上代码:
关键:抓住头结点,就抓住了整个链表
#define _CRT_SECURE_NO_WARNINGS 1
#include<stdio.h>
#include<malloc.h>
/*Linked list of characters. The key is date.*/
typedef struct LinkNode {
char date;
struct LinkNode* next;
}LNode,*LinkList,*NodePtr;
/*Initialize the list with a header.
*The pointer to the haeder.
*/
//创建头结点
LinkList initLinkList() {
NodePtr tempHeader = (NodePtr)malloc(sizeof(LNode));
tempHeader->date = '\0';
tempHeader->next = NULL;
return tempHeader;
}// of initLinkList
/*print the list.
*paraHeader The header of the list.
*/
void printList(NodePtr paraHeader) {
NodePtr p = paraHeader->next;
while (p != NULL) {
printf("%c", p->date);
p = p->next;
}// of while
printf("\r\n");
}// of printList
/*Add an element to the tail
*paraHeader The header of the list
*paraChar The given char
*/
//尾插法
void appendElement(NodePtr paraHeader, char parachar) {
NodePtr p, q;
//Step 1. Construct a new node 创建一个节点
q = (NodePtr)malloc(sizeof(LNode));
q->date = parachar;
q->next = NULL;
//Step 2. Search to the tail 找到尾部节点
p = paraHeader;
while (p->next != NULL) {
p = p->next;
}// of while
//Step 3. Now link 链接
p->next = q;
}// of appendElement
/*Insert an elemnet to the given position.
*paraHeader The header of the list
*paraChar The given char.
paraPosition the given position.
*/
//在链表中插入节点
void insertElement(NodePtr paraHeader, char paraChar, int paraPosition) {
NodePtr p, q;
// Step 1. Search to the posiotion.找到要插入的节点
p = paraHeader;
for (int i = 0; i < paraPosition; i++) {
p = p->next;
if (p == NULL) {
printf("The position %d is beyond the scope of the list.", paraPosition);
return;
}// of if
}// of for i
// Step 2. Construct a new node.
q = (NodePtr)malloc(sizeof(LNode));
q->date = paraChar;
// Step 3. Now link.
printf("linking\r\n");
q->next = p->next;
p->next = q;
}//of insertElement
/*Delete an element from the list.
*paraHeader The header of the list.
paraChar The given char.*/
//删除节点
void deleteElement(NodePtr paraHeader, char paraChar) {
NodePtr p, q;
p = paraHeader;
while ((p->next != NULL) && (p->next->date != paraChar)) {
p = p->next;
}//of while
