例1:同时设置多个属性动画
import QtQuick 2.9
import QtQuick.Window 2.2
Window
{
id:win
visible: true
width: img_bg.width
height: img_bg.height
Image
{
id:img_bg;
source: "qrc:/img/background.png"//背景
Image
{
x: 40; y: 80
source: "qrc:/img/rocket.png"//火箭
NumberAnimation on x //数字动画-位置x值:40~240
{
easing.type: Easing.InSine
to: 240
duration: 4000
loops: Animation.Infinite
//动画运行次数 默认情况下,循环为1:动画将播放一次,然后停止。
//如果设置为Animation.Infinite,动画将持续重复,直到显式停止为止—可以将running属性设置为false,也可以调用stop()方法。
}
RotationAnimation on rotation //旋转动画-角度0~360
{
to: 360
duration: 4000
loops: Animation.Infinite
}
PropertyAnimation on opacity //属性动画-透明度1~0.2
{
to: 0.2
duration: 4000
loops: Animation.Infinite
}
PropertyAnimation on scale//属性动画-缩放比1~1.8
{
to: 1.8
duration: 4000
loops: Animation.Infinite
}
}
}
}
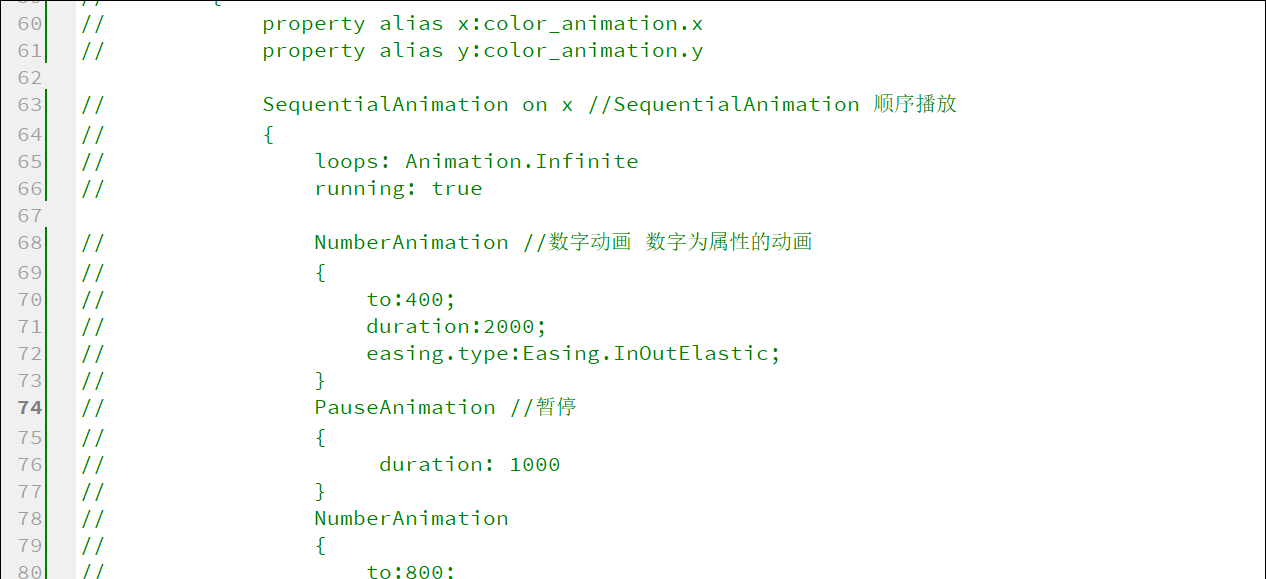
例2:动画分组示例
import QtQuick 2.9
import QtQuick.Window 2.2
Window
{
id:win
visible: true
width: img_bg.width
height: img_bg.height
Image
{
id:img_bg;
source: "qrc:/img/background.png"//背景
}
