emp1 = Employee(10000, ‘John Doe’)
emp1.print_details()
Output:
10000 : John Doe
### 15使用self参数来维护对象的状态
class State(object):
def init(self):
self.field = 5.0
def add(self, x):
self.field += x
def mul(self, x):
self.field *= x
def div(self, x):
self.field /= x
def sub(self, x):
self.field -= x
s = State()
print(s.field)
s.add(2) # Self is implicitly passed.
print(s.field)
s.mul(2) # Self is implicitly passed.
print(s.field)
s.div(2) # Self is implicitly passed.
print(s.field)
s.sub(2) # Self is implicitly passed.
print(s.field)
Output:
5.0
7.0
14.0
7.0
5.0
### 16在 Python 中创建和使用静态类变量
class Employee:
age = 25
print(Employee.age)
e = Employee()
print(e.age)
e.age = 30
print(Employee.age) # 25
print(e.age) # 30
Output:
25
25
25
30
### 17在 Python 中的一个函数上使用多个装饰器
def my_decorator(func):
def wrapper():
print(“Step - 1”)
func()
print(“Step - 3”)
return wrapper
def repeat(func):
def wrapper():
func()
func()
func()
return wrapper
@my_decorator
@repeat
def start_steps():
print(“Step - 2”)
start_steps()
Output:
Step - 1
Step - 2
Step - 2
Step - 2
Step - 3
### 18在 Python 中的方法中同时访问 cls 和 self
class MyClass:
__var2 = ‘var2’
var3 = ‘var3’
def init(self):
self.__var1 = ‘var1’
def normal_method(self):
print(self.__var1)
@classmethod
def class_method(cls):
print(cls.__var2)
def my_method(self):
print(self.__var1)
print(self.__var2)
print(self.class.__var2)
if name == ‘main’:
print(MyClass.dict[‘var3’])
clzz = MyClass()
clzz.my_method()
Output:
var3
var1
var2
var2
### 19从装饰器访问实例方法的类
class Decorator(object):
def init(self, decoratee_enclosing_class):
self.decoratee_enclosing_class = decoratee_enclosing_class
def call(self, original_func):
def new_function(*args, **kwargs):
print('decorating function in ', self.decoratee_enclosing_class)
original_func(*args, **kwargs)
return new_function
class Bar(object):
@Decorator(‘Bar’)
def foo(self):
print(‘in foo’)
class Baz(object):
@Decorator(‘Baz’)
def foo(self):
print(‘in foo’)
print(‘before instantiating Bar()’)
b = Bar()
print(‘calling b.foo()’)
b.foo()
Output:
before instantiating Bar()
calling b.foo()
decorating function in Bar
in foo
### 20使用给定的装饰器获取 Python 类的所有方法
import inspect
def deco(func):
return func
def deco2():
def wrapper(func):
pass
return wrapper
class Test(object):
@deco
def method(self):
pass
@deco2()
def method2(self):
pass
def methodsWithDecorator(cls, decoratorName):
sourcelines = inspect.getsourcelines(cls)[0]
for i, line in enumerate(sourcelines):
line = line.strip()
if line.split(‘(’)[0].strip() == ‘@’ + decoratorName: # leaving a bit out
nextLine = sourcelines[i + 1]
name = nextLine.split(‘def’)[1].split(‘(’)[0].strip()
yield(name)
print(list(methodsWithDecorator(Test, ‘deco’)))
print(list(methodsWithDecorator(Test, ‘deco2’)))
Output:
[‘method’]
[‘method2’]
### 21装饰一个 class
from functools import wraps
def dec(msg=‘default’):
def decorator(klass):
old_foo = klass.foo
@wraps(klass.foo)
def decorated_foo(self, *args, **kwargs):
print(‘@decorator pre %s’ % msg)
old_foo(self, *args, **kwargs)
print(‘@decorator post %s’ % msg)
klass.foo = decorated_foo
return klass
return decorator
@dec(‘foo decorator’)
class Foo(object):
def foo(self, *args, **kwargs):
print(‘foo.foo()’)
@dec(‘subfoo decorator’)
class SubFoo(Foo):
def foo(self, *args, **kwargs):
print(‘subfoo.foo() pre’)
super(SubFoo, self).foo(*args, **kwargs)
print(‘subfoo.foo() post’)
@dec(‘subsubfoo decorator’)
class SubSubFoo(SubFoo):
def foo(self, *args, **kwargs):
print(‘subsubfoo.foo() pre’)
super(SubSubFoo, self).foo(*args, **kwargs)
print(‘subsubfoo.foo() post’)
SubSubFoo().foo()
Output:
@decorator pre subsubfoo decorator
subsubfoo.foo() pre
@decorator pre subfoo decorator
subfoo.foo() pre
@decorator pre foo decorator
foo.foo()
@decorator post foo decorator
subfoo.foo() post
@decorator post subfoo decorator
subsubfoo.foo() post
@decorator post subsubfoo decorator
### 22将类字段作为参数传递给类方法上的装饰器
import functools
# imagine this is at some different place and cannot be changed
def check_authorization(some_attr, url):
def decorator(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
print(f"Welcome Message: ‘{url}’…")
return func(*args, **kwargs)
return wrapper
return decorator
# another dummy function to make the example work
def do_work():
print(“work is done…”)
