Java–GUI–贪吃蛇小游戏
效果示例图
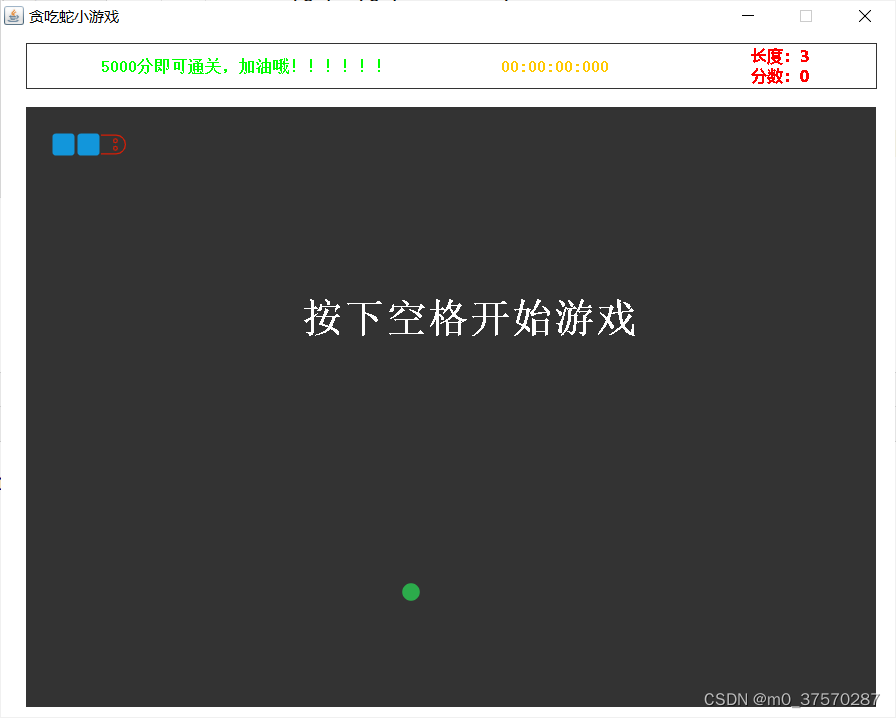
代码示例
启动类
package com.zy.gui.snakegame;
import javax.swing.*;
public class StartGame {
public void init(){
JFrame frame = new JFrame();
frame.add(new GamePanel());
frame.setTitle("贪吃蛇小游戏");
frame.setBounds(200,200,900,720);
frame.setResizable(false);
frame.setVisible(true);
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new StartGame().init();
}
}
数据类
package com.zy.gui.snakegame;
import javax.swing.*;
import java.net.URL;
public class GameData {
public static URL rightUrl = GameData.class.getResource("/snakeimg/right.png");
public static ImageIcon rightIcon = new ImageIcon(rightUrl);
public static URL leftUrl = GameData.class.getResource("/snakeimg/left.png");
public static ImageIcon leftIcon = new ImageIcon(leftUrl);
public static URL downUrl = GameData.class.getResource("/snakeimg/down.png");
public static ImageIcon downIcon = new ImageIcon(downUrl);
public static URL upUrl = GameData.class.getResource("/snakeimg/up.png");
public static ImageIcon upIcon = new ImageIcon(upUrl);
public static URL foodUrl = GameData.class.getResource("/snakeimg/food.png");
public static ImageIcon foodIcon = new ImageIcon(foodUrl);
public static URL bodyUrl = GameData.class.getResource("/snakeimg/body.png");
public static ImageIcon bodyIcon = new ImageIcon(bodyUrl);
}
面板绘制类
package com.zy.gui.snakegame;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Calendar;
import java.util.Date;
import java.util.Random;
public class GamePanel extends JPanel implements KeyListener, ActionListener {
private int snakeLen;
private int[] snakeX = new int[900];
private int[] snakeY = new int[900];
private String orientation;
private boolean startState;
private int foodX;
private int foodY;
private Random foodRandom = new Random();
private boolean overState;
private boolean passState;
private int score;
private long startTime;
private long suspendTime;
private long suspendSumTime;
private long seconds;
private String showTime;
private int delay;
private Timer timer = new Timer(delay,this);
public GamePanel(){
initSnake();
setFocusable(true);
addKeyListener(this);
}
public void initSnake(){
snakeLen = 3;
orientation = "right";
for (int i = 0; i < snakeLen; i++) {
snakeX[i] = 100-i*25;
snakeY[i] = 100;
}
delay = 100;
timer.setDelay(delay);
timer.start();
foodX = 25 + 25*foodRandom.nextInt(34);
foodY = 75 + 25*foodRandom.nextInt(24);
score = 0;
startTime = 0L;
suspendTime = 0L;
suspendSumTime = 0L;
seconds = 0L;
showTime = "00:00:00:000";
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
this.setBackground(Color.white);
g.drawRect(25,11,850,45);
g.fillRect(25,75,850,600);
g.setColor(Color.green);
g.setFont(new Font("宋体",Font.BOLD,16));
g.drawString("5000分即可通关,加油哦!!!!!!",100,40);
g.setColor(Color.orange);
g.setFont(new Font("宋体",Font.BOLD,16));
g.drawString(showTime,500,40);
g.setColor(Color.red);
g.setFont(new Font("微软雅黑",Font.BOLD,16));
g.drawString("长度:"+snakeLen,750,30);
g.drawString("分数:"+score,750,50);
GameData.foodIcon.paintIcon(this,g,foodX,foodY);
