1. C语言的类型转换
#include <iostream>
#include <string>
using namespace std;
void Insert(size_t pos, char ch)
{
size_t _size = 5;
//....
int end = _size - 1;
// size_t end = _size - 1;
while (end >= pos) // end隐式类型转换
{
//_str[end + 1] = _str[end];
--end;
}
}
void Test1()
{
int i = 1;
// 隐式类型转换(意义相近的类型)
double d = i;
printf("%d, %.2f\n", i, d);
int* p = &i;
// 显示的强制类型转换(意义不相近的类型,值转换后有意义)
int address = (int)p;
printf("%x, %d\n", p, address);
Insert(3, 'a');
Insert(0, 'a');// 触发死循环
}
int main()
{
Test1();
return 0;
}
- 隐式类型转换(意义相近的类型)
- 显示的强制类型转换(意义不相近的类型,值转换后有意义)
2. C语言类型转换的缺陷
- 隐式类型转化有些情况下可能会出现问题: 比如数据精度丢失
- 显示类型转换将所有情况混合在一起,代码不够清晰
3. C++强制类型转换
标准C++为了加强类型转换的可视性,引入了四种命名的强制类型转换操作符
3.1 static_cast关键字 -> 隐式类型转换
#include <iostream>
#include <string>
using namespace std;
int main()
{
double d = 12.34;
int a = static_cast<int>(d);
cout << a << endl;
int* p = &a;
//int address = static_cast<int>(p);// 不支持的
return 0;
}
- static_cast用于非多态类型的转换(静态转换),编译器隐式执行的任何类型转换都可用static_cast就像C语言的隐式类型转换,常用于意义相近的类型
- 但是它不能用于两个不相关的类型进行转换
3.2 reinterpret_cast关键字 -> 强制类型转换
#include <iostream>
#include <string>
using namespace std;
int main()
{
int a = 100;
int* p = &a;
int address = reinterpret_cast<int>(p);
return 0;
}
-
reinterpret_cast操作符通常为操作数的位模式提供较低层次的重新解释,用于将一种类型转换为另一种不同的类型,
-
reinterpret_cast就像 C语言的强制类型转换
-
常用于两个类型不相关的
3.3 const_cast关键字->取消变量的const属性
- const_cast最常用的用途就是删除变量的const属性,方便赋值
- volatile关键字取消编译器的优化
3.4 dynamic_cast->父类指针 转换 子类指针
dynamic_cast
用于将一个
父类
对象的指针/引用转换为
子类
对象的指针或引用(动态转换)
向上转型:
子类对象指针/引用->父类指针/引用(不需要转换,赋值兼容规则)->切片
向下转型:
父类对象指针/引用->子类指针/引用(用dynamic_cast转型是安全的)
3.4.1案例一
#include <iostream>
using namespace std;
class A
{
public:
virtual void f(){}
public:
int _a = 0;
};
class B : public A
{
public:
int _b = 1;
};
// A*指针pa有可能指向父类,有可能指向子类
void fun(A* pa)
{
// 如果pa是指向子类,那么可以转换,转换表达式返回正确的地址
// 如果pa是指向父类,那么不能转换,转换表达式返回nullptr
B* pb = dynamic_cast<B*>(pa); // 安全的
//B* pb = (B*)pa; // 不安全
if (pb)
{
cout << "转换成功" << endl;
pb->_a++;
pb->_b++;
cout << pb->_a << ":" << pb->_b << endl;
}
else
{
cout << "转换失败" << endl;
}
}
int main()
{
A aa;
// 父类对象无论如何都是不允许转换成子类对象的
//B bb = dynamic_cast<B>(aa);// error
//B bb = (B)aa;// error
B bb;
fun(&aa);
fun(&bb);
fun(nullptr);
return 0;
}
-
dynamic_cast只能用于 父类含有虚函数的类
-
dynamic_cast会 先检查是否能转换成功 ,能成功则转换,不能则返回0
3.4.2案例一
#include <iostream>
using namespace std;
class A1
{
public:
virtual void f(){}
public:
int _a1 = 0;
};
class A2
{
public:
virtual void f(){}
public:
int _a2 = 0;
};
class B : public A1, public A2
{
public:
int _b = 1;
};
int main()
{
B bb;
A1* ptr1 = &bb;
A2* ptr2 = &bb;
cout << ptr1 << endl;
cout << ptr2 << endl << endl;
B* pb1 = (B*)ptr1;
B* pb2 = (B*)ptr2;
