React Native 版本执行0.57的规则
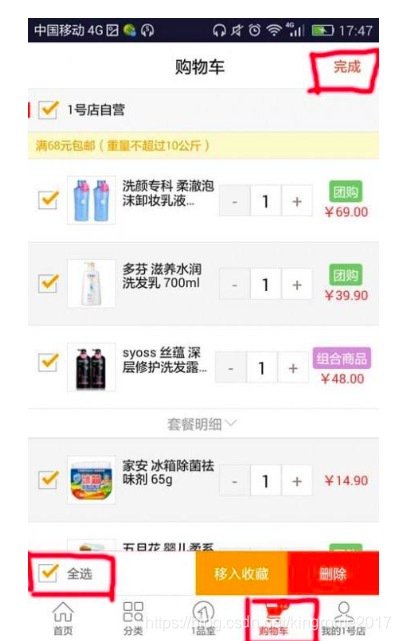
import React, { Component } from 'react';
import { StyleSheet, Text, View, ScrollView, DeviceEventEmitter, Image, TouchableOpacity, StatusBar } from 'react-native';
import TabNav from '../../components/TabNav';
import Px2dp from '../../utils/Px2dp';
import Loading from '../../components/Loading';
import { requestUrl } from '../../network/Url';
import Fetch from '../../network/Fetch'
import Confirm from '../../components/Confirm'
import { NavigationEvents } from "react-navigation";
export default class Cart extends Component {
constructor(props) {
super(props);
this.state = {
isLoading: false,
isdetele: false,
editStatus: false,
cartList: [],
isSelectedAllItem: false,
newCartList: [],
totalPrice: 0
}
}
render() {
const { navigate } = this.props.navigation;
return (
<View style={[styles.container, this.state.cartList.length > 0 ? null : { backgroundColor: Colors.white }]}>
<NavigationEvents
onWillFocus={() => {
this._getShopping()
}}
/>
<StatusBar
animated={true}
hidden={false}
backgroundColor={'#000'}
translucent={false}
showHideTransition="fade"
networkActivityIndicatorVisible={this.state.isLoading}
statusBarStyle={"default"}
barStyle={"default"}
/>
<TabNav title="购物车" rightDom={
<Text style={styles.headTxt}>{this.state.editStatus && this.state.cartList.length != 0 ? "完成" : "编辑"}</Text>
}
rightClick={() => {
this.setState({
editStatus: !this.state.editStatus
})
}} />
<ScrollView>
{}
{
this.state.cartList.length > 0 ?
this.state.newCartList.map((items, indexs) => {
return (
<View style={styles.commonItem} key={indexs}>
{}
<View style={styles.commonItemTop}>
{}
<TouchableOpacity style={styles.itemCheckBox} activeOpacity={0.8} onPress={() => {
let tempArr = this.state.newCartList;
tempArr[indexs].checked = !tempArr[indexs].checked;
tempArr[indexs].items.map((its) => {
its.checked = tempArr[indexs].checked
})
let secected = true
tempArr.map((w) => {
if (!w.checked) {
secected = false;
}
})
this._calculateCountAndPrice()
this.setState({
newCartList: tempArr,
isSelectedAllItem: secected
})
}}>
<Image source={items.checked ? require('../../images/on_icon.png') : require('../../images/off_icon.png')} />
</TouchableOpacity>
<Text style={styles.commonItemTopTit}>{items.providerName}</Text>
</View>
{}
{
items.items.map((item, index) => {
return (
<View key={index}>
<View style={styles.commonItemMiddle}>
{}
<TouchableOpacity style={styles.itemCheckShopBox} activeOpacity={0.8} onPress={() => {
let temp = this.state.newCartList;
temp[indexs].items[index].checked = !temp[indexs].items[index].checked;
let secected = true
temp[indexs].items.map((it) => {
if (!it.checked) {
secected = false;
}
})
temp[indexs].checked = secected
temp.map((j) => {
if (!j.checked) {
secected = false;
}
})
this._calculateCountAndPrice()
this.setState({
newCartList: temp,
isSelectedAllItem: secected
})
}}>
<Image source={item.checked ? require('../../images/on_icon.png') : require('../../images/off_icon.png')} />
</TouchableOpacity>
<Image source={{ uri: item.list_image }} style={{ width: Px2dp(80), height: Px2dp(80), backgroundColor: '#ccc', marginRight: Px2dp(10) }} />
<TouchableOpacity activeOpacity={0.8} onPress={() => {
