1.思维导图
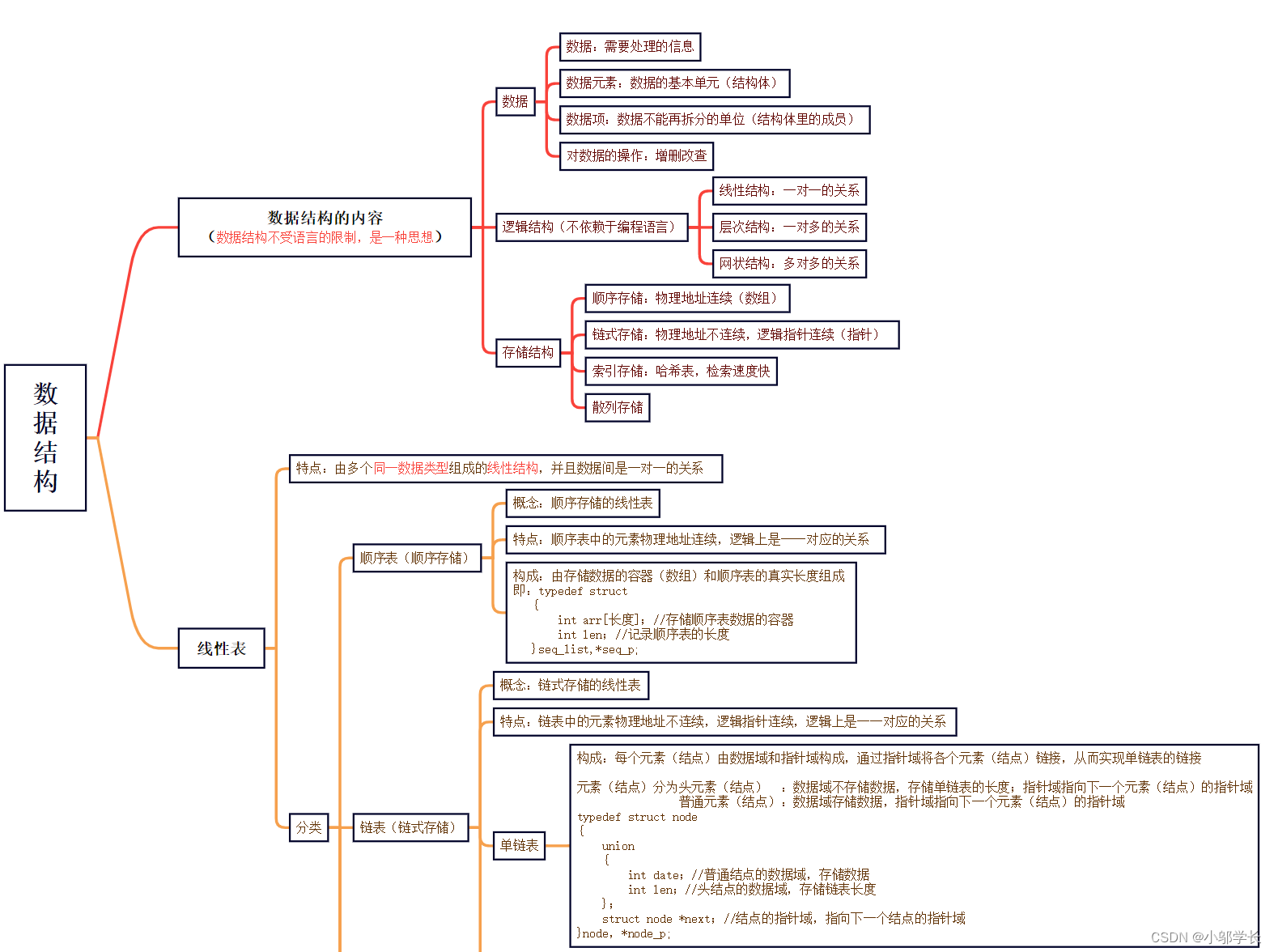
2.创建单链表结构体
typedef int datatype;
typedef struct node
{
union
{
datatype data;//普通及结点数据域,存储数据
int len;//头结点数据域,记录链表长度
};
struct node* next;//结点的指针域,指向下一个结点的指针域
}node,*node_p;
3.创建单链表(头结点)
/*----------创建单链表(头结点)-----------*/
node_p create_link_list(void)
{
node_p Head_node=(node_p)malloc(sizeof(node));
if(Head_node==NULL)
{
printf("空间申请失败\n");
return NULL;
}
Head_node->len=0;
Head_node->next=NULL;
return Head_node;
}
4.创建单链表的新节点
/*---------------创建新节点----------------*/
node_p create_link_node(datatype data)
{
node_p New_node=(node_p)malloc(sizeof(node));
if(New_node==NULL)
{
printf("空间申请失败\n");
return NULL;
}
New_node->data=data;
New_node->next=NULL;
return New_node;
}
5.单链表的判空
/*-------------单链表的判空---------------*/
int empty_link_list(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return -1;
}
return Head_node->next==NULL?1:0;
}
6.单链表的头插
/*-------------单链表的头插---------------*/
void insert_head_link_list(node_p Head_node,datatype newdata)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
node_p New_node=create_link_node(newdata);
New_node->next=Head_node->next;
Head_node->next=New_node;
Head_node->len++;
}
7.单链表的头删
/*-------------单链表的头删---------------*/
void delete_head_link_list(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(empty_link_list(Head_node))
{
printf("单链表已空,无法头删\n");
return;
}
node_p Delete_node=Head_node->next;
Head_node->next=Delete_node->next;
free(Delete_node);
Head_node->len--;
}
8.单链表的尾插
/*-------------单链表的尾插---------------*/
void insert_tail_link_list(node_p Head_node,datatype newdata)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
node_p Tail_node=Head_node->next;
while(Tail_node->next!=NULL)
{
Tail_node=Tail_node->next;
}
node_p New_node=create_link_node(newdata);
New_node->next=NULL;
Tail_node->next=New_node;
Head_node->len++;
}
9.单链表的尾删
/*-------------单链表的尾删---------------*/
void delete_tail_link_list(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(empty_link_list(Head_node))
{
printf("单链表已空,无法头删\n");
return;
}
node_p Penultimate_node=Head_node;
while(Penultimate_node->next->next!=NULL)
{
Penultimate_node=Penultimate_node->next;
}
node_p Delete_node=Penultimate_node->next;
Penultimate_node->next=NULL;
free(Delete_node);
Head_node->len--;
}
10.单链表的按位置插入
/*----------单链表的按位置插入------------*/
void insert_pos_link_list(node_p Head_node,int pos,datatype newdata)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(pos<1||pos>Head_node->len+1)
