串行队列
- (IBAction)SerialQueueSync:(id)sender {
dispatch_queue_t queue = dispatch_queue_create("FirstSerialQueue", DISPATCH_QUEUE_SERIAL);
dispatch_sync(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"++++++++++%@", [NSThread currentThread]);
}
});
NSLog(@"第一个打印完毕");
dispatch_sync(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"----------%@", [NSThread currentThread]);
}
});
NSLog(@"第二个打印完毕");
}
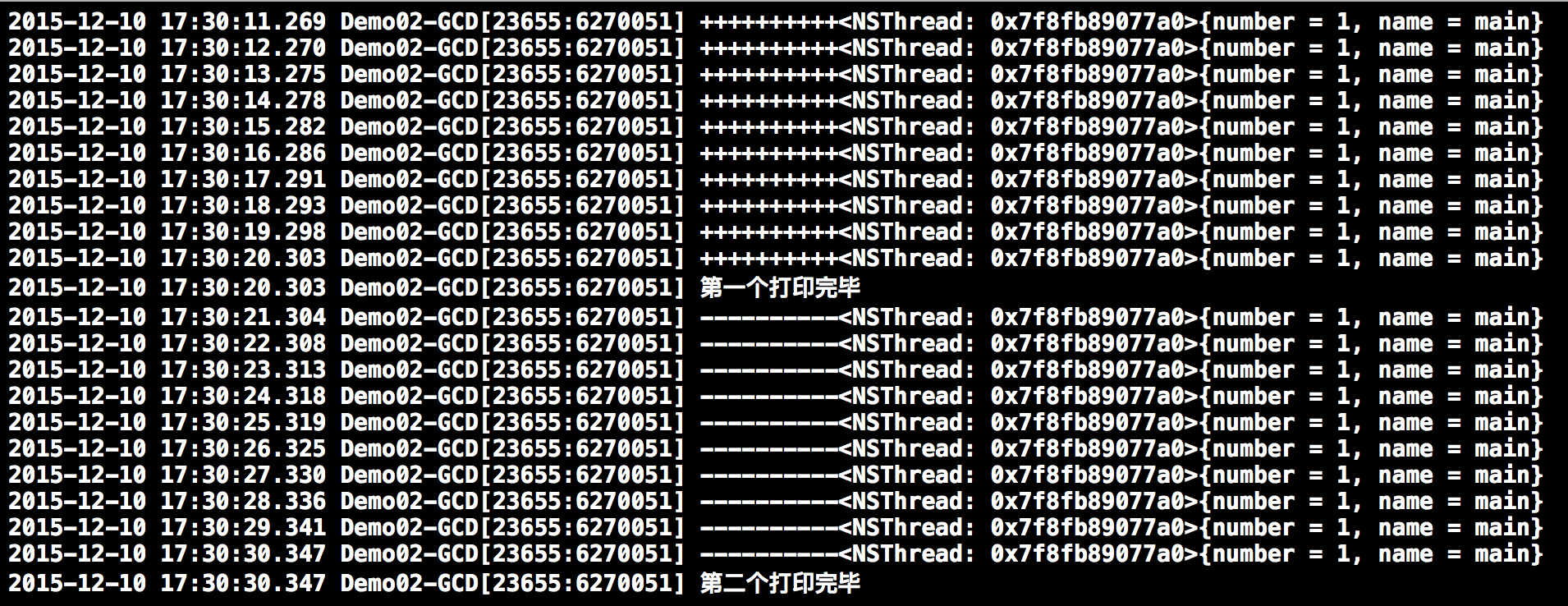
- (IBAction)SerialQueueAsync:(id)sender {
dispatch_queue_t queue = dispatch_queue_create("SecondSerialQueue", DISPATCH_QUEUE_SERIAL);
