实现效果:
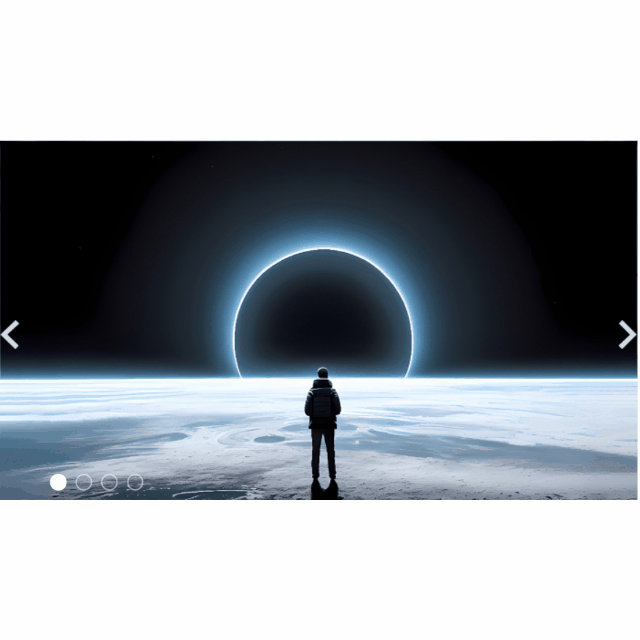
功能实现:
1.鼠标经过轮播图模块,左右按钮显示,离开隐藏左右按钮
2.点击右侧按钮一次,图片往左播放一张,左侧按钮同理。
3.图片播放的同时,下面的小圆圈模块也跟随一起变化。
4.鼠标不经过轮播图,轮播图会自动向右播放图片,经过轮播图之后停止自动播放图片。
代码实现:
html部分
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<link rel="stylesheet" href="index.css" />
<!-- animate.js要在index.js之前引入 -->
<script src="animate.js"></script>
<script src="index.js"></script>
</head>
<body>
<div class="main">
<div class="focus">
<!-- 左侧按钮 -->
<a href="javasrcipt:;" class="arrow-l">
<img src="lunbo/arrow-prev.png" alt="" />
</a>
<!-- 右侧按钮 -->
<a href="javasrcipt:;" class="arrow-r">
<img src="lunbo/arrow-next.png" alt="" />
</a>
<!-- 核心的滚动区域 -->
<ul>
<li>
<a href="javasrcipt:;"><img src="lunbo/00.png" alt="" /></a>
</li>
<li>
<a href="javasrcipt:;"><img src="lunbo/11.png" alt="" /></a>
</li>
<li>
<a href="javasrcipt:;"><img src="lunbo/22.png" alt="" /></a>
</li>
<li>
<a href="javasrcipt:;"><img src="lunbo/33.png" alt="" /></a>
</li>
</ul>
<!-- 小圆圈 -->
<ol class="circle"></ol>
</div>
</div>
</body>
</html>
css部分
index.css
* {
margin: 0;
padding: 0;
}
.main{
position: absolute;
width: 700px;
height: 394px;
/* 水平垂直居中 */
top: 50%;
left: 50%;
transform: translate(-350px,-200px);
}
.focus {
position: relative;
width: 700px;
height: 394px;
background: palegoldenrod;
overflow: hidden;
}
ul {
position: absolute;
width: 500%;
top: 0;
left: 0;
}
ul li {
list-style: none;
float: left;
}
.circle {
position: absolute;
bottom: 10px;
left: 50px;
}
.circle li {
float: left;
border: 2px solid rgba(255, 255, 255, 0.5);
width: 15px;
height: 15px;
margin: 0 5px;
list-style: none;
border-radius: 50%;
cursor: pointer;
}
.arrow-l {
display: none;
position: absolute;
top: 50%;
left: 0;
z-index: 2;
}
.arrow-r {
display: none;
position: absolute;
top: 50%;
right: 0;
z-index: 2;
}
.current{
background: rgb(252, 252, 252);
}
js部分
index.js
window.addEventListener('load', function () {
var arrow_l = document.querySelector(".arrow-l");
var arrow_r = document.querySelector(".arrow-r");
var focus = document.querySelector(".focus");
var ul = focus.querySelector('ul');
var ol = focus.querySelector('ol');
var focusWidth = focus.offsetWidth;
focus.addEventListener("mouseenter", function(){
arrow_l.style.display = 'block';
arrow_r.style.display = 'block';
// 自动停止定时轮播
clearInterval(timer);
timer = null;
})
focus.addEventListener("mouseleave", function(){
arrow_l.style.display = 'none';
