1、tf.where
如果x和y都为空,则此操作返回条件的真元素的坐标。例如,有一个3*3的矩阵,[[True, False, False], [False, True, False], [False, False, True]],返回一个3*2的矩阵,其中的3代表True的个数,2代表矩阵为True的坐标,返回结果为[[0, 0], [1, 1], [2, 2]]
如果x和y不为空,x、y必须有相同的形状,如果x、y是标量,那么condition参数也必须是标量。如果x、y是向量,那么condition必须和x的第一维有相同的形状或者和x形状一致。返回值和x、y有相同的形状,如果condition对应位置值为True那么返回Tensor对应位置为x的值,如果condition对应位置值为False那么返回Tensor对应位置为y的值.
用法:
tf.where
(
condition,
x=None,
y=None,
name=None
)
示例:
## x和y为空
import tensorflow as tf
a = tf.random.normal([3, 3])
mask = a> 0
mask
Out[6]:
<tf.Tensor: shape=(3, 3), dtype=bool, numpy=
array([[ True, True, False],
[ True, False, True],
[False, True, True]])>
tf.boolean_mask(a, mask)
Out[7]:
<tf.Tensor: shape=(6,), dtype=float32, numpy=
array([0.07397593, 0.6274408 , 0.09557381, 1.5277305 , 1.8625971 ,
0.14077592], dtype=float32)>
indices = tf.where(mask)
indices
Out[9]:
<tf.Tensor: shape=(6, 2), dtype=int64, numpy=
array([[0, 0],
[0, 1],
[1, 0],
[1, 2],
[2, 1],
[2, 2]], dtype=int64)>
tf.gather_nd(a, indices)
Out[10]:
<tf.Tensor: shape=(6,), dtype=float32, numpy=
array([0.07397593, 0.6274408 , 0.09557381, 1.5277305 , 1.8625971 ,
0.14077592], dtype=float32)>
# x和y的值不为空
mask
Out[11]:
<tf.Tensor: shape=(3, 3), dtype=bool, numpy=
array([[ True, True, False],
[ True, False, True],
[False, True, True]])>
A = tf.ones([3, 3])*2
B = tf.ones([3, 3])*7
tf.where(mask, A, B)
Out[17]:
<tf.Tensor: shape=(3, 3), dtype=float32, numpy=
array([[2., 2., 7.],
[2., 7., 2.],
[7., 2., 2.]], dtype=float32)>
2、tf.scatter_nd
根据indices将updates散布到新的(初始为零)张量.
根据索引对给定shape的零张量中的单个值或切片应用稀疏updates来创建新的张量.此运算符是tf.gather_nd运算符的反函数,它从给定的张量中提取值或切片.
警告:更新应用的顺序是非确定性的,所以如果indices包含重复项的话,则输出将是不确定的.
indices是一个整数张量,其中含有索引形成一个新的形状shape张量.indices的最后的维度可以是shape的最多的秩:
indices.shape[-1] <= shape.rank
indices的最后一个维度对应于沿着shape的indices.shape[-1]维度的元素的索引(if indices.shape[-1] = shape.rank)或切片(if indices.shape[-1] < shape.rank)的索引.updates是一个具有如下形状的张量:
indices.shape[:-1] + shape[indices.shape[-1]:]
用法:
tf.scatter_nd
(
indices,
updates,
shape,
name=None
)
参数说明
- indices:一个Tensor;必须是以下类型之一:int32,int64;指数张量.
- updates:一个Tensor;分散到输出的更新.
- shape:一个Tensor;必须与indices具有相同的类型;1-d;得到的张量的形状.
- name:操作的名称(可选).
最简单的分散形式是通过索引将单个元素插入到张量中.例如,假设我们想要在8个元素的1级张量中插入4个分散的元素.

