http://www.cnblogs.com/zzandlx/archive/2009/08/10/1542918.html
Socket服务器与客户端双向通信实例
整理整理前些天做的一个关于Socket的客户端与服务器之间相互通信的例子
基于Socket的即时通信 分为:客户端与服务器两部分!
服务器端的Socket绑定本机的ip地址
客户端的Socket则根据服务器的ip地址连接到服务器
之后服务器与客户端之间就能通过Socket进行通信了!
以下是服务器端代码
服务器的主要功能就是一直处于监听状态 监听来自客户端的Socket的请求
点击开始监听按钮之后
客户端则只要使用Socket去连接到服务器端的Socket就可实现往服务器上发信息
运行效果为:
以上采用的是同步Socket通信方式
基于Socket的即时通信 分为:客户端与服务器两部分!
服务器端的Socket绑定本机的ip地址
客户端的Socket则根据服务器的ip地址连接到服务器
之后服务器与客户端之间就能通过Socket进行通信了!
以下是服务器端代码
服务器的主要功能就是一直处于监听状态 监听来自客户端的Socket的请求
using
System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Net;
using System.Net.Sockets; // 添加命名空间
using System.Threading; // 添加命名空间
namespace WFAsynSocket
{
public partial class Form1 : Form
{
Thread LisThread;
Socket LisSocket;
Socket newSocket;
EndPoint point;
string strmes = String.Empty;
int port = 8000 ; // 定义侦听端口号
public Form1()
{
InitializeComponent();
}
private void btn_Listen_Click( object sender, EventArgs e)
{
LisThread = new Thread( new ThreadStart(BeginListern)); // 开线程执行BeginListern方法
LisThread.Start(); // 线程开始执行
}
public IPAddress GetIP()
{ /* 获取本地服务器的ip地址 */
IPHostEntry iep = Dns.GetHostEntry(Dns.GetHostName());
IPAddress ip = iep.AddressList[ 0 ];
return ip;
}
public void BeginListern()
{
LisSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp); // 实例化Socket
IPAddress ServerIp = GetIP(); /* 获取本地服务器的ip地址 */
IPEndPoint iep = new IPEndPoint(ServerIp, port);
LisSocket.Bind(iep); /* 将Socket绑定ip */
toolStripStatusLabel1.Text = iep.ToString() + " 正在监听
"
;
LisSocket.Listen( 50 ); // Socket开始监听
newSocket = LisSocket.Accept(); // 获取连接请求的Socket
/* 接收客户端Socket所发的信息 */
while ( true )
{
try
{
byte [] byteMessage = new byte [ 100 ];
newSocket.Receive(byteMessage); // 接收信息
MessageBox.Show(Encoding.Default.GetString(byteMessage));
Control.CheckForIllegalCrossThreadCalls = false ;
point = newSocket.RemoteEndPoint; // 获取客户端的Socket的相关信息
IPEndPoint IPpoint = (IPEndPoint)point;
strmes += IPpoint.Address.ToString() + " " + DateTime.Now.ToString() + " 说 " + Encoding.Default.GetString(byteMessage).Trim( new char [] { ' /0 ' }) + " /r/n " ;
this .richTextBox1.Text = strmes;
}
catch (SocketException ex)
{
toolStripStatusLabel1.Text += ex.ToString();
}
}
}
private void btn_Cancel_Click( object sender, EventArgs e)
{
try
{
LisSocket.Close(); // 关闭Socket
LisThread.Abort(); // 线程停止
LisThread = null ;
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
finally
{
Application.Exit();
}
}
private void btn_Send_Click( object sender, EventArgs e)
{
byte [] byteData = Encoding.Default.GetBytes( this .richTextBox2.Text);
newSocket.Send(byteData); // 发送信息即由服务器往客户端上发信息
}
}
}
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Net;
using System.Net.Sockets; // 添加命名空间
using System.Threading; // 添加命名空间
namespace WFAsynSocket
{
public partial class Form1 : Form
{
Thread LisThread;
Socket LisSocket;
Socket newSocket;
EndPoint point;
string strmes = String.Empty;
int port = 8000 ; // 定义侦听端口号
public Form1()
{
InitializeComponent();
}
private void btn_Listen_Click( object sender, EventArgs e)
{
LisThread = new Thread( new ThreadStart(BeginListern)); // 开线程执行BeginListern方法
LisThread.Start(); // 线程开始执行
}
public IPAddress GetIP()
{ /* 获取本地服务器的ip地址 */
