System.Text;
using System.IO;
using System.Windows.Browser; // 引入此空间以便于使用Cookie操作
namespace SLCookieOperate
{
public partial class Page : UserControl
{
public Page()
{
InitializeComponent();
}
#region 清除文本输入框
private void txtBxClear()
{
this .txtBxKey.Text = "" ;
this .txtBxValue.Text = "" ;
}
#endregion
private void Button_Click( object sender, RoutedEventArgs e)
{
try
{
string keyStr = this .txtBxKey.Text.ToString();
string valueStr = this .txtBxValue.Text.ToString();
if ( ! (String.IsNullOrEmpty(keyStr) || String.IsNullOrEmpty(valueStr)))
{
SLCookieHelp.SetCookie(keyStr, valueStr);
MessageBox.Show( " 添加成功! " );
txtBxClear();
// string kk = HtmlPage.Document.Cookies;
this .tbCookie.Text = SLCookieHelp.getCookieContent();
}
else
{
MessageBox.Show( " 请输入key-value键值对! " );
}
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
}
private void Button_Click_1( object sender, RoutedEventArgs e)
{
string keyStr = this .txtBxKey.Text.ToString();
if ( ! String.IsNullOrEmpty(keyStr))
{
SLCookieHelp.DeleteCookie(keyStr);
MessageBox.Show( " 成功删除! " );
this .tbCookie.Text = SLCookieHelp.getCookieContent();
}
else
{
MessageBox.Show( " 请输入要删除的Key " );
}
}
private void Button_Click_2( object sender, RoutedEventArgs e)
{
string keyStr = this .txtBxKey.Text.ToString();
if ( ! String.IsNullOrEmpty(keyStr))
{
string valueStr = SLCookieHelp.GetCookie(keyStr);
MessageBox.Show( string .Format( " 对应你输入的Key的Value是: {0} " ,valueStr));
}
else
{
MessageBox.Show( " 请输入要查找的Key " );
}
}
private void Button_Click_3( object sender, RoutedEventArgs e)
{
string keyStr = this .txtBxKey.Text.ToString();
string valueStr = this .txtBxValue.Text.ToString();
if ( ! (String.IsNullOrEmpty(keyStr) || String.IsNullOrEmpty(valueStr)))
{
if (SLCookieHelp.Exists(keyStr, valueStr))
{
MessageBox.Show( " 此key-value对存在于cookie中 " );
}
else
{
MessageBox.Show( " 没有找到此 key-value " );
}
}
else
{
MessageBox.Show( " 请输入要判定的Key与Value对 " );
}
}
private void Button_Click_4( object sender, RoutedEventArgs e)
{
this .tbCookie.Text = SLCookieHelp.getCookieContent();
}
}
}
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.Threading;
using System.Text;
using System.IO;
using System.Windows.Browser; // 引入此空间以便于使用Cookie操作
namespace SLCookieOperate
{
public partial class Page : UserControl
{
public Page()
{
InitializeComponent();
}
#region 清除文本输入框
private void txtBxClear()
{
this .txtBxKey.Text = "" ;
this .txtBxValue.Text = "" ;
}
#endregion
private void Button_Click( object sender, RoutedEventArgs e)
{
try
{
string keyStr = this .txtBxKey.Text.ToString();
string valueStr = this .txtBxValue.Text.ToString();
if ( ! (String.IsNullOrEmpty(keyStr) || String.IsNullOrEmpty(valueStr)))
{
SLCookieHelp.SetCookie(keyStr, valueStr);
MessageBox.Show( " 添加成功! " );
txtBxClear();
// string kk = HtmlPage.Document.Cookies;
this .tbCookie.Text = SLCookieHelp.getCookieContent();
}
else
{
MessageBox.Show( " 请输入key-value键值对! " );
}
}
catch (Exception ex)
{
MessageBox.Show(ex.ToString());
}
}
private void Button_Click_1( object sender, RoutedEventArgs e)
{
string keyStr = this .txtBxKey.Text.ToString();
if ( ! String.IsNullOrEmpty(keyStr))
{
SLCookieHelp.DeleteCookie(keyStr);
MessageBox.Show( " 成功删除! " );
this .tbCookie.Text = SLCookieHelp.getCookieContent();
}
else
{
MessageBox.Show( " 请输入要删除的Key " );
}
}
private void Button_Click_2( object sender, RoutedEventArgs e)
{
string keyStr = this .txtBxKey.Text.ToString();
if ( ! String.IsNullOrEmpty(keyStr))
{
string valueStr = SLCookieHelp.GetCookie(keyStr);
MessageBox.Show( string .Format( " 对应你输入的Key的Value是: {0} " ,valueStr));
}
else
{
MessageBox.Show( " 请输入要查找的Key " );
}
}
private void Button_Click_3( object sender, RoutedEventArgs e)
{
string keyStr = this .txtBxKey.Text.ToString();
string valueStr = this .txtBxValue.Text.ToString();
if ( ! (String.IsNullOrEmpty(keyStr) || String.IsNullOrEmpty(valueStr)))
{
if (SLCookieHelp.Exists(keyStr, valueStr))
{
MessageBox.Show( " 此key-value对存在于cookie中 " );
}
else
{
MessageBox.Show( " 没有找到此 key-value " );
}
}
else
{
MessageBox.Show( " 请输入要判定的Key与Value对 " );
}
}
private void Button_Click_4( object sender, RoutedEventArgs e)
{
this .tbCookie.Text = SLCookieHelp.getCookieContent();
}
}
}
运行效果如下:
原文地址:http://www.cnblogs.com/wsdj-ITtech/archive/2009/08/15/1546815.html