1:注释
1:单行注释:
//+内容
2:多行注释
/*
*内容
* */
3,文档注释
/**
*内容
*
*
*/
2:标识符和关键字
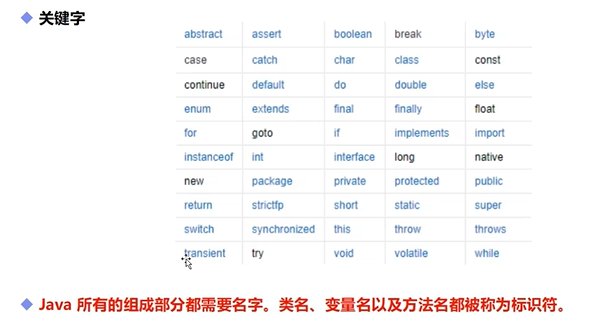
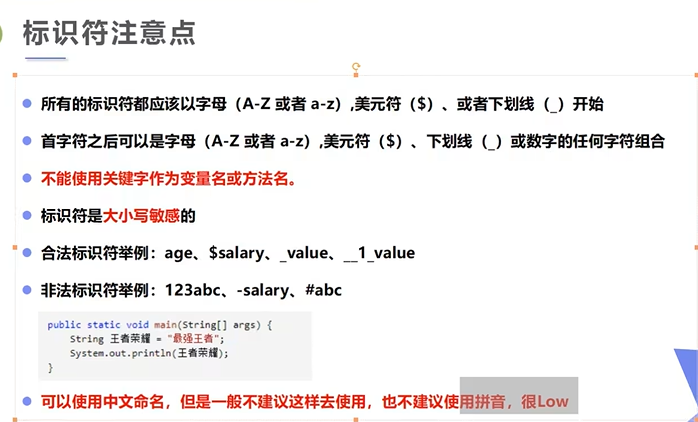
3:数据类型(强数据类型-所有变量必须定义后才能使用)
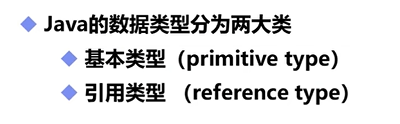
八大基本数据类型:(整数)byte、short、int、long
(小数:浮点型)float、double
(字符)char (字符串)String
(布尔类型)boolean--true、false
注意:float类型必须在值后面加f,long类型加l
float number1 = 0.4f;
引用数据类型:类、接口、数组
扩展:(面试题)
1:整数扩展(进制问题): 二进制0b、 十进制、 八进制0、 十六进制0x
int i1 = 10;
int i2 = 0b10;
int i3 = 0x10;
2:浮点型扩展 银行业务(钱)怎么表示?----使用数学工具类BigDecimal
应完全避免使用浮点数进行比较--有限、离散、舍入误差、大约、接近但不等于
3:转义字符:\t、\n.....
