-
const 注意二:const 修饰的变量必须赋初值```
const name; // 错误,cons修饰的变量必须赋初值
const 有一个很好的应用场景,就是当我们引用第三方库时声明的变量,用 const 来声明可以避免未来不小心重命名而导致出现 bug:
const monent = require('moment')
======================================================================================
ES6 提供了更接近传统语言的写法,引入了 Class(类)这个概念。新的 class 写法让对象原型的写法更加清晰、更像面向对象编程的语法,也更加通俗易懂。
class Animal {
constructor(){
this.type = 'animal'
}
says(say){
console.log(this.type + ' says ' + say)
}
}
let animal = new Animal()
animal.says('hello') //animal says hello
class Cat extends Animal {
constructor(){
super()
this.type = 'cat'
}
}
let cat = new Cat()
cat.says('hello') //cat says hello
=======================================================================================
ES6 最常用的特性之一,用它写 function 比原来的写法简洁清晰很多。
function(i){
return i + 1;
} //ES5
i => i + 1 //ES6 或一个参数时省略为 i=>i+1
如果函数比较复杂,则需要用 `{}` 把代码包起来:
function(x, y) {
x++;
y--;
return x + y;
}
(x, y) => {
x++;
y--;
return x+y
}
```
* * *
JavaScript 语言的 this 对象一直是一个令人头痛的问题,在对象方法中使用 this,必须非常小心:
```
class Animal {
constructor(){
this.type = 'animal'
}
says(say){
setTimeout(function(){
console.log(this.type + ' says ' + say)
}, 1000)
}
}
var animal = new Animal()
animal.says('hi') //undefined says hi
```
运行上面的代码会报错,这是因为 setTimeout 中的 this 指向的是全局对象(闭包中的未定义变量,默认指向全局作用域)。所以为了让它能够正确的运行,传统的解决方法有两种:
1. **将 this 传给 self,再用 self 来指代 this**
```
says(say){
var self = this;
setTimeout(function(){
console.log(self.type + ' says ' + say)
}, 1000)
```
2. **使用 `bind(this)`**
```
says(say){
setTimeout(function(){
console.log(this.type + ' says ' + say)
}.bind(this), 1000)
```
现在有了箭头函数,就不需要这么麻烦了:
```
class Animal {
constructor(){
this.type = 'animal'
}
says(say){
setTimeout( () => {
console.log(this.type + ' says ' + say)
}, 1000)
}
}
var animal = new Animal()
animal.says('hi') //animal says hi
```
使用箭头函数时,函数体内的 this 对象,就是定义时所在的对象,而不是使用时所在的对象;原因是**箭头函数根本没有自己的 this,它的 this 是继承外面的,因此内部的 this 就是外层代码块的this**。
[](https://gitee.com/vip204888/java-p7)模版字符串(template string)
=========================================================================================
传统的使用 `+` 和 `"` 字符串拼接:
```
$("#result").append(
"There are <b>" + basket.count + "</b> " +
"items in your basket, " +
"<em>" + basket.onSale +
"</em> are on sale!"
```
ES6 的新特性模板字符串:使用 `${}` 来引用变量
```
$("#result").append(`
There are <b>${basket.count}</b> items
in your basket, <em>${basket.onSale}</em>
are on sale!