dispatch_async(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"++++++++++%@", [NSThread currentThread]);
}
});
NSLog(@"第一个打印完毕");
dispatch_async(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"----------%@", [NSThread currentThread]);
}
});
NSLog(@"第二个打印完毕");
}
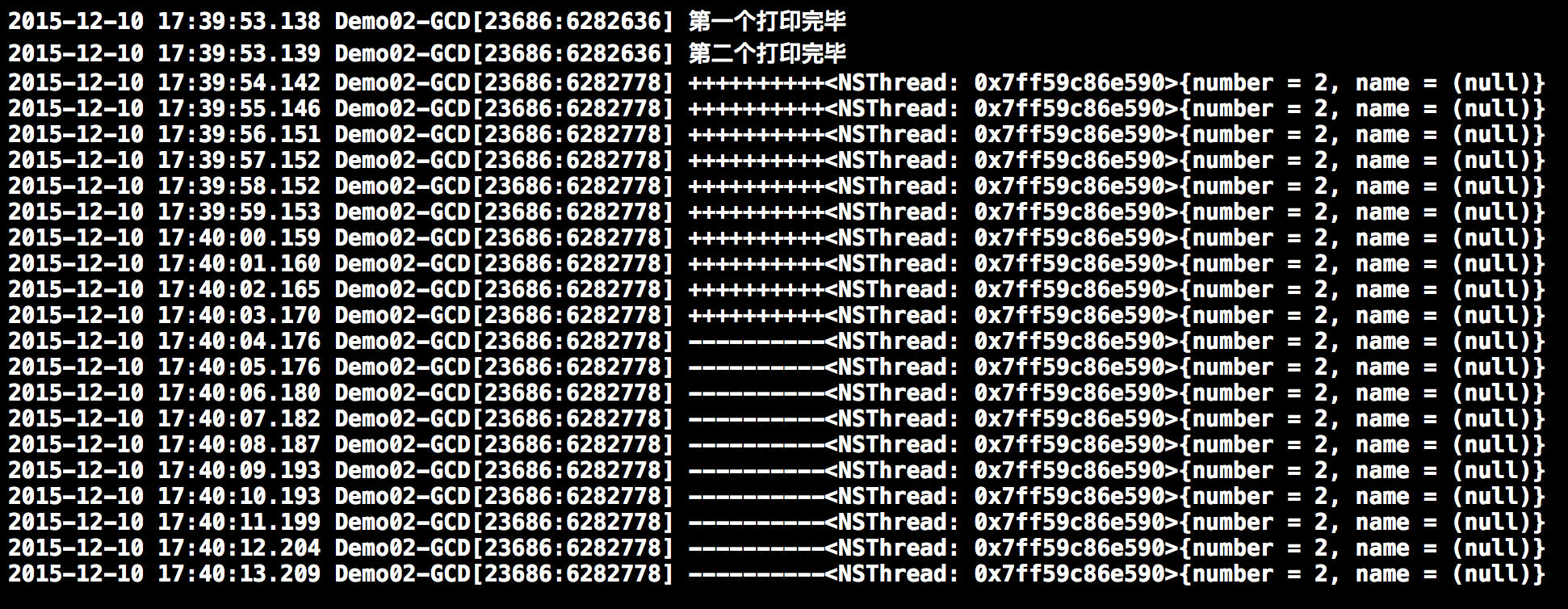
并行队列
- (IBAction)concurrentQueueSync:(id)sender {
dispatch_queue_t queue = dispatch_queue_create("FirstConcurrentQueue", DISPATCH_QUEUE_CONCURRENT);
dispatch_sync(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"++++++++++%@", [NSThread currentThread]);