{
printf("超出插入范围,无法插入\n");
return;
}
node_p Pos_node=Head_node;
int i;
for(i=1;i<pos;i++)
{
Pos_node=Pos_node->next;
}
node_p New_node=create_link_node(newdata);
New_node->next=Pos_node->next;
Pos_node->next=New_node;
Head_node->len++;
}
11.单链表的按位置删除
/*----------单链表的按位置删除------------*/
void delete_pos_link_list(node_p Head_node,int pos)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(empty_link_list(Head_node))
{
printf("链表已空,无法按位置删除\n");
return;
}
if(pos<1||pos>Head_node->len)
{
printf("超出删除范围,无法按位置删除\n");
return;
}
node_p Pos_node=Head_node;
int i;
for(i=1;i<pos;i++)
{
Pos_node=Pos_node->next;
}
node_p Delete_node=Pos_node->next;
Pos_node->next=Delete_node->next;
free(Delete_node);
Head_node->len--;
}
12.单链表的按值查找并返回元素
/*-------单链表按值查找返回元素位置-------*/
void search_data_link_list(node_p Head_node,datatype data)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(empty_link_list(Head_node))
{
printf("链表已空,无法按值查找并返回元素位置\n");
return;
}
int i,j=0;
int arr[100];
node_p Data_node=Head_node->next;
for(i=1;i<=Head_node->len;i++)
{
if(Data_node->data!=data)
{
Data_node=Data_node->next;
}
else
{
arr[j]=i;
Data_node=Data_node->next;
j++;
}
}
if(j==0)
{
printf("链表中无此值\n");
return;
}
printf("所查值对应的结点是\n");
for(i=0;i<j;i++)
{
printf("第%d个结点\t",arr[i]);
}
printf("\n");
}
13.单链表的按位置查找并返回其值
/*-------单链表按位置查找返回值-------*/
datatype search_pos_link_list(node_p Head_node,int pos)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return -1;
}
if(empty_link_list(Head_node))
{
printf("链表已空,无法按位置查找并返回值\n");
return -2;
}
if(pos<1||pos>Head_node->len)
{
printf("超出查找范围,无法按位置查找并返回值\n");
return -3;
}
int i;
node_p Pos_node=Head_node->next;
for(i=1;i<pos;i++)
{
Pos_node=Pos_node->next;
}
return Pos_node->data;
}
14.单链表的逆置
/*-------------单链表的逆置---------------*/
/*--------------数组循环法----------------*/
void invert_link_list_1(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(empty_link_list(Head_node))
{
printf("链表已空,无法逆置\n");
return;
}
if(Head_node->next->next==NULL)
{
printf("结点只有一个,不需要逆置\n");
return;
}
node_p arr[Head_node->len];
node_p Segment_node=Head_node->next;
int i;
for(i=0;i<Head_node->len;i++)
{
arr[i]=Segment_node;
Segment_node=Segment_node->next;
}
Head_node->next=arr[Head_node->len-1];
for(i=Head_node->len-1;i>0;i--)
{
arr[i]->next=arr[i-1];
}
arr[0]->next=NULL;
}
/*-------------单链表的逆置---------------*/
/*----------------迭代法------------------*/
