Eureka作为注册中心:
- 提供了Eureka服务器端与客户端
- 主要用于服务器管理
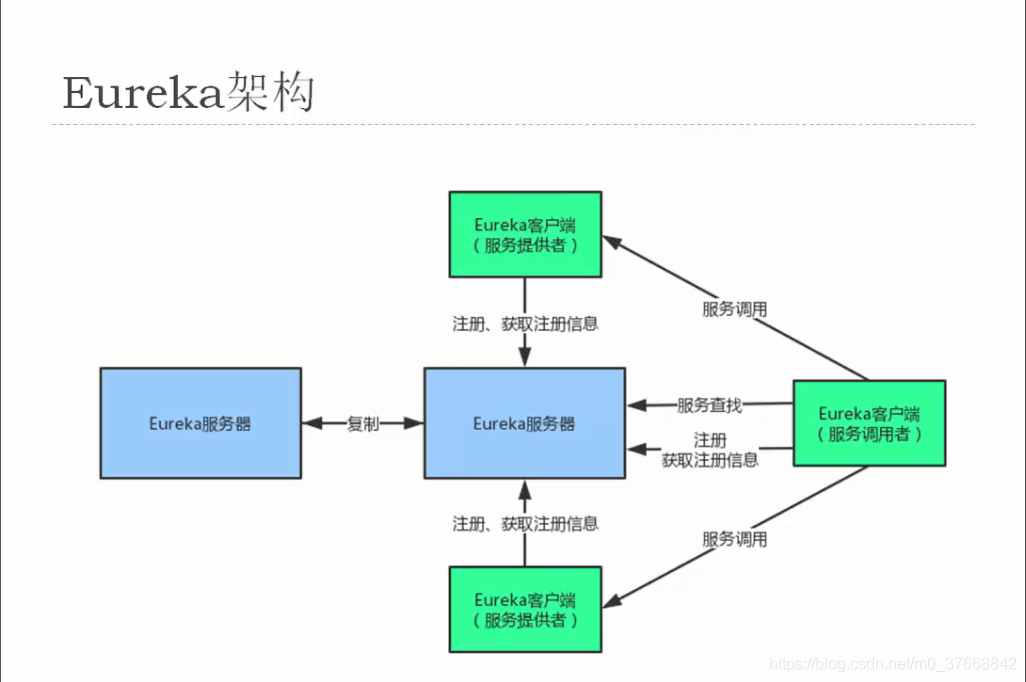
Eureka服务端
1、引入pom文件
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
2、使用@SpringBootApplication:声明是springboot应用
3、使用@EnableEurekaServer:声明是eureka服务
通过@EnableEurekaServer注解启动一个注册中心给其他应用用于对话
4、配置文件
默认设置下,该注册中心也会把自己作为客户端来尝试注册自己,所以要禁止该行为。在application.properties中增加如下配置:
server.port=8100
eureka.instance.hostname=localhost
eureka.client.register-with-eureka=false
eureka.client.fetch-registry=false
eureka.client.serviceUrl.defaultZone=http://${eureka.instance.hostname}:${server.port}/eureka/
eureka.client.register-with-eureka=false :由于自己为注册中心,所以设置成false,代表不想自己注册自己
eureka.client.fetch-registry=false : 由于注册中心的职责就是维护服务实例,它并不需要去检索服务,所以为false
注:如果单个eureka启动,并且这两个属性设置为ture或者没配置(默认为true),则启动可能报错,Cannot execute request on any known server。
eureka.client.serviceUrl.defaultZone:eureka注册地址/管控台访问路径
Eureka客户端
1、引入pom文件
<!--eureka 客户端-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<!--eureka 负载均衡-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-netflix-ribbon</artifactId>
</dependency>
2、使用@SpringBootApplication:声明是springboot应用
3、使用@EnableEurekaClient:声明是eureka客户端
4、配置文件
spring:
application:
name: first-police
# 注册到注册中心
eureka:
instance:
prefer-ip-address: true
client:
service-url:
defaultZone: http://localhost:8100/eureka/
5、写Controller
服务一,被调用方
@RequestMappering(value = "/call/{id}",method = RequestMethod.GET, produces = MediaType.APPLICATION_JSON_VALUE)
public Police call(@PathVariable Integer id){
return new Police(1,"警察叔叔?♀️");
}
服务二,demo,去调用服务一
@Controller
@Configuration //将@Bean注册到容器中,@Bean和该注解一般一起使用
public class TestController{
@Bean
@LoaBalanced //负载均衡的功能
public RestTemplate getRestTemplate(){
return new RestTemplate();
}
@Getting("/router")
@ResponseBody
public String router(){
RestTemplate tp1 = getRestTemplate();
//first-police:是另一个客户端注册到eureka上面的名称
String json = tp1.getForObject("http://first-police/call/1",String.class);
System.out.print("json");
}
}
Eureka集群搭建
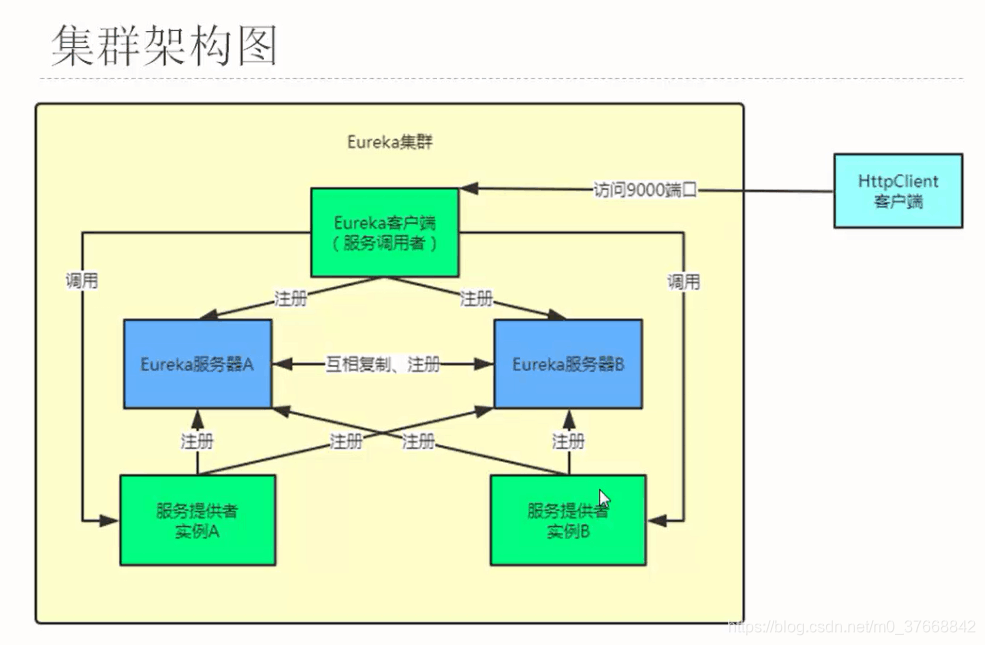
1、配置host地址
127.0.0.1 slave1
127.0.0.1 slace2
2、修改注册中心的yml配置文件
server:
port:8761
spring:
application:
name: cloud-one
profiles: slaveA
eureka:
client:
serviceUrl:
defaultZone: http://slave2:8762/eureka
---
server:
port:8762
spring:
application:
name: cloud-one
profiles: slaveB
eureka:
client:
serviceUrl:
defaultZone: http://slave1:8761/eureka
