导入jar包
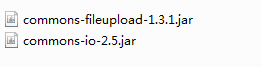
配置xml
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="defaultEncoding" value="UTF-8" />
<property name="maxUploadSize" value="5120000" />
</bean>
上传文件
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="fileupload" method="post" enctype="multipart/form-data">
<input type="file" name="file" />
<input type="submit" value="上传" />
<br/>
<c:forEach items="${images}" var="i">
<div><img src="${i}"></div>
</c:forEach>
</form>
</body>
</html>
@RequestMapping(value = "/fileupload",method = RequestMethod.POST)
public String uploads(MultipartFile file,ServletRequest request) throws IllegalStateException, IOException {
String uploadaddress = request.getServletContext().getRealPath("upload");
String filename = file.getOriginalFilename();
String newfilname = filename.substring(filename.lastIndexOf("."));
String newfileName = UUID.randomUUID() + newfilname;
File savepath = new File(uploadaddress + "/" + newfileName);
request.setAttribute("message","upload/" + newfileName);
file.transferTo(savepath);
return "fileupload";
}
上传多个文件
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="fileupload" method="post" enctype="multipart/form-data">
<input type="file" name="file" />
<input type="file" name="file" />
<input type="file" name="file" />
<input type="submit" value="上传" />
<br/>
<c:forEach items="${images}" var="i">
<div><img src="${i}"></div>
</c:forEach>
</form>
</body>
</html>
public String fileadd(MultipartFile file,ServletRequest request) throws IllegalStateException, IOException {
String uploadaddress = request.getServletContext().getRealPath("upload");
String filename = file.getOriginalFilename();
String newfilname = filename.substring(filename.lastIndexOf("."));
String newfileName = UUID.randomUUID() + newfilname;
File savepath = new File(uploadaddress + "/" + newfileName);
file.transferTo(savepath);
String name="upload/"+newfileName;
return name;
}
@RequestMapping(value = "/fileupload",method = RequestMethod.POST)
public String uploads(@RequestParam("file")MultipartFile[] file,ServletRequest request) throws IllegalStateException, IOException {
List<String> images = new ArrayList<String>();
for (int i = 0; i < file.length; i++) {
images.add(fileadd(file[i],request));
}
request.setAttribute("images", images);
return "fileupload";
}
文件下载
@RequestMapping("/downFile")
public ResponseEntity<byte[]> testdownFile(HttpSession session)throws IOException {
ServletContext servletContext = session.getServletContext();
InputStream in = servletContext.getResourceAsStream("upload/1.gif");
byte[] bytes = FileCopyUtils.copyToByteArray(in);
HttpHeaders header = new HttpHeaders();
header.add("Content-Disposition", "attachment;filename=1.gif");
ResponseEntity<byte[]> entity = new ResponseEntity<byte[]>(bytes, header, HttpStatus.OK);
return entity;
}