采用 Spring + Hibernate + JSTL 开发web项目。
一个表 对应一个 PO,一个Validator,一个VO 和 一个表单(其实是两个,一个新增,一个编辑)
这些POs, VOs, Validators, Forms,全都依赖于表的数据
除了表的数据不一致外,其框架与操作惊人的一致
考虑,基于一个表,是否可以动态生成这些呢?
比如,一个客户表单:
其数据库建表SQL如下:
create table customer (id int identity not null, address varchar(100) null, age int not null, answer varchar(50) null, email varchar(50) null, gender varchar(8) null, intOptional int not null, password varchar(50) null, phone varchar(50) null, question varchar(100) null, realname varchar(20) null, registerTime datetime null, strOptional varchar(100) null, username varchar(50) null, status int not null, score int not null, sd int not null, role int not null, primary key (id));
对应的PO如下:
package
net.kofsky.nmis.po;

import
java.io.Serializable;
import
java.sql.Timestamp;


/** */
/**
* 用户信息
* @author kofsky
*
* @hibernate.class table="customer"
*
*/

public
class
Customer
implements
Serializable
...
{
private static final long serialVersionUID = 1310607107787515532L;

/** *//**
* ID
*/
private int id;

/** *//**
* 用户登录姓名
*/
private String username;


/** *//**
* 用户真实姓名
*/
private String realname;

/** *//**
* 密码
*/
private String password;


/** *//**
* 性别
*/
private String gender;

/** *//**
* 年龄
*/
private int age;

/** *//**
* 联系电话
*/
private String phone;

/** *//**
* 联系地址
*/
private String address;

/** *//**
* email
*/
private String email;

/** *//**
* 密码提示问题
*/
private String question;

/** *//**
* 密码提示问题的答案
*/
private String answer;

/** *//**
* 用户注册时间
*/
private Timestamp registerTime;

/** *//**
* 用户的当前状态
*/
private int status;

/** *//**
* 用户积分
*/
private int score;

/** *//**
* 可选
*/
private String strOptional;

/** *//**
* 可选
*/
private int intOptional;

/** *//**
* 序列号:备用ID
*/
private int sd;

/** *//**
* 用户的角色
*/
private int role;


/** *//**
* @hibernate.id column="id" type="java.lang.Integer"
* generator-class="native"
*/

public int getId() ...{
return id;
}


public void setId(int id) ...{
this.id = id;
}


/** *//**
* @hibernate.property column="address" type="java.lang.String" length="100"
*/

public String getAddress() ...{
return address;
}


public void setAddress(String address) ...{
this.address = address;
}


/** *//**
* @hibernate.property column="age" type="java.lang.Integer" not-null="true"
*/

public int getAge() ...{
return age;
}


public void setAge(int age) ...{
this.age = age;
}


/** *//**
* @hibernate.property column="answer" type="java.lang.String" length="50"
*/

public String getAnswer() ...{
return answer;
}


public void setAnswer(String answer) ...{
this.answer = answer;
}


/** *//**
* @hibernate.property column="email" type="java.lang.String" length="50"
*/

public String getEmail() ...{
return email;
}


public void setEmail(String email) ...{
this.email = email;
}


/** *//**
* @hibernate.property column="gender" type="java.lang.String" length="8"
*/

public String getGender() ...{
return gender;
}


public void setGender(String gender) ...{
this.gender = gender;
}


/** *//**
* @hibernate.property column="intOptional" type="java.lang.Integer" not-null="true"
*/

public int getIntOptional() ...{
return intOptional;
}


public void setIntOptional(int intOptional) ...{
this.intOptional = intOptional;
}