def custom_check_authorization(some_attr):
def decorator(func):
# assuming this will be used only on this particular class
@functools.wraps(func)
def wrapper(self, *args, **kwargs):
# get url
url = self.url
# decorate function with original decorator, pass url
return check_authorization(some_attr, url)(func)(self, *args, **kwargs)
return wrapper
return decorator
class Client(object):
def init(self, url):
self.url = url
@custom_check_authorization(“some_attr”)
def get(self):
do_work()
# create object
client = Client(‘Hello World’)
# call decorated function
client.get()
Output:
Welcome Message: ‘Hello World’…
work is done…
### 23在 Python 中创建多个传入参数列表的类变量
class Employee(object):
def init(self, **kwargs):
for key in kwargs:
setattr(self, key, kwargs[key])
emp = Employee(age=25, name=“John Doe”)
print(emp.age)
print(emp.name)
Output:
25
John Doe
### 24Python 中的 wraps 装饰器
from functools import wraps
def decorator_func_with_args(arg1, arg2):
def decorator(f):
@wraps(f)
def wrapper(*args, **kwargs):
print(“Before orginal function with decorator args:”, arg1, arg2)
result = f(*args, **kwargs)
print(“Ran after the orginal function”)
return result
return wrapper
return decorator
@decorator_func_with_args(“test1”, “test2”)
def hello(name):
“”“A function which prints a greeting to the name provided.
“””
print('Hello ', name)
return 25
print(“Starting script…”)
x = hello(‘John’)
print(“The value of x is:”, x)
print(“The wrapped functions docstring is:”, hello.doc)
print(“The wrapped functions name is:”, hello.name)
Output:
Starting script…
Before orginal function with decorator args: test1 test2
Hello John
Ran after the orginal function
The value of x is: 25
The wrapped functions docstring is: A function which prints a greeting to the name provided.
The wrapped functions name is: hello
### 25使用可选参数构建装饰器
def d(arg):
if callable(arg): # Assumes optional argument isn’t.
def newfn():
print(‘my default message’)
return arg()
return newfn
else:
def d2(fn):
def newfn():
print(arg)
return fn()
return newfn
return d2
@d(‘This is working’)
def hello():
print(‘hello world !’)
@d # No explicit arguments will result in default message.
def hello2():
print(‘hello2 world !’)
@d(‘Applying it twice’)
@d(‘Would also work’)
def hello3():
print(‘hello3 world !’)
hello()
hello2()
hello3()
Output:
This is working
hello world !
my default message
hello2 world !
Applying it twice
Would also work
hello3 world !
### 26在 Python 中将参数传递给装饰器
def decorator_maker_with_arguments(decorator_arg1, decorator_arg2, decorator_arg3):
def decorator(func):
def wrapper(function_arg1, function_arg2, function_arg3):
print(“The wrapper can access all the variables\n”
“\t- from the decorator maker: {0} {1} {2}\n”
“\t- from the function call: {3} {4} {5}\n”
“and pass them to the decorated function”
.format(decorator_arg1, decorator_arg2, decorator_arg3,
function_arg1, function_arg2, function_arg3))
return func(function_arg1, function_arg2, function_arg3)
return wrapper
return decorator
@decorator_maker_with_arguments(“canada”, “us”, “brazil”)
def decorated_function_with_arguments(function_arg1, function_arg2, function_arg3):
print(“This is the decorated function and it only knows about its arguments: {0}”
" {1}" " {2}".format(function_arg1, function_arg2, function_arg3))
decorated_function_with_arguments(“france”, “germany”, “uk”)
Output:
The wrapper can access all the variables
- from the decorator maker: canada us brazil
- from the function call: france germany uk
and pass them to the decorated function
This is the decorated function and it only knows about its arguments: france germany uk
### 27@property 装饰器
class Currency:
def init(self, dollars, cents):
self.total_cents = dollars * 100 + cents
@property
def dollars(self):
return self.total_cents // 100
@dollars.setter
def dollars(self, new_dollars):
self.total_cents = 100 * new_dollars + self.cents
@property
def cents(self):
return self.total_cents % 100
@cents.setter
def cents(self, new_cents):
self.total_cents = 100 * self.dollars + new_cents
currency = Currency(10, 20)
print(currency.dollars, currency.cents, currency.total_cents)
currency.dollars += 5
print(currency.dollars, currency.cents, currency.total_cents)
currency.cents += 15