4:类型转化
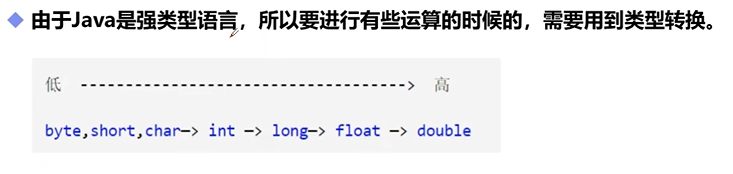
注意:
1:强制类型转化-----高到低。
2:自动类型转化-----低到高。
3:不能对布尔值进行转化。
4:高容量转化到低容量时可能存在"内存溢出"或者"精度不准确"的问题。
5:java变量
定义:Java变量是程序中最基本的存储单元、包括变量名、变量类型和作用域
变量作用域:类变量、实例变量、局部变量
常量:初始化后不能改变值
public class dm3 {
//类变量
static int allClicks = 0;
//实例变量
String str1 = "实例变量";
//常量
final i = 1;
public static void main(String[] args) {
//局部变量
int num =1;
//直接使用类变量
System.out.println(allClicks);
//通过对象实例使用实例变量
dm3 obj1 = new dm3();
System.out.println(obj1.str1);
}
}
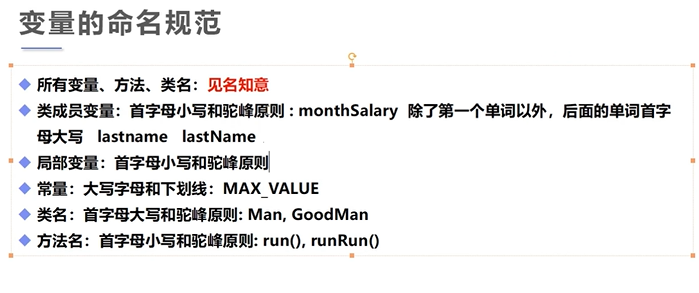
6:运算符
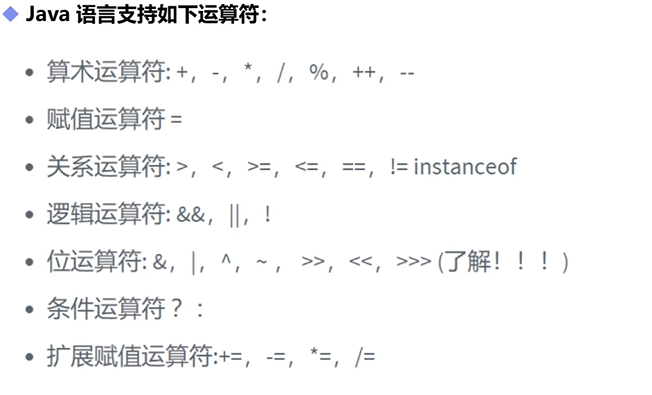
注意:1: a++ ---先运算再+1
2: ++a ---先+1再运输
3:幂运算 ---Math.pow(2,3)=8
4:位运算符:
位运算符
public class dm {
public static void main(String[] args) {
/**
*
* A = 0011 1100
* B = 0000 1101
* -------------
* A&B=0000 1100
* A|B=0011 1101
* A^B=0011 0001-----同0异1
* ~A =1100 0011-----取反
*
*
* 如何计算2*8????
* << *2
* >> /2
*/
System.out.println(2<<3);
System.out.println(4>>1);
}
}
7:用户交互Scanner
方法1:hasNext()与next()
Scanner scanner1 =new Scanner(System.in);
//方法1:hasNext() next()
if (scanner1.hasNext()){
System.out.println("输入为:"+scanner1.next());
}
scanner1.close();
注意:next()方法在输入时不能带有空格的字符串。
next()方法对输入有效字符之前遇到的空白会将其自动去掉
next()方法是以空格为结束符
方法2:hasNextLine()与nextLine()----以回车键为结束符
System.out.println("请输入:");
//方法2:hasNextLine() nextLine()
if (scanner1.hasNextLine()){
String str1 = scanner1.nextLine();
System.out.println("输入为:"+str1);
}
scanner1.close();
方法3:hasNextInt()与nextInt()
......
