题目:
给你一个 无重复元素 的整数数组 candidates 和一个目标整数 target ,找出 candidates 中可以使数字和为目标数 target 的 所有 不同组合 ,并以列表形式返回。你可以按 任意顺序 返回这些组合。
candidates 中的 同一个 数字可以 无限制重复被选取 。如果至少一个数字的被选数量不同,则两种组合是不同的。
对于给定的输入,保证和为 target 的不同组合数少于 150 个。
示例 1:
输入:candidates = [2,3,6,7], target = 7输出:[[2,2,3],[7]]
解释:
2 和 3 可以形成一组候选,2 + 2 + 3 = 7 。注意 2 可以使用多次。
7 也是一个候选, 7 = 7 。
仅有这两种组合。
和leetcode77.组合不同的是,这道题允许一个组合中出现重复的数字,其他并无很大差别
class Solution {
List<List<Integer>> result = new ArrayList<List<Integer>>();
List<Integer> list = new ArrayList<Integer>();
public List<List<Integer>> combinationSum(int[] candidates, int target) {
backtracking(candidates,target,0,0);
return result;
}
public void backtracking(int [] candidates,int target,int sum,int startIndex){
if(sum > target){
//直接结束递归
return;
}
if(sum == target){
//将list集合添加到result中
result.add(new ArrayList<Integer>(list));
//结束递归
return;
}
//利用for循环横向遍历,startIndex用来动态控制每一次执行递归函数时for的第一次起始位置
for(int i = startIndex; i < candidates.length; i++){
//把candidates[i]存到list中
list.add(candidates[i]);
//递归纵向遍历
backtracking(candidates,target,sum + candidates[i],i);
//回溯,删掉list中最后一个数字,为下一组数据腾位置
list.remove(list.size() - 1);
}
}
}
图来自代码随想录:
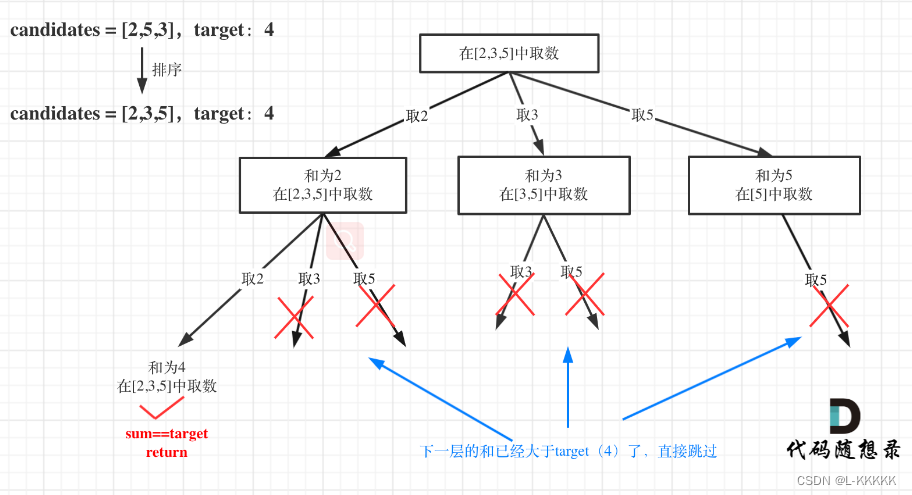
对上述代码剪枝:
通过上图我们可以看到,当当前层的sum + canidates[i]已经大于target,那么我们就直接跳过该循环,不过这样做的前提是首先要对数组candidates做升序排序
代码如下:
class Solution {
List<List<Integer>> result = new ArrayList<List<Integer>>();
List<Integer> list = new ArrayList<Integer>();
public List<List<Integer>> combinationSum(int[] candidates, int target) {
//对candidates进行升序
sort(candidates);
backtracking(candidates,target,0,0);
return result;
}
public void backtracking(int [] candidates,int target,int sum,int startIndex){
if(sum > target){
//直接结束递归
return;
}
if(sum == target){
//将list集合添加到result中
result.add(new ArrayList<Integer>(list));
//结束递归
return;
}
//利用for循环横向遍历,startIndex用来动态控制每一次执行递归函数时for的第一次起始位置。
//在for循环出进行剪枝
for(int i = startIndex; i < candidates.length && sum + candidates[i] <= target; i++){
//把candidates[i]存到list中
list.add(candidates[i]);
//递归纵向遍历
backtracking(candidates,target,sum + candidates[i],i);
//回溯,删掉list中最后一个数字,为下一组数据腾位置
list.remove(list.size() - 1);
}
}
public void sort(int[] candidates){
for(int i = 0 ; i < candidates.length - 1; i++){
for(int j = 0; j < candidates.length - i - 1; j++){
if(candidates[j] > candidates[j + 1]){
int temp = candidates[j];
candidates[j] = candidates[j + 1];
candidates[j + 1] = temp;
}
}
}
}
}