一、什么是设计模式
设计模式就是前人代码设计经验的总结,会使你的代码更加稳定,拓展性更强。是一系列的编程思想。
设计模式有23种。
二、什么是工厂模式
工厂模式是最常用的设计模式之一。这种类型的设计模式属于创建型模式,它提供了一种创建对象的最佳方式。
在工厂模式中,我们在创建对象时不会对客户端暴露创建逻辑,并且是通过使用一个共同的接口来指向新创建的对象。
对我来说,工厂模式类似于分文件编程,就是在主函数调用各种文件函数。
三、举例实现
mainpro.c
#include "animal.h"
#include <string.h>
struct Animal* findUtilByName(char *str,struct Animal *phead)
{
struct Animal *tmp = phead;
if(phead == NULL){
printf("error in link\n");
return NULL;
}else{
while (tmp != NULL)
{
if(strcmp(tmp->name,str) == 0)
{
return tmp;
}
tmp=tmp->next;
}
return NULL;
}
}
int main()
{
char buf[128] = {'\0'};
struct Animal *phead = NULL;
struct Animal *ptmp;
phead = putCatlnLink(phead);
phead = putDoglnLink(phead);
phead = putPersonlnLink(phead);
while(1)
{
printf("input:Tom,huang,xiaoyu\n");
scanf("%s",buf);
ptmp = findUtilByName(buf,phead);
if(ptmp != NULL){
ptmp->pbeat();
ptmp->peat();
}
memset(buf,'\0',sizeof(buf));
}
return 0;
}
cat.c
#include "animal.h"
void catEat()
{
printf("cat eat fish\n");
}
void catBeat()
{
printf("cat beat\n");
}
struct Animal cat = {
.name = "Tom",
.peat = catEat,
.pbeat = catBeat
};
struct Animal* putCatlnLink(struct Animal *phead)
{
if(phead == NULL){
phead = &cat;
return phead;
}else{
cat.next = phead;
phead = &cat;
return phead;
}
}
dog.c
#include "animal.h"
void dogEat()
{
printf("dog eat shit\n");
}
void dogBeat()
{
printf("dog bite\n");
}
struct Animal dog = {
.name = "huang",
.peat = dogEat,
.pbeat = dogBeat
};
struct Animal* putDoglnLink(struct Animal *phead)
{
if(phead == NULL){
phead = &dog;
return phead;
}else{
dog.next = phead;
phead = &dog;
return phead;
}
}
person.c
#include "animal.h"
void personEat()
{
printf("person eat rice\n");
}
void personBeat()
{
printf("person hit\n");
}
struct Animal person = {
.name = "xiaoyu",
.peat = personEat,
.pbeat = personBeat
};
struct Animal* putPersonlnLink(struct Animal *phead)
{
if(phead == NULL){
phead = &person;
return phead;
}else{
person.next = phead;
phead = &person;
return phead;
}
}
animal.h
#include <stdio.h>
struct Animal
{
char name[128];
int age;
int sex;
int other;
void (*peat)();
void (*pbeat)();
void (*test)();
struct Animal *next;
};
struct Animal* putCatlnLink(struct Animal *phead);
struct Animal* putDoglnLink(struct Animal *phead);
struct Animal* putPersonlnLink(struct Animal *phead);
主要的思路讲解,各种的动物之间分别组成一个工厂,这个工厂里面有一个链表组成,猫、狗、人等等的各种动物组成一个链表
我们明显可以看到,现在的是多文件编程,不像以前什么东西都是写进一个main函数里面,多个动物组成一个链表,一个动物一个文件,互不影响与干扰,这就是工厂模式的好处。
四、代码验证
我们在unubtu编译看看。
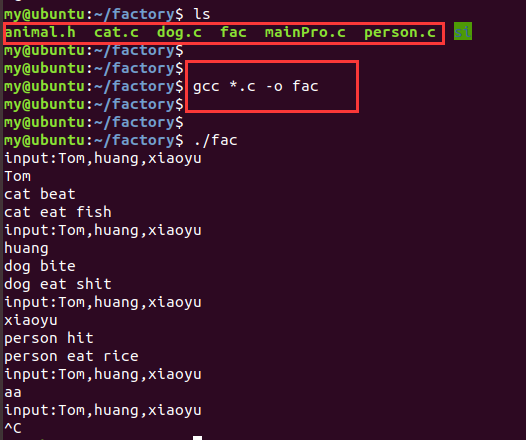
*.c 的意思是所有.c文件的意思,gcc *.c -o fac是编译此目录底下的所有.c文件的意思
学习笔记,仅供参考