navigate('Commoditydetails', { goodsInfo: item })
}} style={styles.commonItemMiddleBox}>
<Text numberOfLines={2} style={styles.commonItemMiddleTit}>{item.full_name}</Text>
<Text style={styles.commonItemMiddleTxt}>规格:XX</Text>
<View style={styles.commonItemMiddleMake}>
<View style={styles.price}>
<Text style={styles.priceBz}>¥</Text>
<Text style={styles.priceNum}>{Number(item.member_price).toFixed(2)}
</Text>
</View>
{}
<View style={styles.handleBox}>
<TouchableOpacity activeOpacity={0.8} onPress={() => {
this._minusShopNum(indexs, index)
}} style={[styles.commonMake, item.num == 1 ? { borderColor: '#e0e0e0' } : null]}>
<Image source={item.num == 1 ? require('../../images/minus_n_icon.png') : require('../../images/minus_y_icon.png')} />
</TouchableOpacity>
<View activeOpacity={0.8} style={styles.commonMake}>
<Text style={styles.numTxt}>{item.num}</Text>
</View>
<TouchableOpacity activeOpacity={0.8} onPress={() => {
this._addShopNum(indexs, index)
}} style={[styles.commonMake, item.num >= item.stock ? { borderColor: '#e0e0e0' } : null]}>
<Image source={item.num >= item.stock ? require('../../images/add_num_n_icon.png') : require('../../images/add_num_icon.png')} />
</TouchableOpacity>
</View>
</View>
</TouchableOpacity>
</View>
{}
{
item.gift_id ?
<TouchableOpacity style={styles.commonItemBom} activeOpacity={0.8} onPress={() => { }}>
<View style={styles.tagBox}>
<Text style={styles.tagBoxTxt}>赠品</Text>
</View>
<Text style={styles.tagContent}>{item.gift_name}</Text>
<Image source={require('../../images/cart_more.png')} />
</TouchableOpacity>
: null
}
</View>
)
})
}
</View>
)
})
:
<View style={styles.noCartList}>
<Image source={require('../../images/cart_no_shops.png')} />
<Text style={styles.noDataBoxTxt}>购物车空空如也,赶紧去挑点好东西吧~</Text>
<TouchableOpacity activeOpacity={0.8} style={styles.noDataBoxBtn}>
<Text style={styles.noDataBoxBtnTxt}>去shopping</Text>
</TouchableOpacity>
</View>
}
</ScrollView>
{}
{
this.state.cartList.length > 0 ?
< View style={styles.cartBar}>
{}
<TouchableOpacity style={styles.itemCheckBox} activeOpacity={0.8} onPress={() => {
this.state.newCartList.map((item) => {
item.checked = !this.state.isSelectedAllItem
item.items.map((ite) => {
ite.checked = !this.state.isSelectedAllItem
})
})
this._calculateCountAndPrice()
this.setState({
newCartList: this.state.newCartList,
isSelectedAllItem: !this.state.isSelectedAllItem
})
}}>
<Image source={this.state.isSelectedAllItem ? require('../../images/on_icon.png') : require('../../images/off_icon.png')} />
<Text style={styles.checkTxt}>全选</Text>
</TouchableOpacity>
<View style={styles.priceTotle}>
<Text style={styles.priceTotleTit}>合计:</Text>
<Text style={styles.priceTotleNum}>¥{Number(this.state.totalPrice).toFixed(2)}</Text>
</View>
<TouchableOpacity activeOpacity={0.8} style={styles.settleBtn} onPress={() => {
if (this.state.editStatus) {
this.setState({
isdetele: true,
})
}
else if (this._get_checked_goods().length != 0) {
navigate('SettlementOrder', { selecteData: this._get_checked_goods() })
}
else {
return true
}
}}>
<Text style={styles.settleTxt}>{this.state.editStatus ? "删除" : "去结算"}</Text>
</TouchableOpacity>
</View>
: null
}
{this.state.isLoading ? <Loading /> : null}
{}
<Confirm visible={this.state.isdetele}
text="确定要删除吗?"