IPHostEntry iep = Dns.GetHostEntry(Dns.GetHostName());
IPAddress ip = iep.AddressList[ 0 ];
return ip;
}
public void BeginListern()
{
LisSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp); // 实例化Socket
IPAddress ServerIp = GetIP(); /* 获取本地服务器的ip地址 */
IPEndPoint iep = new IPEndPoint(ServerIp, port);
LisSocket.Bind(iep); /* 将Socket绑定ip */
toolStripStatusLabel1.Text = iep.ToString() + " 正在监听

LisSocket.Listen( 50 ); // Socket开始监听
newSocket = LisSocket.Accept(); // 获取连接请求的Socket
/* 接收客户端Socket所发的信息 */
while ( true )
{
try
{
byte [] byteMessage = new byte [ 100 ];
newSocket.Receive(byteMessage); // 接收信息
MessageBox.Show(Encoding.Default.GetString(byteMessage));
Control.CheckForIllegalCrossThreadCalls = false ;
point = newSocket.RemoteEndPoint; // 获取客户端的Socket的相关信息
IPEndPoint IPpoint = (IPEndPoint)point;
strmes += IPpoint.Address.ToString() + " " + DateTime.Now.ToString() + " 说 " + Encoding.Default.GetString(byteMessage).Trim( new char [] { ' /0 ' }) + " /r/n " ;
this .richTextBox1.Text = strmes;
}
catch (SocketException ex)
{
toolStripStatusLabel1.Text += ex.ToString();
}
}
}
private void btn_Cancel_Click( object sender, EventArgs e)
{
try
{
LisSocket.Close(); // 关闭Socket
LisThread.Abort(); // 线程停止
LisThread = null ;
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
finally
{
Application.Exit();
}
}
private void btn_Send_Click( object sender, EventArgs e)
{
byte [] byteData = Encoding.Default.GetBytes( this .richTextBox2.Text);
newSocket.Send(byteData); // 发送信息即由服务器往客户端上发信息
}
}
}
点击开始监听按钮之后
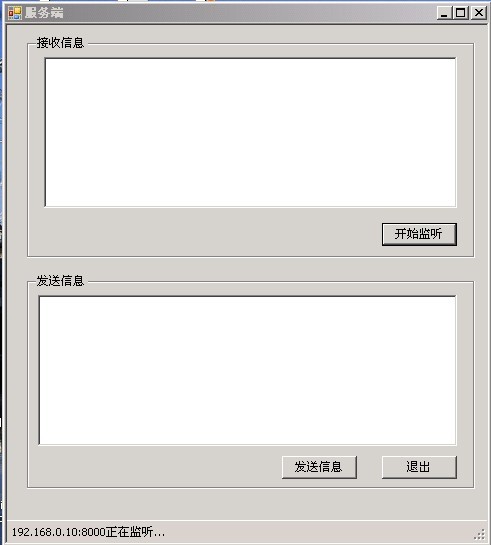
客户端则只要使用Socket去连接到服务器端的Socket就可实现往服务器上发信息
using
System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Threading;
using System.Net.Sockets;
using System.Net;
namespace WFAsynSoketClient
{
public partial class Form1 : Form
{
public static Socket ClientSocket;
public Form1()
{
InitializeComponent();
}
/* 往服务器上发送信息按钮事件 */
private void btn_Send_Click( object sender, EventArgs e)
{
if (ClientSocket.Connected) // 判断Socket是否已连接
{
byte [] SendMessage = new byte [ 100 ];
SendMessage = Encoding.ASCII.GetBytes( this .richTextBox1.Text);
ClientSocket.Send(SendMessage); // 从数据中的指示位置开始将数据发送到连接的Socket。
MessageBox.Show(Encoding.Default.GetString(SendMessage) + " 发送成功! " );
}
else
{
MessageBox.Show( " 未建立连接! " );
}
}
/* 退出按钮 */
private void btn_Cancel_Click( object sender, EventArgs e)
{
ClientSocket.Shutdown(SocketShutdown.Both); // 发送完成之后停止Socket
ClientSocket.Close(); // 发送完成之后关闭Socket
Application.Exit();
}
/* 接收来自服务器上的信息 */
public void targett()
{
this .toolStripStatusLabel1.Text = " 已经建立连接准备接受数据 " ;
while ( true )
{
byte [] bytes = new byte [ 100 ];
int rev = ClientSocket.Receive(bytes,bytes.Length, 0 ); // 将数据从连接的 Socket 接收到接收缓冲区的特定位置。
if (rev <= 0 )
{
break ;
}
string strev = System.Text.Encoding.Default.GetString(bytes);
this .textBox1.AppendText( " 服务器对客户端说: " + strev + " /r/n " );
}
}
private void Form1_Load( object sender, EventArgs e)
{
}
/* 为客户端Socket建立连接到服务器 */
private void button1_Click( object sender, EventArgs e)
{
ClientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
string ip = " 192.168.1.26 " ; // 服务器ip
IPAddress ipa = IPAddress.Parse(ip);
IPEndPoint iep = new IPEndPoint(ipa, 8000 );
this .toolStripStatusLabel1.Text = " 已经建立连接
.
