要求:
要实现的函数:
//无参构造
my_Vector();
//析构函数
~my_Vector();
//有参构造1
my_Vector(size_t n,const T & val);
//show
void show();
//有参构造2
my_Vector(const T* p1,const T* p2);
//拷贝构造
my_Vector(const my_Vector & other);
//拷贝赋值
my_Vector & operator=(const my_Vector & other);
//at
my_Vector & at(size_t loc);
//empty
bool empty();
//full
bool full();
//front
T front();
//back
T back();
//size
size_t size();
//clear
void clear();
//expand
void expand();
//push back
void push_back();
//pop_back
void pop_back();
代码实现过程:
#include <iostream>
#include<cstring>
#include<sstream>
using namespace std;
template <typename T>
class my_Vector{
private:
T* first;
T* end;
T* last;
bool flag = false;
public:
//无参构造
my_Vector():first(){
first=last=end;;
cout << this << " 无参构造" << endl;
}
//析构函数
~my_Vector(){
delete []first;
first = end = last = nullptr;
cout << this << "析构函数" << endl;
}
//有参构造1
my_Vector(const int n,const T val):flag(true){
std::stringstream ss1;
ss1 << (void*)this;
string err = ss1.str()+".有参构造1异常";
if(n>0){
first = new T[n];
end = first+n,last = first;
for(int i=0;i<n;i++){
*last = val;
last++;
}
cout << this << "有参构造1" << endl;
}
else{
throw err;
}
}
//show
void show(){
std::stringstream ss1;
ss1 << (void*)this;
string err = ss1.str()+".show函数异常";
if(!empty()){
for(T* i=first;i!=last;i++)
cout << *i << " ";
}
else
throw err;
cout << endl;
}
//有参构造2
my_Vector(const T* p1,const T* p2):flag(true){
std::stringstream ss1;
ss1 << (void*)this;
string err = ss1.str()+".有参构造2异常";
if(p2-p1>0){
size_t size = p2-p1;
first = new T[size];
end = first+size,last = first+size;
memcpy(first,p1,sizeof(T)*size);
cout << this << "有参构造2" << endl;
}
else
throw err;
}
//拷贝构造
my_Vector(const my_Vector & other):flag(true){
size_t size = other.end-other.first;
first = new T[size];
end = first+size,last = end;
memcpy(first,other.first,sizeof(T)*size);
cout << this << "拷贝构造" << endl;
}
//拷贝赋值
my_Vector<T> & operator=(const my_Vector & other){
ssize_t before_size = size();
size_t ssize = other.end - other.first;
if(ssize>this->size()){
T* temp = new T[ssize];
memcpy(temp,first,sizeof(T)*before_size);
if(flag==true)
delete []first;
first = temp;
last = first+ssize;
end = last;
}
cout << this << "拷贝构造" << endl;
return *this;
}
//at
T & at(const size_t loc){
std::stringstream ss1;
ss1 << (void*)this;
string err = ss1.str();
if(empty()){
throw err+".at调用空容器";
}
if(loc>=0&&loc<this->size()){
return *(first+loc);
}
else{
throw err+".at调用时参数loc异常";
}
}
//empty
bool empty(){
return first==end;
}
//full
bool full(){
return last==end;
}
//front
T front(){
std::stringstream ss1;
ss1 << (void*)this;
string err = ss1.str()+".front调用空容器";
if(!empty())
return *first;
else
throw err;
}
//back
T back(){
return *(end-1);
}
//size
size_t size(){
std::stringstream ss1;
ss1 << (void*)this;
string err = ss1.str()+".size调用空容器";
if(!empty())
return last-first;
else
throw err;
}
//clear
void clear(){
end = last = first;
}
//expand
void expand(){
size_t ssize = size();
cout << "size = " << ssize << endl;
if(full()){
int* temp = new T[ssize*2];
memcpy(temp,first,(sizeof(T)*ssize));
delete []first;
first = temp;
last = first + ssize;
end = first + (ssize*2);
cout << this << " expand" << endl;
}
}
//push back
void push_back(const T val){
if(flag==false){
first = new T;
last = end = first+1;
}
else if(full()){
expand();
}
*last = val;
last++;
}
//pop_back
void pop_back(){
std::stringstream ss1;
ss1 << (void*)this;
string err = ss1.str()+".pop调用空容器";
if(!empty())
last--;
else
throw err;
}
};
int main()
{
try {
my_Vector<int> v1(5,5);
v1.show();
my_Vector<int> v2(v1);
v2.show();
int arr[5] = {1,3,5,7,9};
my_Vector<int> v3(arr+1,arr+4);
v3.show();
cout << v3.front() << endl;
cout << v3.back() << endl;
v3.push_back(9);
v3.show();
v3.pop_back();
v3.show();
cout << v3.at(1) << endl;
my_Vector<int> v4(11,1);
cout << v4.size() << endl;
v3 = v4;
cout << v3.size() << endl;
v1.clear();
v1.show(); //此处抛出异常
} catch (string err){
cout << err << endl;
}
return 0;
}
实现结果:
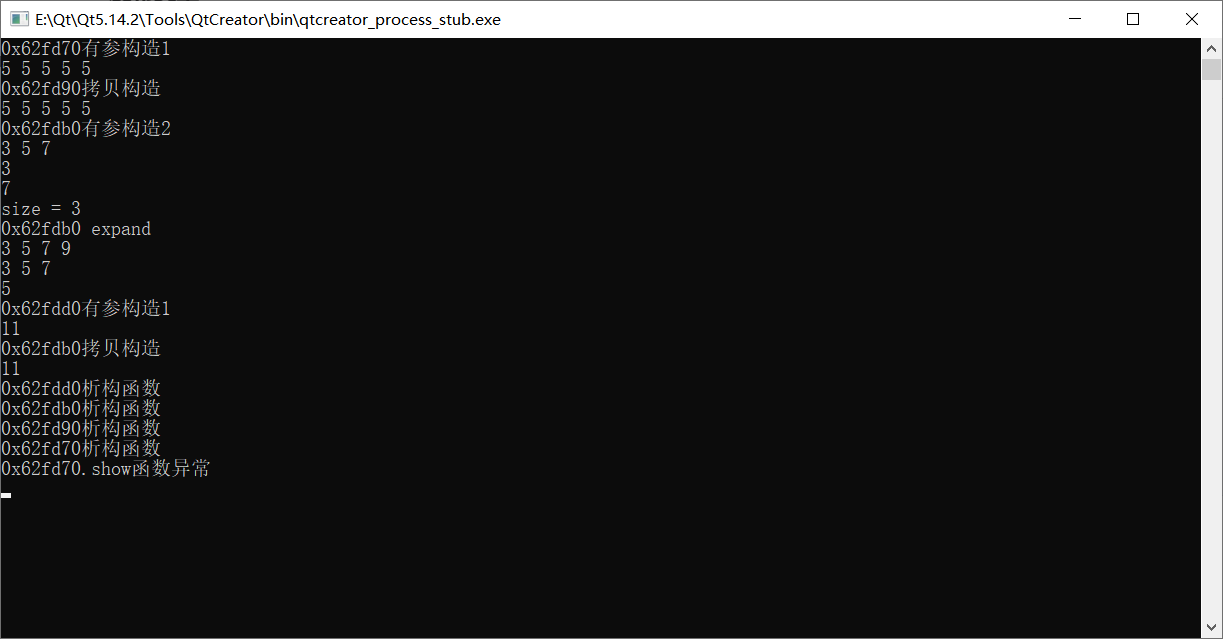