print(currency.dollars, currency.cents, currency.total_cents)
Output:
10 20 1020
15 20 1520
15 35 1535
### 28类和函数的装饰器
from functools import wraps
def decorator(func):
@wraps(func)
def wrapper(*args, **kwargs):
print(‘sth to log: %s : %s’ % (func.name, args))
return func(*args, **kwargs)
return wrapper
class Class_test(object):
@decorator
def sum_func(self, a, b):
print(‘class sum: %s’ % (a + b))
return a + b
print(Class_test.sum_func(1, 5, 16))
Output:
sth to log: sum_func : (1, 5, 16)
class sum: 21
21
### 29Python 中带参数和返回值的装饰器
def calculation(func):
def wrapper(*args, **kwargs):
print(“Inside the calculation function”)
num_sum = func(*args, **kwargs)
print(“Before return from calculation function”)
return num_sum
return wrapper
@calculation
def addition(a, b):
print(“Inside the addition function”)
return a + b
print(“Sum =”, addition(5, 10))
Output:
Inside the calculation function
Inside the addition function
Before return from calculation function
Sum = 15
### 30Python 使用参数 wraps 装饰器
from functools import wraps
def decorator_func_with_args(arg1, arg2):
def decorator(f):
@wraps(f)
def wrapper(*args, **kwargs):
print(“Before orginal function with decorator args:”, arg1, arg2)
result = f(*args, **kwargs)
print(“Ran after the orginal function”)
return result
return wrapper
return decorator
@decorator_func_with_args(“test1”, “test2”)
def hello(name):
“”“A function which prints a greeting to the name provided.
“””
print('Hello ', name)
return 25
print(“Starting script…”)
x = hello(‘John’)
print(“The value of x is:”, x)
print(“The wrapped functions docstring is:”, hello.doc)
print(“The wrapped functions name is:”, hello.name)
Output:
Starting script…
Before orginal function with decorator args: test1 test2
Hello John
Ran after the orginal function
The value of x is: 25
The wrapped functions docstring is: A function which prints a greeting to the name provided.
The wrapped functions name is: hello
### 31Python 装饰器获取类名
def print_name(*args):
def _print_name(fn):
def wrapper(*args, **kwargs):
print(‘{}.{}’.format(fn.module, fn.qualname))
return fn(*args, **kwargs)
return wrapper
return _print_name
class A():
@print_name()
def a():
print(‘Hi from A.a’)
@print_name()
def b():
print(‘Hi from b’)
A.a()
b()
Output:
main.A.a
Hi from A.a
main.b
Hi from b
### 32简单装饰器示例
def my_decorator(func):
def wrapper():
print(“Step - 1”)
func()
print(“Step - 3”)
return wrapper
@my_decorator
def start_steps():
print(“Step - 2”)
start_steps()
Output:
Step - 1
Step - 2
Step - 3
### 33在 Python 中使用 print() 打印类的实例
class Element:
def init(self, name, city, population):
self.name = name
self.city = city
self.population = population
def str(self):
return str(self.class) + ‘\n’ + ‘\n’.join((‘{} = {}’.format(item, self.dict[item]) for item in self.dict))
elem = Element(‘canada’, ‘tokyo’, 321345)
print(elem)
Output:
name = canada
city = tokyo
population = 321345
### 34在 Python 中的类中将装饰器定义为方法
class myclass:
def init(self):
self.cnt = 0
def counter(self, function):
“”"
this method counts the number of runtime of a function
“”"
def wrapper(**args):
function(self, **args)
self.cnt += 1
print('Counter inside wrapper: ', self.cnt)
return wrapper
global counter_object
counter_object = myclass()
@counter_object.counter
def somefunc(self):
print(“Somefunc called”)
somefunc()
print(counter_object.cnt)
somefunc()
print(counter_object.cnt)
somefunc()
print(counter_object.cnt)
Output:
Somefunc called
Counter inside wrapper: 1
1
Somefunc called
Counter inside wrapper: 2
2
Somefunc called
Counter inside wrapper: 3
3
### 35获取在 Python 中修饰的给定类的所有方法
class awesome(object):
def init(self, method):
self._method = method
def call(self, obj, *args, **kwargs):
return self._method(obj, *args, **kwargs)
@classmethod
def methods(cls, subject):
def g():
for name in dir(subject):
method = getattr(subject, name)
if isinstance(method, awesome):
yield name, method
return {name: method for name, method in g()}
class Robot(object):
@awesome
def think(self):
return 0
@awesome
def walk(self):
return 0
def irritate(self, other):
return 0
print(awesome.methods(Robot))
Output:
{‘think’: <main.awesome object at 0x00000213C052AAC0>, ‘walk’: <main.awesome object at 0x00000213C0E33FA0>}
### 36带参数和不带参数的 Python 装饰器
def someDecorator(arg=None):
