一、实验内容
1、请编写重载方法,分别实现两个int类型和两个float类型的数据的求和操作。
提示:方法名:sum
输出描述:
测试int类型用的数值为2,4
测试float类型用的数值为1.5,3.5
输出案例:
int类型的方法求和结果为:6
float类型的方法求和结果为:5.0
public class test301 {
public static void main(String[] args)
{
System.out.println("int类型的方法求和结果为:"+sum(2,4));
System.out.println("float类型的方法求和结果为:"+sum(1.5f,3.5f));
}
public static int sum(int a,int b)
{
return a+b;
}
public static float sum(float a,float b)
{
return a+b;
}
}
2、编写一个类,类中定义一个静态方法,用于求两个整数的和。 请按照以下要求设计一个测试类Test,并进行测试。 要求如下:
1)Test类中有一个静态方法get(int a,int b)该方法用户返回参数a、b两个整数的和;
2)在main()方法中调用get方法并输出计算结果。
输入案例:2 3
输出案例:5
import java.util.Scanner;
public class test302 {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
int a=sc.nextInt();
int b=sc.nextInt();
System.out.println(get(a,b));
}
static int get(int a,int b) //静态方法
{
return a+b;
}
}
3、使用Java类设计一个计算器,计算机具备操作数1,、操作数2、操作符三个属性,还具备计算功能。要求创建计算器对象的时候不能直接对计算器的属性赋值,要把属性都封装起来。 请按照以下要求设计一个计算器类Calculator。 要求如下:
1) 计算器类有三个属性,分别是操作数1(num1)、操作数2(num2)、操作符(option)。
2) 使用封装的思想将所有属性私有化,并对外界提供共有的访问getter和setter方法。
3) 计算器类中有一个计算的方法count(),用于执行加、减、乘、除运算。
4) 在测试类的 main()方法中,创建Calculator的实例对象,并通过键盘输入为num1和num2赋值,然后调用count()方法执行相应的运算。
输入案例:
1 2
输出案例:
num1+num2=3
num1-num2=-1
num1*num2=2
num1/num2=0
import java.util.Scanner;
public class test303{
public static void main(String[] args)
{
Scanner sc=new Scanner(System.in);
int num1=sc.nextInt();
int num2=sc.nextInt();
Calculator c=new Calculator();
c.setNum1(num1);
c.setNum2(num2);
c.count();
}
static class Calculator
{
private int num1;
private int num2;
private char option;
public void setNum1(int num1)
{
this.num1=num1;
}
public void setNum2(int num2)
{
this.num2=num2;
}
public int getNum1(int num1)
{
return num1;
}
public int getNum2(int num2){
return num2;
}
public char getOption(char o) {
return 0;
}
public void count(){
System.out.println("num1+num2="+(num1+num2));
System.out.println("num1-num2="+(num1-num2));
System.out.println("num1*num2="+(num1*num2));
System.out.println("num1/num2="+(num1/num2));
}
}
}
4、设计一个圆类Circle,具有属性:圆心坐标x和y及圆半径r,除具有设置及获取属性的方法外,还具有计算周长的方法perimeter()和计算面积的方法area()。再设计一个圆柱体类Cylinder,Cylinder继承自Circle,增加了属性:高度h,增加了设置和获取h的方法、计算表面积的方法area()和计算体积的方法volume()。
在测试类中,创建Cylinder的类对象,显示其所有属性,计算并显示其表面积和体积。
输入描述:按照 (x y r h)的顺序输入数据。
输入案例:1 2 3 4
输出案例:
The center coordinate of the cylinder is (1.0,2.0),
the radius is 3.0, the height is 4.0,
the surface area is 131.88, the volume is 113.03999999999999
import java.util.Scanner;
public class test304 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double x = sc.nextDouble();
double y = sc.nextDouble();
double r = sc.nextDouble();
double h = sc.nextDouble();
Cylinder cylinder = new Cylinder(x, y, r, h);
cylinder.show();
System.out.println("the radius is " + r + ", the height is " + h+",");
System.out.println("the surface area is " + cylinder.area() + ", the volume is " + cylinder.volume());