if (p->next == NULL) {
printf("Cannot delete %c\r\n", paraChar);
return;
}//of if
q = p->next;
p->next = p->next->next;
free(q);
}//of deleteElement
/*Unit test*/
void appendINsertDeleteTest() {
//Step 1. Initialize an empty list
LinkList tempList = initLinkList();
printList(tempList);
/*Step 2. Add some characters.*/
appendElement(tempList, 'H');
appendElement(tempList, 'e');
appendElement(tempList, 'l');
appendElement(tempList, 'l');
appendElement(tempList, 'o');
appendElement(tempList, '!');
printList(tempList);
/*Step 3. Delete some characters(the first occurrence).*/
appendElement(tempList, 'e');
appendElement(tempList, 'a');
appendElement(tempList, 'o');
printList(tempList);
/*Sterp 4. Insert to a given position*/
insertElement(tempList, 'o', 1);
printList(tempList);
}// of appendInsertDeleteTest
/*Adress test: beyond the book.*/
void basicAdressTest() {
LNode tempNode1, tempNode2;
tempNode1.date = 4;
tempNode2.next = NULL;
tempNode2.date = 6;
tempNode2.next = NULL;
printf("The first node: %d, %d, %d\r\n",
&tempNode1, &tempNode1.date, &tempNode1.next);
printf("The first node: %d, %d, %d\r\n",
&tempNode2, &tempNode2.date, &tempNode2.next);
tempNode1.next = &tempNode2;
}// of basicAdressTest
/*The entrance*/
int main() {
appendINsertDeleteTest();
}// of main
运行结果:
尾插法:
步骤1:先定义临时指针,在利用malloc分配空间,建立节点
步骤2:通过循环利用指针,找到链表尾部节点,关键代码:p=p->next;
步骤3:利用p指针链接节点
/*Add an element to the tail
*paraHeader The header of the list
*paraChar The given char
*/
//尾插法
void appendElement(NodePtr paraHeader, char parachar) {
NodePtr p, q;
//Step 1. Construct a new node 创建一个节点
q = (NodePtr)malloc(sizeof(LNode));
q->date = parachar;
q->next = NULL;
//Step 2. Search to the tail 找到尾部节点
p = paraHeader;
while (p->next != NULL) {
p = p->next;
}// of while
//Step 3. Now link 链接
p->next = q;
}// of appendElement
图示: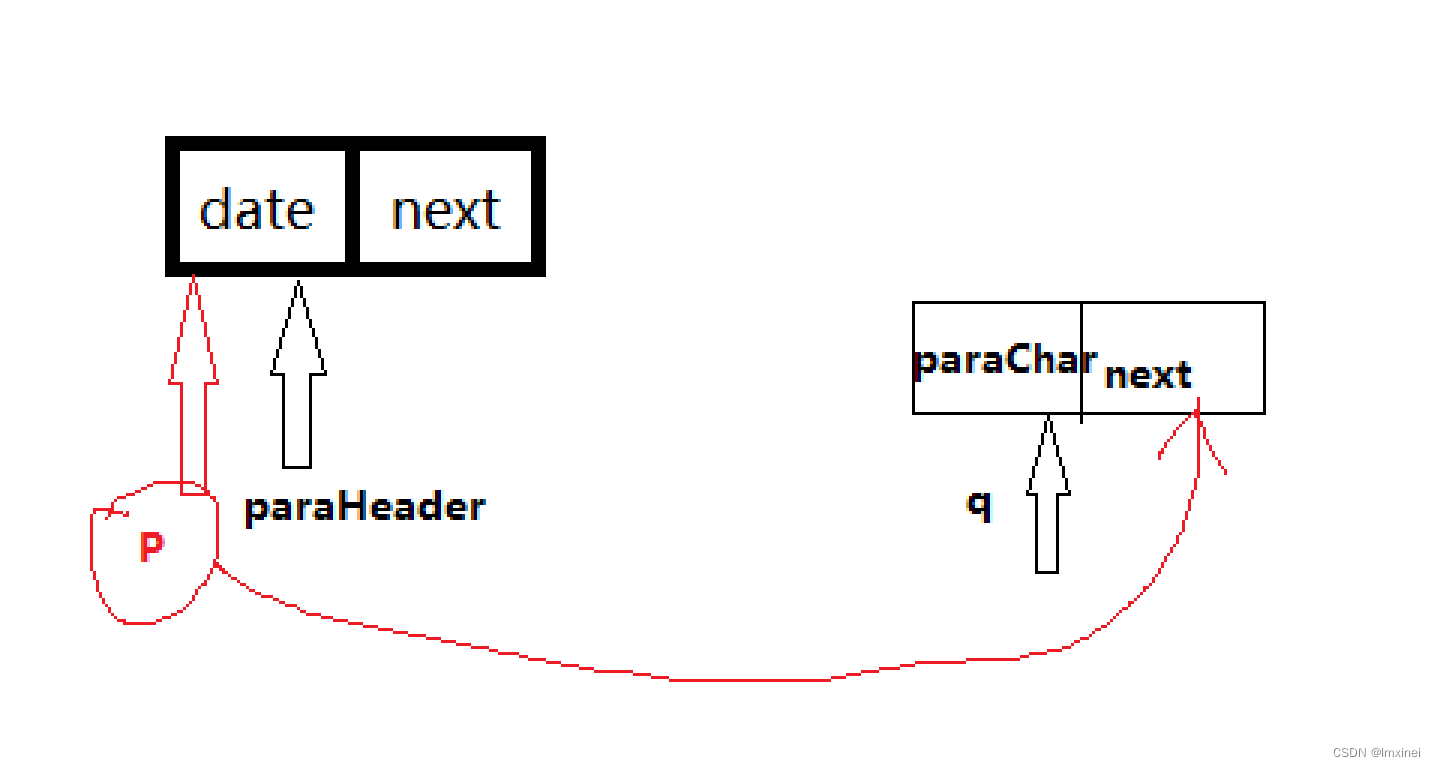
在链表中插入节点:
步骤1:通过for循环,找到position
步骤2:建立要插入的节点
步骤3:链接,
关键代码:q->next=p->next;
p->next=q;
/*Insert an elemnet to the given position.
*paraHeader The header of the list
*paraChar The given char.
paraPosition the given position.