def decorator(func):
def wrapper(*a, **ka):
if not callable(arg):
print(arg)
return func(*a, **ka)
else:
return ‘xxxxx’
return wrapper
if callable(arg):
return decorator(arg) # return ‘wrapper’
else:
return decorator # … or ‘decorator’
@someDecorator(arg=1)
def my_func():
print(‘my_func’)
@someDecorator
def my_func1():
print(‘my_func1’)
if name == “main”:
my_func()
my_func1()
Output:
1
my_func
### 37Python 中带有 self 参数的类方法装饰器
def check_authorization(f):
def wrapper(*args):
print(‘Inside wrapper function argement passed :’, args[0].url)
return f(*args)
return wrapper
class Client(object):
def init(self, url):
self.url = url
@check_authorization
def get(self):
print(‘Inside get function argement passed :’, self.url)
Client(‘Canada’).get()
Output:
Inside wrapper function argement passed : Canada
Inside get function argement passed : Canada
### 38在 Python 中的另一个类中使用隐藏的装饰器
class TestA(object):
def _decorator(foo):
def magic(self):
print(“Start magic”)
foo(self)
print(“End magic”)
return magic
@_decorator
def bar(self):
print(“Normal call”)
_decorator = staticmethod(_decorator)
class TestB(TestA):
@TestA._decorator
def bar(self):
print(“Override bar in”)
super(TestB, self).bar()
print(“Override bar out”)
print(“Normal:”)
test = TestA()
test.bar()
print(‘-’ * 10)
print(“Inherited:”)
b = TestB()
b.bar()
Output:
Normal:
Start magic
Normal call
End magic
Inherited:
Start magic
Override bar in
Start magic
Normal call
End magic
Override bar out
End magic
### 39装饰器内部的 self 对象
import random
def only_registered_users(func):
def wrapper(handler):
print(‘Checking if user is logged in’)
if random.randint(0, 1):
print(‘User is logged in. Calling the original function.’)
func(handler)
else:
print(‘User is NOT logged in. Redirecting…’)
return wrapper
class MyHandler(object):
@only_registered_users
def get(self):
print(‘Get function called’)
m = MyHandler()
m.get()
Output:
Checking if user is logged in
User is logged in. Calling the original function.
Get function called
### 40在 Python 中将多个装饰器应用于单个函数
def multiplication(func):
def wrapper(*args, **kwargs):
num_sum = func(*args, **kwargs)
print(“Inside the multiplication function”, num_sum)
return num_sum * num_sum
return wrapper
def addition(func):
def wrapper(*args, **kwargs):
num_sum = func(*args, **kwargs)
print(“Inside the addition function”, num_sum)
return num_sum + num_sum
return wrapper
@addition
@multiplication
def calculation(a):
print(“Inside the calculation function”, a)
return a
print(“Sum =”, calculation(5))
Output:
Inside the calculation function 5
Inside the multiplication function 5
Inside the addition function 25
Sum = 50
### 41Python 装饰器获取类实例
class MySerial():
def init(self):
pass # I have to have an init
def write(self, *args):
print(args[0])
pass # write to buffer
def read(self):
pass # read to buffer
@staticmethod
def decorator(func):
def func_wrap(cls, *args, **kwargs):
cls.ser.write(func(cls, *args, **kwargs))
return cls.ser.read()
return func_wrap
class App():
def init(self):
self.ser = MySerial()
@MySerial.decorator
def myfunc(self):
self = 100
return [‘canada’, ‘australia’]
App().myfunc()
Output:
[‘canada’, ‘australia’]
### 42**init** 和 **call** 有什么区别
class Counter:
def init(self):
self._weights = []
for i in range(0, 2):
self._weights.append(1)
print(str(self._weights[-2]) + " No. from init")
def call(self, t):
self._weights = [self._weights[-1], self._weights[-1]
+ self._weights[-1]]
print(str(self._weights[-1]) + " No. from call")
num_count = Counter()
for i in range(0, 4):
num_count(i)
Output:
1 No. from init
2 No. from call
4 No. from call
8 No. from call
16 No. from call
### 43在 Python 中使用 **new** 和 **init**
class Shape:
def new(cls, sides, *args, **kwargs):
if sides == 3:
return Triangle(*args, **kwargs)
else:
return Square(args, **kwargs)
class Triangle:
def init(self, base, height):
self.base = base
self.height = height
def area(self):
return (self.base * self.height) / 2
class Square:
def init(self, length):
self.length = length
def area(self):
return self.lengthself.length
a = Shape(sides=3, base=2, height=12)
b = Shape(sides=4, length=2)
print(str(a.class))
print(a.area())
print(str(b.class))
print(b.area())
Output:
class ‘main.Triangle’
12.0
class ‘main.Square’
4