}
});
NSLog(@"第一个打印完毕");
dispatch_sync(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"----------%@", [NSThread currentThread]);
}
});
NSLog(@"第二个打印完毕");
}
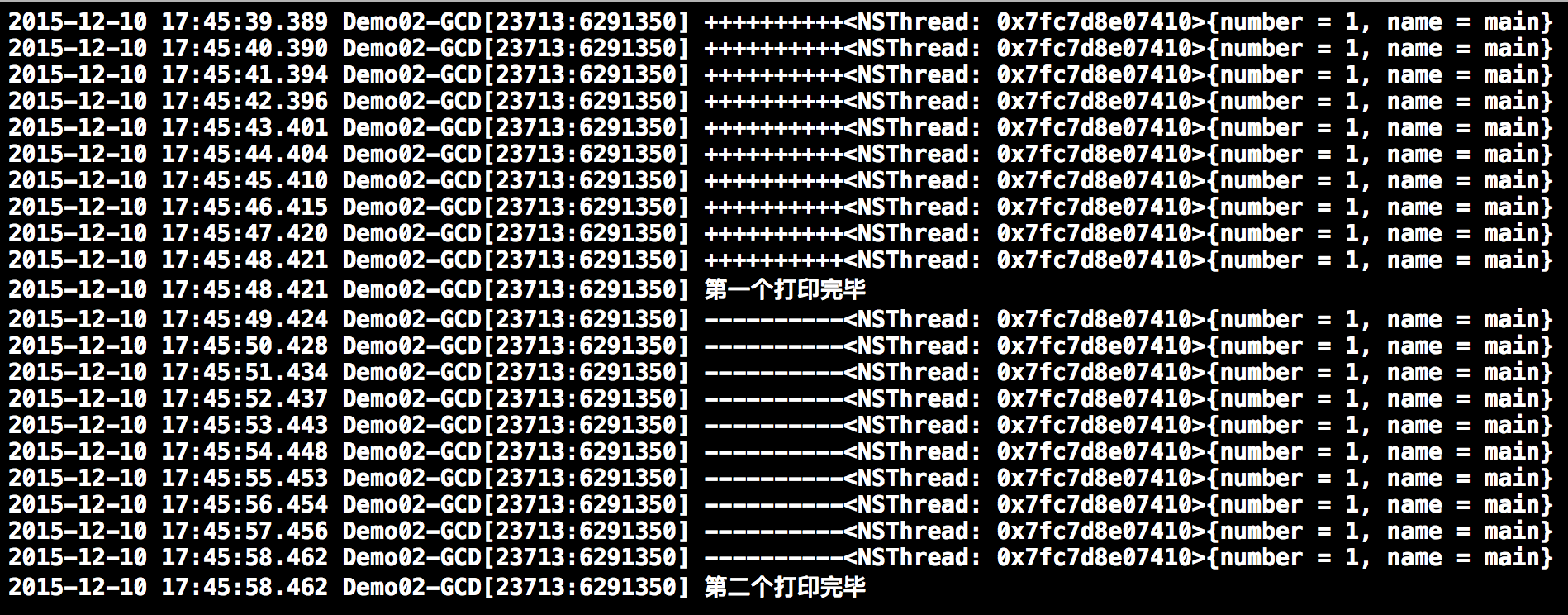
- (IBAction)concurrentQueuAsync:(id)sender {
dispatch_queue_t queue = dispatch_queue_create("SecondConcurrentQueue", DISPATCH_QUEUE_CONCURRENT);
dispatch_async(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"++++++++++%@", [NSThread currentThread]);
}
});
NSLog(@"第一个打印完毕");
dispatch_async(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"----------%@", [NSThread currentThread]);