cout << pb1 << endl;
cout << pb2 << endl << endl;
B* pb3 = dynamic_cast<B*>(ptr1);
B* pb4 = dynamic_cast<B*>(ptr2);
cout << pb3 << endl;
cout << pb4 << endl << endl;
return 0;
}
3.5 类型转换的实质
类型转换是通过临时对象来实现的,且临时对象具有常性,
- 但是尽量不要使用强制类型转换
3.6 常见面试题
- C++中的4种类型转换分别是:____ 、____ 、____ 、____。
- 分别是static_cast、reinterpret_cast、const_cast和dynamic_cast。
- 说说4种类型转换的应用场景。
- static_cast用于相近类型的类型之间的转换,编译器隐式执行的任何类型转换都可用
- reinterpret_cast用于两个不相关类型之间的转换。
- const_cast用于删除变量的const属性,方便赋值。
- dynamic_cast用于安全的将父类的指针(或引用)转换成子类的指针(或引用)
4. RTTI->运行时类型识别
RTTI:Run-time Type identifification的简称,即:运行时类型识别
C++通过以下方式来支持RTTI:
- typeid运算符(获取对象类型字符串)
- dynamic_cast运算符(父类的指针指向父类对象或者子类对象)
- decltype(推导一个对象类型,这个类型可以用来定义另一个对象)
5. C语言的输入与输出
printf/scanf
fprintf/fscanf
sprintf/sscanf
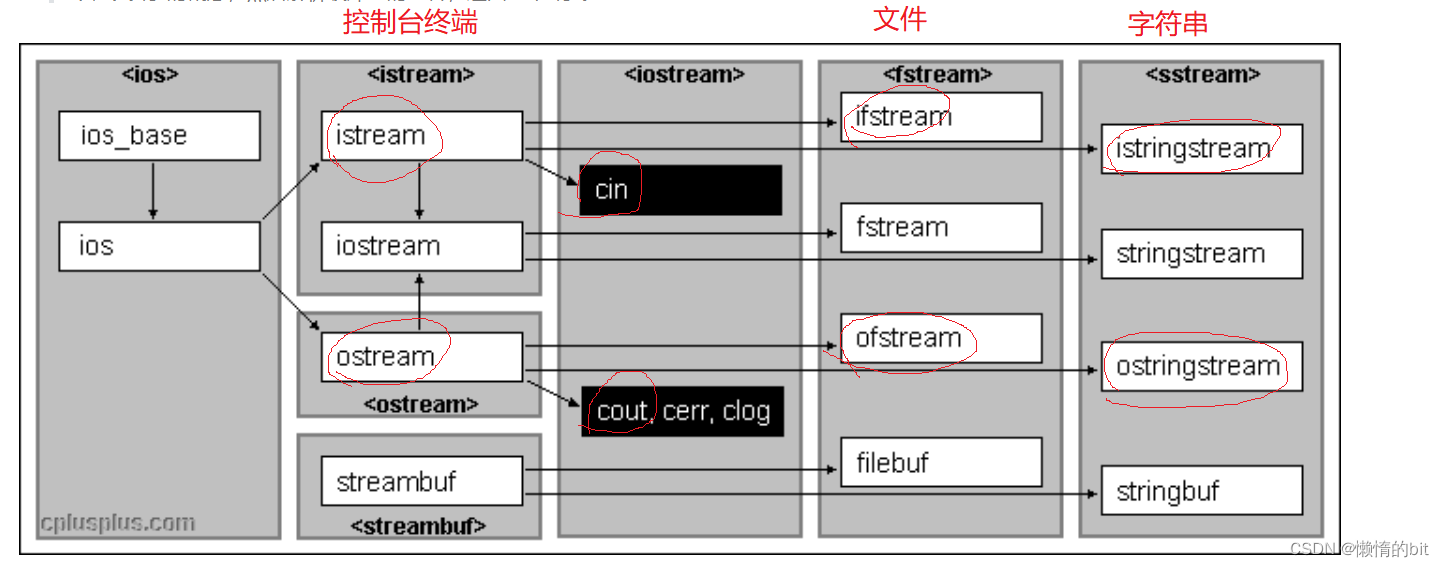
#include <iostream>
using namespace std;
class A
{
public:
// explicit A(int a)
A(int a)
:_a(a)
{}
operator int()
{
return _a;
}
private:
int _a;
};
int main()
{
// 内置类型 转换成自定义类型
A aa1 = 1; // 隐式类型转换 用1构造A临时对象,再拷贝构造aa1,优化后直接1构造aa1
// 自定义类型 转换成内置类型
int i = aa1;
return 0;
}
- int i = aa1;能将自定义类型转换成内置类型,主要因为operator int()
-
explicit关键字: 不允许隐式类型的转换
5.1 多组输入
#include <iostream>
using namespace std;
class Date
{
friend ostream& operator << (ostream& out, const Date& d);
friend istream& operator >> (istream& in, Date& d);
public:
Date(int year = 1, int month = 1, int day = 1)
:_year(year)
, _month(month)
, _day(day)
{}
operator bool()
{
// 这里是随意写的,假设输入_year为0,则结束
if (_year == 0)
return false;
else
return true;
}
private:
int _year;
int _month;
int _day;
};
istream& operator >> (istream& in, Date& d)
{
in >> d._year >> d._month >> d._day;
return in;
}
ostream& operator << (ostream& out, const Date& d)
{
out << d._year << " " << d._month << " " << d._day;
return out;
}
// C++ IO流,使用面向对象+运算符重载的方式
// 能更好的兼容自定义类型,流插入和流提取
int main()
{
// 自动识别类型的本质--函数重载
// 内置类型可以直接使用--因为库里面ostream类型已经实现了
int i = 1;
double j = 2.2;
cout << i << endl;
cout << j << endl;
// 自定义类型则需要我们自己重载<< 和 >>
Date d(2022, 4, 10);
cout << d;
while (d)
{
cin >> d;
cout << d;
}
return 0;
}
- while(cin >> d){}遇到文件退出符才结束,因为库里面实现了operator bool()
5.2 fstream文件流
#include <iostream>
#include <fstream>
using namespace std;
int main()
{
ifstream ifs("Test.cpp");
char ch = ifs.get();
while (ifs)
{
cout << ch;
ch = ifs.get();
}
return 0;
}
- C++中也有对文件进行操作的流fstream
- 它的使用就可以不用打开文件,和关闭文件
- 库里面写的是一个类它会自己调构造,调析构
5.3 C++ 文件操作
#include <iostream>
#include <fstream>
using namespace std;
class Date
{
friend ostream& operator << (ostream& out, const Date& d);
friend istream& operator >> (istream& in, Date& d);
public:
Date(int year = 1, int month = 1, int day = 1)
:_year(year)
