Mobile phones have changed our lifestyle dramatically in the last decade. Mobile phones have a variety of protocols to connect with one another. One of the most popular networks for mobile phones is the GSM (Global System for Mobile Communication) network.
In a typical GSM network, a mobile phone connects with the nearest BTS (Base Transceiver Station). A BSC (Base Station Center) controls several BTSs. Several BSCs are controlled by one MSC (Mobile Services Switching Center), and this MSC maintains a connection with several other MSCs, a PSTN (Public Switched Telecom Network) and an ISDN (Integrated Services Digital Network).
This problem uses a simplified model of the conventional GSM network. Our simplified network is composed of up to fifty BTS towers. When in use, a mobile phone always connects to its nearest BTS tower. The area covered by a single BTS tower is called a cell. When an active mobile phone is in motion, as it crosses cell boundaries it must seamlessly switch from one BTS to another. Given the description of a map consisting of cities, roads and BTS towers, you must determine the minimum number of BTS switches required to go from one city to another.
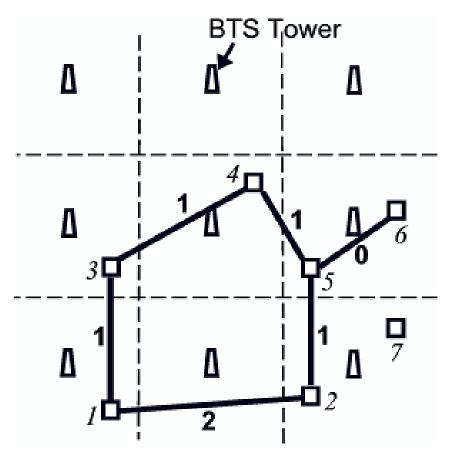
Each tower and each city location is to be considered as a single point in a two-dimensional Cartesian coordinate system. If there is a road between two cities, assume that the road is a straight line segment connecting these two cities. For example, in the figure, traveling on the road from city 1 to city 2 will cross two cell boundaries and thus requires two switches. Traveling from city 2 to city 5 crosses one cell boundary and traveling from city 5 to city 6 requires no switch. Traveling this route from city 1 to city 6 requires three total switches. Note than any other path from city 1 to city 6 requires more than three switches. If there is more than one possible way to get from one city to another, your program must find the optimal route.
Input
The input file contains several test cases. The first line of each test case contains four integers: B(1B
50), the number of BTS towers; C(1
C
50), the number of cities; R(0
R
250), the number of roads; andQ(1
Q
10), the number of queries. Each of the next B lines contains two floating-point numbers x and y, the Cartesian coordinates of a BTS tower. Each of the next C lines contains two floating-point numbers xi,yi that indicate the Cartesian coordinates of the ith city (1
i
C). Each of the next R lines contains two integers m and n (1
m, n
C), which indicate that there is a road between the m-th and the n-th city. Each of the next Q lines contains two integers s and d (1
s, d
C), the source and destination cities.
No coordinate will have an absolute value greater than 1000. No two towers will be at the same location. No two cities will be at the same location, and no city will lie on a cell boundary. No road will be coincident with a cell boundary, nor contain a point lying on the boundary of three or more cells.
The input will end with a line containing four zeros.
Output
For each input set, you should produce Q + 1 lines of output, as shown below. The first line should contain the number of the test case. Q lines should follow, one for each query, each containing an integer indicating the minimum number of switches required to go from city s to city d. If it is not possible to go from city s to city d, print the line `Impossible' instead.
Sample Input
9 7 6 2 5 5 15 5 25 5 5 15 15 15 25 15 5 25 15 25 25 25 8 2 22 3 8 12 18 18 22 12 28 16 28 8 1 2 1 3 2 5 3 4 4 5 5 6 1 6 1 7 0 0 0 0
Samle Output
Case 1: 3 Impossible
#include<stdio.h>
#include<math.h>
#include<string.h>
#include<stdlib.h>
struct coor
{
double x,y;
};
const int MaxB=50;
const int MaxC=50;
const int MaxS=50*50;
int B,C,R,Q,i,j,k,cases;
coor BTS[MaxB],city[MaxC];
int S[MaxC][MaxC];
double dis(double x1,double y1,double x2,double y2)
{
return sqrt((x1-x2)*(x1-x2) + (y1-y2)*(y1-y2));
}
int area(double x,double y)
{
int i,k;
k=0;
double min,tmp;
min=dis(x,y,BTS[0].x,BTS[0].y);
for(i=1;i<B;i++)
{
tmp=dis(x,y,BTS[i].x,BTS[i].y);
if(tmp<min)
{
min=tmp;
k=i;
}
}
return k;
}
int get_switch(double x1,double y1,double x2,double y2)
{
if(area(x1,y1)==area(x2,y2))
return 0;
if(dis(x1,y1,x2,y2)<1e-6)
return 1;
return get_switch(x1,y1,(x1+x2)/2,(y1+y2)/2)+get_switch((x1+x2)/2,(y1+y2)/2,x2,y2);
}
bool init()
{
scanf("%d %d %d %d",&B,&C,&R,&Q);
if(B==0&&C==0&&R==0&&Q==0)
return false;
for(i=0;i<B;i++)
scanf("%lf %lf",&BTS[i].x,&BTS[i].y);
for(i=0;i<C;i++)
scanf("%lf %lf",&city[i].x,&city[i].y);
memset(S,0,sizeof(S));
for(i=0;i<R;i++)
{
scanf("%d %d",&j,&k);
j--;k--;
S[j][k]=S[k][j]=1;
}
for(i=0;i<C;i++)
for(j=i+1;j<C;j++)
{
if(S[i][j]==0)
S[i][j]=MaxS;
else
S[i][j]=get_switch(city[i].x,city[i].y,city[j].x,city[j].y);
S[j][i]=S[i][j];
}
for(i=0;i<C;i++)
S[i][i]=0;
return true;
}
int main()
{
cases=0;
while(init())
{
printf("Case %d:\n",++cases);
for(k=0;k<C;k++)
for(i=0;i<C;i++)
for(j=0;j<C;j++)
if(S[i][j]>S[i][k]+S[k][j])
S[i][j]=S[i][k]+S[k][j];
for(i=0;i<Q;i++)
{
scanf("%d %d",&j,&k);
j--;k--;
if(S[j][k]>=MaxS)
printf("Impossible\n");
else
printf("%d\n",S[j][k]);
}
}
return 0;
}