PAT (Advanced Level) Practice 1127 ZigZagging on a Tree (30 分) 凌宸1642
题目描述:
Suppose that all the keys in a binary tree are distinct positive integers. A unique binary tree can be determined by a given pair of postorder and inorder traversal sequences. And it is a simple standard routine to print the numbers in level-order. However, if you think the problem is too simple, then you are too naive. This time you are supposed to print the numbers in “zigzagging order” – that is, starting from the root, print the numbers level-by-level, alternating between left to right and right to left. For example, for the following tree you must output: 1 11 5 8 17 12 20 15.
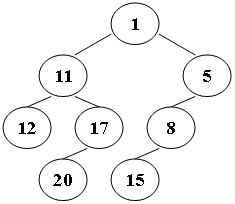
译:假设二叉树中的所有键都是不同的正整数。 唯一的二叉树可以由给定的一对后序和中序遍历序列确定。 它是按级别顺序打印数字的简单标准例程。 但是,如果你认为问题太简单,那你就太天真了。 这次你应该以“锯齿形顺序”打印数字——也就是说,从根开始,逐级打印数字,从左到右和从右到左交替。 例如,对于以下树,您必须输出:1 11 5 8 17 12 20 15。
Input Specification (输入说明):
Each input file contains one test case. For each case, the first line gives a positive integer N (≤30), the total number of nodes in the binary tree. The second line gives the inorder sequence and the third line gives the postorder sequence. All the numbers in a line are separated by a space.
译:每个输入文件包含一个测试用例。 对于每种情况,第一行给出一个正整数 N(≤30),即二叉树中节点的总数。 第二行给出中序序列,第三行给出后序序列。 一行中的所有数字都用空格分隔 。
output Specification (输出说明):
For each test case, print the zigzagging sequence of the tree in a line. All the numbers in a line must be separated by exactly one space, and there must be no extra space at the end of the line.
译:对于每个测试用例,在一行中打印树的锯齿形序列。 一行中的所有数字必须正好用一个空格隔开,行尾不得有多余的空格。
Sample Input (样例输入):
8
12 11 20 17 1 15 8 5
12 20 17 11 15 8 5 1
Sample Output (样例输出):
1 11 5 8 17 12 20 15
The Idea:
- 中序+ 后序 重建二叉树。
- 然后层序遍历的一种特殊形式。
The Codes:
#include<bits/stdc++.h>
using namespace std ;
const int maxn = 33 ;
struct node{
int val ;
node* lchild ;
node* rchild ;
};
int in[maxn] , post[maxn] , n , ans[maxn];
// 中序+ 后序 重建二叉树
node* create(int postL , int postR , int inL , int inR){
if(postL > postR) return NULL ;
node* root = new node() ;
root->val = post[postR] ;
int k = inL ;
for( ; k <= inR ; k ++){
if(in[k] == post[postR]) break ;
}
int numLeft = k - inL ;
root->lchild = create(postL , postL + numLeft - 1 , inL , k - 1) ;
root->rchild = create(postL + numLeft , postR - 1 , k + 1 , inR) ;
return root ;
}
void bfs(node* &root){
queue<node*> q ;
q.push(root) ;
int level = 0 , i = 0 ;
while(!q.empty()){
level ++ ;
int size = q.size() ;
for(int j = 0 ; j < size ; j ++){
node* top = q.front() ;
ans[i ++] = top->val ;
q.pop() ;
if(top->lchild ) q.push(top->lchild) ;
if(top->rchild) q.push(top->rchild) ;
}
if(level % 2) reverse(ans + i - size , ans + i) ;
}
}
int main(){
cin >> n ;
for(int i = 0 ; i < n ; i ++) cin >> in[i] ;
for(int i = 0 ; i < n ; i ++) cin >> post[i] ;
node* root = create(0 , n - 1 , 0 , n - 1) ;
bfs(root) ;
for(int i = 0 ; i < n ; i ++)
cout << ans[i] << ((i == n - 1)?'\n':' ') ;
return 0 ;
}