yesClick={() => {
this.setState({
isdetele: false,
})
this._delete_goods()
}}
noClick={() => {
this.setState({
isdetele: false
})
}}
/>
</View >
);
}
_minusShopNum(indexs, index) {
let tempStatus = this.state.newCartList
let shop = tempStatus[indexs]
let items = shop.items
let item = items[index]
if (item.num <= 1) {
return false
} else {
item.num -= 1;
CartNum -= 1;
}
DeviceEventEmitter.emit('ShopNum', CartNum);
if (item.checked) {
this._calculateCountAndPrice()
}
this._changeGoodsNum(item.id, item.num)
}
_addShopNum(indexs, index) {
let tempStatus = this.state.newCartList
let shop = tempStatus[indexs]
let items = shop.items
let item = items[index]
if (item.num >= item.stock) {
return false
} else {
item.num += 1;
CartNum += 1;
}
DeviceEventEmitter.emit('ShopNum', CartNum);
if (item.checked) {
this._calculateCountAndPrice()
}
this._changeGoodsNum(item.id, item.num)
}
_calculateCountAndPrice() {
let tempTotalPrice = 0
let multiple = 100
let tempStatus = this.state.newCartList
tempStatus.map((shop) => {
shop.items.map((item) => {
if (item.checked) {
tempTotalPrice += Number(item.member_price) * multiple * item.num * multiple
}
})
})
this.setState({ totalPrice: tempTotalPrice / (multiple * multiple) })
}
_get_checked_goods() {
let dataArr = this.state.newCartList
let tempStatusArr = []
dataArr.map((items) => {
let shopObj = {};
shopObj.providerName = items.providerName
let tempItems = [];
items.items.map((item) => {
if (item.checked) {
tempItems.push(item)
}
})
shopObj.items = tempItems
if (tempItems.length != 0) {
tempStatusArr.push(shopObj)
}
})
return tempStatusArr
}
_changeGoodsNum(goodsid, num) {
this.setState({
isLoading: true,
})
Fetch(requestUrl.url, {
"Id": id,
"num": num,
"sellerId": '0',
"goodId": goodsid
}).then(data => {
if (data.status == "SUCCESS") {
this.setState({
isLoading: false,
})
} else if (data.status == "ERROR") {
this.setState({
isLoading: false,
})
ToastShow({ "text": '获取购物车信息失败' })
} else {
this.setState({
isLoading: false,
})
ToastShow({ "text": '获取购物车信息失败' })
}
})
}
_delete_goods() {
let checked_data = this._get_checked_goods()
let ids = []
let num = 0
checked_data.map((shop) => {
shop.items.map((item) => {
ids.push(item.id)
num = item.num
})
})
Fetch(requestUrl.url, {
"Id": id,
"ids": ids
}).then(data => {
if (data.status == "SUCCESS") {
this._getShopping()
DeviceEventEmitter.emit('ShopNum', CartNum -= num);
this.setState({
isLoading: false,
})
} else if (data.status == "ERROR") {
this.setState({
isLoading: false,
})
ToastShow({ "text": '删除购物车商品失败' })
} else {
this.setState({
isLoading: false,
})
ToastShow({ "text": '删除购物车商品失败' })
}
})
}
_getShopping() {
this.setState({
isLoading: true,
})
Fetch(requestUrl.url, {
"Id": id,
}).then(data => {
if (data.status == "SUCCESS") {
this.setState({
isLoading: false,
cartList: data.data
})
console.log(data.data)
this._loopAddChecked()
this._calculateCountAndPrice()
} else if (data.status == "ERROR") {
this.setState({
isLoading: false,
})
ToastShow({ "text": '获取购物车信息失败' })
} else {
this.setState({
isLoading: false,
})
ToastShow({ "text": '获取购物车信息失败' })
}
})
}
_loopAddChecked() {
let tempStatusArr = [];
this.state.cartList.map((item) => {
let shopProductObj = {}
shopProductObj.checked = false
shopProductObj.providerName = item.providerName
let tempItems = [];
item.goodList.map((items) => {