//计算输入数字的和以及平均值,当输入的为字母时停止
public class dm3 {
public static void main(String[] args) {
Scanner sca1 = new Scanner(System.in);
double sum = 0;
int num = 0;
while (sca1.hasNextDouble()){
double i =sca1.nextDouble();
sum += i;
num++;
}
System.out.println("sum="+sum+" ava="+(sum/num));
sca1.close();
}
}
8:if语句
System.out.println("请输入分数:");
Scanner sca = new Scanner(System.in);
int i = sca.nextInt();
if (i == 100){
System.out.println("恭喜你,满分");
} else if (i<100 && i>=90) {
System.out.println("优秀");
} else if (i<90 && i>=70) {
System.out.println("中");
} else if (i<70 && i>=60) {
System.out.println("及格");
} else if (i<60 && i>=0) {
System.out.println("不及格");
}else {
System.out.println("输入不合法");
}
9:switch -- case: -- break
//switch -- case: -- break
String str1 = "你好";
switch (str1){
case ("hello"):
System.out.println("hello");
break;
case ("你好"):
System.out.println("你好");
break;
default:
System.out.println("弄啥!");
}
注意:case 标签必须是字符串常量或字面量
10:循环结构
while 循环-最基本的循环,结构为:
while( 布尔表达式 ) {
//循环内容
//先判断,再循环
}
do...while 循环-至少会执行一次
do{
//循环内容
//先循环,再判断
}while ( 布尔表达式 )
for循环-最有效、灵活的循环结构。结构为:
for(初始值;布尔表达式;更新){
//代码语句
}
增强for循环-结构为:
for(声明语句 : 表达式){
//代码语句
}
int[] array1 = {1,2,3,4,5};
for (int i:array1){
System.out.print(i+"\t");
}
练习1:计算0-100之间的奇数和偶数的和。
练习2:用while和for循环输入1-1000之间能被5整除的数,每行输出3个
练习3:打印九九乘法表
public class dm6 {
public static void main(String[] args) {
int sum1 = 0;
int sum2 = 0;
for (int i = 0; i<=100 ; i++){
if (i % 2 == 0){
sum1+=i;
}else {
sum2+=i;
}
}
System.out.println("奇数和="+sum2+" 偶数和="+sum1);
}
}
public class dm7 {
public static void main(String[] args) {
int j = 0;
int i = 1;
System.out.println("----------for-----------");
for (i=1;i<=1000;i++){
if (i%5==0){
System.out.print(i+"\t");
j++;
if (j%3==0){
System.out.println();
j=0;
}
}
}
System.out.println("----------while-----------");
i = 1;
j = 0;
while(i<=1000){
if (i%5==0){
System.out.print(i+"\t");
j++;
if (j%3==0){
System.out.println();
j=0;
}
}
i++;
}
}
}
public class dm8 {
public static void main(String[] args) {
//打印九九乘法表
System.out.println("-------------for----------------");
for(int i=1;i<10;i++){
for (int j=1;j<=i;j++){
System.out.print(j+"x"+i+"="+(j*i)+"\t");
}
System.out.println();
}
System.out.println("-------------while----------------");
int i =1;
while (i<10){
int j =1;
while (j<=i){
System.out.print(j+"x"+i+"="+(j*i)+"\t");
j++;
}
System.out.println();
i++;
}
}
}
11:Java方法
定义:Java方法是语句的集合,它们在一起执行一个功能,是解决一类问题的步骤的有序组合。
方法设计原则:保持方法的原子性(就是一个方法只完成一个功能,这样利于我们后期的扩展)
方法包含一个方法头和一个方法体。下面是一个方法的所有部分:
修饰符:可选,定义方法的访问类型。如:public,static
返回值类型:方法可能会有返回值。returnValueType是方法返回值的数据类型,但没有返回值。returnValueType的关键字是void。
方法名:方法的实际名称。
参数类型:
-形式参数:在方法被调用时用于接收外界输入的数据。
-实参:调用方法时实际传给方法的数据。
方法体:包含具体的语句,定义该方法的功能。
public class dm1 {
public static void main(String[] args) {
System.out.println(add(1,2));
}
//类变量---加static
//两数相加的方法
public static int add(int a,int b){
return a+b;
}
}
方法的重载
定义:就是在一个类中,有相同的函数名称,但形参不同的函数。
方法重载的规则:
方法名称必须相同。
参数列表必须不同(个数不同、或类型不同、参数排列顺序不同)。
public class dm2 {
public static void main(String[] args) {
System.out.println(add(1,2));
System.out.println(add(1,2,3));
}
//方法重载
public static int add(int a,int b){
return a+b;
}
//方法名相同,但参数个数不同
public static int add(int a,int b,int c){
return a+b+c;
}
}
可变参数
在方法声明中,在指定参数类型后加一个省略号(...)