Rectangle
{
id:color_animation
width: 100
height: 100
x:150
y:20
color: "red"
ParallelAnimation //并行动画 这里x和y同时变化
{
property alias x:color_animation.x
property alias y:color_animation.y
SequentialAnimation on x //串行动画
{
loops: Animation.Infinite
running: true
NumberAnimation //数字动画 数字为属性的动画
{
to:400;
duration:2000;
easing.type:Easing.InOutElastic;
}
PauseAnimation //暂停
{
duration: 1000
}
NumberAnimation
{
to:800;
duration:2000;
easing.type:Easing.InOutElastic;
}
}
SequentialAnimation on y
{
loops: Animation.Infinite
running: true
NumberAnimation
{
to:100;
duration:2000;
easing.type:Easing.InOutElastic;
}
PauseAnimation //暂停
{
duration: 1000
}
NumberAnimation
{
to:200;
duration:2000;
easing.type:Easing.InOutElastic;
}
}
}
SequentialAnimation on color
{
// property alias color:color_animation.color
loops: Animation.Infinite
running: true
ColorAnimation
{
to:"blue";
duration: 2000
}
PauseAnimation
{
duration: 1000
}
ColorAnimation
{
to:"yellow";
duration: 2000
}
}
}
}
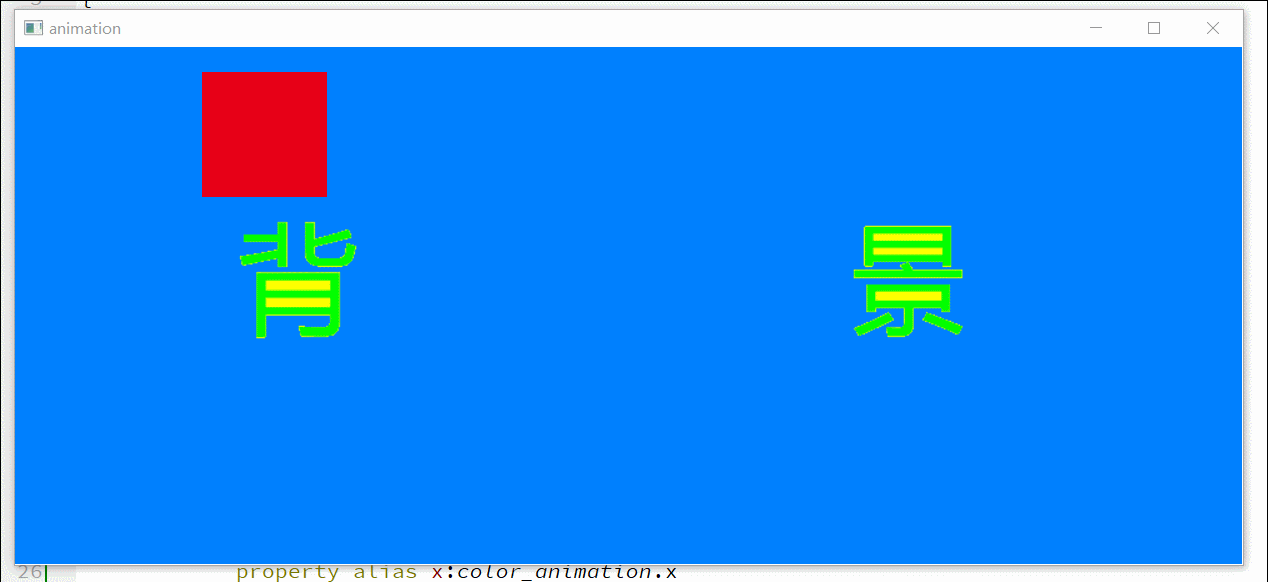
