1.pom 依赖
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-client</artifactId>
<version>2.4.17</version>
</dependency>
2.resources 文件
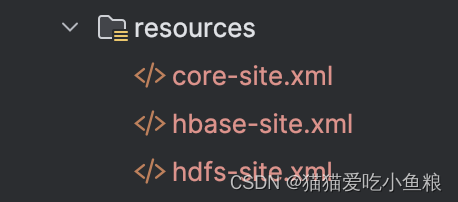
3.异步客户端代码示例
package com.xu.hbase;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.*;
import org.apache.hadoop.hbase.client.*;
import org.apache.hadoop.hbase.util.Bytes;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
public class HbaseUtilAsyncTest {
public static AsyncConnection getAsyncConnection(String zkHost
, String zkPort
, String scanTimeOut
, String threadMax
, String threadCore
, String rpcTimeout) throws ExecutionException, InterruptedException, TimeoutException {
final Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", zkHost);
configuration.set("hbase.zookeeper.property.clientPort", zkPort);
configuration.set("hbase.client.scanner.timeout.period", scanTimeOut);
configuration.set("hbase.hconnection.threads.max", threadMax);
configuration.set("hbase.hconnection.threads.core", threadCore);
configuration.set("hbase.rpc.timeout", rpcTimeout);
return ConnectionFactory.createAsyncConnection(configuration).get(10, TimeUnit.SECONDS);
}
public Boolean isTableExist(AsyncConnection connection, String tableName) throws ExecutionException, InterruptedException {
AsyncAdmin admin = connection.getAdmin();
return admin.tableExists(TableName.valueOf(tableName)).get();
}
public void createTable(AsyncConnection connection, String tableName, String... columnFamilys) throws ExecutionException, InterruptedException {
AsyncAdmin asyncAdmin = connection.getAdmin();
if (columnFamilys.length <= 0) {
System.out.println("请先设置列簇信息");
} else if (asyncAdmin.tableExists(TableName.valueOf(tableName)).get()) {
System.out.println("表 " + tableName + " 已经存在。");
} else {
TableDescriptorBuilder builder = TableDescriptorBuilder.newBuilder(TableName.valueOf(tableName));
List<ColumnFamilyDescriptor> families = new ArrayList<>();
for (String columnFamily : columnFamilys) {
final ColumnFamilyDescriptorBuilder cfdBuilder = ColumnFamilyDescriptorBuilder.newBuilder(ColumnFamilyDescriptorBuilder.of(columnFamily));
final ColumnFamilyDescriptor cfd = cfdBuilder.setMaxVersions(10).setInMemory(true).setBlocksize(8 * 1024)
.setScope(HConstants.REPLICATION_SCOPE_LOCAL).build();
families.add(cfd);
}
final TableDescriptor tableDescriptor = builder.setColumnFamilies(families).build();
asyncAdmin.createTable(tableDescriptor).get();
}
}
public void dropTable(AsyncConnection connection, String tableName) throws ExecutionException, InterruptedException {
AsyncAdmin asyncAdmin = connection.getAdmin();
if (asyncAdmin.tableExists(TableName.valueOf(tableName)).get()) {
asyncAdmin.disableTable(TableName.valueOf(tableName)).get();
asyncAdmin.deleteTable(TableName.valueOf(tableName)).get();
System.out.println("表 " + tableName + " 成功删除");
} else {
System.out.println("表 " + tableName + " 不存在");
}
}
public static void addOneRowData(AsyncConnection asyncConnection
, String tableName
, String rowKey
, String columnFamily
, String column
, String value) throws ExecutionException, InterruptedException {
AsyncTable<AdvancedScanResultConsumer> asyncTable = asyncConnection.getTableBuilder(TableName.valueOf(tableName))
.setMaxAttempts(3)
.setMaxRetries(3)
.setRpcTimeout(3, TimeUnit.SECONDS)
.setOperationTimeout(5, TimeUnit.SECONDS)
.setWriteRpcTimeout(3, TimeUnit.SECONDS)
.build();
//构建Put指令,rowKey必传
Put put = new Put(Bytes.toBytes(rowKey));
//指定版本时间戳
put.addColumn(Bytes.toBytes(columnFamily), Bytes.toBytes(column), System.currentTimeMillis(), Bytes.toBytes(value));
// put.addColumn(Bytes.toBytes(columnFamily), Bytes.toBytes(column), Bytes.toBytes(value));
asyncTable.put(put)
.handleAsync((unused, throwable) -> {
if (throwable != null) {
System.out.println("单条表数据插入异常" + throwable);
} else {
System.out.println("单条表数据插入成功");
}
return null;
})
.get();
}
public static void addMultiRowData(AsyncConnection asyncConnection
, String tableName
, List<Put> data) throws ExecutionException, InterruptedException {
AsyncTable<AdvancedScanResultConsumer> asyncTable = asyncConnection.getTableBuilder(TableName.valueOf(tableName))
.setMaxAttempts(3)
.setMaxRetries(3)
.setRpcTimeout(3, TimeUnit.SECONDS)
.setOperationTimeout(5, TimeUnit.SECONDS)
.build();
List<CompletableFuture<Void>> completableFutures = asyncTable.put(data);
for (CompletableFuture<Void> completableFuture : completableFutures) {
completableFuture.handleAsync((unused, throwable) -> {