一个方法只能指定一个可变参数,它必须是方法的最后一个参数。任何普通的参数必须在它之前声明。
public class dm3 {
public static void main(String[] args) {
double result = out_max(1,2,3,4,5,7,12,1,34);
System.out.println(result);
}
//寻找最大值
public static double out_max(int n1,int... n2){
double max = n1;
for (int i = 0;i<n2.length;i++){
if (max<n2[i]){
max = n2[i];
}
}
return max;
}
}
递归--A方法调用A方法(自己调用自己本身)
为什么要用递归:可以把一个大型复杂的问题层层转化为一个与原问题相似的规模较小的问题来求解。
递归结构包括两个部分:
递归头:什么时候不调用自身方法。若没有头,将陷入死循环。
递归体:什么时候需要调用自身方法。
public class dm5 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入:");
int i = scanner.nextInt();
System.out.println(i+"!="+multiply(i));
}
//计算阶乘
public static int multiply(int i){
if (i == 1){
return 1;
}else {
return i*multiply(i-1);
}
}
}
12:数组
数组是相同数据类型的有序集合。若干个数据按照一定的先后次序排列组合。
其中,每一个数据称作一个数组元素,每个数组元素可以通过下标来访问。
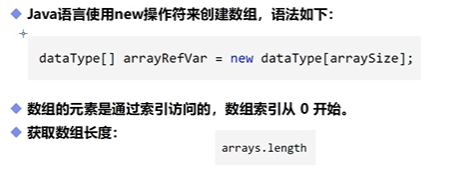
特点:
长度是确定的。数组一旦被创建,它的大小就是不可改变的。
其元素必须是相同类型,不允许出现混合类型。
数组中的元素可以是任何类型,包括基本类型和引用类型。
数组对象本身是在堆中的。
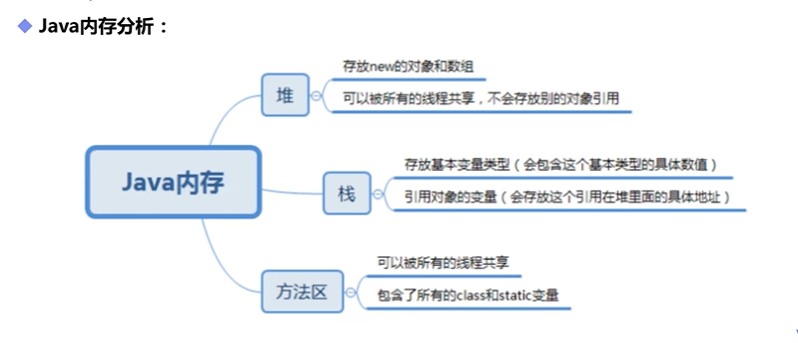
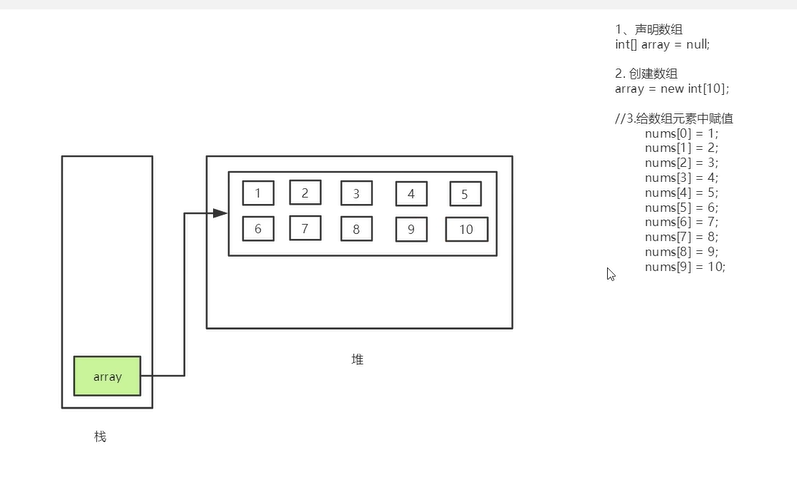
初始化:
public class dm6 {
public static void main(String[] args) {
//静态初始化
int[] arr1 = {1,2,3,4,5,6};
//动态初始化--默认值为0
int[] arr2 = new int[10];
arr2[0] = 1;
arr2[1] = 2;
}
}
数组的使用-遍历、数组作方法入参、数组作返回值
public class dm7 {
public static void main(String[] args) {
int[] arr1 ={1,2,3,4,5};
//遍历输出
for (int i=0;i<arr1.length;i++){
System.out.print(arr1[i]+"\t");
}
System.out.println();
out(arr1);
System.out.println();
arr1 = reverse(arr1);
out(arr1);
}
//遍历输出
public static void out(int[] arr){
for (int i : arr){
System.out.print(i+"\t");
}
}
//反转数组
public static int[] reverse(int[] arr){
int[] arr2 = new int[arr.length];
for (int i=arr.length-1,j=0;i>=0;i--,j++){
arr2[i]=arr[j];
}
return arr2;
}
}
二维数组:
public class dm1 {
public static void main(String[] args) {
int [][] arr1 = {{1,2},{3,4},{5,6}};
for (int i=0;i<arr1.length;i++){