/** *//**
* @hibernate.property column="password" type="java.lang.String" length="50"
*/

public String getPassword() ...{
return password;
}


public void setPassword(String password) ...{
this.password = password;
}


/** *//**
* @hibernate.property column="phone" type="java.lang.String" length="50"
*/

public String getPhone() ...{
return phone;
}


public void setPhone(String phone) ...{
this.phone = phone;
}


/** *//**
* @hibernate.property column="question" type="java.lang.String" length="100"
*/

public String getQuestion() ...{
return question;
}


public void setQuestion(String question) ...{
this.question = question;
}


/** *//**
* @hibernate.property column="realname" type="java.lang.String" length="20"
*/

public String getRealname() ...{
return realname;
}


public void setRealname(String realname) ...{
this.realname = realname;
}


/** *//**
* @hibernate.property column="registerTime" type="java.sql.Timestamp"
*/

public Timestamp getRegisterTime() ...{
return registerTime;
}


public void setRegisterTime(Timestamp registerTime) ...{
this.registerTime = registerTime;
}


/** *//**
* @hibernate.property column="strOptional" type="java.lang.String" length="100"
*/

public String getStrOptional() ...{
return strOptional;
}


public void setStrOptional(String strOptional) ...{
this.strOptional = strOptional;
}


/** *//**
* @hibernate.property column="username" type="java.lang.String" length="50"
*/

public String getUsername() ...{
return username;
}


public void setUsername(String username) ...{
this.username = username;
}


/** *//**
* @hibernate.property column="status" type="java.lang.Integer" not-null="true"
*/

public int getStatus() ...{
return status;
}


public void setStatus(int status) ...{
this.status = status;
}


/** *//**
* @hibernate.property column="score" type="java.lang.Integer" not-null="true"
*/

public int getScore() ...{
return score;
}


public void setScore(int score) ...{
this.score = score;
}


/** *//**
* @hibernate.property column="sd" type="java.lang.Integer" not-null="true"
*/

public int getSd() ...{
return sd;
}


public void setSd(int sd) ...{
this.sd = sd;
}


/** *//**
* @hibernate.property column="role" type="java.lang.Integer" not-null="true"
*/

public int getRole() ...{
return role;
}


public void setRole(int role) ...{
this.role = role;
}
}
VO与PO大致一样的,因此就没有写专门的VO。
其用户表单验证Validator
package
net.kofsky.nmis.validator;

import
net.kofsky.nmis.command.CustomerCommand;

import
org.springframework.validation.Errors;
import
org.springframework.validation.Validator;


/** */
/**
* 注册时对用户注册表单进行校验
* @author 柳锋
*
*/
public
class
RegisterValidator
implements
Validator

...
{
public boolean supports(Class clazz)

...{
return clazz.equals(CustomerCommand.class);
}
public void validate(Object command,Errors errors)

...{
CustomerCommand customerCmd=(CustomerCommand)command;


if (customerCmd.getUsername().trim().length() < 2) ...{
errors.rejectValue("username",
"less4chars",
null,
"用户名长度必须大于等于2个字符");
}

if (customerCmd.getRealname().trim().length()<2) ...{
errors.rejectValue("realname",
"realnameless2chars",
null,
"真实姓名必须大于等于两个汉字");
}

if (customerCmd.getRealname().trim().length()>5) ...{
errors.rejectValue("realname",
"realnameless2chars",
null,
"真实姓名不能超过五个汉字");
}

if (customerCmd.getPassword1().trim().length() < 6) ...{
errors.rejectValue("password1",
"less6chars",
null,
"密码长度必须大于等于6个字母");
}

if (!customerCmd.getPassword1().trim().equals(customerCmd.getPassword2())) ...{
errors.rejectValue("password1",
"pswnotequal",
null,
"两次输入的密码不一致!");
}

if (customerCmd.getPhone().trim().length() < 6) ...{
errors.rejectValue("phone",
"phoneless5chars",
null,
"电话号码的位数不能低于六位");
}
if((customerCmd.getQuestion()!=null)&&(!customerCmd.getQuestion().trim().equals("")))

...{
if(customerCmd.getQuestion().trim().length()<5)

...{
errors.rejectValue("question",
"questionless5chars",
null,
"密码提示问题不能少于5个字符!");
}
if((customerCmd.getAnswer()!=null)&&(customerCmd.getAnswer().trim().length()<5))

...{
errors.rejectValue("answer",
"questionless5chars",
null,
"密码提示问题的答案不能少于5个字符!");
}
}
}
}
其对应的表单如下:
<%
@ page language
=
"
java
"
contentType
=
"
text/html; charset=GB18030
"
%>
<%
@ taglib prefix
=
"
c
"
uri
=
"
http://java.sun.com/jstl/core_rt
"
%>
<%
@ taglib prefix
=
"
spring
"
uri
=
"
http://www.springframework.org/tags
"
%>