例3:锚定动画示例
import QtQuick 2.9
import QtQuick.Window 2.2
Window
{
id:win
visible: true
width: img_bg.width
height: img_bg.height
Image
{
id:img_bg;
source: "qrc:/img/background.png"//背景
}
Rectangle
{
id: container
width: 200
height: 200
x:0
y:100
Rectangle
{
id: myRect
width: 100
height: 100
color: "red"
anchors.left: parent.left
anchors.bottom: parent.bottom
}
states: State
{
name: "reanchored"
AnchorChanges //锚改变
{
target: myRect;
anchors.right: container.right
anchors.top: container.top
anchors.left: container.verticalCenter
anchors.bottom: container.verticalCenter
}
}
transitions: Transition
{
AnchorAnimation //锚改变的的动画
{
duration: 1000
}
}
Component.onCompleted: container.state = "reanchored"
}
}
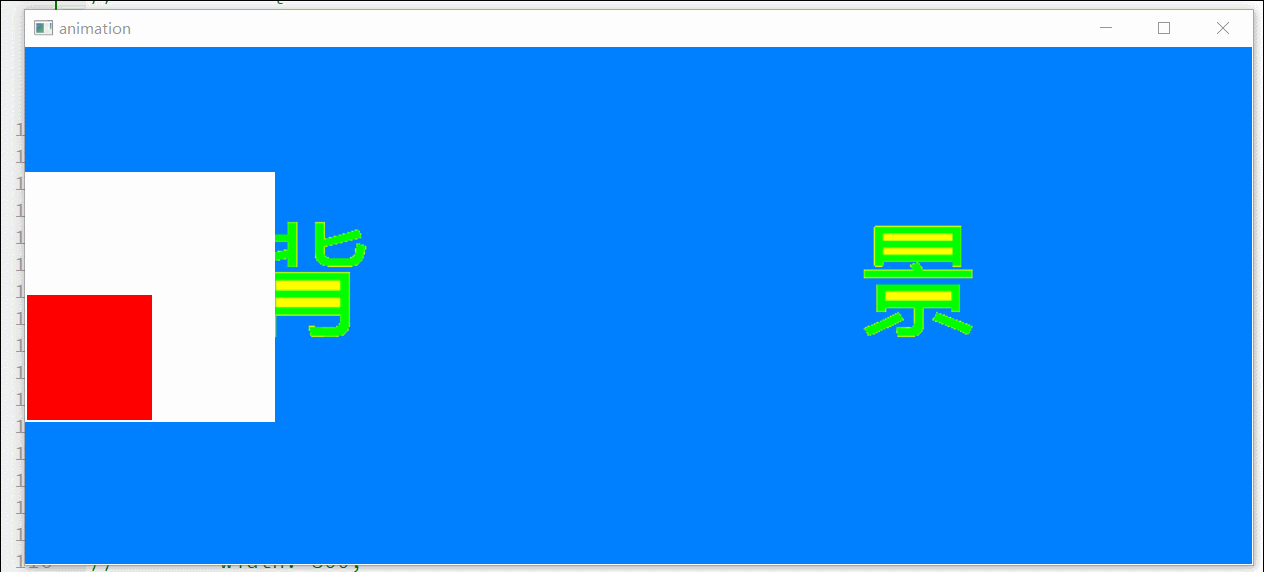
例4:父元素动画
import QtQuick 2.9
import QtQuick.Window 2.2
Window
{
id:win
visible: true
width: img_bg.width
height: img_bg.height
Image
{
id:img_bg;
source: "qrc:/img/background.png"//背景
}
Rectangle
{
id:big_rect
width: 200;
height: 100