}
}
class Circle{
double x;
double y;
double r;
Circle(double x,double y,double r){
this.x=x;
this.y=y;
this.r=r;
}
public void setX(double x){
this.x=x;
}
public void setY(double y){
this.y=y;
}
public void setR(double r){
this.r=r;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public double getR() {
return r;
}
public double area(){
return 3.14*r*r;
}
public double perimeter(){
return 2*r* 3.14;
}
public void show(){
System.out.println("The center coordinate of the cylinder is "+"("+x+","+y+"),");
// System.out.print("the radius is "+r+", the height is "+h);
}
// The center coordinate of the cylinder is (1.0,2.0),
// the radius is 3.0, the height is 4.0,
}
class Cylinder extends Circle {
double h;
Cylinder(double x, double y, double r, double h) {
super(x, y, r);
this.h = h;
}
public void setH(double h) {
this.h = h;
}
public double getH() {
return h;
}
public double area() {
return perimeter() * h + super.area() * 2;
}
public double volume() {
return super.area() * h;
}
}
5、完成下面父类及子类的声明:
(1)声明Student类。
属性包括学号(number)、姓名(name)、英语成绩(englishScore)、数学成绩(mathScore)、计算机成绩(computerScore)和总成绩(totalScore)。
方法包括构造方法、get方法、set方法、compare方法(比较两个学生的总成绩,结果分大于、小于、等于)、sum方法(计算总成绩)和testScore方法(计算评测成绩)。
注:评测成绩可以取三门课成绩的平均分,另外任何一门课的成绩的改变都需要对总成绩进行重新计算。
(2)声明StudentXW(学习委员)类为Student类的子类。
在StudentXW类中增加责任(responsibility)属性,并重写testScore方法(计算评测成绩,评测成绩=三门课的平均分+3)。
(3)声明StudentBZ(班长)类为Student类的子类。
在StudentBZ类中增加责任(responsibility)属性,并重写testScore方法(计算评测成绩,评测成绩=三门课的平均分+5)
(4)声明测试类,生成2个Student类、1个StudentXW类及1个StudentBZ类对象,并分别计算它们的评测成绩,以及互相进行总成绩的对比。
输入描述:按照先2个Student类(number,name,englishScore,mathScore,computerScore),
1个StudentXW类(number,name,englishScore,mathScore,computerScore,responsibility),
1个StudentBZ类(number,name,englishScore,mathScore,computerScore,responsibility)对象输入数据。
输入案例:
101, Lisi, 70, 70, 70
101, Zhaoliu, 70, 70, 70
102, Zhangsan, 90, 90, 90, Receive homework
103, Wangwu, 100, 100, 100, Lead everyone
输出案例:
Lisi's evaluation score is 70.0
Zhangsan's evaluation score is 93.0
Wangwu's evaluation score is 105.0
Zhaoliu's evaluation score is 70.0
Zhangsan's total score is higher than Lisi
Zhangsan's total score is lower than Wangwu
Lisi's total score is equal to Zhaoliu
package com.HelloWorld;
import java.util.Scanner;
public class test305 {
public static void main(String[] args) {
Scanner cin = new Scanner(System.in);
String s = cin.nextLine();
String[] nums = s.split(",");
int number = Integer.parseInt(nums[0]);
String name = String.valueOf(nums[1]);
double englishScore = Double.parseDouble(nums[2]);
double mathScore = Double.parseDouble(nums[3]);
double computerScore = Double.parseDouble(nums[4]);
Student stu1 = new Student(number,name,englishScore,mathScore,computerScore);
s=cin.nextLine();
nums = s.split(",");
number = Integer.parseInt(nums[0]);
name = String.valueOf(nums[1]);