items.checked = false
tempItems.push(items)
})
shopProductObj.items = tempItems
tempStatusArr.push(shopProductObj)
})
this.setState({
newCartList: tempStatusArr
})
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: Colors.mainBg,
},
commonItem: {
backgroundColor: Colors.white,
paddingRight: Px2dp(16),
paddingBottom: Px2dp(16)
},
commonItemTop: {
height: Px2dp(46),
borderBottomWidth: Pixel,
borderBottomColor: '#ececec',
flexDirection: 'row',
alignItems: 'center'
},
commonItemTopTit: {
fontSize: Px2dp(14),
color: Colors.text333
},
itemCheckBox: {
height: Px2dp(46),
paddingLeft: Px2dp(16),
paddingRight: Px2dp(6),
flexDirection: "row",
alignItems: "center",
justifyContent: 'center',
},
commonItemMiddle: {
flexDirection: "row",
marginTop: Px2dp(16)
},
itemCheckShopBox: {
height: Px2dp(80),
alignItems: "center",
justifyContent: 'center',
paddingLeft: Px2dp(16),
paddingRight: Px2dp(8),
},
commonItemMiddleTit: {
fontSize: Px2dp(13),
lineHeight: Px2dp(18),
color: Colors.text333,
width: Px2dp(229)
},
commonItemMiddleTxt: {
fontSize: Px2dp(12),
lineHeight: Px2dp(17),
color: Colors.text888,
marginTop: Px2dp(2),
marginBottom: Px2dp(4)
},
price: {
flexDirection: "row",
alignItems: "flex-end",
flex: 1
},
priceBz: {
fontSize: Px2dp(12),
lineHeight: Px2dp(17),
color: Colors.red,
},
priceNum: {
fontSize: Px2dp(15),
lineHeight: Px2dp(17),
color: Colors.red,
},
commonItemMiddleMake: {
flexDirection: 'row'
},
handleBox: {
flexDirection: 'row',
alignItems: "center",
paddingRight: Px2dp(16)
},
commonMake: {
width: Px2dp(30),
height: Px2dp(20),
borderWidth: Pixel,
borderColor: '#999',
justifyContent: 'center',
alignItems: 'center',
},
numTxt: {
color: Colors.text888
},
commonItemBom: {
flexDirection: "row",
alignItems: 'center',
justifyContent: "center",
paddingLeft: Px2dp(40),
paddingRight: Px2dp(16),
marginTop: Px2dp(16)
},
tagBox: {
width: Px2dp(32),
height: Px2dp(18),
borderColor: '#FF9600',
borderRadius: Px2dp(2),
borderWidth: Pixel,
justifyContent: 'center',
alignItems: 'center',
},
tagBoxTxt: {
fontSize: Px2dp(13),
color: '#FF9600',
paddingLeft: Px2dp(2)
},
tagContent: {
fontSize: Px2dp(13),
color: Colors.text666,
lineHeight: Px2dp(18),
flex: 1,
marginLeft: Px2dp(6)
},
cartBar: {
backgroundColor: Colors.white,
height: Px2dp(50),
flexDirection: "row",
alignItems: 'center',
},
checkTxt: {
fontSize: Px2dp(14),
color: Colors.text333,
lineHeight: Px2dp(20),
marginLeft: Px2dp(8)
},
priceTotle: {
flexDirection: "row",
alignItems: 'center',
justifyContent: 'center',
marginLeft: Px2dp(60),
flex: 1,
marginRight: Px2dp(10)
},
priceTotleTit: {
fontSize: Px2dp(14),
color: Colors.text333
},
priceTotleNum: {
fontSize: Px2dp(15),
color: Colors.red
},
settleBtn: {
width: Px2dp(100),
height: Px2dp(50),
backgroundColor: Colors.red,
justifyContent: 'center',
alignItems: 'center'
},
settleTxt: {
fontSize: Px2dp(14),
color: Colors.white
},
noCartList: {
justifyContent: "center",
alignItems: "center",
paddingTop: SCREEN_HEIGHT * .16,
},
noDataBoxTxt: {
fontSize: Px2dp(14),
color: Colors.text888,
marginTop: Px2dp(20)
},
noDataBoxBtn: {
flexDirection: "row",
alignItems: "center",
justifyContent: "center",
width: Px2dp(185),
height: Px2dp(40),
backgroundColor: "#eb3033",
borderRadius: Px2dp(20),
marginTop: Px2dp(50),
},
noDataBoxBtnTxt: {
fontSize: Px2dp(15),
color: Colors.white,
}
});