}
});
NSLog(@"第二个打印完毕");
}
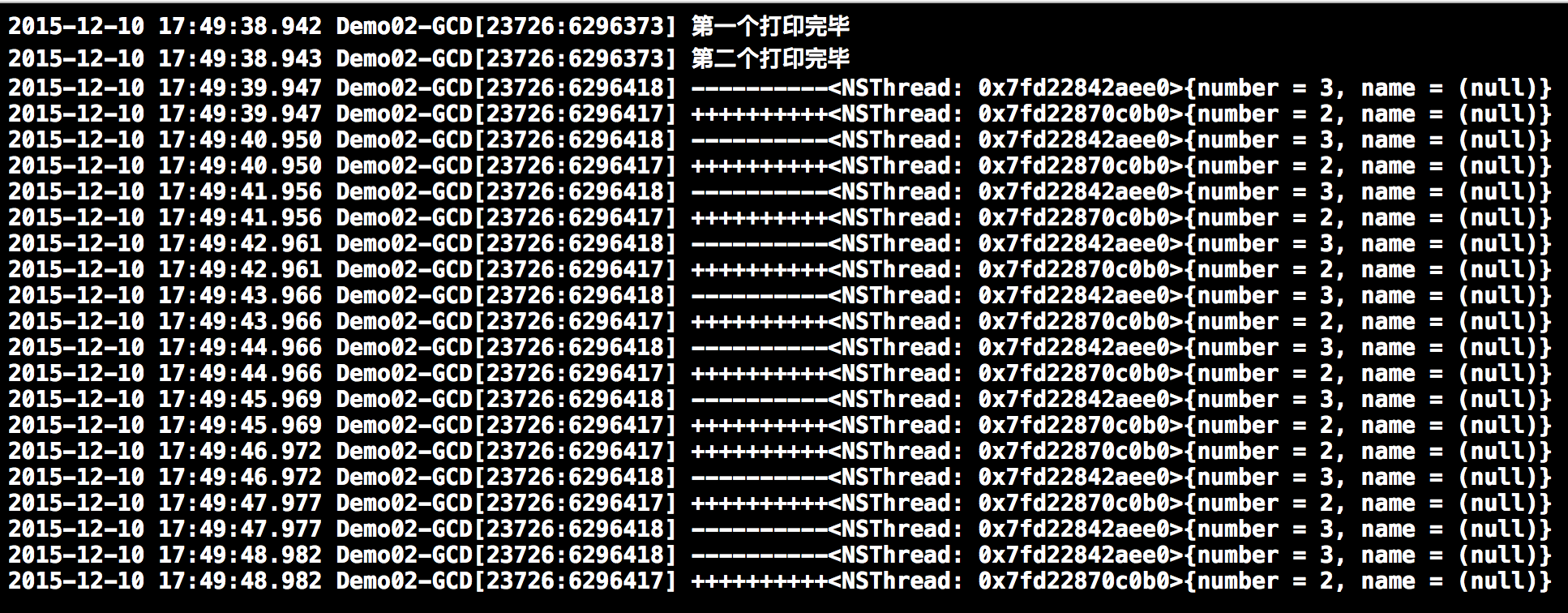
全局队列
- (IBAction)globalQueueAsync:(id)sender {
dispatch_queue_t queue = dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0);
dispatch_async(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"++++++++++++:%@",[NSThread currentThread]);
}
});
NSLog(@"第一个打印完毕");
dispatch_async(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"----------%@", [NSThread currentThread]);
}
});
NSLog(@"第二个打印完毕");
}
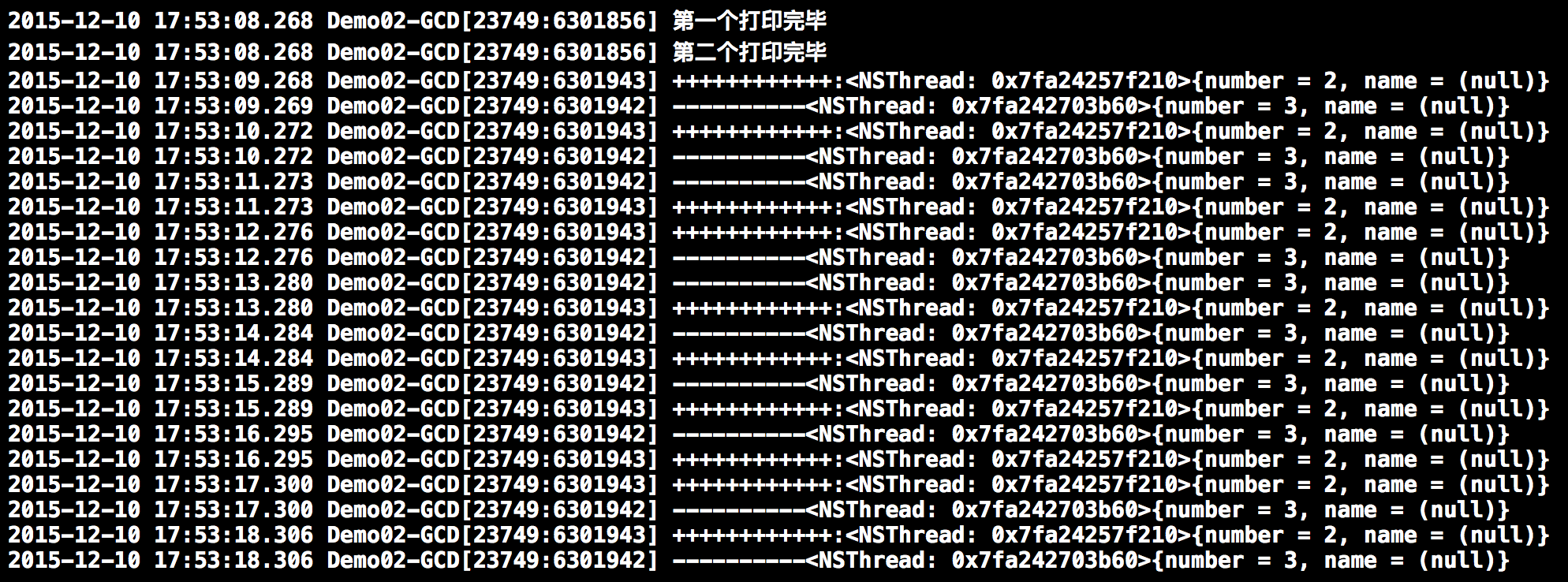
主队列