for (int j=0;j<arr1[i].length;j++){
System.out.print(arr1[i][j]+"\t");
}
}
}
}
Arrays类讲解:
public class dm2 {
public static void main(String[] args) {
int [] arr1 = {1,2,43,3,7,8,54,78};
System.out.println(arr1);
System.out.println(Arrays.toString(arr1));
Arrays.sort(arr1);
System.out.println(Arrays.toString(arr1));
Arrays.fill(arr1,2,4,0);
System.out.println(Arrays.toString(arr1));
}
}
冒泡算法:
public class dm4 {
public static void main(String[] args) {
int [] a={1,43,5,2,9,7,34,11};
System.out.println(Arrays.toString(a));
a = sort(a);
System.out.println(Arrays.toString(a));
}
public static int[] sort(int[] a){
for (int i =0;i<a.length;i++){
boolean flag = false;
for (int j=0;j<a.length-1-i;j++){
if (a[j]<a[j+1]){
int m =a[j];
a[j] = a[j+1];
a[j+1] = m;
flag = true;
}
}
if (flag == false){
return a;
}
}
return a;
}
}
稀疏矩阵:
当一个数组大部分元素为0.或者为同一值的数组时,可以使用稀疏数组来保存该数组。
处理方式:记录数组一共有几行几列,有多少个不同值。把具有不同值元素的行列及值记录在一个小规模的数组中,从而缩小程序的规模。
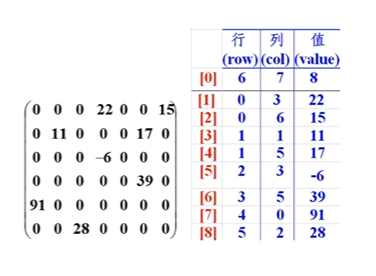
13:面向对象(Object-Oriented Programming,OOP)
本质:以类的方式组织代码,以对象的组织封装数据。
三大特征:封装
继承
多态

类与对象的关系
类是一种抽象的数据类型,它是对某一类事物整体描述/定义,但是并不能代表一个具体的事物。
对象是抽象概念的具体实例
创建与初始化对象
使用new关键字创建对象
类中的构造器也称构造方法,是在进行创建对象时自动调用的。并且构造器有以下两个特点:
必须和类的名字相同
必须没有返回类型,也不能写void
构造器特点:1. new本质在调用构造方法。2.初始化对象的值。
生成构造器快捷:alt+insert
14:封装(数据的隐藏)
程序设计要追求高内聚,低耦合。高内聚就是类的内部数据操作细节自己完成,不允许外部干涉;低耦合:仅暴露少量方法给外部使用。
属性私有,get/set
封装的作用:1:提高程序的安全性,保护数据
2:隐藏代码的实现细节
3:统一接口。
4:系统可维护性增加了。
public class pet {
//属性私有
private String name;
private String species;
public pet() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSpecies() {
return species;
}
public void setSpecies(String species) {
this.species = species;
}
}
public class dm6 {
public static void main(String[] args) {
pet pet1 = new pet();
pet1.setName("乐乐");
pet1.setSpecies("狗");
System.out.println(pet1.getName()+" "+pet1.getSpecies());