`);
```
[](https://gitee.com/vip204888/java-p7)结构(destructuring)
====================================================================================
ES6 允许按照一定模式,从数组和对象中提取值,对变量进行赋值,这被称为 **解构**
ES5 的写法:
```
let cat = 'ken'
let dog = 'lili'
let zoo = {cat: cat, dog: dog}
console.log(zoo) //Object {cat: "ken", dog: "lili"}
```
**ES6 :属性简写**
```
let cat = 'ken'
let dog = 'lili'
let zoo = {cat, dog}
console.log(zoo) //Object {cat: "ken", dog: "lili"}
```
**ES6:解构赋值**
```
let dog = {type: 'animal', many: 2}
let { type, many} = dog //import { validateUserName } from '@/utils/validate'
console.log(type, many) //animal 2
```
[](https://gitee.com/vip204888/java-p7)default、rest
===============================================================================
ES5 指定默认值:
```
function animal(type){
type = type || 'cat'
console.log(type)
}
animal()
```
ES6 指定默认值:
```
function animal(type = 'cat'){
console.log(type)
}
animal()
```
ES6 的 rest 语法:
```
function animals(...types){
console.log(types)
}
animals('cat', 'dog', 'fish') //["cat", "dog", "fish"]
```
> 如果不用 ES6 的话,我们则得使用 ES5 的 arguments
[](https://gitee.com/vip204888/java-p7)ES6 module
=============================================================================