eureka.client.register-with-eureka 和eureka.client.fetch-registry 在集群配置中不设置或者设置为ture;
3、修改服务提供者eureka客户端的yml配置文件
server:
port:8080
spring:
application:
name: cloud-police
eureka:
client:
serviceUrl:
defaultZone: http://localhost:8761/eureka,http://localhost:8762/eureka
Eureka客户端健康监测与常用配置
1、配置服务提供者(eurekaClient)心跳发送 && 元数据metadata-map配置与使用
server:
port:8080
spring:
application:
name: cloud-police
eureka:
instance:
#Eureka客户端向服务器发送心跳间隔,单位为秒,默认30s
lease-renewal-interval-in-seconds: 5
#Eureka服务器在收到最后一次心跳等待的时间上限,单位为秒,超出则剔除,默认为90s
lease-expiration-duration-in-seconds: 10
client:
#同步eureka注册中心的服务列表,默认30s(正式环境中就默认30s就行,很耗费资源的)
registry-fetch-interval-seconds: 5
metadata-map:
company-name: xxxCompany
serviceUrl:
defaultZone: http://localhost:8761/eureka,http://localhost:8762/eureka
#配置com.netflix包的日志级别
logging:
level:
com.netflix: DEBUG
metadata-map属性的使用:
metadata-map属性的使用:
@GetMapping("/meta")
@ResponseBody
public String getMetaData(){
List<ServieInstance> instances = discoveryClient.getInstances("cloud-police");
for(ServiceInstance ins : instances){
String name = ins.getMetadate().get("company-name");
System.out.print(ins.getPort() = "---" + name);
return name;
}
}
2、配置注册中心(eurekaServer)禁用服务保护
server:
port:8761
spring:
application:
name: cloud-one
eureka:
client:
register-with-eureka: false
fetch-registry: false
#配置注册中心关闭自我保护机制
server:
enable-self-preservation: false # 设为false,关闭自我保护,默认为true
# eviction-interval-timer-in-ms:eureka清理服务list中失效服务的定时器的时间,默认60s
eviction-interval-timer-in-ms: 2000 # 清理间隔(一个定时器)(单位毫秒,默认是60*1000) #剔除失效服务时间(间隔2秒剔除一次失效服务)
注:如果一个服务已经超过最大的lease-expiration-duration-in-seconds时间还没有发送心跳,则该服务已经失效,但是如果清理失效服务的定时任务时间eviction-interval-timer-in-ms没到,这个服务仍不会被清除
3、加入Actuator,查看/health端点进行监控
参考网站:https://www.jianshu.com/p/734519d3c383
在pom.xml文件中引入依赖,可以访问health端点
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
<version>2.0.5.RELEASE</version>
</dependency>
配置endpoints属性
server:
port:8080
spring:
application:
name: cloud-police
#暴露端点 某些端口有访问权限,设置敏感端口成均可被访问
#2.0版本以后使用management.endpoints.web.exposure.include=*,以前使用endpoints.sensitive: false
#参考:https://blog.csdn.net/alinyua/article/details/80009435
#注意: * 在YAML中有特殊的含义,所以如果你想包含(或排除)所有的端点,一定要加引号'*'
management:
endpoints:
web:
exposure:
include: '*'
eureka:
instance:
#Eureka客户端向服务器发送心跳间隔,单位为秒,默认30s
lease-renewal-interval-in-seconds: 5
#Eureka服务器在收到最后一次心跳等待的时间上限,单位为秒,超出则剔除,默认为90s
lease-expiration-duration-in-seconds: 10
client:
#同步eureka注册中心的服务列表,默认30s(正式环境中就默认30s就行,很耗费资源的)
registry-fetch-interval-seconds: 5
#每隔5秒扫描一次本地实例,如果有变化向服务重新注册(默认30s)
instance-info-replication-interval-seconds: 5
metadata-map:
company-name: xxxCompany
serviceUrl:
defaultZone: http://localhost:8761/eureka,http://localhost:8762/eureka
#配置com.netflix包的日志级别
logging:
level:
com.netflix: DEBUG
配置完以后启动服务
访问localhost:8080/actuator/env 访问env端点可以看到服务全部环境变量已经配置
访问localhost:8080/actuator/health 访问health端点可以看到服务的状态为up状态
1、模拟数据库down了,查看health状态
Controller{
@Autowired
private DiscoveryClient discoveryClient;
@RequestMapping(value = "/list", method = RequestMethod.GET)
public String serviceInstance() {
List<String> services = discoveryClient.getServices();
for (String serviceInstanceId : services) {
List<ServiceInstance> instances =
discoveryClient.getInstances(serviceInstanceId);
System.out.println(instances);
}
return "success";
}
}
2、自定义健康指示器
package spring.cloud.spring_member.controller;
import org.springframework.boot.actuate.health.Health;
import org.springframework.boot.actuate.health.HealthIndicator;
import org.springframework.boot.actuate.health.Status;
import org.springframework.stereotype.Component;
/**
* 自定义健康指示器,模拟数据库down机,更改应用的down/up状态
*/
@Component
public class MyhealthIndicator implements HealthIndicator {
@Override
public Health health() {
if (MemberController.canVisitDb) {
return new Health.Builder(Status.UP).build();
} else {
return new Health.Builder(Status.DOWN).build();
}
}
}
3、自定义将康监测,每隔instance-info-replication-interval-seconds 秒扫描一下自定义监控检测并同步eureka
package spring.cloud.spring_member.controller;
import com.netflix.appinfo.HealthCheckHandler;
import com.netflix.appinfo.InstanceInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.actuate.health.Status;
import org.springframework.stereotype.Component;
/**
* 健康监测,每个instance-info-replication-interval-seconds 秒执行一次
* 根据health指示器,判断当前应用程序的状态,
* 然后告诉eureka当前应用程序的状态
* 同时还要配置配置文件 instance-info-replication-interval-seconds
* @Date 2019-06-15 19:05
*/
@Component
public class MyHealthCheckHandler implements HealthCheckHandler {
@Autowired
private MyhealthIndicator myhealthIndicator;
@Override
public InstanceInfo.InstanceStatus getStatus(InstanceInfo.InstanceStatus instanceStatus) {
Status status = myhealthIndicator.health().getStatus();
if(status.equals(Status.UP)){
return InstanceInfo.InstanceStatus.UP;
}else{
return InstanceInfo.InstanceStatus.DOWN;
}
}
}