if (throwable != null) {
System.out.println("批量插入中的一条数据插入失败=" + throwable);
} else {
System.out.println("批量插入中的一条数据插入完成");
}
return null;
})
.get();
}
}
public static boolean getRowData(AsyncConnection asyncConnection, String tableName, String row) throws ExecutionException, InterruptedException {
AsyncTable<AdvancedScanResultConsumer> asyncTable = asyncConnection.getTableBuilder(TableName.valueOf(tableName))
.setMaxAttempts(3)
.setMaxRetries(3)
.setRpcTimeout(3, TimeUnit.SECONDS)
.setOperationTimeout(5, TimeUnit.SECONDS)
.build();
// 按照 rowKey 查询
Get get = new Get(Bytes.toBytes(row));
return asyncTable.get(get)
.handleAsync((result, throwable) -> {
if (throwable != null) {
System.out.println("查询出现异常=" + throwable);
} else {
for (Cell cell : result.rawCells()) {
System.out.println("rowkey:" + Bytes.toString(CellUtil.cloneRow(cell)));
System.out.println(" 列簇:" + Bytes.toString(CellUtil.cloneFamily(cell)));
System.out.println(" 列:" + Bytes.toString(CellUtil.cloneQualifier(cell)));
System.out.println(" 值:" + Bytes.toString(CellUtil.cloneValue(cell)));
System.out.println(" 版本时间戳:" + cell.getTimestamp());
System.out.println("==========================");
}
}
return result.isEmpty();
})
.get();
}
public static HashMap<String, Boolean> getBatchRowData(AsyncConnection asyncConnection, String tableName, List<String> row) throws IOException, ExecutionException, InterruptedException, TimeoutException {
AsyncTable<AdvancedScanResultConsumer> asyncTable = asyncConnection.getTableBuilder(TableName.valueOf(tableName))
.setMaxAttempts(3)
.setMaxRetries(3)
.setRpcTimeout(3, TimeUnit.SECONDS)
.setOperationTimeout(5, TimeUnit.SECONDS)
.build();
ArrayList<Get> gets = new ArrayList<>();
for (String value : row) {
Get get = new Get(Bytes.toBytes(value));
gets.add(get);
}
List<CompletableFuture<Result>> completableFutures = asyncTable.get(gets);
HashMap<String, Boolean> res = new HashMap<>();
for (CompletableFuture<Result> completableFuture : completableFutures) {
completableFuture.handleAsync((result, throwable) -> {
if (throwable != null) {
System.out.println("查询出现异常=" + throwable);
} else {
if (!result.isEmpty()) {
for (Cell cell : result.rawCells()) {
res.put(Bytes.toString(CellUtil.cloneRow(cell)), true);
}
}
}
return null;
})
.get();
}
return res;
}
public static void main(String[] args) throws IOException, ExecutionException, InterruptedException, TimeoutException {
HbaseUtilAsyncTest baseDemo = new HbaseUtilAsyncTest();
AsyncConnection asyncConnection = baseDemo.getAsyncConnection("host"
, "port"
, "10000"
, "32"
, "8"
, "60000");
String tableName = "my_ns:Student";
String basicCF = "basicinfo";
String studyCF = "studyinfo";
//判断表是否存在
System.out.println(tableName + "=表是否存在=>" + baseDemo.isTableExist(asyncConnection, tableName));
//建表
baseDemo.createTable(asyncConnection, tableName, "basicinfo", "studyinfo");
//删除表
baseDemo.dropTable(asyncConnection, tableName);
//插入数据
//插入一条数据
baseDemo.addOneRowData(asyncConnection, tableName, "1005", basicCF, "age", "10");
baseDemo.addOneRowData(asyncConnection, tableName, "1005", basicCF, "name", "roy");
baseDemo.addOneRowData(asyncConnection, tableName, "1005", studyCF, "grade", "100");
baseDemo.addOneRowData(asyncConnection, tableName, "1006", basicCF, "age", "11");
baseDemo.addOneRowData(asyncConnection, tableName, "1006", basicCF, "name", "yula");
baseDemo.addOneRowData(asyncConnection, tableName, "1006", studyCF, "grade", "99");
//插入多条数据
ArrayList<Put> puts = new ArrayList<>();
puts.add(new Put(Bytes.toBytes("1007")).addColumn(Bytes.toBytes(basicCF), Bytes.toBytes("age"), Bytes.toBytes("12")));
puts.add(new Put(Bytes.toBytes("1007")).addColumn(Bytes.toBytes(basicCF), Bytes.toBytes("name"), Bytes.toBytes("sophia")));
puts.add(new Put(Bytes.toBytes("1007")).addColumn(Bytes.toBytes(studyCF), Bytes.toBytes("grade"), Bytes.toBytes("120")));
baseDemo.addMultiRowData(asyncConnection, tableName, puts);
//根据 rowKey 读取数据
//根据单个 rowKey 查询
boolean isRowKeyExist = baseDemo.getRowData(asyncConnection, tableName, "1005");
System.out.println("rowKey=1005 是否为空=>" + isRowKeyExist);
//根据多个 rowKey 查询
ArrayList<String> rows = new ArrayList<>();
rows.add("1006");
rows.add("1008");
HashMap<String, Boolean> batchRowData = baseDemo.getBatchRowData(asyncConnection, tableName, rows);
// 异步任务直接返回了,导致查询没拿到结果
for (Map.Entry<String, Boolean> entry : batchRowData.entrySet()) {
System.out.println("key=" + entry.getKey() + ",value=" + entry.getValue());
}
asyncConnection.close();
}
}