x:500
y:250
color:"#666666"
Rectangle
{
id: redRect
width: 100; height: 100
color: "red"
}
Rectangle
{
id: blueRect
x: redRect.width
width: 50;
height: 50
color: "blue"
states: State
{
name: "reparented"
ParentChange //处于此状态时,blueRect的父项由big_rect变成redRect,ParentAnimation是展示变化过程的变化
{
target: blueRect;
parent: redRect;
x: 10;
y: 10
}
}
transitions: Transition
{
ParentAnimation
{
NumberAnimation
{
properties: "x,y";
duration: 1000
}
}
}
MouseArea
{
anchors.fill: parent;
onClicked: blueRect.state = "reparented"
}
}
}
}
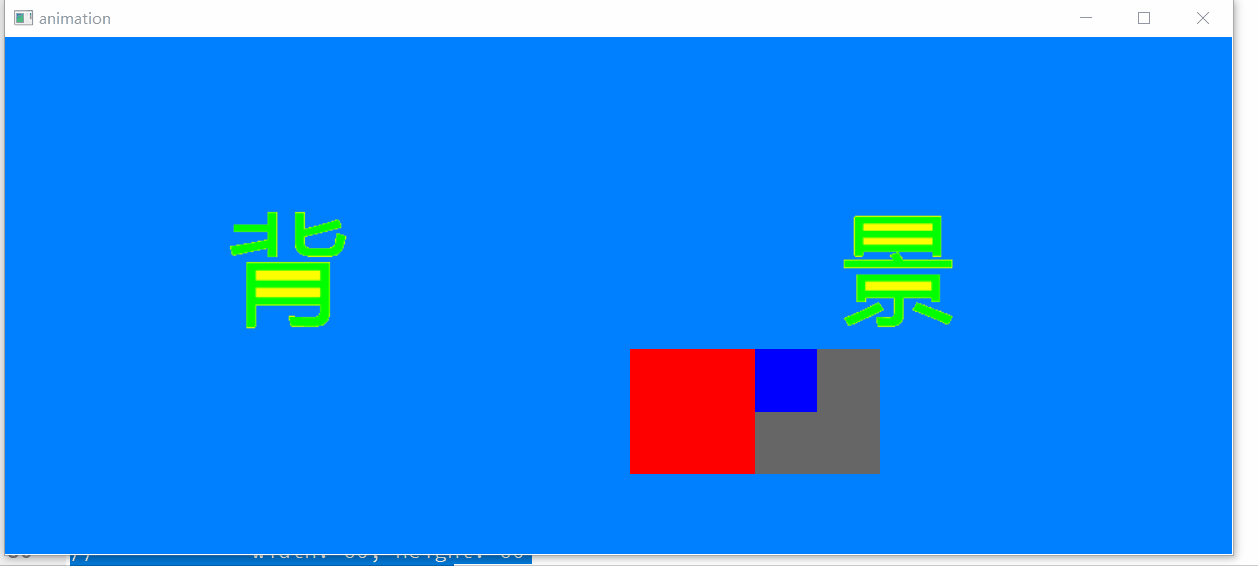
例5:平滑动画示例
import QtQuick 2.9
import QtQuick.Window 2.2
Window
{
id:win
visible: true
width: 800
height: 600
Rectangle
{
width: 800;
height: 600
color: "blue"
Rectangle
{
width: 60; height: 60
x: rect1.x - 5;
y: rect1.y - 5
color: "green"
Behavior on x //属性策略:x改变时不是瞬间变化,使用平滑动画缓慢变化
{
SmoothedAnimation
{
velocity: 200//速度
}
}
Behavior on y
{
SmoothedAnimation
{
velocity: 200
}
}
}
Rectangle
{
id: rect1
width: 50;
height: 50
color: "red"
}