void invert_link_list_2(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(empty_link_list(Head_node))
{
printf("链表已空,无法逆置\n");
return;
}
if(Head_node->next->next==NULL)
{
printf("结点只有一个,不需要逆置\n");
return;
}
node_p Start_node=NULL;
node_p Middle_node=Head_node->next;
node_p End_node=Head_node->next->next;
while(1)
{
Middle_node->next=Start_node;
if(End_node==NULL)
{
break;
}
Start_node=Middle_node;
Middle_node=End_node;
End_node=End_node->next;
}
Head_node->next=Middle_node;
}
/*-------------单链表的逆置---------------*/
/*----------------头插法------------------*/
void invert_link_list_3(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(empty_link_list(Head_node))
{
printf("链表已空,无法逆置\n");
return;
}
if(Head_node->next->next==NULL)
{
printf("结点只有一个,不需要逆置\n");
return;
}
node_p First_node=Head_node->next;
node_p New_first_node=NULL;
node_p Temp_node=NULL;
while(First_node!=NULL)
{
Temp_node=First_node;
First_node=Temp_node->next;
Temp_node->next=New_first_node;
New_first_node=Temp_node;
}
Head_node->next=New_first_node;
}
/*-------------单链表的逆置---------------*/
/*--------------直接逆置法----------------*/
void invert_link_list_4(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(empty_link_list(Head_node))
{
printf("链表已空,无法逆置\n");
return;
}
if(Head_node->next->next==NULL)
{
printf("结点只有一个,不需要逆置\n");
return;
}
node_p First_node=Head_node->next;
node_p Begin_node=First_node;
node_p End_node=Begin_node->next;
while(End_node!=NULL)
{
Begin_node->next=End_node->next;
End_node->next=First_node;
First_node=End_node;
End_node=Begin_node->next;
}
Head_node->next=First_node;
}
15.单链表的清空
/*-------------单链表的清空---------------*/
void clear_link_list(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
if(empty_link_list(Head_node))
{
printf("链表已空,不能重复清空\n");
return;
}
int i;
node_p Clear_node=Head_node->next;
for(i=1;i<=Head_node->len;i++)
{
Clear_node->data=0;
Clear_node=Clear_node->next;
}
Head_node->len=0;
}
16.单链表的释放
/*-------------单链表的释放---------------*/
void free_link_list(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
clear_link_list(Head_node);
node_p Free_node;
while(Head_node!=NULL)
{
Free_node=Head_node;
Head_node=Head_node->next;
free(Free_node);
}
}//需要在主函数中手动将Head_Node手动指空
17.单链表的输出
/*-------------单链表的输出---------------*/
void show_link_list(node_p Head_node)
{
if(Head_node==NULL)
{
printf("参数为空,请检查\n");
return;
}
node_p first_node=Head_node->next;
while(first_node!=NULL)
{
printf("%d\n",first_node->data);
first_node=first_node->next;
}
}