for (int i = 0; i < snakeLen; i++) {
if(i == 0){
switch (orientation){
case "right":
GameData.rightIcon.paintIcon(this,g,snakeX[i],snakeY[i]);
break;
case "left":
GameData.leftIcon.paintIcon(this,g,snakeX[i],snakeY[i]);
break;
case "down":
GameData.downIcon.paintIcon(this,g,snakeX[i],snakeY[i]);
break;
case "up":
GameData.upIcon.paintIcon(this,g,snakeX[i],snakeY[i]);
break;
}
}else{
GameData.bodyIcon.paintIcon(this,g,snakeX[i],snakeY[i]);
}
}
if(!startState){
g.setColor(Color.white);
g.setFont(new Font("宋体",Font.BOLD,40));
g.drawString("按下空格开始游戏",300,300);
}
if(overState){
g.setColor(Color.orange);
g.setFont(new Font("宋体",Font.BOLD,40));
g.drawString("游戏失败,按下空格重新开始",200,300);
}
if(passState){
g.setColor(Color.green);
g.setFont(new Font("宋体",Font.BOLD,40));
g.drawString("游戏通关,按下空格重新开始",200,300);
}
g.setColor(Color.black);
}
@Override
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
if(keyCode == KeyEvent.VK_SPACE){
if(overState){
overState = false;
initSnake();
}else if(passState){
passState = false;
initSnake();
}else{
startState = !startState;
repaint();
}
}
if(startState && !overState && !passState){
switch (keyCode){
case KeyEvent.VK_UP:
orientation = "up";
repaint();
break;
case KeyEvent.VK_DOWN:
orientation = "down";
repaint();
break;
case KeyEvent.VK_LEFT:
orientation = "left";
repaint();
break;
case KeyEvent.VK_RIGHT:
orientation = "right";
repaint();
break;
}
}
}
@Override
public void actionPerformed(ActionEvent e) {
if(startState && !overState && !passState){
if(startTime == 0){
startTime = System.currentTimeMillis();
}
seconds = System.currentTimeMillis() - startTime - suspendSumTime;
showTime = format(seconds);
if(snakeX[0] == foodX && snakeY[0] == foodY){
snakeLen++;
score += 10;
foodX = 25 + 25*foodRandom.nextInt(34);
foodY = 75 + 25*foodRandom.nextInt(24);
if(snakeLen%10 == 0){
delay += 50;
timer.setDelay(delay);
}
if(snakeLen>=500){
passState = true;
}
}
for (int i = snakeLen - 1; i > 0; i--) {
snakeX[i] = snakeX[i-1];
snakeY[i] = snakeY[i-1];
}
switch (orientation){
case "right":
snakeX[0] += 25;
if(snakeX[0]>850){
snakeX[0] = 25;
}
break;
case "left":
snakeX[0] -= 25;
if(snakeX[0]<25){
snakeX[0] = 850;
}
break;
case "down":
snakeY[0] += 25;
if(snakeY[0]>650){
snakeY[0] = 75;
}
break;
case "up":
snakeY[0] -= 25;
if(snakeY[0]<75){
snakeY[0] = 650;
}
break;
}
for (int i = 1; i < snakeLen; i++) {
if(snakeX[0] == snakeX[i] && snakeY[0] == snakeY[i]){
overState = true;
}
}
repaint();
}else if(!startState && seconds>0){
suspendTime = System.currentTimeMillis();
suspendSumTime += (System.currentTimeMillis() - suspendTime);
}
timer.start();
}
@Override
public void keyReleased(KeyEvent e) {}
@Override
public void keyTyped(KeyEvent e) {}
private String format(long timekeeping){
int hour,minute,second,milli;
milli = (int) (timekeeping%1000);
timekeeping /= 1000;
second = (int) (timekeeping%60);
timekeeping /= 60;
minute = (int) (timekeeping%60);
timekeeping /= 60;
hour = (int) (timekeeping%60);
return String.format("%02d:%02d:%02d:%03d",hour,minute,second,milli);
}
}