*/
//在链表中插入节点
void insertElement(NodePtr paraHeader, char paraChar, int paraPosition) {
NodePtr p, q;
// Step 1. Search to the posiotion.找到要插入的节点
p = paraHeader;
for (int i = 0; i < paraPosition; i++) {
p = p->next;
if (p == NULL) {
printf("The position %d is beyond the scope of the list.", paraPosition);
return;
}// of if
}// of for i
// Step 2. Construct a new node.
q = (NodePtr)malloc(sizeof(LNode));
q->date = paraChar;
// Step 3. Now link.
printf("linking\r\n");
q->next = p->next;
p->next = q;
}//of insertElement
图示: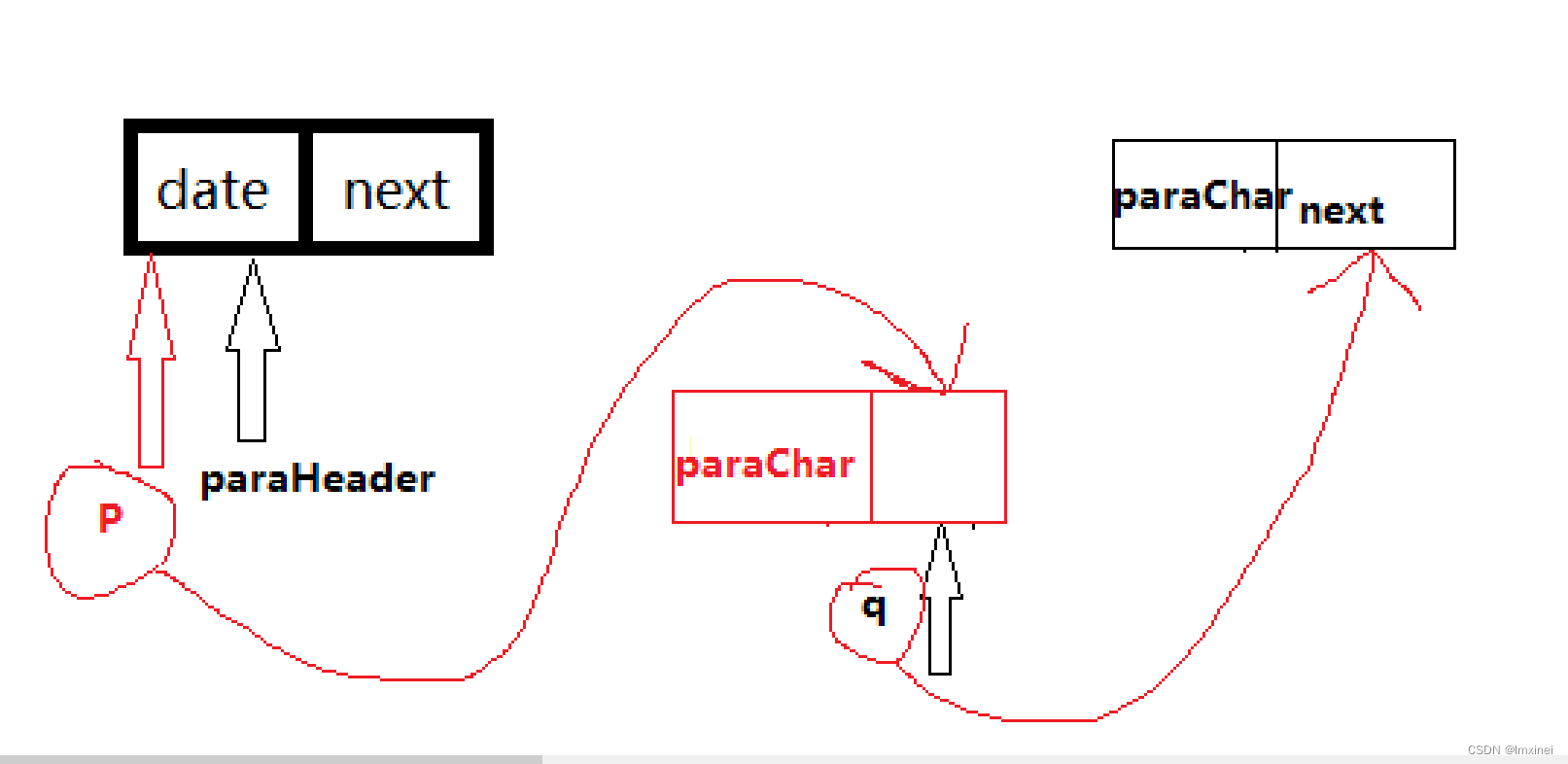
删除节点:
步骤1:循环找到要删除的节点
步骤2:将p的指针域赋值给q,p指向q的下一节点
关键代码:q=p->next;
p->next=p->next->next;
/*Delete an element from the list.