<!
DOCTYPE HTML PUBLIC
"
-//w3c//dtd html 4.0 transitional//en
"
>
<
html
>
<
head
>
<
title
>
欢迎注册xxxx系统
</
title
>
<
link rel
=
"
stylesheet
"
type
=
"
text/css
"
href
=
"
<%=request.getContextPath()%>/scripts/style.css
"
>
<
script type
=
"
text/javascript
"
src
=
"
<%=request.getContextPath()%>/scripts/validation.js
"
></
script
>
</
head
>
<
body
>
<
br
><
br
>
<
div id
=
"
center
"
style
=
"
width:500;align:center
"
>
<
div
class
=
"
bai
"
>
<
div
class
=
"
bg
"
>
<
div
class
=
"
rg1
"
></
div
><
div
class
=
"
rg2
"
></
div
>
<
div
class
=
"
t1
"
>
欢迎注册xxxx系统
</
div
>
<
div
class
=
"
bc
"
>
<
div
class
=
"
bai
"
>
<
form method
=
'
post
'
action
=
'
register.htm
'
onSubmit
=
"
return Validator.Validate(this,3)
"
>
<
table width
=
500
align
=
"
center
"
border
=
"
1
"
>
<
tr
>
<
spring:bind path
=
"
command.*
"
>
<
font color
=
"
#FF0000
"
>
<
c:forEach items
=
"
${status.errorMessages}
"
var
=
"
error
"
>
错误:
<
c:
out
value
=
"
${error}
"
/><
br
>
</
c:forEach
>
</
font
>
</
spring:bind
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
><
font color
=
red
>
用户名称
*</
font
></
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.username
"
>

<
input type
=
"
text
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"
name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
LimitB
"
min
=
"
2
"
max
=
"
24
"
msg
=
"
用户名必须在2到24个字母之间
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
登录名必须大于等于2个字符
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
><
font color
=
red
>
真实姓名
*</
font
></
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.realname
"
>

<
input type
=
"
text
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"

name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
Chinese
"
msg
=
"
真实姓名必须为中文
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
这里请添入您真实的姓名
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
><
font color
=
red
>
密
&
nbsp;
&
nbsp;
&
nbsp;
&
nbsp;码
*</
font
></
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.password1
"
>

<
input type
=
"
password
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"

name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
LimitB
"
min
=
"
6
"
max
=
"
24
"
msg
=
"
密码必须在6到24个字母之间
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
密码必须大于等于6个字符
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
><
font color
=
red
>
确认密码
*</
font
></
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.password2
"
>

<
input type
=
"
password
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"

name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
Repeat
"
to
=
"
password1
"
msg
=
"
两次输入的密码不一致
"
type
=
"
password
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
确认输入您的密码
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
><
font color
=
red
>
性
&
nbsp;
&
nbsp;
&
nbsp;
&
nbsp;别
*</
font
></
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
select name
=
"
gender
"
>
<
c:
if
test
=
"
${command.gender!='女'}
"
>
<
option value
=
"
男
"
selected
>
男
</
option
>
<
option value
=
"
女
"
>
女
</
option
>
</
c:
if
>
<
c:
if
test
=
"
${command.gender=='女'}
"
>
<
option value
=
"
男
"
>
男
</
option
>
<
option value
=
"
女
"
selected
>
女
</
option
>
</
c:
if
>
</
select
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
请选择您的性别
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
><
font color
=
red
>
年
&
nbsp;
&
nbsp;
&
nbsp;
&
nbsp;龄
*</
font
></
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.age
"
>

<
input type
=
"
text
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"

name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
Range
"
min
=
"
16
"
max
=
"
60
"
msg
=
"
年龄必须在16~60之间
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
您的年龄信息
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
><
font color
=
red
>
联系电话
*</
font
></
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.phone
"
>