}
}
15:继承
继承是类与类之间的一种关系。除此之外,类和类之间的关系还有依赖、组合、聚集等。
继承关系的两个类,一个为子类(派生类),一个为父类(基类)。子类继承父类,使用关键字extends来表示。
注意:JAVA中只有单继承,没有多继承。
super
super注意点:
1:super调用父类的构造方法,必须在构造方法的第一个
2:super只能出现在子类的方法或者构造方法中
3:super与this的区别-
代表的对象不同:
this:本身调用者的这个对象。
super:代表父类对象的应用。
构造方法不同:
this();本类的构造
super();父类的构造
使用前提:
this:没有继承也可以使用
super:只能在继承条件下才可以使用
方法重写:需要有继承关系,子类重写父类的方法.方法体不同!
方法名必须相同
参数列表必须相同
修饰符:范围可以扩大但不能缩小: public > protected >default >private
为什么要重写:父类的功能,子类不一定需要,或者不一定满足。
public class person {
String province;
public person() {
System.out.println("person=>create");
}
public void out(){
System.out.println("person say out");
}
}
public class student extends person{
String name;
public student() {
//1:super调用父类的构造方法,必须在构造方法的第一个
super();
System.out.println("Student=>create");
}
public void out(){
System.out.println("student say out");
}
}
public class dm1 {
public static void main(String[] args) {
student stu1 = new student();
stu1.out();
//student say out
}
}
16:动态-即同一方法可以根据对象的不同而采用多种不同的行为方式。
多态存在条件:
有继承的关系
子类重写父类方法
父类引用指向子类对象
注意:多态是方法的多态,属性没有多态性。
public class dm2 {
public static void main(String[] args) {
student student1 = new student();
//person类父类型,可以指向子类,但是不能调用子类独有的方法
person student2 = new student();
Object student3 = new student();
//对象执行哪些方法,主要看对象左边的类型,和右边关系不大。
student1.out();
student2.out();
}
}
instanceof和类型转化
public class student extends person{
String name;
public student() {
//1:super调用父类的构造方法,必须在构造方法的第一个
super();
}
public void out(){
System.out.println("student say out");
}
public void go(){
System.out.println("student say go");
}
public class dm3 {
public static void main(String[] args) {
Object stu1 = new student();
//instanceof:判断是否存在关系
//Object > person > student
System.out.println(stu1 instanceof person);//true
System.out.println(stu1 instanceof student);//true
System.out.println(stu1 instanceof String);//false