- (IBAction)mainQueueAsync:(id)sender {
dispatch_queue_t queue = dispatch_get_main_queue();
dispatch_async(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"++++++++++%@", [NSThread currentThread]);
}
});
NSLog(@"第一个打印完毕");
dispatch_async(queue, ^{
for (int i = 0; i < 10; i++) {
[NSThread sleepForTimeInterval:1];
NSLog(@"----------%@", [NSThread currentThread]);
}
});
NSLog(@"第二个打印完毕");
}
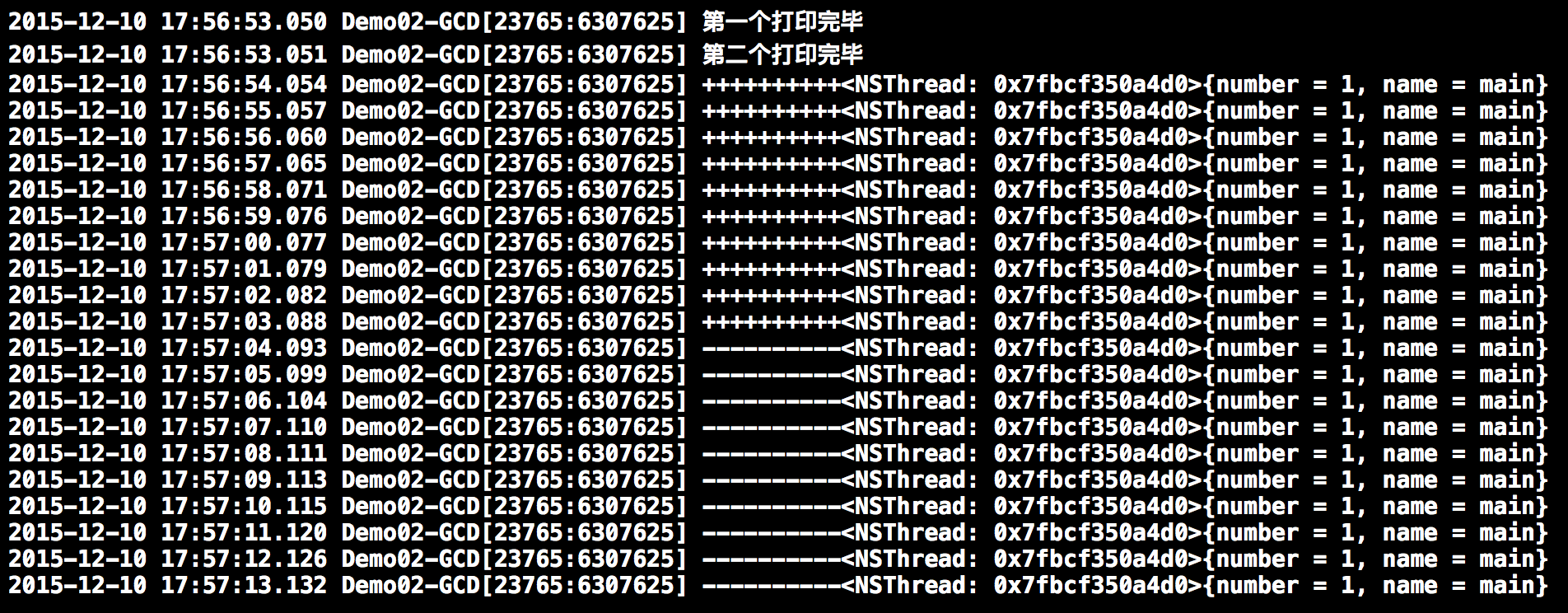
- (IBAction)mainQueueSync:(id)sender {
NSLog(@"任务一");
dispatch_sync(dispatch_get_main_queue(), ^{
NSLog(@"任务二");
});
NSLog(@"任务三");
}