, _month(month)
, _day(day)
{}
operator bool()
{
// 这里是随意写的,假设输入_year为0,则结束
if (_year == 0)
return false;
else
return true;
}
private:
int _year;
int _month;
int _day;
};
istream& operator >> (istream& in, Date& d)
{
in >> d._year >> d._month >> d._day;
return in;
}
ostream& operator << (ostream& out, const Date& d)
{
out << d._year << " " << d._month << " " << d._day;
return out;
}
int main()
{
ifstream ifs("test.txt");// 默认以读的方式打开
//fscanf("%d%s%f", )
int i;
string s;
double d;
Date de;
ifs >> i >> s >> d >> de;
cout << i << s << d << de;
return 0;
}
5.4 二进制文件的读写 && 文本文件的读写
#include <iostream>
#include <fstream>
using namespace std;
struct ServerInfo
{
char _address[32];
//string _address;
int _port; // 100
// Date _date;
};
struct ConfigManager
{
public:
ConfigManager(const char* filename = "server.config")
:_filename(filename)
{}
// 二进制文件的写
void WriteBin(const ServerInfo& info)
{
ofstream ofs(_filename, ios_base::out | ios_base::binary);
ofs.write((char*)&info, sizeof(info));
}
// 二进制文件的写
void ReadBin(ServerInfo& info)
{
ifstream ifs(_filename, ios_base::in | ios_base::binary);
ifs.read((char*)&info, sizeof(info));
}
// 文本文件的写
void WriteText(const ServerInfo& info)
{
ofstream ofs(_filename, ios_base::out);
ofs << info._address << info._port;
}
// 文本文件的读
void ReadText(ServerInfo& info)
{
ifstream ifs(_filename, ios_base::in | ios_base::binary);
ifs >> info._address >> info._port;
}
private:
string _filename; // 配置文件
};
int main()
{
// 二进制的写
ServerInfo winfo = { "127.0.0.1", 888 };
ConfigManager cm;
cm.WriteBin(winfo);
// cm.WriteText(winfo);// 文本的写
// 二进制的读
ServerInfo rinfo;
ConfigManager rm;
rm.ReadBin(rinfo);
// rm.ReadText(rinfo);// 文本的读
cout << rinfo._address << endl;
cout << rinfo._port << endl;
return 0;
}
-
二进制读写:在内存如何存储,就如何写到磁盘文件
-
优点:快
-
缺点:写出去内容看不见
-
- 文本读写:对象数据序列化字符串写出来,读回来也是字符串,反序列化转成对象数据
-
优点:可以看见写出去是什么
-
缺点:存在一个转换过程,要慢一些
-
5.5 字符串流-- stringstream
#include <iostream>
#include <fstream>
#include<sstream>
using namespace std;
struct ChatInfo
{
string _name; // 名字
int _id; // id
string _msg; // 聊天信息
};
int main()
{
// 序列化
ChatInfo winfo = { "张三", 135246, "晚上一起看电影吧" };
//ostringstream oss;
stringstream oss;
oss << winfo._name << endl;
oss << winfo._id << endl;
oss << winfo._msg << endl;
string str = oss.str();
cout << str << endl;
// 网络传输str,另一端接收到了字符串串信息数据
// 反序列化
ChatInfo rInfo;
//istringstream iss(str);
stringstream iss(str);
iss >> rInfo._name;
iss >> rInfo._id;
iss >> rInfo._msg;
cout << "----------------------------------" << endl;
cout << rInfo._name << "[" << rInfo._id << "]:>" << rInfo._msg << endl;
cout << "----------------------------------" << endl;
return 0;
}