系统结构
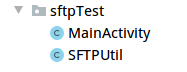
Step1 引入jar包
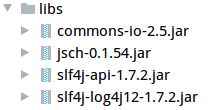
commons-net
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>1.4.1</version>
</dependency>
com.jcraft.jsch
<dependency>
<groupId>com.jcraft</groupId>
<artifactId>jsch</artifactId>
<version>0.1.54</version>
</dependency>
slf4j-api-1.7.2.jar
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.2</version>
</dependency>
slf4j-log4j12-1.7.2.jar
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
<version>1.7.2</version>
</dependency>
Step2 SFTPUtil.java
import android.annotation.SuppressLint;
import android.util.Log;
import com.jcraft.jsch.Channel;
import com.jcraft.jsch.ChannelSftp;
import com.jcraft.jsch.ChannelSftp.LsEntry;
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.JSchException;
import com.jcraft.jsch.Session;
import com.jcraft.jsch.SftpATTRS;
import com.jcraft.jsch.SftpException;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Iterator;
import java.util.Properties;
import java.util.Vector;
public class SftpUtil {
private String host;
private String username;
private String password;
private int port = Utils.SERVER_PORT;
private ChannelSftp sftp = null;
private Session sshSession = null;
public SftpUtil(String host, String username, String password) {
this.host = host;
this.username = username;
this.password = password;
}
public ChannelSftp connect() {
JSch jsch = new JSch();
try {
sshSession = jsch.getSession(username, host, port);
sshSession.setPassword(password);
Properties sshConfig = new Properties();
sshConfig.put("StrictHostKeyChecking", "no");
sshSession.setConfig(sshConfig);
sshSession.connect();
Channel channel = sshSession.openChannel("sftp");
if (channel != null) {
channel.connect();
} else {
LogUtils.logd("SFTP :: channel connecting failed");
}
sftp = (ChannelSftp) channel;
} catch (JSchException exception) {
exception.printStackTrace();
}
return sftp;
}
public void disconnect() {
if (this.sftp != null) {
if (this.sftp.isConnected()) {
this.sftp.disconnect();
LogUtils.logd("SFTP :: SFTP is closed already");
}
}
if (this.sshSession != null) {
if (this.sshSession.isConnected()) {
this.sshSession.disconnect();
LogUtils.logd("SFTP :: sshSession is closed already");
}
}
}
public boolean uploadFile(String remotePath, String remoteFileName,
String localPath, String localFileName) {
FileInputStream in = null;
try {
createDir(remotePath);
File file = new File(localPath + localFileName);
in = new FileInputStream(file);
sftp.put(in, remoteFileName);
return true;
} catch (FileNotFoundException exception) {
exception.printStackTrace();
} catch (SftpException exception) {
exception.printStackTrace();
} finally {
if (in != null) {
try {
in.close();
} catch (IOException exception) {
exception.printStackTrace();
}
}
}
return false;
}
public boolean bacthUploadFile(String remotePath, String localPath,
boolean del) {
try {
File file = new File(localPath);
File[] files = file.listFiles();
for (int i = 0; i < files.length; i++) {
if (files[i].isFile()
&& files[i].getName().indexOf("bak") == -1) {
synchronized (remotePath) {
if (this.uploadFile(remotePath, files[i].getName(),
localPath, files[i].getName())
&& del) {
deleteFile(localPath + files[i].getName());
}
}
}
}
return true;
} catch (Exception exception) {
exception.printStackTrace();
} finally {
this.disconnect();
}
return false;
}
public boolean deleteFile(String filePath) {
File file = new File(filePath);
if (!file.exists()) {
return false;
}
if (!file.isFile()) {
return false;
}
return file.delete();
}
public void createDir(String createpath) {
try {
if (isDirExist(createpath)) {
this.sftp.cd(createpath);
return;
}
String[] pathArry = createpath.split("/");
StringBuffer filePath = new StringBuffer("/");
for (String path : pathArry) {
if (path.equals("")) {
continue;
}
filePath.append(path + "/");
if (isDirExist(filePath.toString())) {
sftp.cd(filePath.toString());
} else {
sftp.mkdir(filePath.toString());
sftp.cd(filePath.toString());
}
}
this.sftp.cd(createpath);
} catch (SftpException exception) {
LogUtils.loge("STFP Error: 文件创建失败");
exception.printStackTrace();
}
}
@SuppressLint("DefaultLocale")
public boolean isDirExist(String directory) {
boolean isDirExistFlag = false;
try {
SftpATTRS sftpAttrs = sftp.lstat(directory);
isDirExistFlag = true;
return sftpAttrs.isDir();
} catch (Exception exception) {
if (exception.getMessage().toLowerCase().equals("no such file")) {
isDirExistFlag = false;
}
}
return isDirExistFlag;
}
public void deleteSftp(String directory, String deleteFile) {
try {
sftp.cd(directory);
sftp.rm(deleteFile);
} catch (Exception exception) {
exception.printStackTrace();
}
}
public void mkdirs(String path) {
File file = new File(path);
String fs = file.getParent();
file = new File(fs);
if (!file.exists()) {
file.mkdirs();
}
}
@SuppressWarnings("rawtypes")
public Vector listFiles(String directory) throws SftpException {
return sftp.ls(directory);
}
}
Step3 MainActivity.java
public void uploadTest() {
mSftp = new SftpUtil("IP", "用户名", "密码");
new Thread() {
@Override
public void run() {
super.run();
String localPath = 本地路径;
String remotePath = 服务器路径;
mSftp.connect();
if (mSftp.connect() != null) {
LogUtils.logd("Connection Successful");
mSftp.bacthUploadFile(remotePath, localPath, false);
if (mSftp.bacthUploadFile(remotePath, localPath, true)) {
LogUtils.logd("UPLOAD SUCCESSFUL!");
} else {
LogUtils.logd("UPLOAD FAILED!");
}
mSftp.disconnect();
} else {
LogUtils.logd("CONNECTION FAILED!");
}
}
}.start();
}