arrow_r.style.display = 'none';
// 自动重启定时轮播
timer = setInterval(function () {
arrow_r.click();
}, 2000)
})
for(var i = 0; i < ul.children.length; i++){
// 动态创建li
var li = document.createElement("li");
// 1、li添加索引号index
li.setAttribute('index',i);
// 将li添加到ol
ol.appendChild(li);
// 2、点击的圆圈高亮,排他思想
li.addEventListener('click', function(){
for(var i = 0; i < ol.children.length; i++){
ol.children[i].className = '';
}
this.className = 'current';
// 3、点击圆圈得到对应的index
var index = this.getAttribute('index');
// num、index 赋值的作用是点击圆圈的同时改变num和index的默认值,防止出现bug
num = index;
circle = index;
// console.log(index);
// console.log(focusWidth);
// console.log(-index * focusWidth);
// 4、点击圆圈图片滚动
animate(ul, -index * focusWidth);
// 遇到的bug:index和focusWidth数值确实有,animate函数也没问题,但是动画没有滑动
// 原因是animate的obj参数中没有使用绝对定位
})
}
// 圆圈默认第一个
ol.children[0].className = 'current';
// 5、点击左右侧按钮图片无缝滚动
var num = 0;
var circle = 0;
var firstImg = ul.children[0].cloneNode(true); //深克隆(true)第一张图片,并添加至最后
ul.appendChild(firstImg);
// 右侧按钮
arrow_r.addEventListener('click',function(){
if(num == ul.children.length - 1){
// 走到最后一张图片是迅速定位到第一张图片
ul.style.left = 0;
num = 0; //要重置为0
}
num++;
animate(ul, -num * focusWidth);
// 点击按钮后圆圈跟着变化
circle++;
if(circle == ol.children.length){
circle = 0; //要重置为0
}
for(var i = 0; i < ol.children.length; i++){
ol.children[i].className = '';
}
ol.children[circle].className = 'current'
})
// 左侧按钮
arrow_l.addEventListener('click',function(){
if(num == 0){
// 走到第一张图片是迅速定位到最后一张图片
ul.style.left = -(ul.children.length - 1) * focusWidth + 'px';
num = ul.children.length - 1; //要重置为0
}
num--;
animate(ul, -num * focusWidth);
// 点击按钮后圆圈跟着变化
circle--;
if(circle < 0){
circle = ol.children.length - 1; //要重置为0
}
for(var i = 0; i < ol.children.length; i++){
ol.children[i].className = '';
}
ol.children[circle].className = 'current'
})
// 自动播放,加一个定时器
var timer = setInterval(function () {
arrow_r.click();
}, 2000)
})
animate.js
function animate(obj, target, callback) {
clearInterval(obj.timer); //缺少清除定时器可能会出现bug
obj.timer = setInterval(function () {
var step = (target - obj.offsetLeft) / 10; // 原理公式
step = step > 0 ? Math.ceil(step) : Math.floor(step);
if (obj.offsetLeft == target) {
clearInterval(obj.timer);
// if (callback) {
// callback();
// }
callback && callback();
}
//匀速动画
// obj.style.left = obj.offsetLeft + 1 + "px";
//缓动动画
obj.style.left = obj.offsetLeft + step + "px";
}, 10);
}