有n架飞机,每一架飞机有两个着陆时间,且相继着陆的两架飞机着陆时间必须相差t以上,现在要我们求出最大的t。
枚举t值,在根据2-SAT建图。
建图:
枚举间隔t;
如果 |a[i]-a[j]|<t 连 (i,j+n) (j,i+n)
如果|a[i]-b[j]|<t 连 (i,j) (j+n,i+n)
如果|b[i]-a[j]|<t 连 (i+n,j+n) (j,i)
如果|b[i]-b[j]|<t 连 (i+n,j) (j+n,i)
Time Limit: 9000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
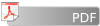
As you must have experienced, instead of landing immediately, an aircraft sometimes waits in a holding loop close to the runway. This holding mechanism is required by air traffic controllers to space apart aircraft as much as possible on the runway (while keeping delays low). It is formally defined as a ``holding pattern'' and is a predetermined maneuver designed to keep an aircraft within a specified airspace (see Figure 1 for an example).
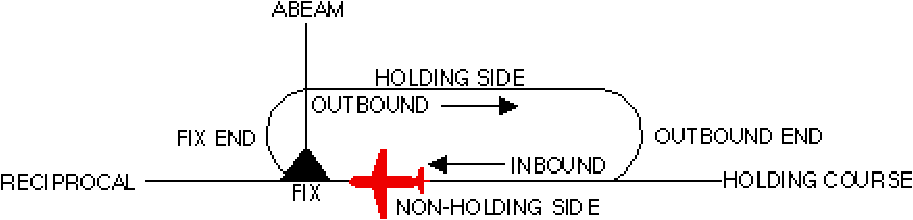
Jim Tarjan, an air-traffic controller, has asked his brother Robert to help him to improve the behavior of the airport.
Input
The input file, that contains all the relevant data, contains several test cases
Each test case is described in the following way. The first line contains the number n of aircraft ( 2n
2000). This line is followed by nlines. Each of these lines contains two integers, which represent the early landing time and the late landing time of an aircraft. Note that all times t are such that 0
t
107.
Output
For each input case, your program has to write a line that conttains the maximal security gap between consecutive landings.
Sample Input
10 44 156 153 182 48 109 160 201 55 186 54 207 55 165 17 58 132 160 87 197
Sample Output
10
Note: The input file corresponds to Table 1.
Robert's Hints
-
Optimization vs. Decision
- Robert advises you to work on the decision variant of the problem. It can then be stated as follows: Given an integer p, and an instance of the optimization problem, the question is to decide if there is a solution with security gap p or not. Note that, if you know how to solve the decision variant of an optimization problem, you can build a binary search algorithm to find the optimal solution. On decision
-
Robert believes that the decision variant of the problem can be
modeled as a very particular boolean satisfaction problem. Robert suggests to associate a boolean variable per aircraft stating whether the aircraft is early (variable takes value ``true'') or late (value ``false''). It should then be easy to see that for some aircraft to land at some time has consequences for the landing times of other aircraft. For instance in Table 1 and with a delay of 10, if aircraft
A1 lands early, then aircraft
A3 has to land late. And of course, if aircraft
A3 lands early, then aircraft
A1 has to land late. That is, aircraft
A1 and
A3 cannot both land early and formula
(A1
¬A3)
(A3
¬A1) must hold.
And now comes Robert's big insight: our problem has a solution, if and only if we have no contradiction. A contradiction being something like Ai ¬Ai.
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<string>
#include<cstring>
#include<queue>
using namespace std;
#define MAXN 60000
struct node
{
int to,next;
};
node edge[25000010];
int head[MAXN],en,n;
void add(int a,int b)
{
edge[en].to=b;
edge[en].next=head[a];
head[a]=en++;
}
int low[MAXN],dfn[MAXN];
int stack[MAXN],top,set[MAXN],col,num;
bool vis[MAXN],instack[MAXN];
void tarjan(int u)
{
vis[u]=1;
dfn[u]=low[u]=++num;
instack[u]=true;
stack[++top]=u;
for(int i=head[u];i!=-1;i=edge[i].next)
{
int v=edge[i].to;
if(!vis[v])
{
tarjan(v);
low[u]=min(low[u],low[v]);
}
else
if(instack[v])
low[u]=min(dfn[v],low[u]);
}
if (dfn[u]==low[u])
{
int j;
col++;
do
{
j=stack[top--];
instack[j]=false;
set[j]=col;
}
while (j!=u);
}
}
void solve()
{
int i;
top=col=num=0;
memset(instack,0,sizeof(instack));
memset(vis,0,sizeof(vis));
memset(set,-1,sizeof(set));
for (i=1;i<=2*n;i++)
if (!vis[i])
tarjan(i);
}
bool twosat()
{
solve();
for(int i=1;i<=n;i++)
if(set[i]==set[i+n]) return false;
return true;
}
int a[MAXN],b[MAXN];
void build(int len)
{
memset(head,-1,sizeof(head));
en=0;
for(int i=1;i<=n;i++)
{
for(int j=i+1;j<=n;j++)
{
if(abs(a[i]-a[j])<len)
{
add(i,j+n);
add(j,i+n);
}
if(abs(a[i]-b[j])<len)
{
add(i,j);
add(j+n,i+n);
}
if(abs(b[i]-a[j])<len)
{
add(i+n,j+n);
add(j,i);
}
if(abs(b[i]-b[j])<len)
{
add(i+n,j);
add(j+n,i);
}
}
}
}
int main()
{
while(~scanf("%d",&n))
{
int l=0,r=0,m;
for(int i=1;i<=n;i++)
{
scanf("%d%d",&a[i],&b[i]);
r=max(r,b[i]);
}
int ans;
while(l<r)
{
//二分的时候注意
m=l+(r-l+1)/2;
build(m);
if(twosat())
{
ans=m;
l=m;
}
else
r=m-1;
}
printf("%d\n",ans);
}
return 0;
}