示例1:
indices = tf.constant([[4], [3], [1], [7]])
updates = tf.constant([9, 10, 11, 12])
shape = tf.constant([8])
tf.scatter_nd(indices, updates, shape)
Out[21]: <tf.Tensor: shape=(8,), dtype=int32, numpy=array([ 0, 11, 0, 10, 9, 0, 0, 12])>
我们也可以一次插入一个更高阶张量的整个片.例如,如果我们想要在具有两个新值的矩阵的第三维张量中插入两个切片.
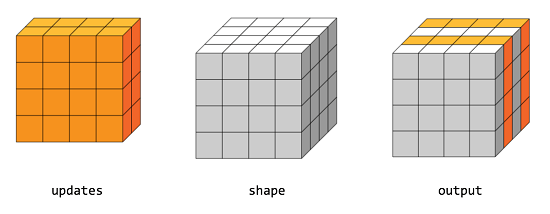
示例2:
indices = tf.constant([[0], [2]])
updates = tf.constant([[[5, 5, 5, 5], [6, 6, 6, 6],
[7, 7, 7, 7], [8, 8, 8, 8]],
[[5, 5, 5, 5], [6, 6, 6, 6],
[7, 7, 7, 7], [8, 8, 8, 8]]])
shape = tf.constant([4, 4, 4])
scatter = tf.scatter_nd(indices, updates, shape)
print(scatter)
tf.Tensor(
[[[5 5 5 5]
[6 6 6 6]
[7 7 7 7]
[8 8 8 8]]
[[0 0 0 0]
[0 0 0 0]
[0 0 0 0]
[0 0 0 0]]
[[5 5 5 5]
[6 6 6 6]
[7 7 7 7]
[8 8 8 8]]
[[0 0 0 0]
[0 0 0 0]
[0 0 0 0]
[0 0 0 0]]], shape=(4, 4, 4), dtype=int32)
3、tf.meshgrid
meshgrid用于从数组a和b产生网格。生成的网格矩阵A和B大小是相同的。它也可以是更高维的。用法: [A,B]=Meshgrid(a,b),生成size(b)*size(a)大小的矩阵A和B。
它相当于a从一行重复增加到size(b)行,把b转置成一列再重复增加到size(a)列
官方用法:
tf.meshgrid
(
*args,
**kwargs
)
示例:
y = tf.linspace(-2., 2, 5)
y
Out[29]: <tf.Tensor: shape=(5,), dtype=float32, numpy=array([-2., -1., 0., 1., 2.], dtype=float32)>
x = tf.linspace(-3., 1, 5)
points_x, points_y = tf.meshgrid(x, y)
points_x, points_y
Out[37]:
(<tf.Tensor: shape=(5, 5), dtype=float32, numpy=
array([[-3., -2., -1., 0., 1.],
[-3., -2., -1., 0., 1.],
[-3., -2., -1., 0., 1.],
[-3., -2., -1., 0., 1.],
[-3., -2., -1., 0., 1.]], dtype=float32)>,
<tf.Tensor: shape=(5, 5), dtype=float32, numpy=
array([[-2., -2., -2., -2., -2.],
[-1., -1., -1., -1., -1.],
[ 0., 0., 0., 0., 0.],
[ 1., 1., 1., 1., 1.],
[ 2., 2., 2., 2., 2.]], dtype=float32)>)
## 变成点的形式
points = tf.stack([points_x, points_y], axis= 2)
points
Out[39]:
<tf.Tensor: shape=(5, 5, 2), dtype=float32, numpy=
array([[[-3., -2.],
[-2., -2.],
[-1., -2.],
[ 0., -2.],
[ 1., -2.]],
[[-3., -1.],
[-2., -1.],
[-1., -1.],
[ 0., -1.],
[ 1., -1.]],
[[-3., 0.],
[-2., 0.],
[-1., 0.],
[ 0., 0.],
[ 1., 0.]],
[[-3., 1.],
[-2., 1.],
[-1., 1.],
[ 0., 1.],
[ 1., 1.]],
[[-3., 2.],
[-2., 2.],
[-1., 2.],
[ 0., 2.],
[ 1., 2.]]], dtype=float32)>