### 44Python 中的迭代重载方法
class Counter:
def init(self, low, high):
self.current = low
self.high = high
def iter(self):
return self
def next(self):
if self.current > self.high:
raise StopIteration
else:
self.current += 1
return self.current - 1
for num in Counter(5, 15):
print(num)
Output:
5
6
…
…
15
### 45在 Python 中使用迭代器反转字符串
class Reverse:
def init(self, data):
self.data = data
self.index = len(data)
def iter(self):
return self
def next(self):
if self.index == 0:
raise StopIteration
self.index = self.index - 1
return self.data[self.index]
test = Reverse(‘Python’)
for char in test:
print(char)
Output:
n
o
h
t
y
P
### 46Python 中 **reversed** 魔术方法
class Count:
def init(self, start, end):
self.start = start
self.end = end
self.current = None
def iter(self):
self.current = self.start
while self.current < self.end:
yield self.current
self.current += 1
def next(self):
if self.current is None:
self.current = self.start
if self.current > self.end:
raise StopIteration
else:
self.current += 1
return self.current-1
def reversed(self):
self.current = self.end
while self.current >= self.start:
yield self.current
self.current -= 1
obj1 = Count(0, 5)
for i in obj1:
print(i)
obj2 = reversed(obj1)
for i in obj2:
print(i)
Output:
0
1
2
…
2
1
0
### 47Python 中的 **getitem** 和 **setitem**
class Counter(object):
def init(self, floors):
self._floors = [None]*floors
def setitem(self, floor_number, data):
self._floors[floor_number] = data
def getitem(self, floor_number):
return self._floors[floor_number]
index = Counter(4)
index[0] = ‘ABCD’
index[1] = ‘EFGH’
index[2] = ‘IJKL’
index[3] = ‘MNOP’
print(index[2])
Output:
IJKL
### 48在 Python 中使用 **getattr** 和 **setattr** 进行属性赋值
class Employee(object):
def init(self, data):
super().setattr(‘data’, dict())
self.data = data
def getattr(self, name):
if name in self.data:
return self.data[name]
else:
return 0
def setattr(self, key, value):
if key in self.data:
self.data[key] = value
else:
super().setattr(key, value)
emp = Employee({‘age’: 23, ‘name’: ‘John’})
print(emp.age)
print(emp.name)
print(emp.data)
print(emp.salary)
emp.salary = 50000
print(emp.salary)
Output:
23
John
{‘age’: 23, ‘name’: ‘John’}
0
50000
### 49什么是 **del** 方法以及如何调用它
class Employee():
def init(self, name=‘John Doe’):
print('Hello ’ + name)
self.name = name
def developer(self):
print(self.name)
def del(self):
print('Good Bye ’ + self.name)
emp = Employee(‘Mark’)
print(emp)
emp = ‘Rocky’
print(emp)
Output:
Hello Mark
<main.Employee object at 0x00000000012498D0>
Good Bye Mark
Rocky
### 50创建类的私有成员
class Test(object):
__private_var = 100
public_var = 200
def __private_func(self):
print(‘Private Function’)
def public_func(self):
print(‘Public Function’)
print(self.public_var)
def call_private(self):
self.__private_func()
print(self.__private_var)
t = Test()
print(t.call_private())
print(t.public_func())
Output:
Private Function
100
None
Public Function
200
None
### 51一个 Python 封装的例子
class Encapsulation:
__name = None
def init(self, name):
self.__name = name
def get_name(self):
return self.__name
pobj = Encapsulation(‘Rocky’)
print(pobj.get_name())
Output:
Rocky
### 52一个 Python 组合的例子
class Salary:
def init(self, pay):
self.pay = pay
def get_total(self):
return (self.pay*12)
class Employee:
def init(self, pay, bonus):
self.pay = pay
self.bonus = bonus
self.obj_salary = Salary(self.pay)
def annual_salary(self):
return "Total: " + str(self.obj_salary.get_total() + self.bonus)
obj_emp = Employee(600, 500)
print(obj_emp.annual_salary())
Output:
Total: 7700
### 53一个Python聚合的例子
class Salary:
def init(self, pay):
self.pay = pay
def get_total(self):
return (self.pay*12)
class Employee:
def init(self, pay, bonus):
self.pay = pay
self.bonus = bonus
def annual_salary(self):
return "Total: " + str(self.pay.get_total() + self.bonus)
obj_sal = Salary(600)
obj_emp = Employee(obj_sal, 500)
print(obj_emp.annual_salary())
Output:
Total: 7700
### 54Python 中的单级、多级和多级继承
# Single inheritence
class Apple:
manufacturer = ‘Apple Inc’
contact_website = ‘www.apple.com/contact’
name = ‘Apple’
def contact_details(self):
print('Contact us at ', self.contact_website)
class MacBook(Apple):
def init(self):
self.year_of_manufacture = 2018
def manufacture_details(self):
print(‘This MacBook was manufactured in {0}, by {1}.’
