react native流程组件展示
一、使用
let approval_process = [
{
status: "已提交",
name: "巴啦啦小魔仙",
dept: "施工组织",
person: "拟稿",
time: "07-19 10:21",
remark: "",
},
{
status: "已通过",
name: "我自己",
dept: "华夏",
person: "拟稿",
time: "07-19 10:21",
remark: "",
},
{
status: "未通过",
name: "猫之使徒",
dept: "宇宙中心五道口职业",
person: "拟稿",
time: "07-19 10:21",
remark: "按照规定进行修改",
},
{
status: "待审核",
name: "陈大伟",
dept: "中哈u认为呢",
person: "审批",
time: "07-19 10:21",
remark: "同意",
},
];
<ApprovalProcess
data={approval_process}
status={"已完成"}
/>
二、效果
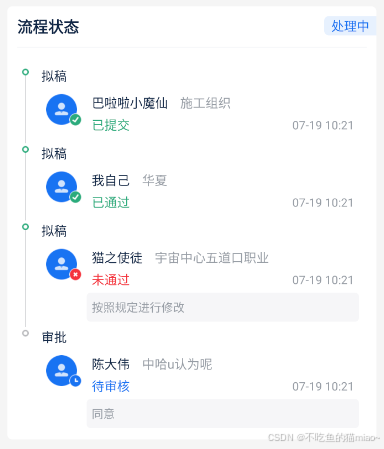
三、代码
import React, { Component } from "react";
import {
Text,
View,
StyleSheet,
ListView,
Dimensions,
Image,
} from "react-native";
import PropTypes from "prop-types";
const height = Dimensions.get("window").height;
const width = Dimensions.get("window").width;
class ApprovalProcess extends Component {
constructor(props) {
super(props);
this.dataSource = new ListView.DataSource({
rowHasChanged: (row1, row2) => row1 !== row2,
});
this.state = {
data: this.props.data,
status: this.props.status,
};
}
static propTypes = {
title: PropTypes.string,
};
static defaultProps = {
title: "流程状态",
};
renderCustomList(item, sectionID, rowID) {
var img = require("../../../resources/technicalManagement/success.png");
if (item.status === "待审核") {
img = require("../../../resources/technicalManagement/waiting.png");
} else if (item.status === "未通过") {
img = require("../../../resources/technicalManagement/fail.png");
}
var circle = require("../../../resources/technicalManagement/circle.png");
if (parseInt(rowID) < this.props.data.length - 1) {
circle = require("../../../resources/technicalManagement/circleGreen.png");
}
return (
<View style={styles.warp}>
<View
style={[styles.leftWarp, { height: item.remark === "" ? 70 : 100 }]}
>
<Image
source={circle}
resizeMode="stretch"
style={{ width: 7, height: 7 }}
/>
{parseInt(rowID) < this.props.data.length - 1 ? (
<View
style={[
styles.lineLeft,
{ height: item.remark === "" ? 70 : 100 },
]}
/>
) : (
<View style={[{ height: item.remark === "" ? 70 : 100 }]} />
)}
</View>
<View
style={[styles.rightWarp, { height: item.remark === "" ? 70 : 100 }]}
>
<Text style={styles.shen}>{item.person}</Text>
<View style={styles.centerLine}>
<Image
source={require("../../../resources/technicalManagement/avaort.png")}
resizeMode="stretch"
style={{ width: 32, height: 32 }}
/>
<Image source={img} resizeMode="stretch" style={styles.position} />
<View style={{ marginLeft: 10 }}>
<Text style={styles.shen}>
{item.name}
<Text style={styles.dept}>{" " + item.dept}</Text>
</Text>
<View style={styles.rows}>
{item.status == "待审核" ? (
<Text style={[styles.status, { color: "#1873F2" }]}>
{item.status}
</Text>
) : item.status == "未通过" ? (
<Text style={[styles.status, { color: "#F4333C" }]}>
{item.status}
</Text>
) : (
<Text style={[styles.status, { color: "#2BAB7C" }]}>
{item.status}
</Text>
)}
<Text style={styles.time}>{item.time}</Text>
</View>
{item.remark == "" ? null : (
<View style={styles.remarkWarp}>
<Text style={styles.remark}>{item.remark}</Text>
</View>
)}
</View>
</View>
</View>
</View>
);
}
render() {
let statusArr = [
{
key: "YWC",
value: "已完成",
bgColor: "rgba(45, 190, 137, 0.1)",
color: "#2DBE89",
},
{
key: "CLZ",
value: "处理中",
bgColor: "rgba(24, 115, 242, 0.1)",
color: "#1873F2",
},
{
key: "YTH",
value: "已退回",
bgColor: "rgba(244, 51, 60, 0.1)",
color: "#F4333C",
},
];
return (
<View style={styles.processWarp}>
<Text style={styles.valueCenter}>{this.props.title}</Text>
<View
style={[styles.statusWarp, { backgroundColor: statusArr[1].bgColor }]}
>
<Text style={[styles.status, { color: statusArr[1].color }]}>
{statusArr[1].value}
</Text>
</View>
<View style={styles.line} />
<View style={styles.row}>
<ListView
dataSource={this.dataSource.cloneWithRows(this.state.data)}
renderRow={this.renderCustomList.bind(this)}
initialListSize={this.props.initialListSize}
enableEmptySections
removeClippedSubviews={false}
/>
</View>
</View>
);
}
}
export default ApprovalProcess;
const styles = StyleSheet.create({
processWarp: {
width: width - 30,
marginHorizontal: 15,
marginBottom: 10,
minHeight: 90,
backgroundColor: "#fff",
padding: 10,
borderRadius: 5,
},
valueCenter: {
color: "#0F2646",
fontSize: 16,
fontWeight: "500",
},
row: {
flexDirection: "row",
alignItems: "center",
justifyContent: "space-between",
marginTop: 5,
},
line: {
height: 1,
backgroundColor: "#F0F2F5",
marginVertical: 10,
width: width - 50,
},
warp: {
width: width - 30,
padding: 5,
flexDirection: "row",
alignItems: "center",
},
leftWarp: {
width: 15,
justifyContent: "center",
paddingTop: 10,
},
rightWarp: {
width: width - 50,
},
lineLeft: {
width: 1,
backgroundColor: "#D3D5D7",
marginLeft: 3,
},
shen: {
color: "#0F2646",
fontSize: 13,
fontWeight: "400",
marginLeft: 5,
},
dept: {
color: "#979DA5",
fontSize: 13,
paddingLeft: 10,
},
centerLine: {
flexDirection: "row",
margin: 10,
},
rows: {
flexDirection: "row",
alignItems: "center",
justifyContent: "space-between",
margin: 5,
width: width - 140,
},
status: {
fontSize: 12,
fontWeight: "400",
},
time: {
color: "#979DA5",
fontSize: 12,
},
remarkWarp: {
width: width - 130,
minHeight: 30,
backgroundColor: "#F6F6F8",
borderRadius: 4,
justifyContent: "center",
paddingHorizontal: 5,
},
remark: {
color: "#979DA5",
fontSize: 12,
},
position: {
width: 13,
height: 13,
position: "absolute",
top: 20,
left: 24,
},
statusWarp: {
width: 54,
height: 20,
justifyContent: "center",
alignItems: "center",
borderTopLeftRadius: 5,
borderBottomLeftRadius: 5,
position:'absolute',
right:0,
top:10,
},
status: {
fontSize: 13,
},
});