Each role has some models, in different model the role has different super skill. Such as Ryu, he has two model -- "Metsu Hadoken" and "Metsu Shoryuken". In "Metsu Hadoken" model, Ryu could beat Chun-Li easyly and in "Metsu Shoryuken" he could beat Ken.
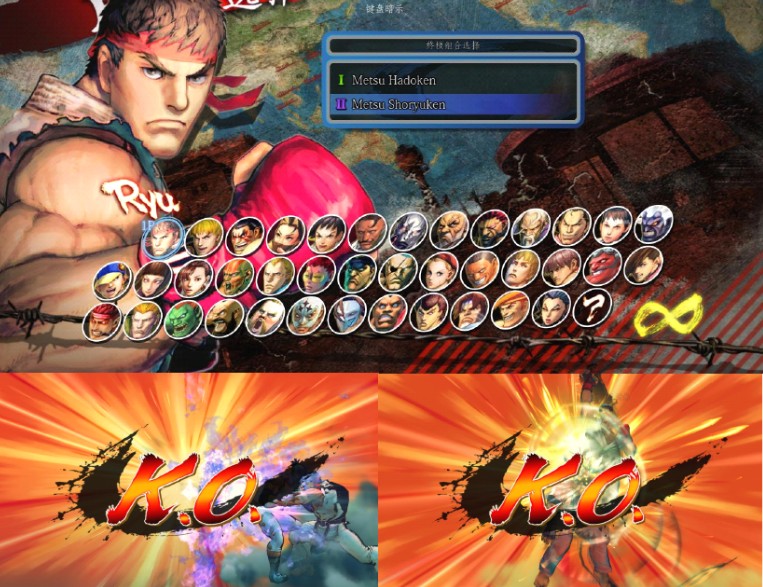
Giving the information of which role in which model could beat which role in which model. Your task is choosing minimum roles in certain model to beat other roles in any model.(each role could only be chosen once)
The first line of each case contains a integers N(2<=N<=25), indication the number of roles. (roles numbered from 0 to N - 1).
Then N blocks follow, each block contain the information of a role.
The first of each block contains a integer M(1<=M<=2), indication the number of model of this role.(models numbered from 0 to M - 1)
Then M lines follow, each line contain a number K(1<=K<=10), then K pairs integers(role id and model id) follow, indicating the current role in this model could beat that role in such model.
2 2 2 1 1 0 1 1 1 2 1 0 0 1 0 1 4 2 2 1 0 1 1 1 2 0 2 2 3 0 0 1 1 2 0 2 2 0 0 0 1 1 1 0 2 2 2 0 2 1 1 1 0
Case 1: 2 Case 2: 2HintIn the first sample, you must select all of roles, because one role couldn't beat the other role in any model. In the second sample, you can select role 0 with its model 0, and role 3 with its model 0, then role 1 and role 2 will to be defeated no matter which model they use.
//
街霸游戏中,有n种角色,每种角色有1~2种人物,比如说Ryu有两种人:Metsu Hadoke和Metsu Shoryuken。Ryu用Metsu Hadoke可以轻易打败Chun-Li,用Metsu Shoryuke可以打败Ken。从n种角色中选出哪些角色在那种人物上,可以击败其余的任何角色的任何人物。求选取的最少人物。
//
显然,行是代表选择,一共最多2*n行,分别表示人物和模式。列代表约束,显然,每个角色的每种人物必须至少被覆盖一次(重复覆盖),最多2*n列(等于行数),其次,每种角色只能选一次。增加n列,代表每种角色只能选一样,这n列必须满足每列不能多于2个1(精确覆盖)。这样,前面最多2*n列用重复覆盖,后面n列用精确覆盖。结束时只需判断前最多2*n列重复覆盖即可。
//
#include<iostream>
#include<cstdio>
#include<cstring>
#include<algorithm>
using namespace std;
//重复覆盖+精确覆盖 前n列重复覆盖,后m-n列精确覆盖
//共四处修改 注意
#define N 550
#define V 360000
int n,m;//n行 m列
int L[V],R[V];
int D[V],U[V];
int C[V];
int S[N],H[N];
int ak,size;//ak 最少多少行可以覆盖所有列(可重复)
void Link(int r,int c)
{
S[c]++;C[size]=c;
U[size]=U[c];D[U[c]]=size;
D[size]=c;U[c]=size;
if(H[r]==-1) H[r]=L[size]=R[size]=size;
else
{
L[size]=L[H[r]];R[L[H[r]]]=size;
R[size]=H[r];L[H[r]]=size;
}
size++;