.format(self.year_of_manufacture, self.manufacturer))
macbook = MacBook()
macbook.manufacture_details()
# Multiple inheritence
class OperatingSystem:
multitasking = True
name = ‘Mac OS’
class MacTower(OperatingSystem, Apple):
def init(self):
if self.multitasking is True:
print(‘Multitasking system’)
# if there are two superclasses with the sae attribute name
# the attribute of the first inherited superclass will be called
# the order of inhertence matters
print(‘Name: {}’.format(self.name))
mactower = MacTower()
# Multilevel inheritence
class MusicalInstrument:
num_of_major_keys = 12
class StringInstrument(MusicalInstrument):
type_of_wood = ‘Tonewood’
class Guitar(StringInstrument):
def init(self):
self.num_of_strings = 6
print(‘The guitar consists of {0} strings,’ +
‘it is made of {1} and can play {2} keys.’
.format(self.num_of_strings,
self.type_of_wood, self.num_of_major_keys))
guitar = Guitar()
Output:
This MacBook was manufactured in 2018, by Apple Inc.
Multitasking system
Name: Mac OS
The guitar consists of 6 strings, it is made of Tonewood and can play 12 keys.
### 55在 Python 中获取一个类的父类
class A(object):
pass
class B(object):
pass
class C(A, B):
pass
print(C.bases)
Output:
(< class ‘main.A’ >, < class ‘main.B’ >)
### 56Python 中的多态性
# Creating a shape Class
class Shape:
width = 0
height = 0
# Creating area method
def area(self):
print("Parent class Area … ")
# Creating a Rectangle Class
class Rectangle(Shape):
def init(self, w, h):
self.width = w
self.height = h
# Overridding area method
def area(self):
print("Area of the Rectangle is : ", self.widthself.height)
# Creating a Triangle Class
class Triangle(Shape):
def init(self, w, h):
self.width = w
self.height = h
# Overridding area method
def area(self):
print("Area of the Triangle is : ", (self.widthself.height)/2)
rectangle = Rectangle(10, 20)
triangle = Triangle(2, 10)
rectangle.area()
triangle.area()
Output:
如果你也是看准了Python,想自学Python,在这里为大家准备了丰厚的免费**学习**大礼包,带大家一起学习,给大家剖析Python兼职、就业行情前景的这些事儿。