englishScore = Double.parseDouble(nums[2]);
mathScore = Double.parseDouble(nums[3]);
computerScore = Double.parseDouble(nums[4]);
Student stu2 = new Student(number,name,englishScore,mathScore,computerScore);
s=cin.nextLine();
nums = s.split(",");
number = Integer.parseInt(nums[0]);
name = String.valueOf(nums[1]);
englishScore = Double.parseDouble(nums[2]);
mathScore = Double.parseDouble(nums[3]);
computerScore = Double.parseDouble(nums[4]);
String responsibility = String.valueOf(nums[5]);
Student stu3 = new StudentXW(number,name,englishScore,mathScore,computerScore,responsibility);
s=cin.nextLine();
nums = s.split(",");
number = Integer.parseInt(nums[0]);
name = String.valueOf(nums[1]);
englishScore = Double.parseDouble(nums[2]);
mathScore = Double.parseDouble(nums[3]);
computerScore = Double.parseDouble(nums[4]);
responsibility = String.valueOf(nums[5]);
Student stu4 = new StudentBZ(number,name,englishScore,mathScore,computerScore,responsibility);
System.out.println(stu1.name+"'s evaluation score is "+stu1.testScore());
System.out.println(stu3.name+"'s evaluation score is "+stu3.testScore());
System.out.println(stu4.name+"'s evaluation score is "+stu4.testScore());
System.out.println(stu2.name+"'s evaluation score is "+stu2.testScore());
System.out.println();
stu3.compare(stu1);
stu3.compare(stu4);
stu1.compare(stu2);
}
}
class Student {
int number;
String name;
double englishScore;
double mathScore;
double computerScore;
double totalScore;
public Student(int number, String name, double englishScore, double mathScore, double computerScore) {
this.number = number;
this.name = name;
this.englishScore = englishScore;
this.mathScore = mathScore;
this.computerScore = computerScore;
this.totalScore = englishScore + mathScore + computerScore;
}
public int getNumber() {
return number;
}
public void setNumber(int number) {
this.number = number;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getEnglishScore() {
return englishScore;
}
public void setEnglishScore(int englishScore) {
this.englishScore = englishScore;
}
public double getMathScore() {
return mathScore;
}
public void setMathScore(int mathScore) {
this.mathScore = mathScore;
}
public double getComputerScore() {
return computerScore;
}
public void setComputerScore(int computerScore) {
this.computerScore = computerScore;
}
public double getTotalScore() {
return totalScore;
}
public void setTotalScore(int totalScore) {
this.totalScore = totalScore;
}
public void compare(Student name ) {
if (this.totalScore > name.totalScore)//stu1.name
System.out.println(this.name + "'s total score is higher than " + name.name);
else if (this.totalScore == name.totalScore)
System.out.println(this.name + "'s total score is equal to " + name.name);
else
System.out.println(this.name + "'s total score is lower than " + name.name);
}
