.Send.c:#include <sys/msg.h>#include <sys/types.h>#include <sys/ipc.h>#include <stdio.h>#include <stdlib.h>#include <string.h>#define msg_key 114 struct msg{ /* 声明消息结构体 */ long msg_types; /* 消息类型成员 */ char msg_buf[511]; /* 消息 */};int main ( void ){ int qid; int pid; key_t key; key = msg_key; char text[500]={0}; struct msg pmsg; /* 一个消息的结构体变量 */ pmsg.msg_types = getpid(); /* 消息类型为当前进程的 ID*/ printf( "This is a write_process\n"); qid = msgget(msg_key,IPC_CREAT|0666);/*创建消息队列*/ if(qid <0){ perror ( "failed to create msq" ); exit (1) ; } printf ("successfully get a queue: %d \n", qid); while(1){ scanf("%s",text); strcpy(pmsg.msg_buf,text ); if ( (msgsnd(qid, &pmsg, sizeof(struct msg)-sizeof(long), 0 )) < 0 ){ /* 向消息队列中发送消息 */ perror ( "msgsn" ); exit ( 1 ); } if(strcmp(text,"end") == 0) break; } exit ( 0 ) ; }Recv.c:#include <sys/msg.h>#include <sys/types.h>#include <sys/ipc.h>#include <stdio.h>#include <stdlib.h>#define msg_key 114struct msg{ /* 声明消息结构体 */ long msg_types; /* 消息类型成员 */ char msg_buf[511]; /* 消息 */};int main() { int qid; int len; char text[500]; struct msg pmsg; /* 从指定队列读取消息 */ while(1){ qid = msgget(msg_key,IPC_EXCL );/*检查消息队列是否存在 */ while(qid < 0){ printf("msq not existed!\n"); sleep(1); } msgrcv ( qid, &pmsg, sizeof(struct msg)-sizeof(long), 0, 0 ); printf("%s\n",pmsg.msg_buf); if(strcmp(pmsg.msg_buf,"end") == 0) break; } exit ( 0 ) ;}2.Client.c:#include <sys/msg.h>#include <sys/types.h>#include <sys/ipc.h>#include <stdio.h>#include <stdlib.h>#include <string.h>#define client_key 114 #define server_key 514struct msg{ /* 声明消息结构体 */ long msg_types; /* 消息类型成员 */ char msg_buf[511]; /* 消息 */};int main ( void ){ //创建消息队列 int qid, qqid; int pid; char text[500]={0}; struct msg pmsg; /* 一个消息的结构体变量 */ pmsg.msg_types = getpid(); /* 消息类型为当前进程的 ID*/ printf( "This is a client_process\nenter \"end\" to close.\n"); qid = msgget(client_key,IPC_CREAT|0666);/*创建消息队列*/ if(qid <0){ perror ( "failed to create msq" ); exit (1) ; } printf ("successfully get a queue: %d \n", qid); while(1){ scanf("%s",text); strcpy(pmsg.msg_buf,text ); if ( (msgsnd(qid, &pmsg, sizeof(struct msg)-sizeof(long), 0 )) < 0 ){ /* 向消息队列中发送消息 */ perror ( "msgsn" ); exit ( 1 ); } if(strcmp(text,"end") == 0) break; qqid = msgget(server_key,IPC_EXCL );/*检查消息队列是否存在 */ while(qqid < 0){ printf("wait..."); qqid = msgget(server_key,IPC_EXCL ); sleep(1); } msgrcv ( qqid, &pmsg, sizeof(struct msg)-sizeof(long), 0, 0 ); printf("%s=%s\n", text, pmsg.msg_buf); } exit ( 0 ) ; }Server.c:#include <sys/msg.h>#include <sys/types.h>#include <sys/ipc.h>#include <stdio.h>#include <stdlib.h>#include <string.h>#define client_key 114#define server_key 514 struct msg{ /* 声明消息结构体 */ long msg_types; /* 消息类型成员 */ char msg_buf[511]; /* 消息 */};int main() { int qid,qqid; int len; int num1 = 0,num2 = 0,i = 0; char a; char text[511],result[10]={}; struct msg pmsg; printf( "This is a server_process\n"); qqid = msgget(server_key,IPC_CREAT|0666);/*创建消息队列*/ if(qqid <0){ perror ( "failed to create msq" ); exit (1) ; } printf ("successfully get a queue: %d \n", qqid); /* 从指定队列读取消息 */ while(1){ qid = msgget(client_key,IPC_EXCL );/*检查消息队列是否存在 */ while(qid < 0){ printf("wait..."); qid = msgget(client_key,IPC_EXCL ); sleep(1); } msgrcv ( qid, &pmsg, sizeof(struct msg)-sizeof(long), 0, 0 ); //printf("%s=\n",pmsg.msg_buf); if(strcmp(pmsg.msg_buf,"end") == 0) break; strcpy(text,pmsg.msg_buf); printf("receive: %s\n",text); while(text[i] >= '0' && text[i] <= '9') num1 = num1 * 10 + (text[i++]-'0'); a = text[i++]; while(text[i] != '\0' && text[i] >= '0' && text[i] <= '9') num2 = num2 * 10 + (text[i++]-'0'); if('+' == a) sprintf(pmsg.msg_buf,"%d",num1 + num2); else if('-' == a) sprintf(pmsg.msg_buf,"%d",num1 - num2); else if('*' == a) sprintf(pmsg.msg_buf,"%d",num1 * num2); else if('/' == a) sprintf(pmsg.msg_buf,"%f",(double)num1 / (double)num2); else sprintf(pmsg.msg_buf,"%s","error..."); printf("send: %s\n",pmsg.msg_buf); msgsnd(qqid, &pmsg, sizeof(struct msg)-sizeof(long), 0 ); num1 = 0; num2 = 0; i=0; } exit ( 0 ) ;}
操作系统作业
最新推荐文章于 2024-09-07 11:53:47 发布
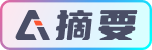