focus: true
Keys.onRightPressed: rect1.x = rect1.x + 100
Keys.onLeftPressed: rect1.x = rect1.x - 100
Keys.onUpPressed: rect1.y = rect1.y - 100
Keys.onDownPressed: rect1.y = rect1.y + 100
}
}
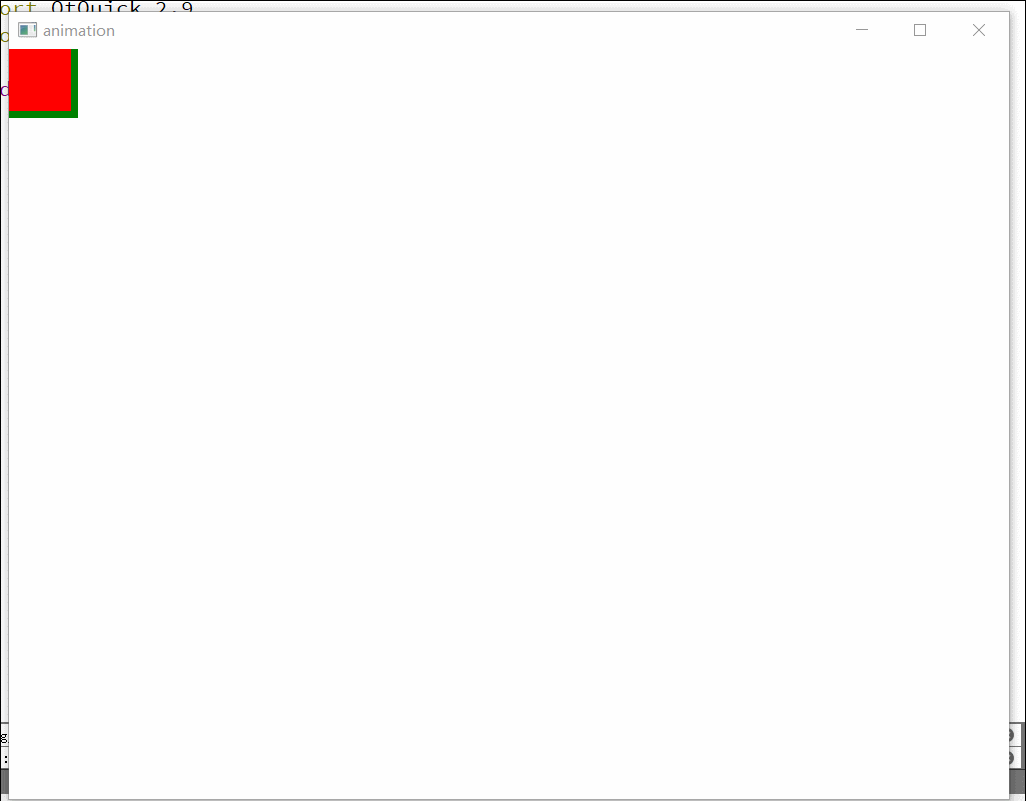
例6:弹簧动画示例
import QtQuick 2.9
import QtQuick.Window 2.2
Window
{
id:win
visible: true
width: 800
height: 600
Rectangle
{
id: rootItem
anchors.fill: parent
Rectangle
{
id: rect
width: 100
height: 100
x: 20
y: 20
color: "red"
}
ParallelAnimation //并行动画
{
id:parallelId
SpringAnimation //弹簧动画 属性变化的弹簧效果
{
id: springX
target: rect
property: "x"
spring: 3
damping: 0.06
epsilon: 0.25
}
SpringAnimation
{
id: springY
target: rect
property: "y"
spring: 3
damping: 0.06
epsilon: 0.25
}
}
MouseArea
{
anchors.fill: parent
onClicked:(mouse)=>
{
springX.from = rect.x
springX.to = mouse.x
springY.from = rect.y
springY.to = mouse.y
parallelId.start()
}
}
}
}
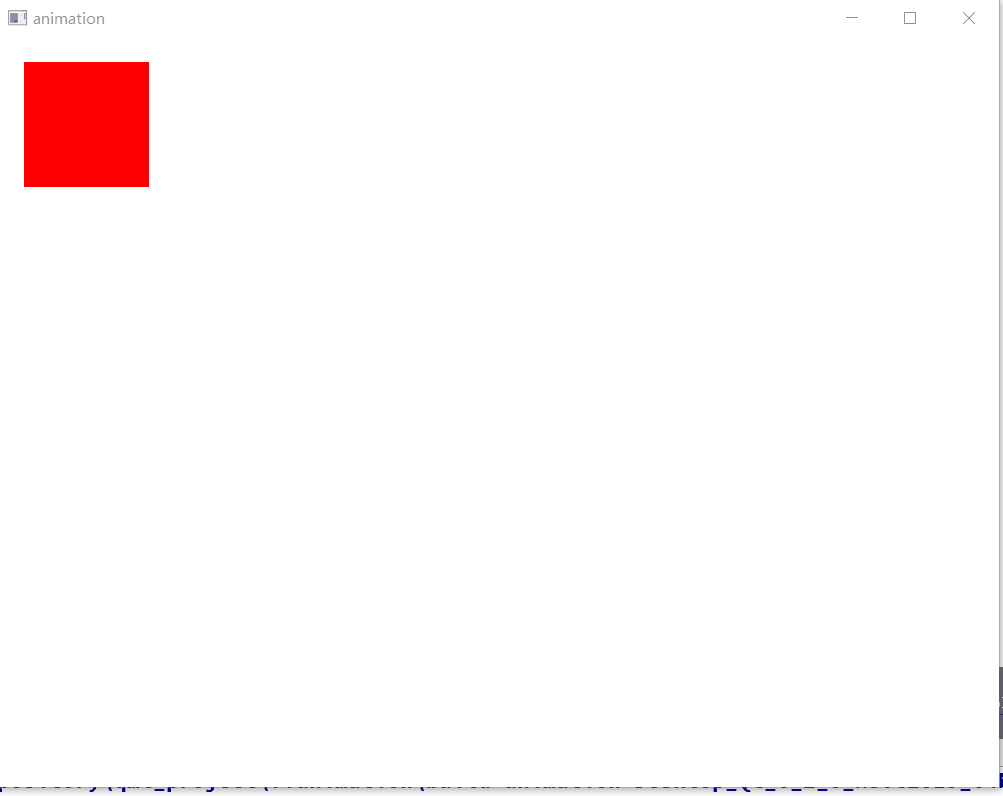
例7:路径动画示例
import QtQuick 2.9
import QtQuick.Window 2.2
Window
{
id:win
visible: true
