1.font属性
<body>
<canvas id="myCanvas" width="300" height="150" style="border:1px solid #d3d3d3;">
Your browser does not support the HTML5 canvas tag.
</canvas>
<script type="text/javascript">
var c = document.getElementById('myCanvas');
var ctx = c.getContext('2d');
ctx.font = "40px Arial";
ctx.fillText('hello world',10,50);
</script>
</body>
font 属性设置或返回画布上文本内容的当前字体属性。
属性值
值 | 描述 |
---|---|
font-style | 规定字体样式。可能的值:
|
font-variant | 规定字体变体。可能的值:
|
font-weight | 规定字体的粗细。可能的值:
|
font-size / line-height | 规定字号和行高,以像素计。 |
font-family | 规定字体系列。 |
caption | 使用标题控件的字体(比如按钮、下拉列表等)。 |
icon | 使用用于标记图标的字体。 |
menu | 使用用于菜单中的字体(下拉列表和菜单列表)。 |
message-box | 使用用于对话框中的字体。 |
small-caption | 使用用于标记小型控件的字体。 |
status-bar | 使用用于窗口状态栏中的字体。 |
2.textAlign属性
<body>
<canvas id="myCanvas" width="300" height="150" style="border:1px solid #d3d3d3;">
Your browser does not support the HTML5 canvas tag.
</canvas>
<script type="text/javascript">
var c = document.getElementById('myCanvas');
var ctx = c.getContext('2d');
ctx.strokeStyle = 'blue';
ctx.moveTo(150,20);
ctx.lineTo(150,170);
ctx.stroke();
ctx.textAlign = 'start';
ctx.fillText('textAlign=start',150,60);
ctx.textAlign = 'end';
ctx.fillText('textAlign=end',150,80);
ctx.textAlign = 'left';
ctx.fillText('textAlign=left',150,100);
ctx.textAlign = 'center';
ctx.fillText('textAlign=center',150,120);
ctx.textAlign = 'right';
ctx.fillText('textAlign=right',150,140);
</script>
</body>
textAlign 属性根据锚点,设置或返回文本内容的当前对齐方式。
通常,文本会从指定位置开始,不过,如果您设置为 textAlign="right" 并将文本放置到位置 150,那么会在位置 150 结束。
默认值: | start |
---|---|
JavaScript 语法: | context.textAlign="center|end|left|right|start"; |
3.textBaseline属性
<body>
<canvas id="myCanvas" width="300" height="150" style="border:1px solid #d3d3d3;">
Your browser does not support the HTML5 canvas tag.
</canvas>
<script type="text/javascript">
var c = document.getElementById('myCanvas');
var ctx = c.getContext('2d');
ctx.strokeStyle = 'blue';
ctx.moveTo(50,100);
ctx.lineTo(395,100);
ctx.stroke();
ctx.textBaseline = 'top';
ctx.fillText('textBaseline=top',5,100);
ctx.textBaseline = 'bottom';
ctx.fillText('textAlign=bottom',50,100);
ctx.textBaseline = 'middle';
ctx.fillText('textAlign=middle',120,100);
ctx.textBaseline = 'alphabetic';
ctx.fillText('textAlign=alphabetic',190,100);
ctx.textAlign = 'hanging';
ctx.fillText('textAlign=hanging',290,100);
</script>
</body>
textBaseline 属性设置或返回在绘制文本时的当前文本基线。
下面的图示演示了 textBaseline 属性支持的各种基线:
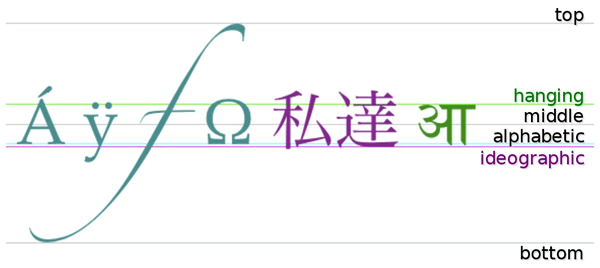
注释:fillText() 或 strokeText() 方法在画布上定位文本时,将使用指定的 textBaseline 值。
默认值: | alphabetic |
---|---|
JavaScript 语法: | context.textBaseline="alphabetic|top|hanging|middle|ideographic|bottom"; |
属性值
值 | 描述 |
---|---|
alphabetic | 默认。文本基线是普通的字母基线。 |
top | 文本基线是 em 方框的顶端。。 |
hanging | 文本基线是悬挂基线。 |
middle | 文本基线是 em 方框的正中。 |
ideographic | 文本基线是表意基线。 |
bottom | 文本基线是 em 方框的底端。 |
4.fillText()方法
<body>
<canvas id="myCanvas" width="300" height="150" style="border:1px solid #d3d3d3;">
Your browser does not support the HTML5 canvas tag.
</canvas>
<script type="text/javascript">
var c = document.getElementById('myCanvas');
var ctx = c.getContext('2d');
ctx.font = '20px Georgia';
ctx.fillText('hello world',10,50);
ctx.font = '30px Verdana';
var gra = ctx.createLinearGradient(0,0,c.width,0);
gra.addColorStop('0','magenta');
gra.addColorStop('0.5','blue');
gra.addColorStop('1.0','red');
ctx.fillStyle = gra;
ctx.fillText('w3school.com.cn',10,90);
</script>
</body>
fillText() 方法在画布上绘制填色的文本。文本的默认颜色是黑色。
提示:请使用 font 属性来定义字体和字号,并使用 fillStyle 属性以另一种颜色/渐变来渲染文本。
context.fillText(text,x,y,maxWidth);
参数 | 描述 |
---|---|
text | 规定在画布上输出的文本。 |
x | 开始绘制文本的 x 坐标位置(相对于画布)。 |
y | 开始绘制文本的 y 坐标位置(相对于画布)。 |
maxWidth | 可选。允许的最大文本宽度,以像素计。 |
5.strokeText()方法
strokeText() 方法在画布上绘制文本(没有填色)。文本的默认颜色是黑色。
提示:请使用 font 属性来定义字体和字号,并使用 strokeStyle 属性以另一种颜色/渐变来渲染文本
context.strokeText(text,x,y,maxWidth);
参数 | 描述 |
---|---|
text | 规定在画布上输出的文本。 |
x | 开始绘制文本的 x 坐标位置(相对于画布)。 |
y | 开始绘制文本的 y 坐标位置(相对于画布)。 |
maxWidth | 可选。允许的最大文本宽度,以像素计。 |
6.measureText()方法
<body>
<canvas id="myCanvas" width="300" height="150" style="border:1px solid #d3d3d3;">
Your browser does not support the HTML5 canvas tag.
</canvas>
<script type="text/javascript">
var c = document.getElementById('myCanvas');
var ctx = c.getContext('2d');
ctx.font = '30px Verdana';
var txt = 'hello world';
ctx.fillText('width:' + ctx.measureText(txt).width,10,50);
ctx.fillText(txt,10,100);
</script>
</body>
measureText() 方法返回包含一个对象,该对象包含以像素计的指定字体宽度。
提示:如果您需要在文本向画布输出之前,就了解文本的宽度,那么请使用该方法。
context.measureText(text).width;
参数 | 描述 |
---|---|
text | 要测量的文本。 |