*paraHeader The header of the list.
paraChar The given char.*/
//删除节点
void deleteElement(NodePtr paraHeader, char paraChar) {
NodePtr p, q;
p = paraHeader;
while ((p->next != NULL) && (p->next->date != paraChar)) {
p = p->next;
}//of while
if (p->next == NULL) {
printf("Cannot delete %c\r\n", paraChar);
return;
}//of if
q = p->next;
p->next = p->next->next;
free(q);
}//of deleteElement
图示: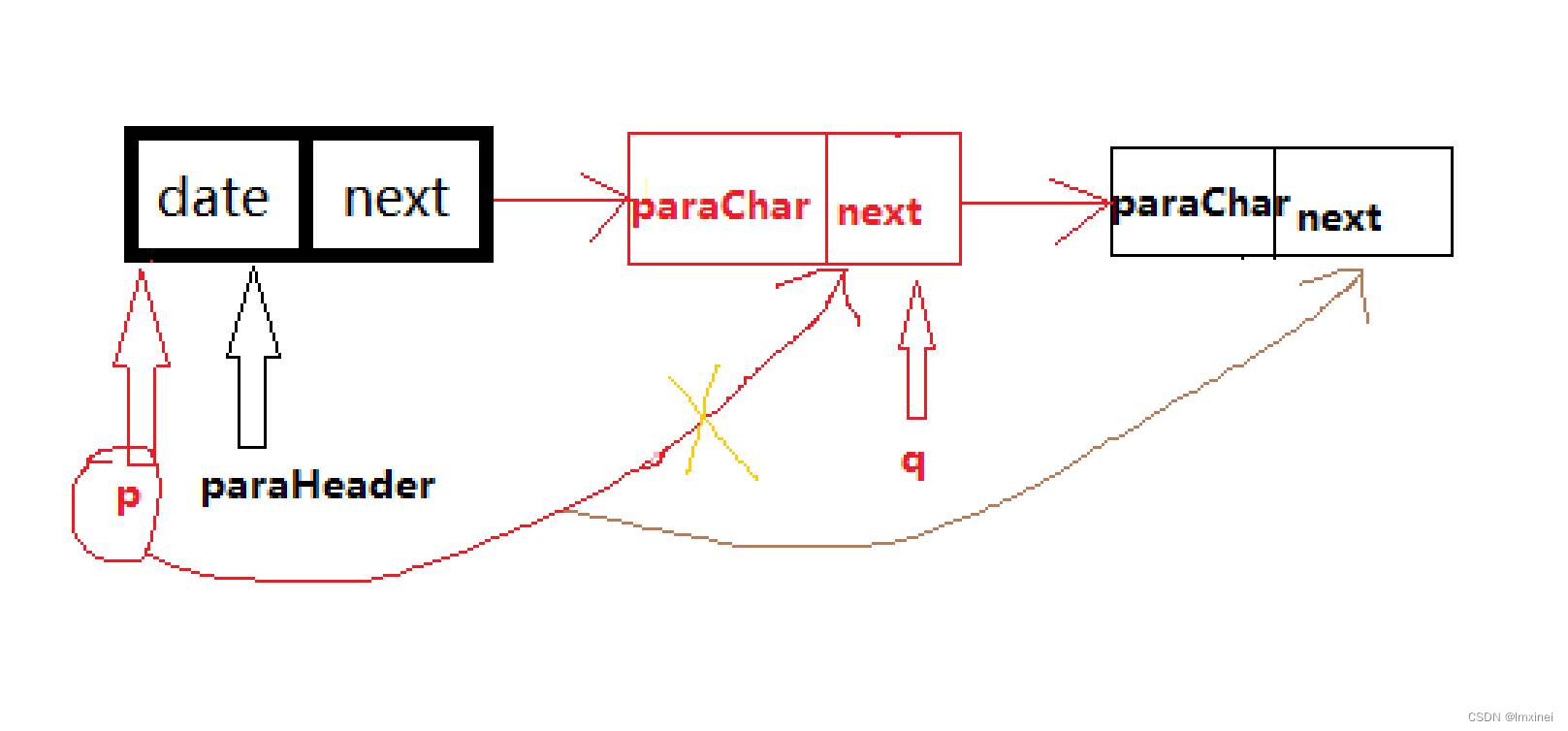
上课收获:
第三次数据结构收获,抄代码也是一门学问,要感受作者的逻辑思维,变量名称,代码规范的格式,在抄代码的过程中,小编也在不断学习,精进自己的代码书写格式和老师的精妙之处。
第三次老师授课,让我更重视基础了,对“抄代码”有不一样的认识,以前我只是简单的crtl cv,然后自己理解代码,但事实上知识根本没有真正的进脑,老是抄了就忘,忘了就抄,通过几次的手抄代码,对这一板块的知识也更理解了