width: 800
height: 600
Rectangle
{
id: window
anchors.fill: parent
color: "#000040"
Canvas
{
id: canvas
anchors.fill: parent
smooth: true
onPaint:
{
var context = canvas.getContext("2d")
context.clearRect(0, 0, width, height)
context.strokeStyle = "white"
context.path = pathAnim.path
context.stroke()
}
}
Item
{
id: rocket
x: 0; y: 0
width: 128; height: 96
Image
{
source: "qrc:/img/rocket.png"
anchors.centerIn: parent
rotation: 90
}
MouseArea
{
anchors.fill: parent
onClicked: pathAnim.running ? pathAnim.stop() : pathAnim.start()
}
}
PathAnimation
{
id: pathAnim
duration: 2000
easing.type: Easing.InOutQuad//设置缓冲曲线
target: rocket
orientation: PathAnimation.RightFirst
anchorPoint: Qt.point(rocket.width/2, rocket.height/2)
path: Path
{
startX: rocket.width/2; startY: rocket.height/2
PathCubic
{
x: window.width - rocket.width/2
y: window.height - rocket.height/2
control1X: x; control1Y: rocket.height/2
control2X: rocket.width/2; control2Y: y
}
}
}
}
}
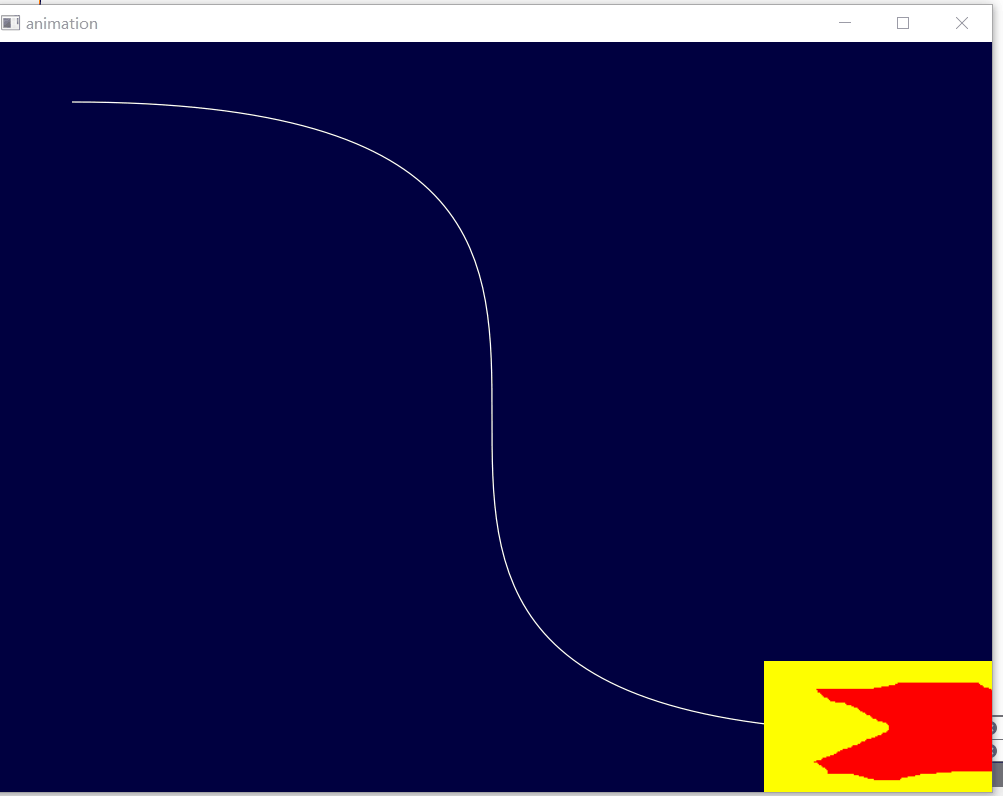