"
;
Control.CheckForIllegalCrossThreadCalls = false ;
try
{
ClientSocket.Connect(iep); // 连接到服务器
Thread thread = new Thread( new ThreadStart(targett));
thread.Start();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
}
客户端界面为:
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Threading;
using System.Net.Sockets;
using System.Net;
namespace WFAsynSoketClient
{
public partial class Form1 : Form
{
public static Socket ClientSocket;
public Form1()
{
InitializeComponent();
}
/* 往服务器上发送信息按钮事件 */
private void btn_Send_Click( object sender, EventArgs e)
{
if (ClientSocket.Connected) // 判断Socket是否已连接
{
byte [] SendMessage = new byte [ 100 ];
SendMessage = Encoding.ASCII.GetBytes( this .richTextBox1.Text);
ClientSocket.Send(SendMessage); // 从数据中的指示位置开始将数据发送到连接的Socket。
MessageBox.Show(Encoding.Default.GetString(SendMessage) + " 发送成功! " );
}
else
{
MessageBox.Show( " 未建立连接! " );
}
}
/* 退出按钮 */
private void btn_Cancel_Click( object sender, EventArgs e)
{
ClientSocket.Shutdown(SocketShutdown.Both); // 发送完成之后停止Socket
ClientSocket.Close(); // 发送完成之后关闭Socket
Application.Exit();
}
/* 接收来自服务器上的信息 */
public void targett()
{
this .toolStripStatusLabel1.Text = " 已经建立连接准备接受数据 " ;
while ( true )
{
byte [] bytes = new byte [ 100 ];
int rev = ClientSocket.Receive(bytes,bytes.Length, 0 ); // 将数据从连接的 Socket 接收到接收缓冲区的特定位置。
if (rev <= 0 )
{
break ;
}
string strev = System.Text.Encoding.Default.GetString(bytes);
this .textBox1.AppendText( " 服务器对客户端说: " + strev + " /r/n " );
}
}
private void Form1_Load( object sender, EventArgs e)
{
}
/* 为客户端Socket建立连接到服务器 */
private void button1_Click( object sender, EventArgs e)
{
ClientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
string ip = " 192.168.1.26 " ; // 服务器ip
IPAddress ipa = IPAddress.Parse(ip);
IPEndPoint iep = new IPEndPoint(ipa, 8000 );
this .toolStripStatusLabel1.Text = " 已经建立连接

Control.CheckForIllegalCrossThreadCalls = false ;
try
{
ClientSocket.Connect(iep); // 连接到服务器
Thread thread = new Thread( new ThreadStart(targett));
thread.Start();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
}
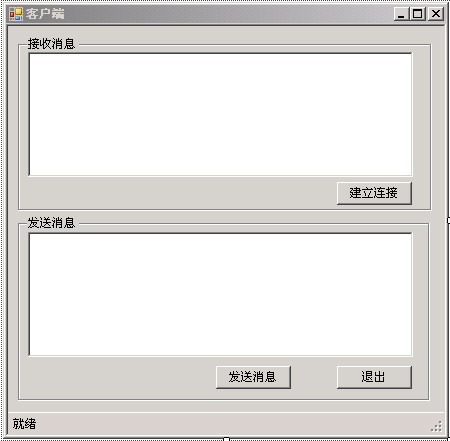
运行效果为:
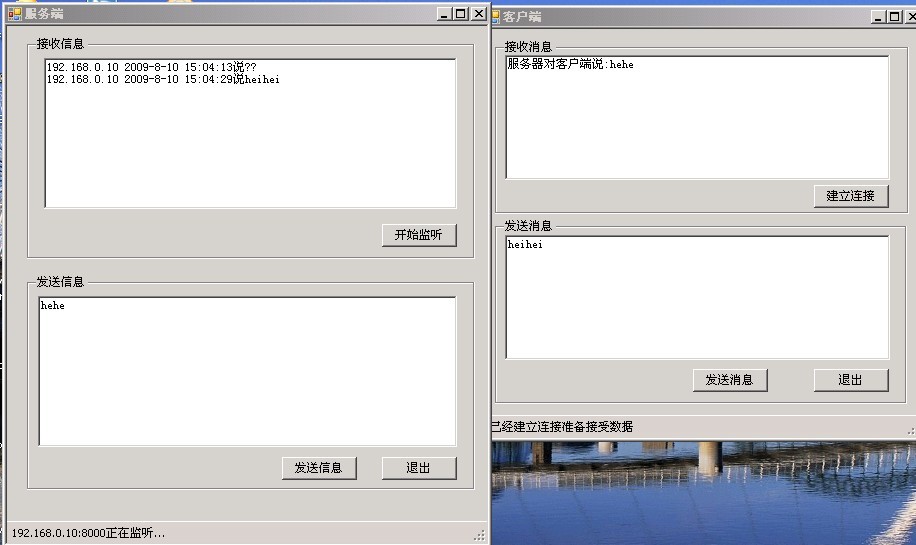
以上采用的是同步Socket通信方式