person person1 = new student();
System.out.println(person1 instanceof student);//true
System.out.println(person1 instanceof Object);//true
// System.out.println(person1 instanceof String);编译报错
//类型转化
//将父类强转成子类
person person2 = new student();
((student)person2).go();
}
}
补充:static关键字详解
public class teacher {
//匿名代码块
{
System.out.println("匿名代码块");
}
//静态代码块只在第一个对象创建是会执行
static {
System.out.println("静态代码块");
}
public teacher(){
System.out.println("构造方法");
}
public static void main(String[] args) {
teacher teacher1 = new teacher();
System.out.println("===================");
teacher teacher2 = new teacher();
}
}
输出:
静态代码块
匿名代码块
构造方法
===================
匿名代码块
构造方法
public class dm4 {
String str1;
static String str2;
public void out1(){
//可以直接调用static方法
out2();
System.out.println("no static");
}
public static void out2(){
System.out.println("static");
}
public static void main(String[] args) {
//静态属性通过 类名+静态属性名 直接使用
dm4.out2();
}
}
17:抽象类
abstract修饰符可以用来修饰方法和修饰类。
抽象类中可以没有抽象方法,但有抽象方法的类一定要声明为抽象类。
抽象类,不能使用new关键字来创建对象,它是用来让子类继承的。
抽象方法,只有方法的声明,没有方法的实现,它是用来让子类实现的。
子类继承抽象类,那么就必须实现抽象类没有实现的抽象方法,否则该子类也要声明为抽象类。
18:接口
作用:
约束。
在接口中定义一些方法,在不同的类实现。
接口中的方法属性都为 public abstract。
接口中的属性都为 public static final。
接口不能被实例化,接口中没有构造方法。
implements可以实现多个接口。
必须要重写接口中的方法。
public interface dm1 {
public void add(String name);
void delete(String name);
void revise(String name);
void search(String name);
}
public interface dm3 {
}
public class dm2 implements dm1,dm3{
@Override
public void add(String name) {
}
@Override
public void delete(String name) {
}
@Override
public void revise(String name) {
}
@Override
public void search(String name) {
}
}
19:异常
Error和Exception的区别:Error通常是灾难性的致命的错误,是程序无法控制和处理的,当出现这些异常时,Java虚拟机(JVM)一般会选择终止线程;Exception通常情况下是可以被程序处理的,并且在程序中应该尽可能的去处理这些异常。
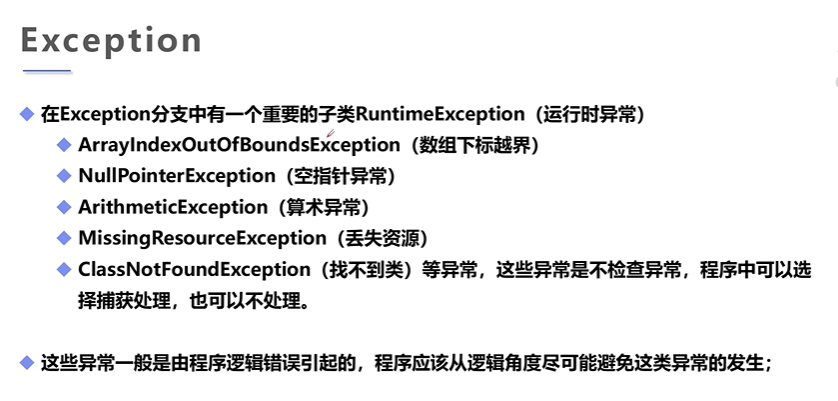