### 一、Python所有方向的学习路线
Python所有方向路线就是把Python常用的技术点做整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
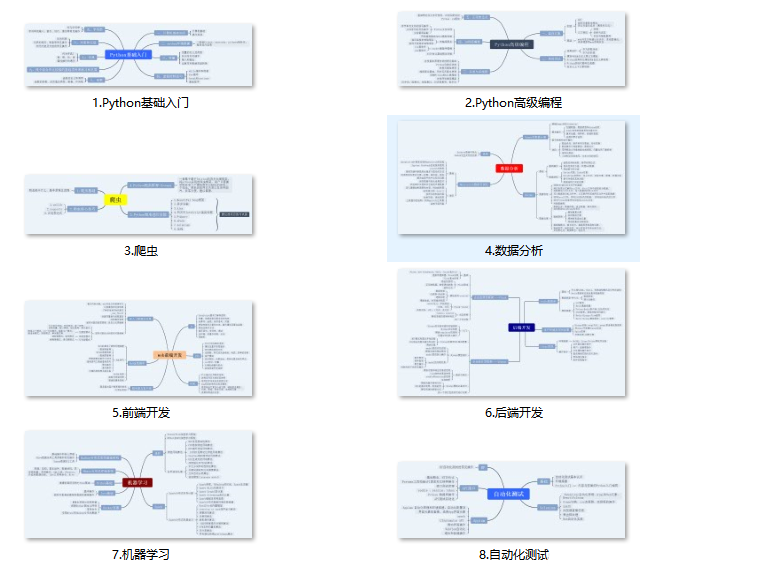
### 二、学习软件
工欲善其必先利其器。学习Python常用的开发软件都在这里了,给大家节省了很多时间。
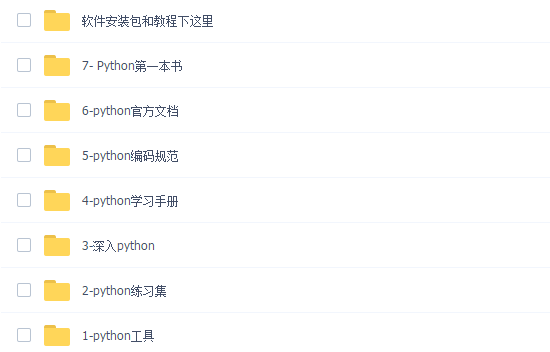
### 三、全套PDF电子书
书籍的好处就在于权威和体系健全,刚开始学习的时候你可以只看视频或者听某个人讲课,但等你学完之后,你觉得你掌握了,这时候建议还是得去看一下书籍,看权威技术书籍也是每个程序员必经之路。
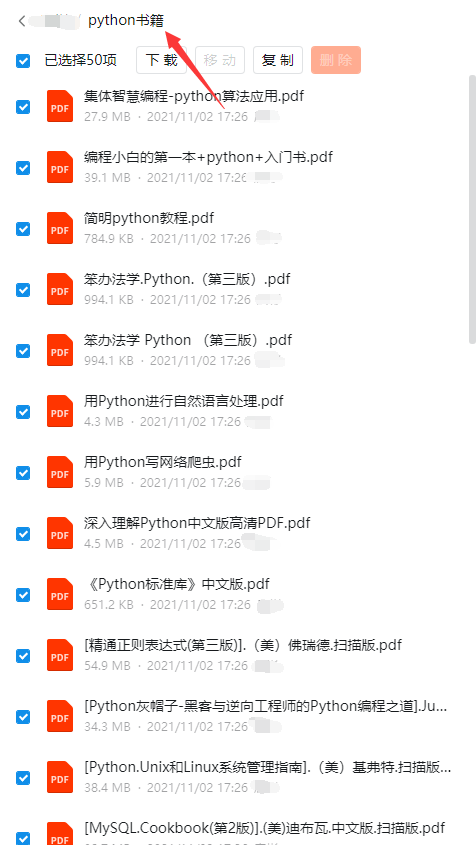
### 四、入门学习视频
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
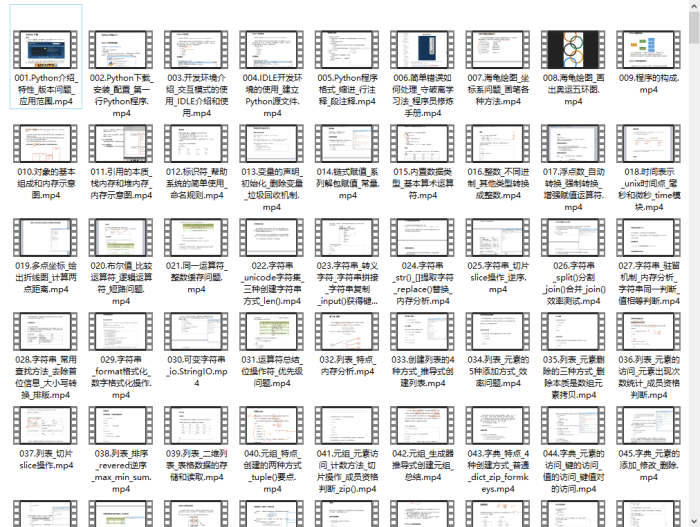
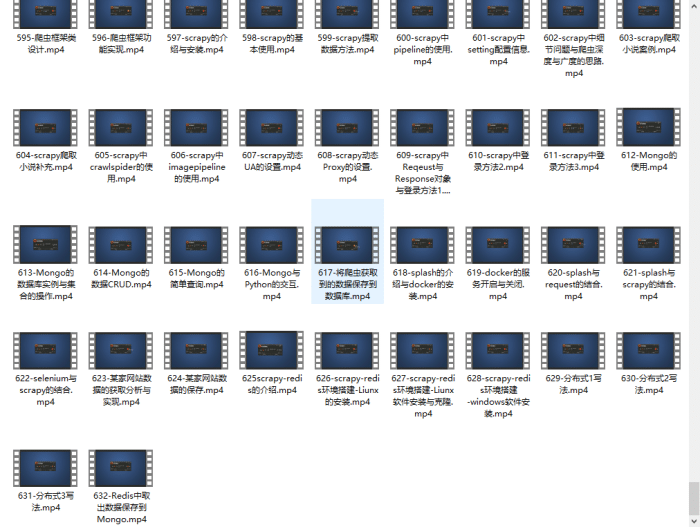
### 四、实战案例
光学理论是没用的,要学会跟着一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
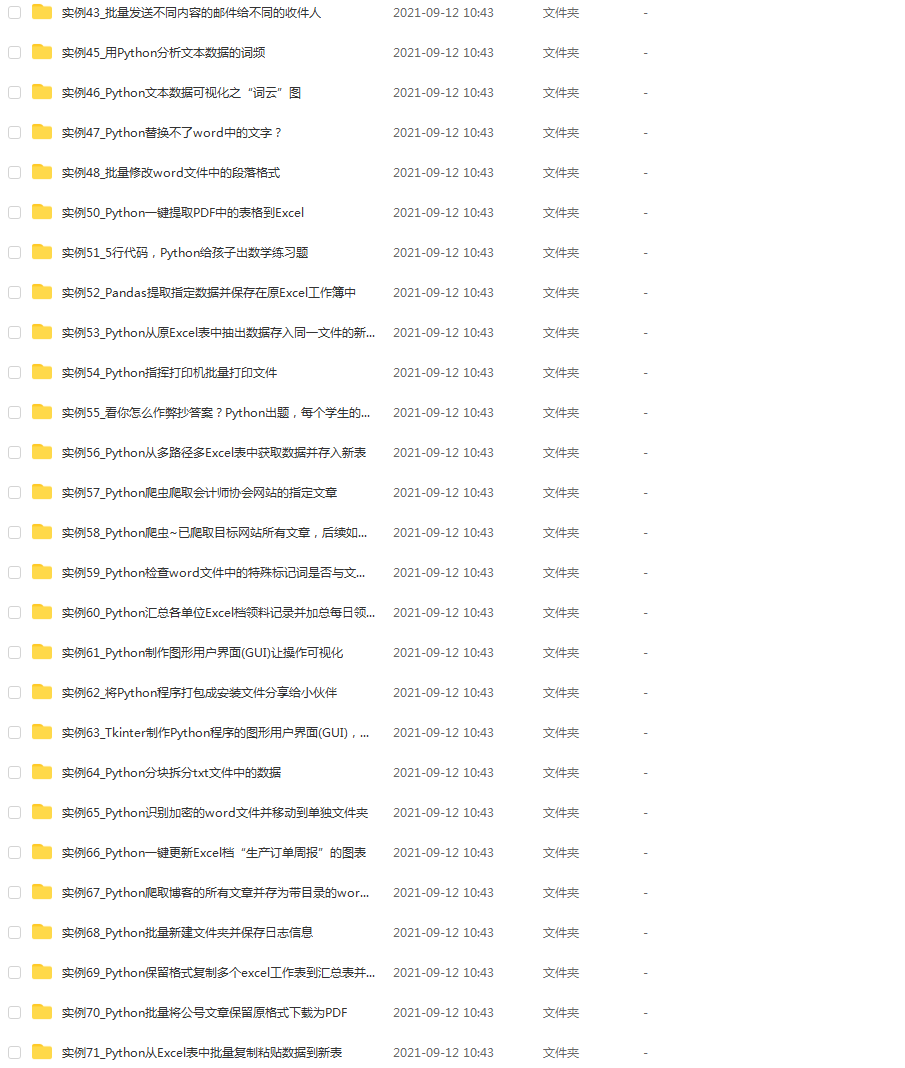
### 五、面试资料
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
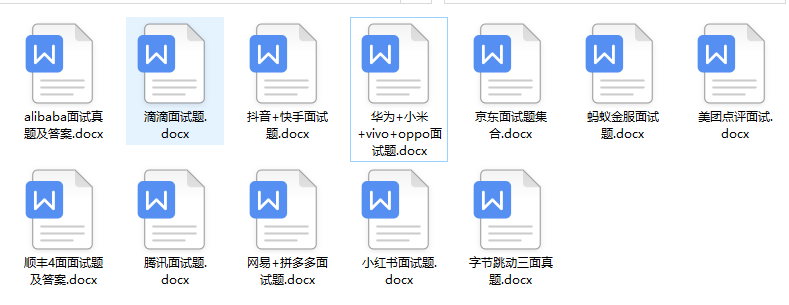
成为一个Python程序员专家或许需要花费数年时间,但是打下坚实的基础只要几周就可以,如果你按照我提供的学习路线以及资料有意识地去实践,你就有很大可能成功!
最后祝你好运!!!
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里无偿获取](https://bbs.csdn.net/topics/618317507)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**