}
//重复覆盖
void remove1(int c)
{
int i;
for(i=D[c];i!=c;i=D[i])
L[R[i]]=L[i],R[L[i]]=R[i];
}
void resume1(int c)
{
int i;
for(i=U[c];i!=c;i=U[i])
L[R[i]]=R[L[i]]=i;
}
int h()
{
int i,j,k,count=0;
bool visit[N];
memset(visit,0,sizeof(visit));
for(i=R[0];i&&i<=n;i=R[i])//注意 添加i<=n
{
if(visit[i]) continue;
count++;
visit[i]=1;
for(j=D[i];j!=i;j=D[j])
{
for(k=R[j];k!=j;k=R[k])
visit[C[k]]=1;
}
}
return count;
}
//精确覆盖
void remove(int c)//删除列
{
int i,j;
L[R[c]]=L[c];
R[L[c]]=R[c];
for(i=D[c];i!=c;i=D[i])
{
for(j=R[i];j!=i;j=R[j])
{
U[D[j]]=U[j],D[U[j]]=D[j];
S[C[j]]--;
}
}
}
void resume(int c)
{
int i,j;
for(i=U[c];i!=c;i=U[i])
{
for(j=L[i];j!=i;j=L[j])
{
U[D[j]]=j;D[U[j]]=j;
S[C[j]]++;
}
}
L[R[c]]=c;
R[L[c]]=c;
}
void Dance(int k)
{
int i,j,c,Min,ans;
ans=h();
if(k+ans>=ak) return;
if(!R[0]||R[0]>n)//注意 添加R[0]>n
{
if(k<ak) ak=k;
return;
}
for(Min=N,i=R[0];i&&i<=n;i=R[i])//注意 添加i<=n
if(S[i]<Min) Min=S[i],c=i;
for(i=D[c];i!=c;i=D[i])//注意 先重复 后精确
{
remove1(i);
for(j = R[i]; j != i; j = R[j])
if(C[j] <= n)
remove1(j);
for(j = R[i]; j != i; j = R[j])
if(C[j] > n)
remove(C[j]);
Dance(k+1);
for(j = L[i]; j != i; j = L[j])
if(C[j] > n)
resume(C[j]);
for(j = L[i]; j != i; j = L[j])
if(C[j] <= n)
resume1(j);
resume1(i);
}
return;
}
int tn;//role number
int model[30];//model number
int p[30][3];//role i model j对应的编号
int kill[30][3];//role i model j杀掉多少人
int s[30][3][30],t[30][3][30];//被role i model j杀掉的人的状态
int mat[N][N];
int main()
{
int ci,pl=1;scanf("%d",&ci);
while(ci--)
{
scanf("%d",&tn);
n=0;//行
for(int i=0;i<tn;i++)
{
scanf("%d",&model[i]);
for(int j=0;j<model[i];j++)
{
p[i][j]=++n;
scanf("%d",&kill[i][j]);
for(int k=0;k<kill[i][j];k++)
{
scanf("%d%d",&s[i][j][k],&t[i][j][k]);
}
}
}
m=n+tn;//列
memset(mat,0,sizeof(mat));
for(int i=0;i<tn;i++)
{
for(int j=0;j<model[i];j++)
{
int st=p[i][j];//重复覆盖
for(int k=0;k<model[i];k++)
{
int ed=p[i][k];//st杀掉自己
mat[st][ed]=1;
}
for(int k=0;k<kill[i][j];k++)
{
int ed=p[s[i][j][k]][t[i][j][k]];//st杀掉ed
mat[st][ed]=1;
}
mat[st][n+i+1]=1;//对应roll序号 精确覆盖
}
}
//DLX
for(int i=0;i<=m;i++)
{
S[i]=0;
D[i]=U[i]=i;
L[i+1]=i;R[i]=i+1;
}R[m]=0;
size=m+1;
memset(H,-1,sizeof(H));
for(int i=1;i<=n;i++)
{
for(int j=1;j<=m;j++)
{
if(mat[i][j]) Link(i,j);
}
}
ak=N;
Dance(0);
printf("Case %d: %d\n",pl++,ak);
}
return 0;
}