<
input type
=
"
text
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"

name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
LimitB
"
min
=
"
6
"
max
=
"
20
"
msg
=
"
联系电话须在6到20个字母之间
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
您的联系电话,最好是移动电话
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
>
地
&
nbsp;
&
nbsp;
&
nbsp;
&
nbsp;址
</
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.address
"
>

<
input type
=
"
text
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"

name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
LimitB
"
max
=
"
100
"
msg
=
"
地址信息必须在100个字母以内
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
您的联系地址,可不填
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
>
电子邮箱
</
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.email
"
>

<
input type
=
"
text
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"

name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
LimitB
"
max
=
"
50
"
msg
=
"
电子邮箱信息必须在50个字母以内
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
您的电子邮箱
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
>
提示问题
&
nbsp;
</
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.question
"
>

<
input type
=
"
text
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"

name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
LimitB
"
max
=
"
100
"
msg
=
"
提示问题必须在100个字母以内
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
提示问题将会帮您找回遗失的密码
</
td
>
</
tr
>
<
tr
>
<
td height
=
"
23
"
width
=
"
100
"
class
=
"
bb
"
>
问题答案
&
nbsp;
</
td
>
<
td height
=
"
23
"
width
=
"
200
"
class
=
"
data
"
>
<
spring:bind path
=
"
command.answer
"
>

<
input type
=
"
text
"
class
=
"
fullinputbox
"
value
=
"
<c:out value=
"
$
...
{status.value}
"
/>
"

name
=
"
<c:out value=
"
$
...
{status.expression}
"
/>
"
dataType
=
"
LimitB
"
max
=
"
50
"
msg
=
"
提示问题答案必须在50个字母以内
"
>
</
spring:bind
>
</
td
>
<
td height
=
"
23
"
width
=
"
250
"
class
=
"
bb
"
>
提示问题的答案
</
td
>
</
tr
>
<
tr
>
<
td
><
input type
=
'
submit
'
class
=
"
bnsrh
"
value
=
'
注册
'
></
td
>
<
td
><
input type
=
'
reset
'
class
=
"
bnsrh
"
value
=
'
重置
'
></
td
>
<
td
><
input type
=
"
button
"
class
=
"
bnsrh
"
onclick
=
"
history.back()
"
value
=
"
返回
"
></
td
>
</
tr
>
</
table
>
</
form
>
</
div
>
</
div
>
<
div
class
=
"
bai
"
><
div
class
=
"
rg3
"
></
div
><
div
class
=
"
rg4
"
></
div
></
div
>
</
div
>
</
div
>
</
div
>
<
br
>
<
SCRIPT language
=
JavaScript src
=
"
Copyright.js
"
>
</
SCRIPT
>
</
body
>
</
html
>
(页面显示的CSS代码与客户端验证的JS代码都已分离出来了)
以上 SQL, Java PO, Java VO, Validator, Form 各个属性之间一一对应的关系非常的明显。
可否考虑,通过配置文件
动态生成这些 PO,VO,Validator,Form呢?
xdoclet已经为此类自动化提供了很好的样例
好好考虑下
在表单数量比较多,而其关联度又比较低的表单情况下,此类代码的自动化肯定可以大大提高效率
这些代码可能不会做到100%的自动化生成,但,50%应该可以自动化生成吧?
think about it
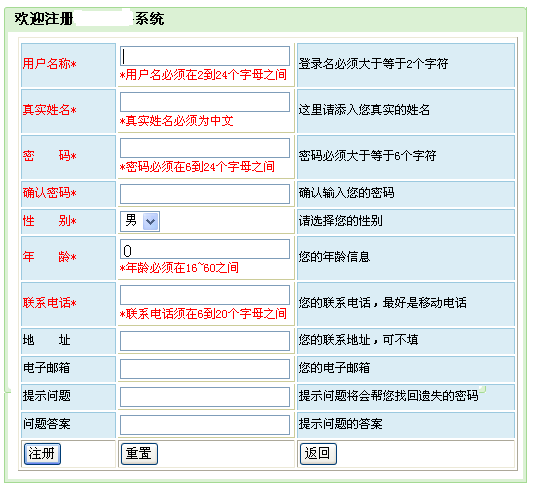