public double testScore() {
double t = this.englishScore + this.computerScore + this.mathScore;
return t / 3;
}
}
class StudentXW extends Student{
String responsibility;
public StudentXW(int number,String name,double englishScore,double mathScore,double computerScore,String responsibility) {
super(number, name, englishScore, mathScore, computerScore);
this.responsibility=responsibility;
}
public double testScore() {
return super.testScore()+3;
}
}
class StudentBZ extends Student{
String responsibility;
public StudentBZ(int number,String name,double englishScore,double mathScore,double computerScore,String responsibility) {
super(number, name, englishScore, mathScore, computerScore);
this.responsibility=responsibility;
}
public double testScore() {
// TODO 自动生成的方法存根
return super.testScore()+5;
}
}
二、实验结果与分析(包括:输入数据、输出数据、程序效率及正确性等)(此处写清题号与其答案,可截图)
程序运行结果截图如下:
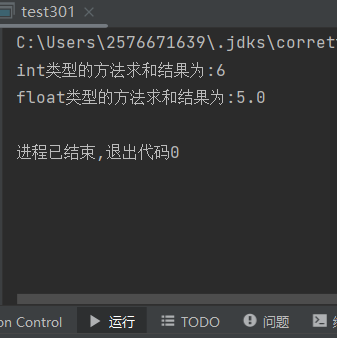
程序运行结果截图如下:
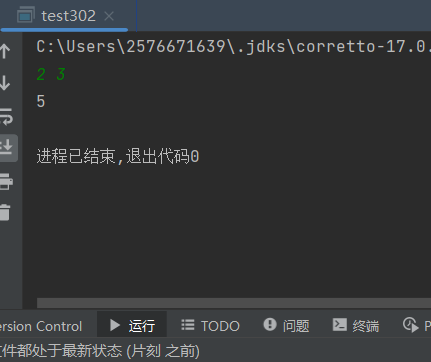
程序运行结果截图如下:
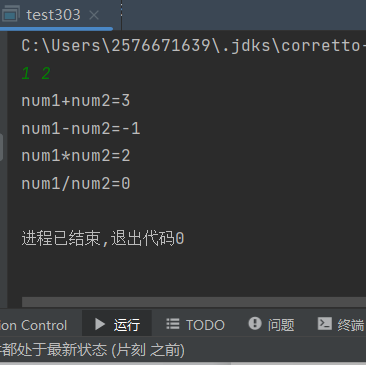
程序运行结果截图如下:
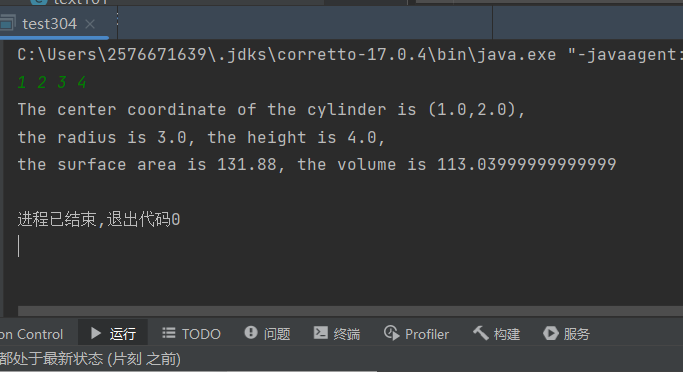
程序运行结果截图如下:
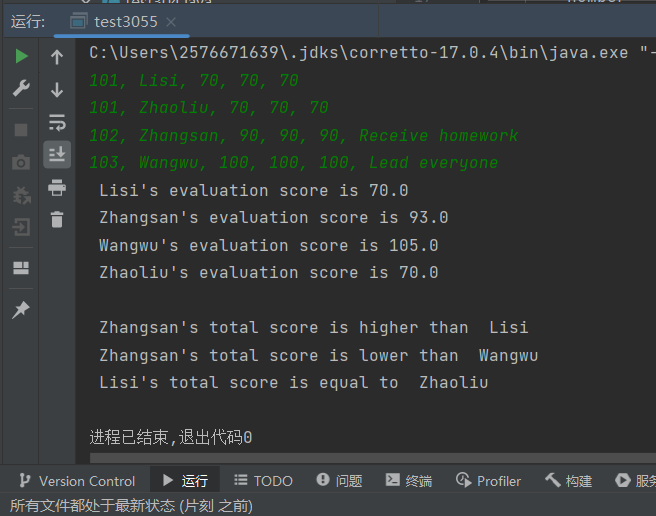
三、问题与讨论
1.JAVA中split函数的用法
只写经常使用的,并不完整。
1.基本用法,将字符串按照指定字符串进行分割,例如:
public class Main {
public static void main(String[] args) {
String ss = "abcabcdefg";
String[] split = ss.split("bc");
for(String st:split){
System.out.println(st);
}
System.out.println("分隔后字符串数组长度为");
System.out.println(split.length);
}
}
2.需要使用多个字符进行分割:使用split("[]"),其中[]里面存放需要分割的字符,注意,现在是按照字符来分割,例如:
public class Main {
public static void main(String[] args) {
String ss = "abcabcdefg";
String[] split = ss.split("[bc]");
for(String st:split){
System.out.println(st);
}
System.out.println("分隔后字符串数组长度为");
System.out.println(split.length);
}
}
用法其实上面两条差不多就可以了,重要的是一些特殊情况:
情况1:字符串中有连续的分割符,例如2中,b也是分割符c也是分隔符,那么在分割bc时会产生一个空字符(""),n个连续的分割符会产生n-1个空字符。下面是调试时的信息:
可以看到中间产生了空字符。
情况2:分割符出现在首部,那么出现几个分割符,就有多少个空字符。例如
public class Main {
public static void main(String[] args) {
String ss = "aaabcabcdefg";
String[] split = ss.split("[a]");
for(String st:split){
System.out.println(st);
}
System.out.println("分隔后字符串数组长度为");
System.out.println(split.length);
}
}
情况3:有些正则表达式,这是一种特殊情况,如,*等符号需要添加转义字符\。
解决方法:
针对出现空字符,可以写一个遍历,长度为0的过滤掉。
for(String st:split){
if(st.length()>0){
list.add(st);
}
}
针对转义字符,编译器会报错,就试试添加\。
2.例子
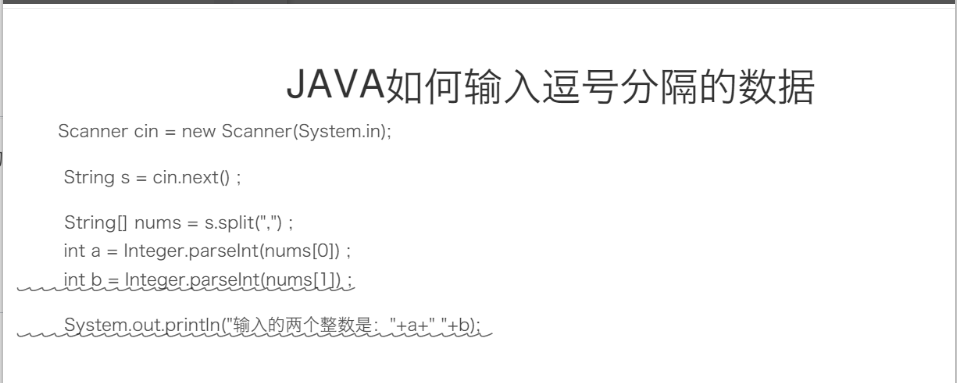
3.例子:import java.util.Scanner;
public class test3055 {
public static void main(String[] args) {
Scanner cin = new Scanner(System.in);
String s = cin.nextLine();
String[] nums = s.split(",");
int number = Integer.parseInt(nums[0]);
String name = String.valueOf(nums[1]);
double englishScore = Double.parseDouble(nums[2]);
double mathScore = Double.parseDouble(nums[3]);
double computerScore = Double.parseDouble(nums[4]);
Student stu1 = new Student(number,name,englishScore,mathScore,computerScore);