异常分类:
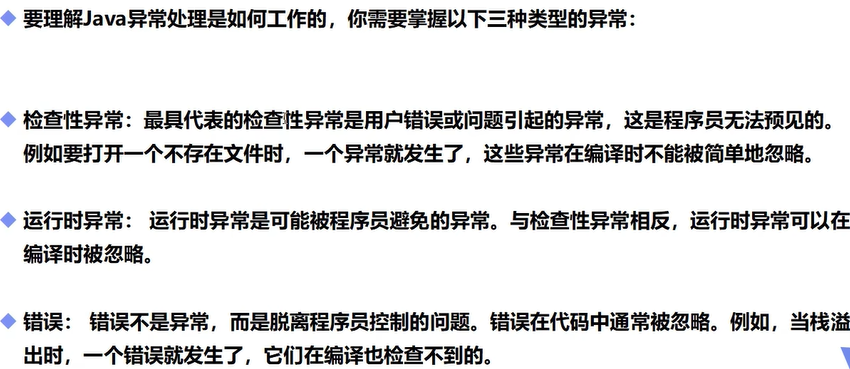
异常处理机制
异常处理的五个关键字:try、catch、finally、throw、throws
快捷键:Ctrl + Alt + T
捕获异常:Exception in thread "main" java.lang.ArithmeticException: / by zero
public class dm4 {
public static void main(String[] args) {
int a=1;
int b=0;
try {//监控区域
System.out.println(a/b);
}catch (ArithmeticException e){//catch(想要捕获的异常类型)
System.out.println("捕获异常");
}finally {//finally始终会执行
System.out.println("finally");
}
}
}
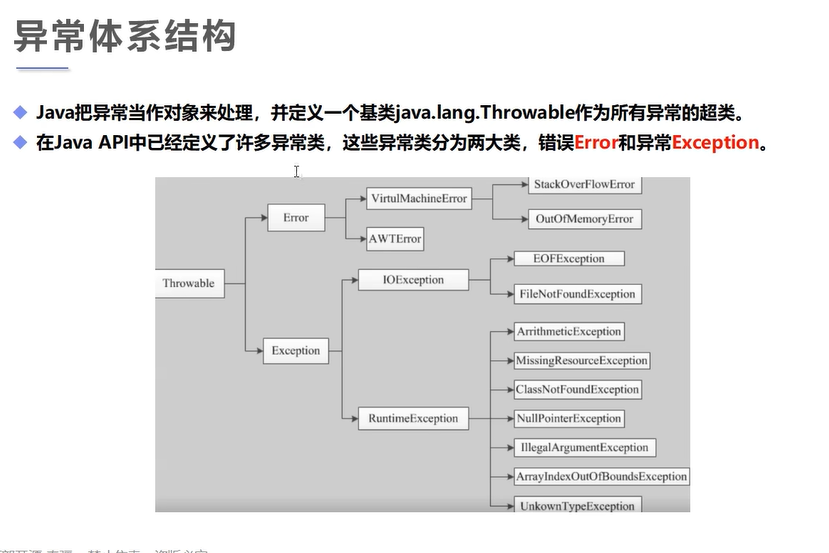
throw的使用:
public class dm5 {
public static void main(String[] args) {
int i=1;
int b=0;
try {
if (b==0){
throw new ArithmeticException();//抛出异常
}
}catch (Throwable throwable){
}
}
}
throws的使用:
public class dm1 {
public static void main(String[] args) {
dm1 dm = new dm1();
try {
dm.fun1();
} catch (ArithmeticException e) {
System.out.println(e);
}
}
//假设这个方法中,处理不了这个异常。在方法上抛出异常
public void fun1() throws ArithmeticException{
int a = 1;
int b = 0;
if (b == 0) {
throw new ArithmeticException();//主动抛出异常,一般在方法中声明
}
}
}
自定义异常
使用Java内置的异常类可以描述在编程时出现的大部分异常情况。除此之外,用户还可以自定义异常。用户自定义异常类,只需要继承Exception类即可。
自定义异常类步骤:
创建自定义异常类。
在方法中通过throw关键字抛出异常。
如果在当前抛出异常的方法中处理异常,可以使用try-catch语句捕获并处理;否则在方法的声明处通过throws关键字指明要抛出给方法调用者异常,继续进行下一步操作。
在出现异常方法的调用者中捕获并处理异常。
public class MyException extends Exception{
private int message;
public MyException(int message) {
this.message = message;
}
@Override
public String toString() {
return "MyException{" +
"message=" + message +
'}';
}
}
public class dm2 {
public static void main(String[] args) {
try {
new dm2().fun1(10);
} catch (MyException e) {
System.out.println(e);
}
}
public void fun1(int a) throws MyException{
throw new MyException(a);
}
}
处理异常在实际应用中的经验总结
处理运行异常时,采用逻辑去合理规避同时辅助try-catch处理
在多重catch块后面,可以加一个catch(Exception)来处理可能会被遗漏的异常
对于不确定的代码,也可以加上try-catch,处理潜在的异常
尽量去处理异常,切忌只是简单地打印输出
尽量使用finally语句块去释放占用的资源