[](https://gitee.com/vip204888/java-p7)import、export
--------------------------------------------------------------------------------
ES6 之前为解决 JavaScript 的模块化问题,常常利用一些第三方方案,主要有 **CommonJS**(服务器端)、**AMD**(浏览器端,如 require.js)
ES6 的 module 实现非常简单,可以成为服务器和浏览器通用的模块解决方案。
require.js 解决方案:
```
//content.js
define('content.js', function(){
return 'A cat';
})
# 更多:Java进阶核心知识集
包含:JVM,JAVA集合,网络,JAVA多线程并发,JAVA基础,Spring原理,微服务,Zookeeper,Kafka,RabbitMQ,Hbase,MongoDB,Cassandra,设计模式,负载均衡,数据库,一致性哈希,JAVA算法,数据结构,加密算法,分布式缓存等等
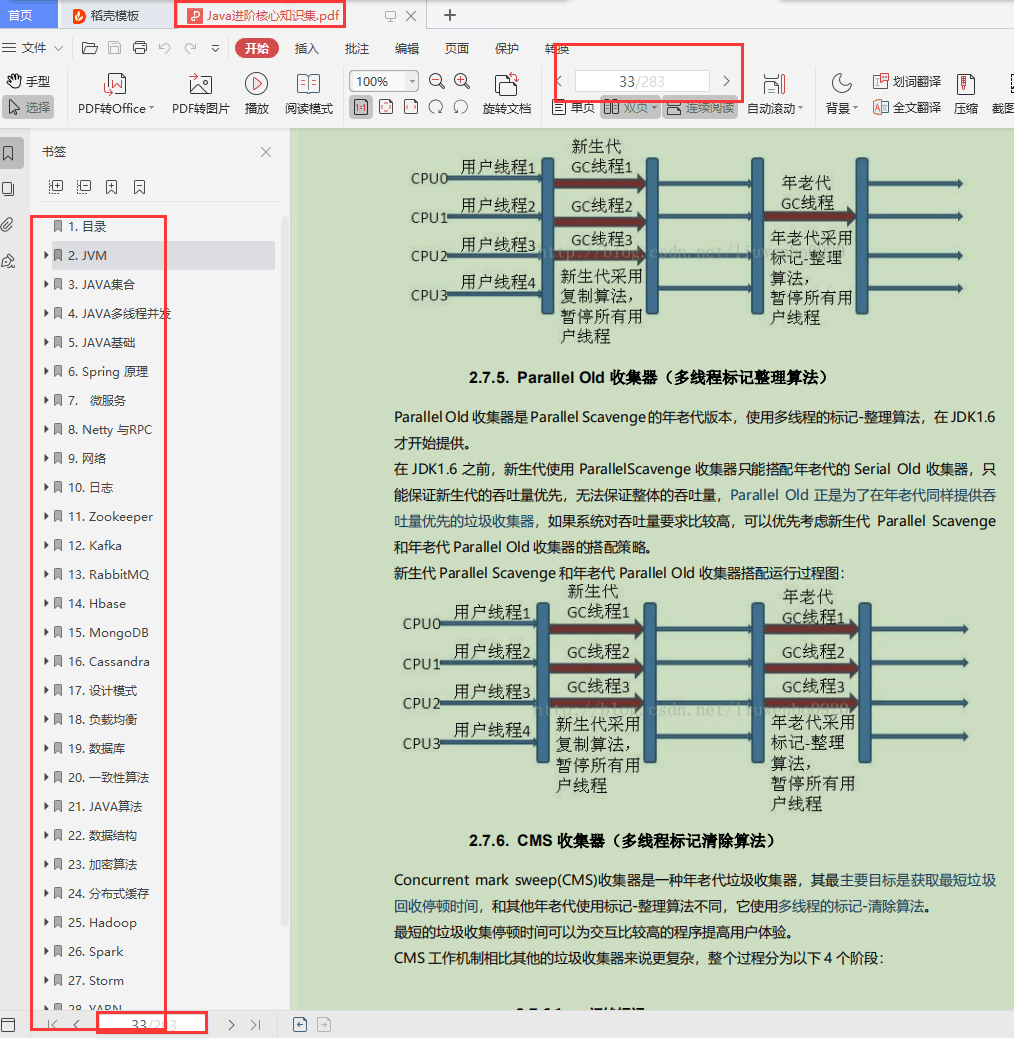
> **[点击免费领取我的学习笔记:学习视频+大厂面试真题+微服务+MySQL+Java+Redis+算法+网络+Linux+Spring全家桶+JVM+学习笔记图](https://gitee.com/vip204888/java-p7)**
# 高效学习视频
的模块解决方案。
require.js 解决方案:
```
//content.js
define('content.js', function(){
return 'A cat';
})
# 更多:Java进阶核心知识集
包含:JVM,JAVA集合,网络,JAVA多线程并发,JAVA基础,Spring原理,微服务,Zookeeper,Kafka,RabbitMQ,Hbase,MongoDB,Cassandra,设计模式,负载均衡,数据库,一致性哈希,JAVA算法,数据结构,加密算法,分布式缓存等等
[外链图片转存中...(img-6Yo0x3ik-1628510874855)]
> **[点击免费领取我的学习笔记:学习视频+大厂面试真题+微服务+MySQL+Java+Redis+算法+网络+Linux+Spring全家桶+JVM+学习笔记图](https://gitee.com/vip204888/java-p7)**
# 高效学习视频
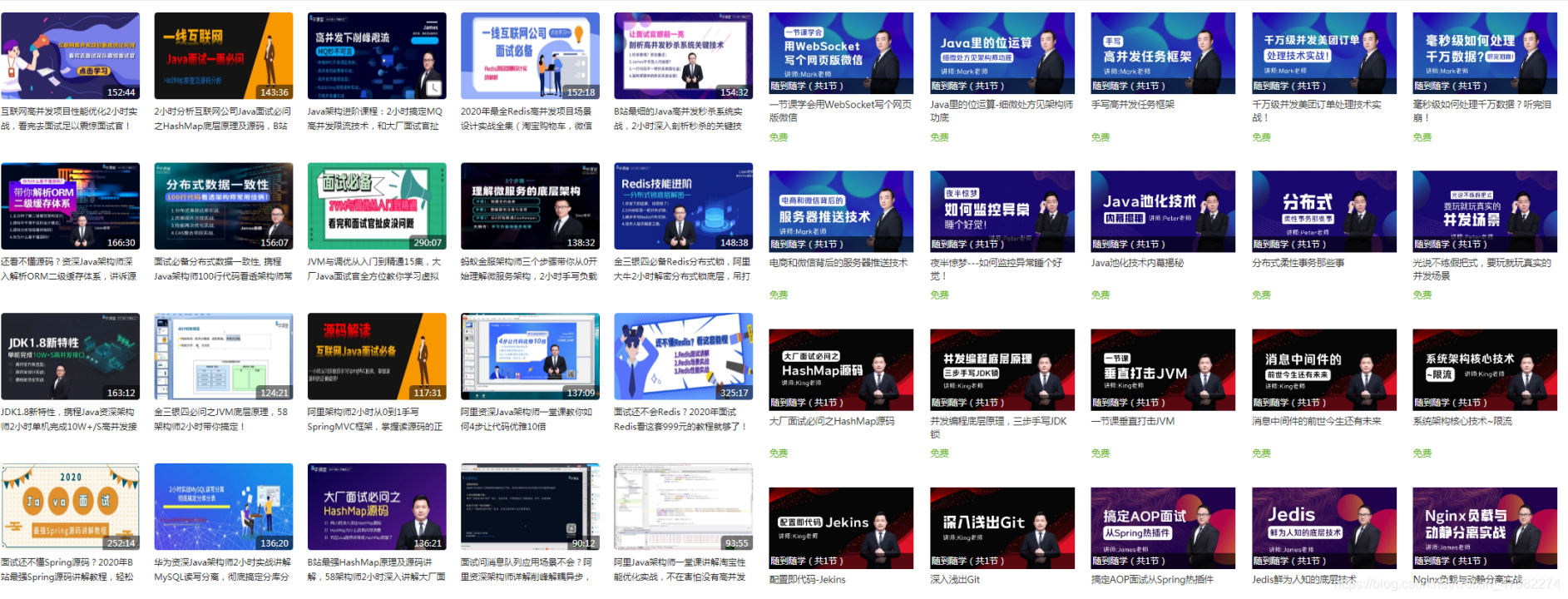